Why You Forget Basic Concepts During Coding Interviews (and How to Overcome It)
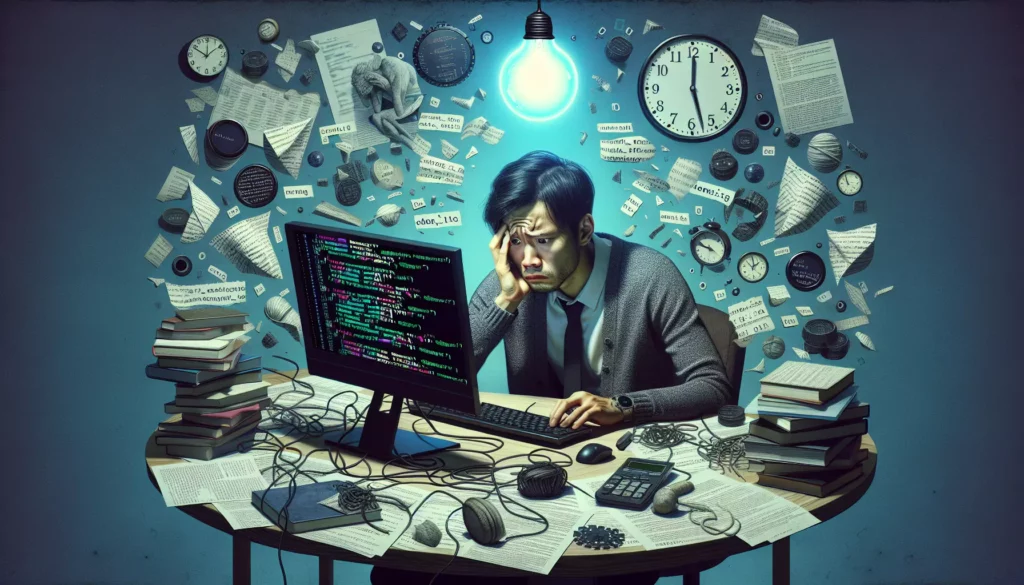
Have you ever been in a coding interview, faced with a seemingly straightforward problem, only to find your mind going completely blank? That sinking feeling when basic concepts you’ve known for years suddenly become inaccessible is not just frustrating—it’s surprisingly common. Even experienced developers regularly report “forgetting” fundamental knowledge during high-pressure interview situations.
In this comprehensive guide, we’ll explore why this phenomenon occurs and provide actionable strategies to overcome interview amnesia. Whether you’re preparing for your first technical interview or your fifteenth, understanding the psychological mechanisms behind interview performance can dramatically improve your outcomes.
Table of Contents
- Why We Forget: The Science Behind Interview Amnesia
- Most Commonly Forgotten Concepts in Coding Interviews
- Prevention Strategies: Before the Interview
- Recovery Techniques: During the Interview
- Effective Practice Methods to Build Lasting Knowledge
- Mindset Shifts for Interview Success
- Case Studies: How Successful Candidates Overcome Forgetting
- Conclusion: Building Resilient Knowledge
Why We Forget: The Science Behind Interview Amnesia
Forgetting during interviews isn’t a sign of incompetence—it’s your brain responding to stress in predictable ways. Understanding these mechanisms is the first step toward counteracting them.
The Stress Response and Cognitive Function
When you enter a high-stakes interview environment, your body often triggers what’s known as the “fight or flight” response. This evolutionary mechanism floods your system with stress hormones like cortisol and adrenaline, which can:
- Reduce blood flow to the prefrontal cortex (responsible for complex thinking)
- Impair working memory function
- Narrow cognitive focus (sometimes called “tunnel vision”)
- Disrupt retrieval pathways to stored knowledge
Dr. Sian Beilock, cognitive scientist and author of “Choke: What the Secrets of the Brain Reveal About Getting It Right When You Have To,” explains that “pressure-filled situations deplete a part of the brain’s processing resources… resources that are used for tasks like analytical reasoning and problem-solving.”
Working Memory Overload
Working memory—our mental “workspace” that holds information temporarily available for processing—has limited capacity. During interviews, this capacity gets taxed by multiple demands:
- Processing the interview question
- Recalling relevant concepts and algorithms
- Monitoring the interviewer’s reactions
- Managing performance anxiety
- Tracking time constraints
When these cognitive demands exceed working memory capacity, retrieval failures occur even for well-learned material.
Context-Dependent Memory
Another fascinating aspect of memory is its context-dependent nature. We often learn programming concepts in comfortable, low-pressure environments (like our home, office, or classroom). The interview context is radically different, creating what psychologists call a “context mismatch” that can impair recall.
Research has consistently shown that information is more easily recalled when the retrieval context matches the learning context. This explains why concepts that seem crystal clear when you’re practicing at home can become elusive in the unfamiliar interview setting.
Most Commonly Forgotten Concepts in Coding Interviews
Certain programming concepts and algorithms seem particularly vulnerable to being forgotten under pressure. Recognizing these “high-risk” topics allows you to give them special attention during preparation.
Algorithm Fundamentals
- Recursion logic and base cases: The recursive approach often “makes sense” during study but becomes confusing under pressure
- Dynamic programming: The subtleties of memoization and tabulation approaches
- Graph traversal algorithms: The specific steps of BFS vs. DFS and their implementations
- Binary search edge cases: Handling the boundary conditions correctly
Data Structure Operations
- Hash table implementation details: Collision handling and load factor considerations
- Tree balancing operations: Rotations in AVL or Red-Black trees
- Heap operations: The bubble-up and bubble-down procedures
- Linked list manipulation: Pointer updates for insertion and deletion
Language-Specific Details
- Syntax for less-common operations: Like bit manipulation or advanced string methods
- Standard library function parameters: The exact order and types of arguments
- Exception handling patterns: Proper try/catch/finally structure
- Memory management details: Especially in languages like C++ or Rust
One particularly common interview scenario involves forgetting the exact syntax for initializing data structures. Here’s an example of a common initialization pattern in Python that candidates often struggle to recall under pressure:
# Creating an adjacency list representation of a graph
graph = collections.defaultdict(list)
# Creating a 2D matrix with specific dimensions
dp = [[0 for _ in range(cols)] for _ in range(rows)]
# Initializing a counter for character frequencies
counter = collections.Counter(string)
Prevention Strategies: Before the Interview
The best way to combat interview amnesia is to prepare in ways that make your knowledge more resilient to stress. These strategies focus on strengthening retrieval pathways and making recall more automatic.
Spaced Repetition Systems
Spaced repetition leverages the psychological spacing effect to maximize long-term retention. Instead of cramming all your review into a single session, space your practice over time with increasing intervals between reviews.
- Digital tools: Applications like Anki, RemNote, or SuperMemo can help implement spaced repetition for programming concepts
- Creating effective flashcards: Focus on active recall by putting the question on the front (“How does quicksort work?”) and the answer on the back
- Optimal scheduling: Review items just as you’re about to forget them to strengthen memory traces
A sample spaced repetition schedule might look like this:
- Initial learning: Day 1
- First review: Day 2 (1-day interval)
- Second review: Day 4 (2-day interval)
- Third review: Day 8 (4-day interval)
- Fourth review: Day 16 (8-day interval)
Deliberate Practice Under Pressure
To overcome context-dependent memory limitations, gradually introduce stress elements into your practice sessions:
- Timed practice: Set strict time limits that are slightly shorter than you’d have in a real interview
- Mock interviews: Practice with friends, mentors, or through platforms like Pramp or interviewing.io
- Distraction training: Practice while dealing with minor distractions to build focus resilience
- Standing practice: Some companies conduct interviews at whiteboards, so practice coding while standing
Concept Mapping and Knowledge Integration
Isolated facts are more vulnerable to forgetting than connected knowledge. Build robust mental models by:
- Creating concept maps: Visually connect related programming concepts
- Teaching others: Explaining concepts forces you to organize and integrate your knowledge
- Comparing and contrasting: Explicitly note similarities and differences between related algorithms or data structures
- Building a “knowledge tree”: Organize concepts hierarchically from fundamentals to advanced applications
For example, a concept map for sorting algorithms might connect various methods based on their approach (divide-and-conquer, comparison-based, etc.), time complexity, space requirements, and stability characteristics.
Creating Cheat Sheets
The process of creating concise reference materials enhances memory, even if you never use them during the interview:
- Algorithm templates: Create skeleton implementations of common algorithms
- Time/space complexity charts: Summarize the efficiency of different data structures and algorithms
- Language-specific syntax guides: Focus on easily confused or rarely used features
Here’s what a section of a personal cheat sheet might look like for graph algorithms:
# BFS Template (Finding shortest path in unweighted graph)
from collections import deque
def bfs(graph, start, target):
queue = deque([start])
visited = set([start])
while queue:
node = queue.popleft()
if node == target:
return True # Found the target
for neighbor in graph[node]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
return False # Target not found
Recovery Techniques: During the Interview
Even with thorough preparation, you might experience moments of forgetfulness during an interview. These techniques can help you recover quickly and effectively.
Structured Problem Solving as Memory Aid
Following a consistent problem-solving framework not only impresses interviewers but also helps jog your memory:
- Clarify the problem: Asking questions about inputs, outputs, and edge cases often triggers relevant concept recall
- Work through examples: Manual tracing of small examples can remind you of algorithm patterns
- Consider approaches systematically: Methodically evaluating data structures and algorithms often surfaces the solution
- Plan before coding: Writing pseudocode activates procedural memory
Verbalization Techniques
Explaining your thought process aloud has multiple benefits:
- It engages different neural pathways than silent thinking, potentially unlocking blocked memories
- It slows your thinking, reducing cognitive overload
- It demonstrates your problem-solving ability even when stuck
- It invites helpful prompts from the interviewer
Practice verbalizing with phrases like: “I’m considering using a hash map here because…” or “I’m trying to recall the exact approach for handling this edge case…”
Graceful Recovery When Blanking
When you truly can’t remember a concept, these approaches can help:
- Work from first principles: Derive the solution from basic concepts you do remember
- Use analogies: “This reminds me of problem X, where we used approach Y…”
- Be honest but constructive: “I’m having trouble recalling the exact implementation of heap’s bubble-up operation. Let me work through the logic…”
- Ask targeted questions: “Would a breadth-first or depth-first approach be more appropriate here?”
Stress Management in the Moment
Physiological techniques can help reduce stress and improve cognitive function:
- Controlled breathing: Take a few deep breaths (4 seconds in, 4 seconds out) to reduce cortisol levels
- Brief mindfulness: Spend 10 seconds focusing on sensory input to break rumination cycles
- Positive self-talk: Replace “I’m blanking” with “I’m working through this step by step”
- Physical adjustments: Uncross your arms, sit up straight, or stand to change your physiological state
Effective Practice Methods to Build Lasting Knowledge
Not all practice is equally effective at building stress-resistant knowledge. These methods leverage cognitive science principles to maximize retention and recall.
Interleaved Practice
Rather than mastering one topic before moving to the next (blocked practice), interleaving involves mixing different problem types in a single session:
- Why it works: Creates stronger retrieval cues and improves discrimination between concepts
- Implementation: Mix tree problems with array problems, or dynamic programming with graph algorithms
- Caution: May feel more difficult initially, but leads to better long-term retention
A sample interleaved practice session might include:
- A binary tree traversal problem
- A string manipulation problem
- A graph search problem
- A dynamic programming problem
- Return to another binary tree problem
Retrieval Practice
Testing yourself is more effective than passively reviewing material:
- Why it works: Strengthens retrieval pathways that you’ll need during the interview
- Implementation: Close your notes and implement algorithms from memory, then check for errors
- Elaboration: After retrieving information, elaborate on why it works and how it connects to other concepts
Variability Training
Practicing the same concept in different contexts builds more robust understanding:
- Problem variations: Solve the same core problem with different constraints or data types
- Implementation variations: Implement the same algorithm in different programming languages
- Context variations: Practice in different environments (IDE, whiteboard, paper)
For example, after implementing a standard binary search, try these variations:
- Finding the first occurrence of a target
- Finding the last occurrence of a target
- Finding the closest element to a target
- Implementing it recursively vs. iteratively
Deliberate Difficulty
Introducing controlled difficulties during practice can enhance learning:
- Time constraints: Gradually reduce allowed solution time
- Explanation requirements: Practice explaining your solution as if to different audiences (peer, non-technical person, interviewer)
- Code without running: Write complete solutions without execution testing
- Constraint challenges: Solve problems with artificial constraints (no extra space, single pass, etc.)
Mindset Shifts for Interview Success
Your mental approach to interviews significantly impacts your ability to access knowledge under pressure. These mindset shifts can make a substantial difference.
Reframing Anxiety as Excitement
Research by Harvard psychologist Alison Wood Brooks shows that reframing anxiety as excitement improves performance:
- Physiological similarity: Anxiety and excitement produce similar physical responses
- Cognitive difference: Anxiety is a threat mindset; excitement is an opportunity mindset
- Implementation: Literally tell yourself “I’m excited” before interviews
Adopting a Growth Mindset
Carol Dweck’s research on mindset demonstrates that how you view challenges affects performance:
- Fixed mindset: “My performance shows my inherent ability” (increases pressure)
- Growth mindset: “This interview is a chance to learn and improve” (reduces pressure)
- Practical application: View mistakes as learning opportunities, not judgment on your worth
The Interviewer as Collaborator
Seeing the interviewer as an adversary increases stress; viewing them as a collaborator reduces it:
- Reality check: Most interviewers want you to succeed and will provide hints when needed
- Collaborative approach: “Let’s solve this together” vs. “I need to impress them”
- Question framing: “What do you think about this approach?” vs. “Is this right?”
Embracing the Process Over Outcomes
Focusing exclusively on getting the job increases pressure; focusing on the problem-solving process reduces it:
- Process goals: “I’ll clearly communicate my thinking” rather than “I must get the optimal solution”
- Learning orientation: Each interview provides valuable experience regardless of outcome
- Realistic expectations: Even top candidates make mistakes and have knowledge gaps
Case Studies: How Successful Candidates Overcome Forgetting
Learning from the experiences of others can provide valuable insights and practical strategies.
Case Study 1: The Systematic Preparer
Sarah, a software engineer who received offers from Google and Amazon, describes her approach:
“I created a systematic review schedule covering all core computer science topics. For each concept, I first explained it in my own words, then implemented key algorithms from scratch, and finally solved related problems. I repeated this cycle every two weeks, gradually spacing out reviews of topics I felt comfortable with.
During interviews, when I felt myself starting to blank, I would take a deep breath and verbalize what I did know about the problem. This often triggered the memory of the specific technique I needed. When truly stuck, I’d break the problem down into smaller parts and solve what I could, which usually led me back to the complete solution.”
Key takeaways:
- Systematic, spaced review of all core topics
- Implementation practice, not just conceptual understanding
- Verbalization as a memory trigger
- Breaking down problems when stuck
Case Study 2: The Pressure Simulator
Michael, who transitioned from a non-CS background to roles at Microsoft and Facebook, focused on simulating interview pressure:
“I realized early that I knew the material when studying but froze during interviews. So I created artificial pressure: I recorded myself solving problems, did mock interviews with friends who would intentionally make me nervous, and practiced in noisy environments.
I also developed a ‘panic protocol.’ If I forgot something, I’d acknowledge it directly: ‘I’m trying to recall the exact implementation of Dijkstra’s algorithm. Let me work through what I remember about shortest path algorithms…’ This honest approach actually impressed interviewers and often jogged my memory.”
Key takeaways:
- Deliberate simulation of interview pressure
- Development of a specific “panic protocol”
- Transparent communication about memory lapses
- Working from related concepts when specific details are forgotten
Case Study 3: The Conceptual Mapper
Lee, a senior developer who successfully interviewed at multiple FAANG companies, emphasized conceptual understanding:
“Instead of memorizing solutions to specific problems, I focused on deeply understanding about 20 core algorithms and data structures. For each, I created a concept map showing when to use it, its limitations, and related approaches.
During interviews, even if I couldn’t remember the exact implementation details, I could reason through the solution based on first principles. Interviewers seemed more impressed by this approach than by candidates who had memorized solutions but couldn’t explain why they worked.”
Key takeaways:
- Focus on deep understanding over memorization
- Creation of concept maps connecting related ideas
- Reasoning from first principles when specific implementations are forgotten
- Communicating thought process clearly to interviewers
Conclusion: Building Resilient Knowledge
Forgetting concepts during interviews is not a reflection of your abilities as a programmer but rather a natural response to a high-pressure situation. By understanding the cognitive mechanisms behind this phenomenon, you can develop strategies to build more resilient knowledge and recover gracefully when blanking does occur.
The most successful approach combines:
- Strategic preparation: Using spaced repetition, interleaved practice, and deliberate difficulty to strengthen memory
- Pressure inoculation: Gradually introducing stress elements into practice to build resistance
- Conceptual integration: Developing connected understanding rather than isolated facts
- Recovery techniques: Having specific strategies ready for when memory lapses occur
- Mindset management: Approaching interviews with a growth-oriented, process-focused attitude
Remember that even the most experienced developers sometimes forget concepts under pressure. What sets successful candidates apart isn’t perfect recall but rather how they handle these inevitable moments of uncertainty.
By implementing the strategies in this guide, you’ll not only improve your interview performance but also develop learning habits that will serve you throughout your programming career. The goal isn’t just to pass interviews but to build knowledge that remains accessible even in challenging situations—a skill that distinguishes truly exceptional software engineers.
Technical interviews may always involve some stress, but with the right preparation and mindset, you can ensure that your knowledge remains accessible when you need it most. Good luck with your interviews!