Why You Don’t Understand the Problem (And How to Change That)
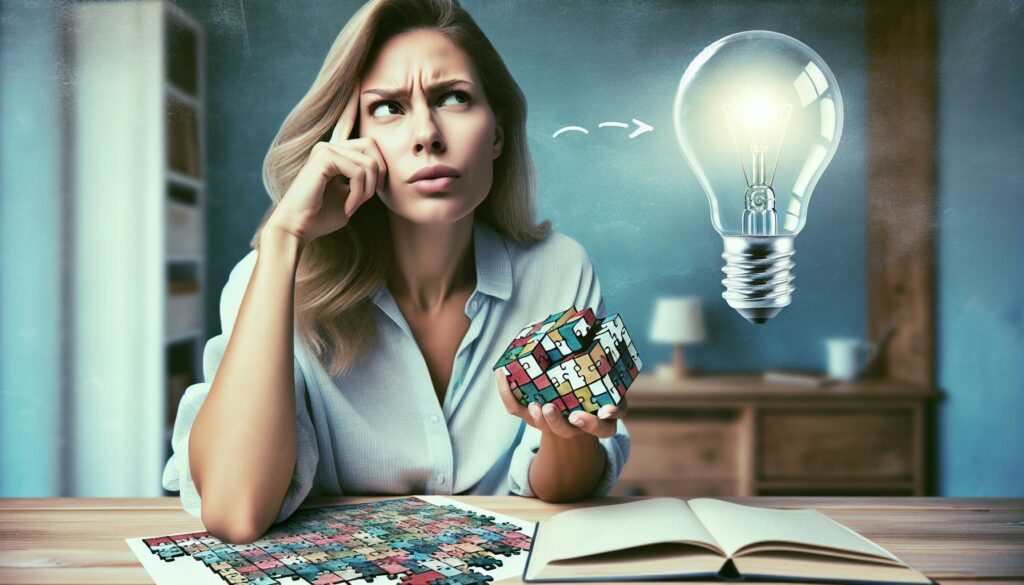
As aspiring programmers and coding enthusiasts, we’ve all been there: staring at a problem statement, feeling completely lost and overwhelmed. Whether you’re tackling a LeetCode challenge, preparing for a technical interview with a FAANG company, or simply trying to solve a coding puzzle, understanding the problem is the crucial first step. Yet, it’s often the step where many of us stumble. In this comprehensive guide, we’ll explore why you might be struggling to understand coding problems and, more importantly, how you can overcome these obstacles to become a more effective problem-solver.
The Importance of Problem Understanding in Coding
Before we dive into the reasons behind your struggles and the solutions to overcome them, let’s establish why understanding the problem is so critical in the world of programming:
- Foundation for Solution: A clear understanding of the problem forms the basis for developing an effective solution. Without it, you’re essentially trying to build a house without a blueprint.
- Efficiency: Properly grasping the problem can lead to more efficient solutions, saving time and computational resources.
- Interview Success: In technical interviews, especially for prestigious companies like those in FAANG, demonstrating a thorough understanding of the problem is often as important as solving it.
- Real-world Application: In professional settings, misunderstanding requirements can lead to wasted effort and incorrect implementations.
Common Reasons Why You Don’t Understand the Problem
Now, let’s explore some of the most common reasons why you might be struggling to understand coding problems:
1. Rushing Through the Problem Statement
In the excitement to start coding or the pressure of time constraints, many programmers skim through the problem statement without giving it the attention it deserves. This rush can lead to missing crucial details or misinterpreting the requirements.
2. Lack of Context or Domain Knowledge
Some problems are rooted in specific domains or real-world scenarios. If you’re unfamiliar with the context, understanding the problem becomes significantly more challenging.
3. Overwhelmed by Complexity
Complex problems with multiple components or advanced concepts can be intimidating. This intimidation can lead to a mental block, preventing you from breaking down the problem into manageable parts.
4. Insufficient Background in Algorithms and Data Structures
Many coding problems, especially those in technical interviews, require a solid foundation in algorithms and data structures. Without this background, the problem’s requirements might seem unclear or impossible.
5. Language Barriers
For non-native English speakers or those learning to code in a language different from their primary one, nuances in problem descriptions can be challenging to grasp.
6. Lack of Practice in Problem Analysis
Problem-solving is a skill that improves with practice. If you’re not accustomed to analyzing problems methodically, you might struggle to extract the key information needed to solve them.
7. Fixation on Implementation Details
Sometimes, programmers get too caught up in thinking about how to implement the solution before fully understanding what needs to be solved. This premature focus on coding can cloud your understanding of the problem itself.
How to Change Your Approach and Improve Problem Understanding
Now that we’ve identified common obstacles, let’s explore strategies to overcome them and enhance your ability to understand coding problems:
1. Slow Down and Read Carefully
Take your time when reading the problem statement. Read it multiple times if necessary. Pay attention to every word, as even small details can significantly impact the solution.
Action Step: For your next coding challenge, set a timer for 5 minutes dedicated solely to reading and re-reading the problem statement before you even think about coding.
2. Break Down the Problem
Divide complex problems into smaller, more manageable parts. This approach can make intimidating problems less overwhelming and easier to understand.
Example: If you’re tackling a problem involving manipulating a binary tree, break it down into steps like:
- Understanding the tree structure
- Traversing the tree
- Performing the required operation on each node
- Reconstructing or returning the result
3. Visualize the Problem
Create diagrams, flowcharts, or even simple sketches to represent the problem visually. This can help you grasp abstract concepts and see relationships between different components of the problem.
Tool Recommendation: Use online tools like draw.io or even a simple notepad to sketch out your understanding of the problem.
4. Restate the Problem in Your Own Words
After reading the problem, try to explain it to yourself or someone else in your own words. This exercise can help identify areas where your understanding might be lacking.
Technique: Use the “Rubber Duck Debugging” method. Explain the problem to an inanimate object (like a rubber duck) as if you’re teaching it. This process often helps clarify your thoughts and identify misunderstandings.
5. Identify Input, Output, and Constraints
Clearly define what inputs the problem expects, what output it should produce, and any constraints or special conditions mentioned. This structured approach can provide clarity and direction.
Example Format:
Input: An array of integers
Output: The maximum sum of any contiguous subarray
Constraints:
- Array length is between 1 and 10^5
- Each element is between -10^4 and 10^4
6. Look for Keywords and Patterns
Certain words or phrases in problem statements often hint at specific algorithms or data structures. Learning to recognize these can fast-track your understanding and solution approach.
Common Keywords:
- “in-order”, “pre-order”, “post-order” → Tree traversal
- “shortest path” → Graph algorithms like Dijkstra’s or BFS
- “dynamic programming” → Breaking problem into subproblems
7. Solve Simpler Versions First
If the problem seems too complex, try solving a simpler version first. This can help you understand the core concept before tackling the full complexity.
Example: If you’re asked to find the kth largest element in an unsorted array, start by solving for the largest element, then the second largest, before generalizing to the kth largest.
8. Use Concrete Examples
Create your own examples or test cases. Working through specific scenarios can illuminate the problem’s requirements and edge cases.
Tip: Always include edge cases in your examples, such as empty inputs, single-element inputs, or inputs with repeated elements.
9. Research Similar Problems
If you’re stuck, look for similar problems you’ve solved before or search for related concepts online. This can provide context and help you draw parallels to familiar territory.
Resource: Websites like LeetCode and AlgoCademy often group similar problems together, which can be helpful for understanding problem patterns.
10. Improve Your Algorithmic Thinking
Strengthen your foundation in algorithms and data structures. This knowledge will make it easier to recognize common problem patterns and solution approaches.
Study Plan: Dedicate time each week to learn or review a specific algorithm or data structure. Platforms like AlgoCademy offer structured learning paths for this purpose.
11. Practice Active Reading
Engage with the problem text actively. Underline key points, make notes, and jot down questions as you read. This active engagement can improve comprehension and retention.
Tool: Use a note-taking app or a physical notebook dedicated to problem analysis. Review your notes regularly to reinforce your understanding.
12. Leverage AI-Powered Assistance
Utilize AI tools designed to assist with problem understanding and solution development. These can provide explanations, hints, and alternative perspectives on the problem.
Caution: While AI tools can be helpful, rely on them as aids rather than crutches. The goal is to improve your own understanding, not to have the AI solve the problem for you.
Putting It All Together: A Step-by-Step Approach
To help you implement these strategies effectively, here’s a step-by-step approach you can follow when faced with a new coding problem:
- Initial Read-through: Carefully read the entire problem statement without trying to solve it.
- Identify Key Components: Note the input, expected output, and any constraints or special conditions.
- Visualize and Sketch: Create a visual representation of the problem if applicable.
- Restate the Problem: Explain the problem in your own words, either to yourself or someone else.
- Break It Down: Divide the problem into smaller, manageable sub-problems.
- Generate Examples: Create your own test cases, including edge cases.
- Research if Needed: If the problem seems familiar, look for similar problems you’ve encountered before.
- Plan Your Approach: Outline a high-level strategy for solving the problem before diving into code.
- Implement: Start coding your solution, referring back to your problem analysis as needed.
- Review and Refine: After implementing, review your solution against the original problem statement to ensure you’ve met all requirements.
Common Pitfalls to Avoid
As you work on improving your problem understanding skills, be aware of these common pitfalls:
- Assumption Overload: Don’t make assumptions about the problem that aren’t explicitly stated. If something is unclear, it’s better to ask for clarification (in an interview setting) or to consider multiple interpretations.
- Ignoring Constraints: Pay close attention to any constraints mentioned in the problem. These often guide you towards the expected solution and can rule out certain approaches.
- Solving the Wrong Problem: In your eagerness to start coding, make sure you’re solving the problem asked, not a similar but different problem you’re more familiar with.
- Overlooking Edge Cases: Always consider edge cases like empty inputs, extremely large inputs, or inputs with special characteristics (e.g., all negative numbers in an array).
- Fixating on One Approach: If your initial approach doesn’t work, be willing to take a step back and reconsider the problem from a different angle.
The Role of Practice in Improving Problem Understanding
Like any skill, the ability to understand and analyze coding problems improves with practice. Here are some ways to incorporate regular practice into your routine:
- Daily Problem Solving: Set aside time each day to tackle a new coding problem, focusing on thorough analysis before implementation.
- Study Groups: Join or form a study group where you can discuss problems and solution approaches with peers. Explaining problems to others can significantly boost your own understanding.
- Mock Interviews: Participate in mock technical interviews where you’re required to think aloud as you analyze and solve problems.
- Review and Reflect: After solving a problem, take time to reflect on your problem-solving process. Identify areas where you struggled to understand the problem and think about how you can improve next time.
- Diverse Problem Types: Expose yourself to a wide variety of problem types and domains to broaden your analytical skills.
Leveraging AlgoCademy for Enhanced Problem Understanding
AlgoCademy offers several features that can aid in developing your problem understanding skills:
- Interactive Tutorials: These guide you through the process of breaking down and analyzing problems step-by-step.
- Problem Categories: Problems are organized by type and difficulty, helping you recognize patterns and common problem structures.
- AI-Powered Assistance: Get hints and explanations tailored to your specific areas of confusion.
- Community Forums: Discuss problem interpretations and analysis strategies with other learners.
- Progress Tracking: Monitor your improvement in problem analysis and solving over time.
Conclusion: Embracing the Journey of Problem Understanding
Understanding coding problems is a fundamental skill that underpins all aspects of programming, from daily coding tasks to acing technical interviews at top tech companies. By recognizing the common obstacles to problem understanding and actively applying strategies to overcome them, you can significantly enhance your problem-solving capabilities.
Remember, the goal isn’t just to solve problems, but to develop a systematic approach to understanding and analyzing them. This skill will serve you well throughout your programming career, enabling you to tackle increasingly complex challenges with confidence.
As you continue your coding journey, whether you’re preparing for technical interviews, working on personal projects, or advancing your skills on platforms like AlgoCademy, make conscious efforts to improve your problem understanding techniques. With patience, practice, and persistence, you’ll find that what once seemed like insurmountable coding challenges become exciting puzzles waiting to be unraveled.
Happy coding, and may your journey of problem understanding lead you to new heights in your programming adventures!