Why You Don’t See Patterns Across Different Coding Problems (And How to Fix That)
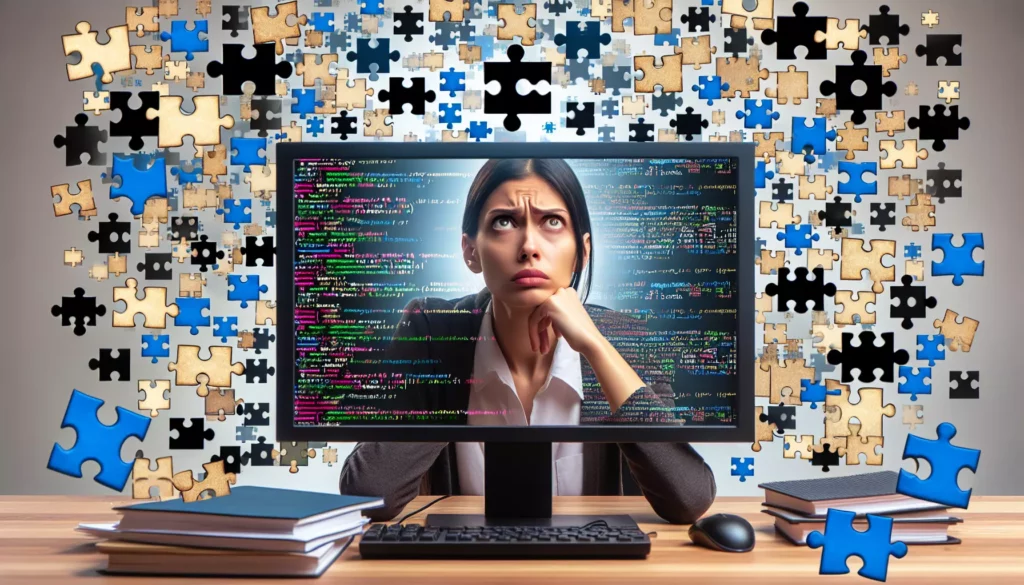
Have you ever found yourself solving a coding problem, only to face a similar one later and feel completely stumped? You’re not alone. Many programmers, from beginners to those preparing for technical interviews at major tech companies, struggle with pattern recognition across different coding challenges.
This inability to transfer knowledge from one problem to another can be frustrating and inefficient. You solve one sliding window problem, then encounter another and fail to recognize the connection. You master binary search for sorted arrays but don’t think to apply it when facing a different problem with a sorted input.
In this comprehensive guide, we’ll explore why programmers struggle to see patterns across different problems and provide actionable strategies to develop this crucial skill. By the end, you’ll have a framework for recognizing algorithmic patterns that will make you a more effective problem solver.
Table of Contents
- Why Pattern Recognition Matters in Programming
- Common Obstacles to Pattern Recognition
- Fundamental Algorithmic Patterns You Should Know
- Strategies for Improving Pattern Recognition
- Practical Exercises to Develop Pattern Recognition
- Case Studies: Seeing Patterns in Action
- Advanced Techniques for Pattern Mastery
- Conclusion: Building Your Pattern Recognition Muscle
Why Pattern Recognition Matters in Programming
Pattern recognition isn’t just a nice-to-have skill; it’s fundamental to becoming an efficient programmer and problem solver. Here’s why:
Efficiency in Problem Solving
When you can identify that a new problem fits a pattern you’ve already learned, you don’t need to reinvent the wheel. Instead of starting from scratch, you can adapt a solution approach you already understand. This dramatically reduces the time needed to solve problems.
Technical Interview Success
Technical interviews, especially at major tech companies, test your ability to recognize and apply common algorithmic patterns under pressure. Interviewers are often less interested in whether you’ve memorized a specific algorithm and more concerned with your ability to recognize which approach fits the problem.
Code Reusability and Maintenance
Recognizing patterns helps you write more modular, reusable code. When you see similarities across different features or requirements, you can design solutions that accommodate multiple use cases, leading to cleaner codebases that are easier to maintain.
Continuous Learning Acceleration
As you build a mental library of patterns, learning new concepts becomes easier. New information connects to existing knowledge, creating a network of understanding rather than isolated facts.
Common Obstacles to Pattern Recognition
If pattern recognition is so valuable, why do many programmers struggle with it? Let’s explore the common obstacles:
Superficial Understanding of Solutions
One of the biggest obstacles is learning solutions without truly understanding the underlying principles. When you merely memorize how to solve a specific problem without grasping why the approach works, you’ll struggle to apply that knowledge to different contexts.
For example, you might learn how to implement a specific dynamic programming solution for the “Climbing Stairs” problem, but if you don’t understand the core principle of breaking down a problem into overlapping subproblems, you won’t recognize when to apply dynamic programming to other challenges.
Problem-Specific Thinking
Many programmers approach each problem as a unique entity rather than looking for connections to previously solved problems. This isolated thinking prevents the formation of mental bridges between similar challenges.
Consider these two problems:
- Finding the maximum sum subarray
- Finding the maximum product subarray
While they have different operations (sum vs. product), they share the same underlying pattern: Kadane’s algorithm. If you’re stuck in problem-specific thinking, you might not see this connection.
Overemphasis on Implementation Details
Getting caught up in language-specific syntax or implementation details can obscure the algorithmic patterns. When your focus is predominantly on how to code a solution rather than understanding the problem-solving approach, pattern recognition suffers.
Lack of Systematic Categorization
Without a framework for categorizing problems and solutions, it’s difficult to make connections. If you don’t explicitly organize knowledge into patterns like “sliding window,” “two pointers,” or “graph traversal,” you’re less likely to recognize when a new problem fits these categories.
Insufficient Deliberate Practice
Random problem solving without reflection doesn’t build pattern recognition skills effectively. Many programmers jump from problem to problem without taking time to analyze similarities and differences, missing opportunities to strengthen their pattern recognition abilities.
Fundamental Algorithmic Patterns You Should Know
Before diving into how to improve pattern recognition, let’s outline some of the most common algorithmic patterns you should be familiar with. Understanding these foundational patterns will give you a framework for categorizing new problems.
Two Pointers Pattern
This pattern uses two pointers to iterate through a data structure, often moving in tandem or in opposite directions.
Common applications:
- Finding pairs in a sorted array with a target sum
- Removing duplicates from a sorted array
- Palindrome verification
- Container with most water
Example problem: Given a sorted array, find a pair of elements that sum to a specific target.
function findPairWithSum(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left < right) {
const currentSum = arr[left] + arr[right];
if (currentSum === target) {
return [arr[left], arr[right]];
} else if (currentSum < target) {
left++;
} else {
right--;
}
}
return null;
}
Sliding Window Pattern
This pattern involves creating a “window” that can expand or contract as it moves through an array or string, maintaining a subset of elements within the current window.
Common applications:
- Finding the longest substring with certain properties
- Finding maximum/minimum sum subarrays of a fixed size
- String permutations
- Longest repeating character replacement
Example problem: Find the maximum sum of a subarray of size k.
function maxSubarraySum(arr, k) {
if (arr.length < k) return null;
let maxSum = 0;
let windowSum = 0;
// Calculate sum of first window
for (let i = 0; i < k; i++) {
windowSum += arr[i];
}
maxSum = windowSum;
// Slide the window and update maxSum
for (let i = k; i < arr.length; i++) {
windowSum = windowSum - arr[i - k] + arr[i];
maxSum = Math.max(maxSum, windowSum);
}
return maxSum;
}
Fast and Slow Pointers (Floyd’s Cycle Detection)
This pattern uses two pointers moving at different speeds to identify cycles or find specific elements in linked lists or sequences.
Common applications:
- Detecting cycles in linked lists
- Finding the middle of a linked list
- Finding the start of a cycle
- Determining if a number is happy
Example problem: Detect a cycle in a linked list.
function hasCycle(head) {
if (!head || !head.next) return false;
let slow = head;
let fast = head;
while (fast && fast.next) {
slow = slow.next;
fast = fast.next.next;
if (slow === fast) {
return true;
}
}
return false;
}
Merge Intervals Pattern
This pattern deals with overlapping intervals or ranges and how to merge or manage them.
Common applications:
- Merging overlapping intervals
- Finding conflicting appointments
- Finding the minimum number of meeting rooms required
Example problem: Merge overlapping intervals.
function mergeIntervals(intervals) {
if (!intervals.length) return [];
intervals.sort((a, b) => a[0] - b[0]);
const result = [intervals[0]];
for (let i = 1; i < intervals.length; i++) {
const currentInterval = intervals[i];
const lastMergedInterval = result[result.length - 1];
if (currentInterval[0] <= lastMergedInterval[1]) {
// Overlapping intervals, update the end time
lastMergedInterval[1] = Math.max(lastMergedInterval[1], currentInterval[1]);
} else {
// Non-overlapping interval, add to result
result.push(currentInterval);
}
}
return result;
}
Binary Search Pattern
This pattern divides the search space in half at each step, allowing for efficient searching in sorted arrays or on a range of values.
Common applications:
- Finding an element in a sorted array
- Finding the first or last occurrence of an element
- Finding the smallest element greater than a target
- Search in rotated sorted arrays
Example problem: Find the first and last position of an element in a sorted array.
function searchRange(nums, target) {
const findFirst = () => {
let left = 0;
let right = nums.length - 1;
let result = -1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (nums[mid] >= target) {
right = mid - 1;
if (nums[mid] === target) result = mid;
} else {
left = mid + 1;
}
}
return result;
};
const findLast = () => {
let left = 0;
let right = nums.length - 1;
let result = -1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (nums[mid] <= target) {
left = mid + 1;
if (nums[mid] === target) result = mid;
} else {
right = mid - 1;
}
}
return result;
};
return [findFirst(), findLast()];
}
Breadth-First Search (BFS) Pattern
This pattern explores all neighbor nodes at the present depth before moving to nodes at the next depth level.
Common applications:
- Level-order traversal of trees
- Finding shortest path in unweighted graphs
- Connected components in graphs
- Word ladder problems
Example problem: Level order traversal of a binary tree.
function levelOrder(root) {
if (!root) return [];
const result = [];
const queue = [root];
while (queue.length) {
const levelSize = queue.length;
const currentLevel = [];
for (let i = 0; i < levelSize; i++) {
const node = queue.shift();
currentLevel.push(node.val);
if (node.left) queue.push(node.left);
if (node.right) queue.push(node.right);
}
result.push(currentLevel);
}
return result;
}
Depth-First Search (DFS) Pattern
This pattern explores as far as possible along each branch before backtracking.
Common applications:
- Tree traversals (preorder, inorder, postorder)
- Path finding problems
- Cycle detection in graphs
- Topological sorting
Example problem: Find all paths from source to target in a directed acyclic graph.
function allPathsSourceTarget(graph) {
const target = graph.length - 1;
const result = [];
function dfs(node, path) {
path.push(node);
if (node === target) {
result.push([...path]);
} else {
for (const neighbor of graph[node]) {
dfs(neighbor, path);
}
}
path.pop();
}
dfs(0, []);
return result;
}
Dynamic Programming Pattern
This pattern breaks down complex problems into simpler subproblems and stores their solutions to avoid redundant computations.
Common applications:
- Fibonacci sequence
- Knapsack problems
- Longest common subsequence
- Coin change problems
Example problem: Climbing stairs problem.
function climbStairs(n) {
if (n <= 2) return n;
const dp = new Array(n + 1);
dp[1] = 1;
dp[2] = 2;
for (let i = 3; i <= n; i++) {
dp[i] = dp[i - 1] + dp[i - 2];
}
return dp[n];
}
Strategies for Improving Pattern Recognition
Now that we’ve covered common algorithmic patterns, let’s explore strategies to improve your pattern recognition skills:
Study Problems by Pattern Category
Instead of solving random problems, organize your learning by pattern categories. Spend a week focusing on sliding window problems, then move to two-pointer problems, and so on. This focused approach helps your brain recognize the similarities within each pattern group.
Implementation:
- Create a study plan organized by algorithmic patterns
- For each pattern, study 5-10 problems of increasing difficulty
- Before moving to a new pattern, ensure you can identify when to apply the current one
Solve Problems Multiple Ways
For each problem you solve, challenge yourself to find alternative solutions. This expands your understanding of how different patterns can apply to the same problem.
Implementation:
- After solving a problem, ask: “Could I use a different approach?”
- Implement at least one alternative solution
- Compare the time and space complexity of different approaches
Analyze and Annotate Solutions
Don’t just implement solutions; annotate them with explanations of why certain approaches work. This deepens your understanding and makes patterns more explicit.
Implementation:
- Create a template for solution annotations that includes:
- Problem pattern identification
- Key insights that led to the solution
- Why this pattern applies to this problem
- Potential variations of the problem
- Maintain a personal repository of annotated solutions
Teach and Explain Problems to Others
Teaching forces you to articulate your understanding, which strengthens pattern recognition. When you explain why a particular approach works, you reinforce your own mental models.
Implementation:
- Join or create a study group
- Write blog posts explaining algorithmic patterns
- Create video explanations of problem solutions
- Participate in forums where you can answer questions about algorithms
Practice Pattern Identification Exercises
Deliberately practice identifying patterns in problems before solving them. This trains your brain to look for pattern indicators.
Implementation:
- Before coding, write down which pattern you think applies and why
- Review a list of problems and categorize them by pattern without solving them
- Create flashcards with problem descriptions on one side and the applicable pattern on the other
Create Visual Representations
Visual representations of algorithms can help you see patterns more clearly. Create diagrams, flowcharts, or animations to visualize how different patterns work.
Implementation:
- Draw out the execution of algorithms step by step
- Create mind maps connecting related problems and patterns
- Use visualization tools to animate algorithm execution
Develop a Pattern Recognition Framework
Create a systematic approach to analyzing new problems that helps you identify applicable patterns.
Implementation: When faced with a new problem, ask yourself:
- What is the input structure? (Array, string, tree, graph, etc.)
- What are we looking for? (A specific value, optimization, counting, etc.)
- Are there any constraints that suggest a pattern? (Sorted data suggests binary search, etc.)
- Can the problem be broken down into subproblems? (Suggests dynamic programming)
- Are we processing elements sequentially or need to track a range? (Suggests sliding window)
Practical Exercises to Develop Pattern Recognition
Let’s explore some specific exercises designed to strengthen your pattern recognition abilities:
Pattern Classification Exercise
Take a list of 20 problem descriptions (without solutions) and classify them according to the patterns they likely use. Then check your answers against expert classifications.
Example:
- Problem: “Find all pairs of numbers in a sorted array that sum to a target value.”
- Your classification: Two Pointers
- Problem: “Find the longest substring without repeating characters.”
- Your classification: Sliding Window
Pattern Transformation Exercise
Take a problem that uses one pattern and modify it slightly to require a different pattern. This helps you understand the boundaries between patterns.
Example:
- Original problem: “Find a pair of numbers in a sorted array that sum to a target.” (Two Pointers)
- Transformed problem: “Find a pair of numbers in an unsorted array that sum to a target.” (Hash Table)
Pattern Recognition Speed Drills
Set a timer and try to identify the appropriate pattern for as many problem descriptions as possible within a time limit. This builds your ability to quickly recognize patterns.
Example: Set a 5-minute timer and classify 15 problem descriptions by pattern.
Pattern Application Case Studies
Analyze real-world code bases or applications to identify algorithmic patterns in production code. This helps connect theoretical patterns to practical implementations.
Example: Analyze how a database query optimizer uses dynamic programming to determine the optimal query execution plan.
Cross-Pattern Problem Solving
Deliberately practice solving problems that combine multiple patterns. This builds your ability to recognize when problems require hybrid approaches.
Example: A problem that requires both BFS and a sliding window, such as “minimum genetic mutation” problems.
Case Studies: Seeing Patterns in Action
Let’s examine a few case studies that demonstrate how recognizing patterns leads to elegant solutions:
Case Study 1: From Brute Force to Pattern-Based Solution
Problem: Maximum Subarray Sum
Brute Force Approach: Calculate the sum of all possible subarrays and find the maximum.
function maxSubarrayBruteForce(nums) {
let maxSum = -Infinity;
for (let i = 0; i < nums.length; i++) {
let currentSum = 0;
for (let j = i; j < nums.length; j++) {
currentSum += nums[j];
maxSum = Math.max(maxSum, currentSum);
}
}
return maxSum;
}
Pattern Recognition: This is a classic Kadane’s algorithm problem, which is a form of dynamic programming.
function maxSubarrayKadane(nums) {
let maxSoFar = nums[0];
let maxEndingHere = nums[0];
for (let i = 1; i < nums.length; i++) {
maxEndingHere = Math.max(nums[i], maxEndingHere + nums[i]);
maxSoFar = Math.max(maxSoFar, maxEndingHere);
}
return maxSoFar;
}
Key Insight: By recognizing the dynamic programming pattern, we reduced the time complexity from O(n²) to O(n).
Case Study 2: Recognizing a Pattern Across Different Problem Domains
Problem 1: Detect a cycle in a linked list.
Problem 2: Determine if a number is a “happy number”.
These problems seem unrelated, but they both use the Fast and Slow Pointers pattern (Floyd’s Cycle Detection).
Linked List Cycle Detection:
function hasCycle(head) {
if (!head || !head.next) return false;
let slow = head;
let fast = head;
while (fast && fast.next) {
slow = slow.next;
fast = fast.next.next;
if (slow === fast) {
return true;
}
}
return false;
}
Happy Number Detection:
function isHappy(n) {
function getNext(number) {
let totalSum = 0;
while (number > 0) {
const digit = number % 10;
totalSum += digit * digit;
number = Math.floor(number / 10);
}
return totalSum;
}
let slow = n;
let fast = getNext(n);
while (fast !== 1 && slow !== fast) {
slow = getNext(slow);
fast = getNext(getNext(fast));
}
return fast === 1;
}
Key Insight: Both problems involve detecting cycles in a sequence, even though one deals with a linked list and the other with a number sequence. Recognizing this common pattern allows you to apply the same solution approach.
Case Study 3: Adapting a Pattern to a New Context
Problem: Find the length of the longest substring with at most K distinct characters.
This is a sliding window problem, but with a dynamic window size based on a constraint.
function longestSubstringWithKDistinct(s, k) {
if (s.length === 0 || k === 0) return 0;
let maxLength = 0;
let start = 0;
const charFrequency = new Map();
for (let end = 0; end < s.length; end++) {
const rightChar = s[end];
charFrequency.set(rightChar, (charFrequency.get(rightChar) || 0) + 1);
// Shrink the window until we have at most k distinct characters
while (charFrequency.size > k) {
const leftChar = s[start];
charFrequency.set(leftChar, charFrequency.get(leftChar) - 1);
if (charFrequency.get(leftChar) === 0) {
charFrequency.delete(leftChar);
}
start++;
}
maxLength = Math.max(maxLength, end - start + 1);
}
return maxLength;
}
Key Insight: By recognizing this as a sliding window problem, we can adapt the pattern to handle the constraint of having at most K distinct characters.
Advanced Techniques for Pattern Mastery
Once you’ve developed basic pattern recognition skills, consider these advanced techniques:
Pattern Hybridization
Learn to combine multiple patterns to solve complex problems. For example, using both binary search and dynamic programming in the “Allocate Minimum Number of Pages” problem.
Implementation:
- Study problems that use multiple patterns
- Practice creating problems that require pattern combinations
- Analyze how different patterns can complement each other
Pattern Generalization
Learn to generalize patterns to solve entire classes of problems. For example, understanding how the sliding window pattern can be generalized to handle various constraints.
Implementation:
- Create templates for each pattern that can be adapted to different problems
- Practice applying these templates to new problems
- Identify the key parameters that change between similar problems
Problem Design
Design your own problems that use specific patterns. This deepens your understanding of when and why patterns apply.
Implementation:
- For each pattern, create 2-3 original problems
- Test these problems on peers and analyze their approaches
- Refine the problems to better highlight the target pattern
Pattern Recognition in Real-World Systems
Study how algorithmic patterns are used in real-world systems and applications.
Implementation:
- Analyze open-source codebases to identify algorithmic patterns
- Study system design case studies to see patterns at scale
- Connect theoretical patterns to practical applications
Meta-Learning Techniques
Develop systems to track and improve your pattern recognition abilities over time.
Implementation:
- Keep a pattern recognition journal documenting your insights
- Regularly review and update your pattern catalog
- Track your accuracy in pattern identification and set improvement goals
Conclusion: Building Your Pattern Recognition Muscle
Pattern recognition is not an innate talent but a skill that can be developed through deliberate practice and structured learning. By understanding common obstacles, studying fundamental patterns, implementing effective strategies, and engaging in targeted exercises, you can dramatically improve your ability to see connections across different coding problems.
Remember that developing this skill takes time. Be patient with yourself and celebrate small victories as you begin to recognize patterns more readily. Over time, you’ll build a robust mental library of algorithmic patterns that will make you a more effective problem solver and programmer.
The ability to transfer knowledge from one problem to another is what separates experienced programmers from novices. By investing in your pattern recognition skills, you’re not just preparing for technical interviews; you’re developing a fundamental capability that will serve you throughout your programming career.
Start today by choosing one pattern from this guide and solving 3-5 problems that use it. Pay attention to the similarities and differences between the problems, and explicitly note the pattern indicators. With consistent practice, you’ll soon find yourself saying, “I’ve seen something like this before,” when facing new challenges—the first step to becoming a more efficient and effective problem solver.
Happy pattern hunting!