Why You Don’t Know Which Solution Approach to Choose (And How to Fix It)
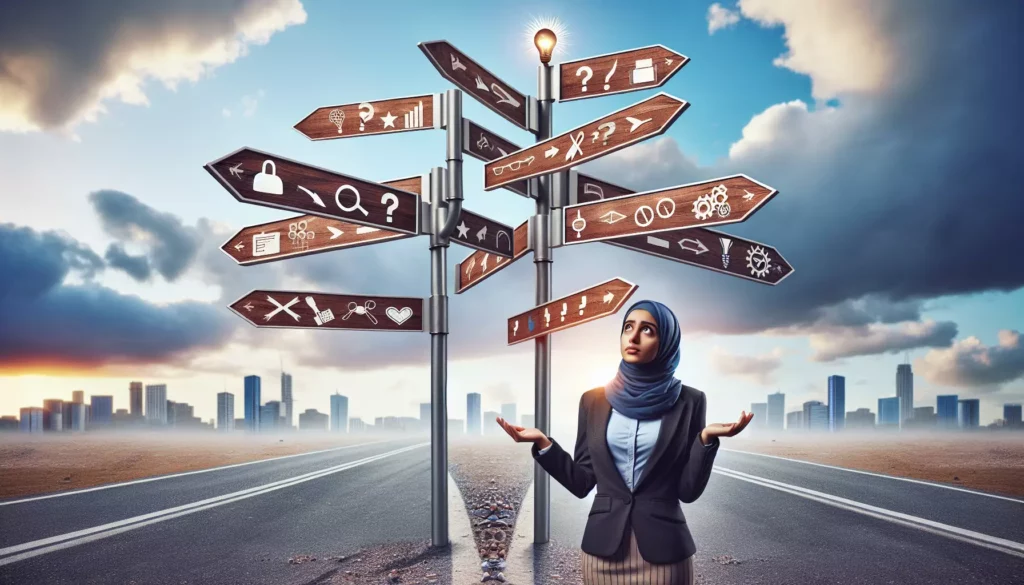
When facing a coding problem, do you often freeze, unsure which approach to take? You’re not alone. Many programmers, from beginners to those preparing for FAANG interviews, struggle with selecting the right solution strategy. This decision paralysis can significantly slow your coding progress and diminish your confidence.
In this comprehensive guide, we’ll explore why choosing a solution approach is challenging and provide practical strategies to overcome this common obstacle. By the end, you’ll have a systematic framework for tackling any coding problem with greater clarity and confidence.
The Problem of Solution Approach Paralysis
Solution approach paralysis occurs when you understand a coding problem but cannot decide how to solve it. You might recognize elements of different algorithms or data structures that could work, but you’re uncertain which path will lead to the most efficient solution.
Consider this scenario: You’re given a problem about finding the shortest path in a graph. Your mind races through possibilities: Dijkstra’s algorithm? Breadth-first search? A* search? The more options you know, the more overwhelming the choice can become.
Why This Happens: The Root Causes
1. Knowledge Gaps in Fundamentals
Often, indecision stems from incomplete understanding of the fundamental algorithms and data structures. When you don’t fully grasp how a binary search tree differs from a hash table in practical applications, choosing between them becomes guesswork rather than an informed decision.
2. Limited Pattern Recognition
Experienced programmers see patterns in problems that immediately suggest certain approaches. Without this pattern recognition ability, each problem feels unique and disconnected from previous experience.
3. Fear of Inefficiency
Many programmers, especially those preparing for technical interviews, worry about selecting a suboptimal solution. This fear can lead to decision paralysis as you overthink potential time and space complexity tradeoffs.
4. Lack of Systematic Approach
Without a methodical way to evaluate solution options, decision-making becomes random and inconsistent. You might choose approaches based on what you’ve used recently rather than what best fits the current problem.
5. Overexposure to Solutions
Paradoxically, studying too many solutions without understanding their fundamental principles can increase confusion. You might remember fragments of different approaches without knowing when to apply each one.
The Cost of Indecision
Being unable to select a solution approach has several negative consequences:
- Wasted time as you cycle through options without making progress
- Reduced confidence in your programming abilities
- Poor performance in timed settings like coding interviews
- Incomplete or abandoned projects
- Difficulty advancing to more complex programming challenges
Building a Solution Selection Framework
The good news is that choosing a solution approach is a learnable skill. Let’s develop a systematic framework to help you make these decisions more effectively.
Step 1: Problem Analysis
Before considering any solution, thoroughly understand the problem:
Identify the Core Task
Distill the problem to its essence. Are you searching, sorting, transforming data, finding patterns, or optimizing a value?
Clarify Constraints
Understand the limitations and requirements:
- Input size and range
- Time and space complexity requirements
- Special constraints (in-place modification, no extra space, etc.)
Recognize the Data Type
Different data types often suggest different approaches:
- Arrays/Lists: Two pointers, sliding window, divide and conquer
- Strings: Dynamic programming, tries, KMP algorithm
- Trees: Depth-first search, breadth-first search, recursion
- Graphs: BFS, DFS, Dijkstra’s, topological sort
- Numbers: Math properties, bit manipulation
Step 2: Pattern Matching
Connect the current problem to patterns you’ve encountered before:
Common Problem Patterns
Learn to recognize these frequent patterns:
- Search problems: Binary search, linear search, depth/breadth-first search
- Optimization problems: Dynamic programming, greedy algorithms
- Transformation problems: Recursion, iteration with data structures
- Connectivity problems: Graph algorithms, union-find
- Ordering problems: Sorting algorithms, priority queues
Look for Clues in the Problem Statement
Certain keywords often hint at specific approaches:
- “Minimum” or “maximum” often suggests dynamic programming or greedy algorithms
- “All possible combinations” might indicate backtracking
- “Shortest path” points to BFS or Dijkstra’s algorithm
- “Sorted array” likely involves binary search
- “Balanced” might suggest a tree structure
Step 3: Evaluate Multiple Approaches
Instead of immediately committing to a solution, consider multiple approaches:
The Brute Force Baseline
Always start by identifying the simplest solution, even if inefficient. This provides a baseline and ensures you have at least one workable approach.
Consider Time and Space Complexity
For each potential approach, estimate:
- Time complexity: How runtime scales with input size
- Space complexity: How memory usage scales with input size
- Constant factors: Even with the same Big O, some algorithms are faster for practical input sizes
Assess Implementation Complexity
Some theoretically optimal solutions are difficult to implement correctly, especially under time pressure. Consider how prone to bugs each approach might be.
Step 4: Make an Informed Decision
With multiple approaches evaluated, it’s time to decide:
Prioritize Based on Context
- In interviews: Balance optimal complexity with implementation simplicity
- In production code: Consider readability and maintainability
- In competitive programming: Optimize for speed of implementation and correctness
Start Simple, Then Optimize
When uncertain, implement the simplest correct solution first, then optimize if needed. This incremental approach often leads to insights about more efficient solutions.
Practical Examples: Applying the Framework
Let’s apply our framework to some common coding problems:
Example 1: Finding a Target in a Sorted Array
Step 1: Problem Analysis
- Core task: Searching for a value
- Data type: Sorted array
- Constraints: No specific constraints mentioned
Step 2: Pattern Matching
- This is a search problem
- “Sorted array” strongly suggests binary search
Step 3: Evaluate Multiple Approaches
- Linear search: O(n) time, O(1) space
- Binary search: O(log n) time, O(1) space
Step 4: Decision
Binary search is clearly superior for sorted arrays, with O(log n) being much better than O(n) for large inputs. The implementation is relatively straightforward:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = left + (right - left) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
Example 2: Finding the Maximum Subarray Sum
Step 1: Problem Analysis
- Core task: Finding maximum value (optimization)
- Data type: Array of integers (can be positive or negative)
- Constraints: Need to find contiguous subarray
Step 2: Pattern Matching
- This is an optimization problem
- Involves finding a maximum value
- Requires considering subarrays
Step 3: Evaluate Multiple Approaches
- Brute force: Check all subarrays – O(n²) time, O(1) space
- Divide and conquer: O(n log n) time, O(log n) space
- Kadane’s algorithm: O(n) time, O(1) space
Step 4: Decision
Kadane’s algorithm provides the optimal solution with linear time complexity and constant space. It’s also relatively simple to implement:
def max_subarray_sum(arr):
current_sum = max_sum = arr[0]
for num in arr[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
Example 3: Finding All Permutations of a String
Step 1: Problem Analysis
- Core task: Generate all possible arrangements
- Data type: String
- Constraints: Need all permutations (factorial growth)
Step 2: Pattern Matching
- This is a combinatorial problem
- “All possible arrangements” suggests backtracking or recursion
Step 3: Evaluate Multiple Approaches
- Recursive backtracking: O(n!) time due to n! permutations, O(n) space for recursion stack
- Iterative approach: Still O(n!) time, potentially less overhead
Step 4: Decision
Backtracking is the natural choice for permutation problems. The recursive implementation is more intuitive:
def generate_permutations(s):
result = []
s = list(s) # Convert string to list for easier manipulation
def backtrack(start):
if start == len(s):
result.append(''.join(s))
return
for i in range(start, len(s)):
# Swap characters
s[start], s[i] = s[i], s[start]
# Recursively generate permutations for the rest
backtrack(start + 1)
# Backtrack (undo the swap)
s[start], s[i] = s[i], s[start]
backtrack(0)
return result
Common Solution Approaches and When to Use Them
Let’s explore the most common solution approaches and the indicators that suggest when to use each one.
1. Brute Force
When to Use:
- When the input size is very small
- As a starting point to understand the problem better
- When more efficient algorithms are too complex to implement quickly
Indicators:
- Time constraints are lenient
- Problem statement is straightforward but no obvious efficient algorithm comes to mind
2. Divide and Conquer
When to Use:
- When a problem can be broken down into similar subproblems
- For optimization in sorting (merge sort, quicksort) and searching
- For problems involving arrays, binary trees, or matrices
Indicators:
- The problem involves sorting, searching, or optimization
- Subproblems appear independent of each other
- The problem size reduces significantly when divided
3. Dynamic Programming
When to Use:
- When the problem has overlapping subproblems
- When there’s an optimal substructure (optimal solution contains optimal solutions to subproblems)
- For optimization problems involving sequences or grids
Indicators:
- Keywords like “maximum,” “minimum,” “longest,” “shortest”
- The problem asks for counting the number of ways to do something
- Direct recursive solutions lead to repeated computations
4. Greedy Algorithms
When to Use:
- When local optimal choices lead to a global optimum
- For optimization problems with no need to reconsider choices
- When a simple, efficient solution is needed
Indicators:
- Problems involving scheduling or selection
- Problems where you need to maximize or minimize a value
- When the problem has a “matroid” structure
5. Backtracking
When to Use:
- When you need to find all possible solutions
- For constraint satisfaction problems
- When the problem involves exploring a search space
Indicators:
- Phrases like “all possible,” “every combination,” or “find ways”
- Problems involving permutations, combinations, or subsets
- Problems with multiple constraints that must be satisfied
6. Graph Algorithms
When to Use:
- When relationships between entities are important
- For problems involving networks, paths, or connectivity
- When the problem naturally maps to nodes and edges
Indicators:
- The problem involves finding paths, cycles, or connected components
- Keywords like “network,” “connection,” or “dependency”
- The data represents relationships between objects
7. Binary Search
When to Use:
- When searching in a sorted collection
- For optimization problems where you can use a decision procedure
- When you need to find a specific value or boundary
Indicators:
- The collection is sorted or can be sorted
- You need to find a specific value or the first/last occurrence
- The problem involves finding a minimum or maximum value that satisfies certain conditions
8. Two Pointers / Sliding Window
When to Use:
- When dealing with sorted arrays or linked lists
- For problems involving subarrays or subsequences
- When you need to track a range of elements
Indicators:
- Problems involving subarrays or subsequences
- The problem asks for pairs or ranges with certain properties
- You need to track a continuous segment of data
Developing Your Solution Approach Intuition
Beyond the framework, here are strategies to build your intuition for selecting solution approaches:
1. Deliberate Practice with Problem Categories
Instead of solving random problems, focus on problems grouped by approach. Spend a week on dynamic programming problems, then a week on graph problems, and so on. This focused practice helps you recognize patterns more quickly.
2. Analyze Multiple Solutions
For each problem you solve, research alternative solutions. Understanding different approaches to the same problem builds your solution repertoire.
3. Create a Personal Problem Catalog
Maintain a catalog of problems you’ve solved, categorized by the approach used. Review this catalog regularly to reinforce pattern recognition.
4. Practice Solution Mapping
When reading a new problem, before solving it, write down which approaches might work and why. This exercise strengthens your solution selection muscles.
5. Time-Boxed Decision Making
Set a timer for 3-5 minutes when deciding on an approach. This practice prevents overthinking and builds confidence in your initial instincts.
Common Pitfalls in Solution Selection
Be aware of these common mistakes when choosing solution approaches:
1. Premature Optimization
Jumping to the most efficient algorithm before understanding the problem fully often leads to unnecessary complexity or incorrect solutions.
2. Algorithm Fixation
Getting stuck on a particular approach because it’s familiar, even when it’s not the best fit for the current problem.
3. Complexity Intimidation
Avoiding certain approaches (like dynamic programming) because they seem difficult, even when they’re the most appropriate solution.
4. Ignoring Problem Constraints
Selecting an approach without considering the specific constraints of the problem, such as input size or memory limitations.
5. Reinventing the Wheel
Trying to create a novel solution when a well-established algorithm would work perfectly.
Real-world Application: Technical Interviews
Solution approach selection is particularly critical in technical interviews. Here’s how to apply our framework in interview settings:
Think Aloud
Verbalize your thought process as you analyze the problem and consider different approaches. This demonstrates your problem-solving methodology even if you don’t immediately arrive at the optimal solution.
Start Simple and Iterate
Begin with a brute force approach, analyze its complexity, and then work toward optimizing it. This shows your ability to improve solutions incrementally.
Ask Clarifying Questions
Use the problem analysis step to ask meaningful questions about constraints, input sizes, and edge cases. This demonstrates thoroughness and attention to detail.
Justify Your Choice
Explain why you selected a particular approach over alternatives, discussing the tradeoffs involved. This shows your ability to make reasoned technical decisions.
Building a Lifelong Learning System
Developing solution selection skills is an ongoing process. Here’s how to continue improving:
Regular Review and Reflection
Periodically review problems you’ve solved and reflect on the approaches used. Consider whether different approaches might have worked better.
Study Algorithm Design Principles
Beyond specific algorithms, understand the principles behind algorithm design. Books like “Algorithm Design Manual” by Steven Skiena provide valuable insights into the thinking process.
Participate in Coding Communities
Engage with communities like LeetCode, HackerRank, or CodeForces. Discussing problems with others exposes you to different approaches and perspectives.
Teach Others
Explaining solution approaches to others deepens your understanding and forces you to articulate your decision-making process clearly.
Conclusion: From Paralysis to Confidence
Solution approach selection is a skill that improves with structured practice and reflection. By following the framework outlined in this guide, you can transform from someone who freezes when facing coding problems to someone who confidently evaluates options and selects the most appropriate approach.
Remember that even experienced programmers occasionally choose suboptimal approaches. The goal isn’t perfection but a systematic process that leads to continuous improvement. With each problem you solve, your pattern recognition abilities will strengthen, and your solution selection will become more intuitive.
Start by applying the four-step framework to your next coding challenge:
- Analyze the problem thoroughly
- Match it to known patterns
- Evaluate multiple approaches
- Make an informed decision
Over time, you’ll develop the confidence to tackle any programming challenge, knowing that you have a reliable system for choosing the right solution approach.
Happy coding!