Why You Don’t Know Where to Start With New Coding Problems
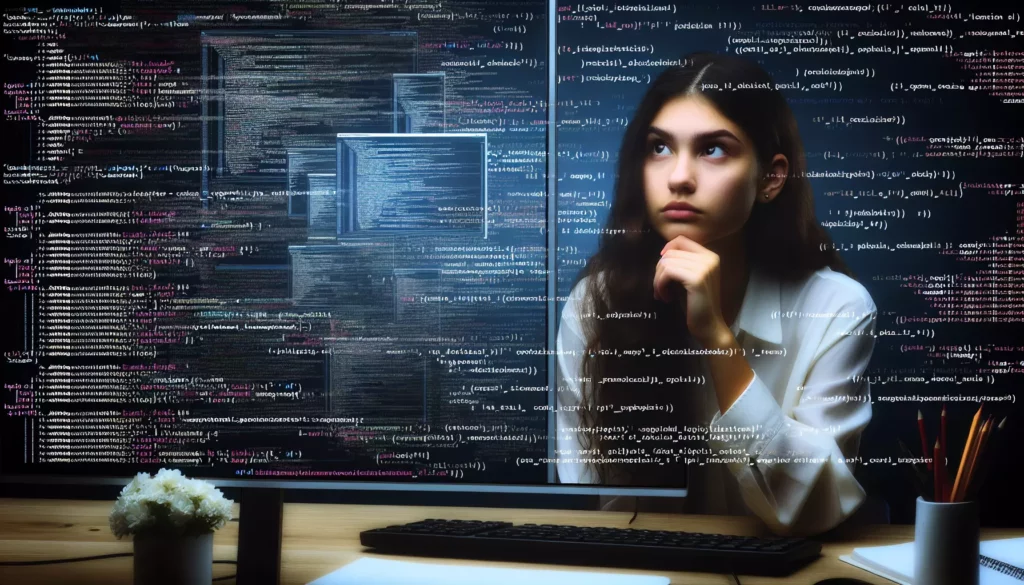
We’ve all been there. You open a new coding problem, read the description, and suddenly your mind goes blank. The cursor blinks mockingly on an empty editor, and despite your knowledge and experience, you simply don’t know where to begin.
This phenomenon isn’t unique to beginners. Even seasoned developers frequently encounter problems that leave them temporarily paralyzed, unsure of which first step to take. It’s a universal experience in the programming journey that causes frustration, self doubt, and sometimes leads people to question their abilities altogether.
In this comprehensive guide, we’ll explore why this happens, the psychological barriers that prevent us from starting, and practical strategies to overcome this common challenge. Whether you’re preparing for technical interviews at major tech companies or simply trying to improve your algorithmic thinking, understanding how to approach unfamiliar problems is a crucial skill for any programmer.
The Psychology Behind Problem Solving Paralysis
Before we discuss specific coding strategies, it’s important to understand the psychological factors that contribute to that “blank mind” feeling when facing new problems.
The Overwhelm of Complexity
When we encounter a new problem, our brains immediately try to process its entirety. For complex coding challenges, this means simultaneously considering input validation, edge cases, algorithmic approach, data structures, time complexity, space complexity, and implementation details. This cognitive load can overwhelm our working memory, leading to a mental shutdown.
Research in cognitive psychology shows that humans can typically only hold about 4-7 chunks of information in working memory at once. A complex coding problem easily exceeds this capacity, especially when we try to solve it all at once.
Fear of Failure and Perfectionism
Many programmers, especially those with high standards or imposter syndrome, fear writing “bad” or inefficient code. This perfectionism creates a paralysis where you feel that unless you can see the optimal solution immediately, you shouldn’t start at all.
This is particularly common among mid level developers who know enough to recognize multiple potential approaches but lack the confidence to commit to one without guaranteeing it’s the best solution.
The Knowledge Gap Illusion
Sometimes we mistake unfamiliarity with a specific problem type for a lack of fundamental knowledge. When facing a new algorithmic challenge, your brain might signal “I don’t know how to solve this” when what it really means is “I haven’t seen this specific pattern before.”
This illusion makes us believe we need to learn more before attempting the problem, when in reality we already possess the building blocks necessary for a solution.
Common Patterns in Problem Solving Paralysis
Let’s examine some typical scenarios where programmers get stuck at the starting line:
The Blank Editor Syndrome
You read the problem statement multiple times, understand what’s being asked, but still can’t type the first line of code. You might start thinking about various approaches, but none seem complete enough to begin implementing.
The Optimization Trap
You immediately recognize that a brute force solution exists, but you’re so focused on finding the optimal solution that you refuse to start with the simpler approach. This pursuit of optimization before implementation often leads to complete inaction.
The Framework Freeze
You’ve learned about various algorithmic patterns (two pointers, sliding window, dynamic programming, etc.), but when facing a new problem, you spend too much time trying to categorize it rather than exploring the problem space.
The Loop of Doubt
You start implementing a solution, second guess yourself, delete it, try another approach, doubt that one too, and eventually find yourself back at square one with nothing written.
Why Starting Is the Hardest Part
In programming, as in many disciplines, beginning is often the most challenging hurdle. Here’s why:
The Blank Canvas Problem
Artists often speak of the intimidation of a blank canvas, and programmers face the same with an empty code editor. Without constraints or direction, the infinite possibilities can be paralyzing rather than liberating.
Mental Models Take Time to Form
Our brains need time to construct a mental model of a problem. The initial reading rarely provides a complete understanding, but many programmers expect immediate clarity before proceeding.
The Illusion of Linear Problem Solving
We often expect problem solving to be a linear process: understand, plan, implement, test. In reality, it’s highly iterative. Understanding deepens through implementation attempts, not just passive reading.
Practical Strategies to Overcome Starting Paralysis
Now that we understand the barriers, let’s explore concrete techniques to overcome the initial paralysis when facing new coding problems.
1. Start with Examples and Edge Cases
Before writing any code, work through the provided examples manually. Trace the expected output given the input, and understand why that output is correct. Then, create additional examples, especially edge cases:
- Empty inputs
- Single element inputs
- Extremely large inputs
- Negative numbers (if applicable)
- Duplicates
- Minimum and maximum possible values
This process helps you build intuition about the problem and often reveals patterns or special considerations.
2. Verbalize the Problem
Explain the problem out loud as if teaching it to someone else. This technique, often called “rubber duck debugging,” forces you to articulate your understanding and can reveal gaps or insights you missed when reading silently.
Try answering these questions verbally:
- What are the inputs and their constraints?
- What is the expected output format?
- What are the key relationships between inputs and outputs?
- What special cases need consideration?
3. Write Pseudocode First
Instead of diving straight into implementation, start with high level pseudocode. This removes the pressure of syntax correctness and lets you focus purely on the logical flow.
// Example pseudocode for a two sum problem
function twoSum(nums, target):
For each number at index i:
For each number at index j (where j > i):
If nums[i] + nums[j] equals target:
Return [i, j]
Return no solution found
This approach breaks the problem into manageable steps without getting bogged down in language specific details.
4. Embrace the Brute Force Solution
Many programmers skip implementing the brute force solution because they know it’s inefficient. This is a mistake. Starting with brute force:
- Confirms your understanding of the problem
- Provides a working reference implementation
- Often reveals insights that lead to optimizations
- Gives you something to optimize rather than nothing at all
Remember, a suboptimal working solution is infinitely better than no solution.
5. Use the Input/Output Method
Focus on transforming the input into the output step by step. For each step, ask:
- What do I have now? (current state of data)
- What do I need next? (next transformation)
- How do I get there? (operation needed)
This incremental approach breaks down the intimidating gap between raw input and final output into manageable steps.
6. Start with Data Structure Selection
Sometimes choosing the right data structure is half the battle. Ask yourself:
- Do I need fast lookups? (Consider hash maps/sets)
- Do I need ordered data? (Consider arrays, sorted arrays, or trees)
- Do I need to track frequencies? (Consider hash maps)
- Do I need to process elements in a specific order? (Consider queues or stacks)
Once you’ve selected appropriate data structures, the algorithm often becomes more apparent.
7. Solve a Simpler Version First
If the problem seems too complex, solve a simplified version first:
- Reduce the dimensions (e.g., 1D array instead of 2D)
- Solve for smaller input sizes
- Remove constraints temporarily
- Solve a special case before the general case
This technique builds confidence and creates stepping stones toward the full solution.
Practical Example: Breaking Down a Problem
Let’s walk through a typical coding problem to demonstrate these techniques in action:
Problem: Given a string s, find the length of the longest substring without repeating characters.
Example: Input: “abcabcbb” Output: 3 (The answer is “abc”, with a length of 3)
Step 1: Explore Examples
Let’s work through the given example manually:
- “a” – length 1, no repeats
- “ab” – length 2, no repeats
- “abc” – length 3, no repeats
- “abca” – has a repeat ‘a’, so we stop at “abc” with length 3
Let’s try more examples:
- Empty string “” → output: 0
- Single character “a” → output: 1
- All same characters “aaaa” → output: 1
- No repeats “abcdef” → output: 6
Step 2: Verbalize the Problem
“I need to find the longest substring without any repeating characters. A substring is a contiguous sequence of characters. For each possible substring, I need to check if it contains any repeated characters, and if not, track its length. The answer is the maximum length found.”
Step 3: Write Pseudocode
function lengthOfLongestSubstring(s):
If s is empty, return 0
maxLength = 0
For each starting position i in s:
Create an empty set to track characters
For each position j starting from i:
If s[j] is already in the set:
Break the inner loop
Add s[j] to the set
Update maxLength if current substring length is larger
Return maxLength
Step 4: Implement the Brute Force Solution
function lengthOfLongestSubstring(s) {
if (s.length === 0) return 0;
let maxLength = 0;
for (let i = 0; i < s.length; i++) {
const charSet = new Set();
for (let j = i; j < s.length; j++) {
if (charSet.has(s[j])) {
break;
}
charSet.add(s[j]);
maxLength = Math.max(maxLength, j - i + 1);
}
}
return maxLength;
}
Step 5: Optimize
Now that we have a working solution, we can identify inefficiencies. The brute force approach rechecks many of the same substrings. We can use a sliding window approach instead:
function lengthOfLongestSubstring(s) {
if (s.length === 0) return 0;
let maxLength = 0;
let left = 0;
const charMap = new Map(); // Maps character to its index
for (let right = 0; right < s.length; right++) {
const currentChar = s[right];
// If we've seen this character before and it's within our current window
if (charMap.has(currentChar) && charMap.get(currentChar) >= left) {
// Move left pointer to position after the previous occurrence
left = charMap.get(currentChar) + 1;
}
// Update the character's position
charMap.set(currentChar, right);
// Update max length
maxLength = Math.max(maxLength, right - left + 1);
}
return maxLength;
}
This optimized solution has O(n) time complexity compared to the O(n²) of the brute force approach.
Overcoming Common Obstacles in Problem Solving
Even with the strategies above, you may encounter specific obstacles that block your progress. Here's how to address them:
When You Can't Visualize the Solution
Some problems are difficult to solve because they're hard to visualize. Try these techniques:
- Draw it out: Use pen and paper to sketch the problem, data structures, or algorithm steps
- Use physical objects: For array or list problems, use cards or sticky notes to represent elements
- Trace with tables: Create a table to track variable changes through iterations
Visual representations externalize the problem, reducing the cognitive load and making patterns more apparent.
When You Know Multiple Approaches But Can't Decide
Sometimes the problem isn't lack of ideas but too many competing approaches:
- List pros and cons: Write down the advantages and disadvantages of each approach
- Consider constraints: Which approach best addresses the given constraints?
- Start with simplicity: Choose the approach that's easiest to implement correctly
- Time box exploration: Give yourself a fixed time to explore each approach
Remember that in real world engineering, it's often better to implement a good solution now than to spend excessive time seeking the perfect solution.
When You Keep Getting Stuck on Implementation Details
Sometimes the high level approach is clear, but you get bogged down in implementation:
- Modularize: Break the solution into smaller helper functions
- Stub first: Write function signatures with comments before implementation
- Focus on critical sections: Implement the core algorithm first, then handle edge cases
- Use pseudocode as scaffolding: Convert pseudocode to real code one line at a time
Building a Systematic Approach to Problem Solving
Beyond tackling individual problems, developing a systematic approach will help you start confidently with any new challenge:
The UMPIRE Framework
One popular framework for technical interview preparation is UMPIRE:
- Understand: Clarify the problem and inputs/outputs
- Match: Identify similar problems or patterns you've seen before
- Plan: Outline your approach before coding
- Implement: Write clean, readable code
- Review: Check for bugs and edge cases
- Evaluate: Analyze time and space complexity
This structured approach ensures you don't skip important steps in the problem solving process.
Building Your Pattern Recognition Library
As you solve more problems, categorize them by pattern rather than by specific details. Common algorithmic patterns include:
- Two pointers
- Sliding window
- Binary search
- Breadth first search
- Depth first search
- Dynamic programming
- Greedy algorithms
- Backtracking
When you encounter a new problem, ask yourself which pattern it most closely resembles before diving into implementation.
Deliberate Practice Techniques
Improve your ability to start problems confidently through:
- Time constrained practice: Give yourself a fixed time (5-10 minutes) to start a solution
- Explanation practice: After reading a problem, immediately explain your approach out loud
- Variation practice: Solve the same problem with different constraints or data structures
The Role of Mental Models in Problem Solving
Developing strong mental models is crucial for quickly understanding and starting new problems.
What Are Mental Models?
Mental models are frameworks or representations that help us understand how things work. In programming, they help us visualize data structures, algorithms, and problem patterns.
Key Mental Models for Algorithmic Problem Solving
- State and transitions: Viewing problems as states that transform through operations
- Graph perspectives: Seeing relationships between elements as nodes and edges
- Recurrence relations: Breaking problems into smaller subproblems
- Invariants: Identifying properties that remain unchanged through transformations
When you encounter a new problem, try applying these mental models to see which provides clarity.
Building New Mental Models
After solving a problem, reflect on what made it challenging initially and how you eventually approached it. This reflection helps build new mental models that will serve you in future problems.
The Mindset Shift: From Paralysis to Progress
Beyond techniques and frameworks, overcoming starting paralysis requires fundamental mindset shifts:
Embracing Imperfection
Accept that your first attempt will likely be imperfect. Programming is an iterative process, and the path to an elegant solution often passes through inelegant attempts.
Viewing Problems as Exploration
Instead of seeing a problem as a test to pass, view it as a territory to explore. Each attempt, even unsuccessful ones, provides valuable information about the problem space.
The Growth Mindset in Programming
Embrace challenges as opportunities to develop your skills rather than as judgments of your ability. The feeling of not knowing where to start is not a sign of incompetence but an invitation to grow.
Practical Exercises to Improve Your Starting Ability
Here are some exercises specifically designed to improve your ability to start new problems:
Exercise 1: The Five Minute Start
For any new problem:
- Set a timer for 5 minutes
- Within this time, you must write something related to the solution
- This could be pseudocode, example traces, or even just the function signature
This exercise builds the habit of taking immediate action rather than overthinking.
Exercise 2: Multiple Approaches Practice
For a single problem:
- List three different approaches to solving it
- For each approach, write 2-3 sentences about its pros and cons
- Implement the approach that seems most promising
This develops your ability to see problems from multiple angles.
Exercise 3: Decomposition Challenge
For a complex problem:
- Break it down into at least 5 smaller subproblems or steps
- Write pseudocode for each step
- Implement each step one by one
This improves your ability to tackle complex problems by breaking them into manageable pieces.
Leveraging Tools and Resources
Don't hesitate to use appropriate tools and resources to help you get started:
Algorithmic Templates
Having templates for common algorithms can help you start quickly. For example, having a basic template for breadth first search or binary search saves time and reduces cognitive load.
Interactive Visualization Tools
Tools like algorithm visualizers can help you understand how different approaches work, making it easier to select an appropriate starting point.
AI Assisted Problem Solving
Modern AI tools can provide hints, suggest approaches, or help you understand problem statements. While you shouldn't rely on them for complete solutions, they can help overcome initial paralysis.
Conclusion: The Art of Beginning
The ability to start confidently on new programming problems is a skill that improves with practice and mindful approach. Remember that even the most experienced programmers face moments of uncertainty when confronting unfamiliar challenges.
The key insights to take away are:
- Starting is often the hardest part of problem solving
- Breaking problems down into smaller pieces makes them more approachable
- Having a systematic approach reduces anxiety and uncertainty
- Brute force solutions are valuable stepping stones to optimized approaches
- The right mindset is as important as technical knowledge
By implementing the strategies discussed in this article, you'll find yourself more capable of tackling new problems, less likely to experience that paralyzing blank mind feeling, and better equipped to grow as a programmer.
Remember that problem solving is not just about finding answers but about developing a process that works for you. Each problem you start, regardless of whether you immediately solve it, builds your capacity to face the next challenge with greater confidence and clarity.
So the next time you face a blank editor and a challenging problem, you'll know exactly where to begin.