Why You Don’t Know When Your Solution Is Good Enough
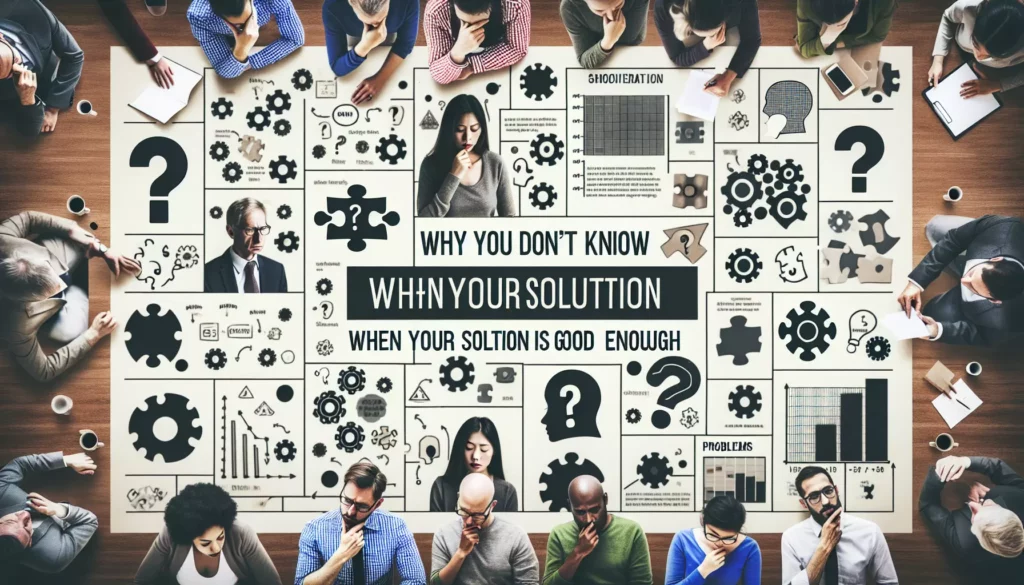
In the world of programming and software development, a common challenge many developers face is determining when their solution is “good enough.” Whether you’re a beginner learning to code or an experienced developer preparing for technical interviews at top tech companies, the question of solution adequacy plagues us all.
This uncertainty isn’t just about perfectionism; it reflects a deeper challenge in software development: balancing theoretical ideals with practical constraints. In this article, we’ll explore why determining when a solution is good enough is so difficult and provide practical strategies to help you make this assessment with confidence.
The Elusive Definition of “Good Enough”
What makes a coding solution “good enough”? The answer varies widely depending on context, but generally encompasses several key factors:
- Correctness: Does the solution solve the problem accurately for all valid inputs?
- Efficiency: Does it use computational resources (time and space) optimally?
- Readability: Can other developers (or your future self) understand the code?
- Maintainability: How easily can the code be modified or extended?
- Robustness: Does it handle edge cases and invalid inputs gracefully?
The challenge is that these factors often compete with each other. A highly optimized solution might sacrifice readability. A beautifully elegant solution might fail on edge cases. This creates an inherent tension that makes it difficult to know when to stop improving your code.
The Psychology Behind the Uncertainty
Imposter Syndrome and Perfectionism
Many developers suffer from imposter syndrome, the persistent feeling that they’re not as competent as others perceive them to be. This psychological phenomenon can manifest as an endless cycle of tweaking and optimizing code, driven by the fear that anything less than perfect will expose them as frauds.
Perfectionism compounds this problem. The desire to create flawless code can lead to diminishing returns, where hours are spent optimizing aspects of the solution that provide minimal real-world benefit.
The Dunning-Kruger Effect
The Dunning-Kruger effect describes a cognitive bias where people with limited knowledge in a domain overestimate their competence, while those with more expertise tend to underestimate their abilities. In programming, this often manifests as:
- Beginners who write inefficient or problematic code but believe it’s excellent
- Experienced developers who are painfully aware of the limitations and edge cases their solution might not address
This cognitive bias creates a situation where, paradoxically, the more you learn about programming, the less confident you might feel about your solutions.
Technical Factors That Create Uncertainty
The Optimization Spectrum
Every coding problem exists on a spectrum of optimization possibilities. Consider a simple algorithm to find the maximum value in an array:
function findMax(arr) {
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
This solution has O(n) time complexity, which is optimal for this problem. But even here, you might wonder:
- Should I add input validation?
- What if the array is empty?
- Could I make it more efficient for specific data distributions?
- Should I optimize for readability by using array methods instead?
For more complex problems, these questions multiply exponentially.
Theoretical vs. Practical Efficiency
Computer science education often emphasizes Big O notation and theoretical efficiency. While these concepts are crucial, they sometimes create a disconnect between theoretical and practical performance.
For example, an algorithm with O(n log n) time complexity might outperform an O(n) algorithm for small inputs due to lower constant factors or better cache utilization. This discrepancy can leave developers uncertain about whether their theoretically optimal solution is actually the best choice in practice.
The Moving Target of Requirements
Software requirements frequently evolve, making “good enough” a moving target. A solution that perfectly addresses today’s needs might be inadequate tomorrow. This reality creates anxiety about future proofing code, leading to questions like:
- Should I implement a more flexible solution now, even if it’s more complex?
- Am I over-engineering this solution for requirements that might never materialize?
- Will this approach scale if the input size grows dramatically?
The Context-Dependent Nature of “Good Enough”
Interview Settings vs. Production Code
The definition of “good enough” varies dramatically between contexts. In a technical interview at a top tech company, the emphasis might be on:
- Algorithmic efficiency
- Clean, bug-free implementation
- Clear communication of your approach
- Consideration of edge cases
In contrast, production code might prioritize:
- Maintainability and readability
- Integration with existing systems
- Robustness and error handling
- Performance under specific workloads
This contextual shift means that a solution that’s “good enough” in one setting might be inadequate in another.
The Scale Factor
Scale dramatically affects what constitutes a good enough solution. An algorithm that works perfectly for 100 users might crumble under the load of 1 million. Consider this naive approach to finding duplicates in an array:
function findDuplicates(arr) {
const duplicates = [];
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] === arr[j] && !duplicates.includes(arr[i])) {
duplicates.push(arr[i]);
}
}
}
return duplicates;
}
This O(n²) solution is perfectly adequate for small arrays but becomes prohibitively slow for large datasets. The question becomes: how large might your input grow in the future?
Business Constraints
In professional settings, business constraints significantly influence what’s considered “good enough”:
- Deadlines: Sometimes a working solution now is better than a perfect solution later
- Resource limitations: Engineering time is finite and expensive
- Risk tolerance: Some applications (like medical devices) require higher standards than others
These constraints create a pragmatic definition of “good enough” that often differs from academic or theoretical ideals.
Common Scenarios Where Developers Struggle
Algorithmic Puzzles and Coding Challenges
Platforms like LeetCode, HackerRank, and AlgoCademy present algorithmic puzzles that often have multiple valid solutions. The uncertainty usually revolves around:
- Is my O(n log n) solution good enough, or should I strive for the O(n) solution?
- Have I handled all the edge cases?
- Is my code clean and readable enough?
- Could there be an even more optimal approach I’m missing?
This uncertainty is particularly acute when preparing for technical interviews, where candidates want to demonstrate their best possible work.
System Design Decisions
System design presents even greater uncertainty because the solution space is vast and the tradeoffs complex. When designing a distributed system, questions abound:
- Is this architecture scalable enough?
- Have I considered all possible failure modes?
- Is this over-engineered for the current requirements?
- Am I making the right technology choices?
The lack of immediate feedback makes these decisions particularly challenging, as the consequences might not be apparent until months or years later.
Refactoring Existing Code
When refactoring, the question of “good enough” becomes especially tricky. Developers must balance improvement against the risk of introducing new bugs:
- How much of this legacy code should I refactor?
- Is this clean enough, or should I continue restructuring?
- Am I making meaningful improvements or just changing code to match my personal preferences?
The absence of clear metrics for “better” code makes these judgments largely subjective.
Strategies for Determining When Your Solution Is Good Enough
Define Success Criteria Before You Start
One of the most effective ways to combat uncertainty is to define success criteria before you begin coding. This might include:
- Time and space complexity requirements
- Expected edge cases to handle
- Performance benchmarks
- Code quality standards
By establishing these criteria upfront, you create an objective measure of “good enough” that can guide your development process and help you recognize when you’ve reached your goal.
Utilize Test-Driven Development
Test-driven development (TDD) provides a structured approach to determining when your solution is complete. By writing tests before implementing the solution, you create a clear definition of what constitutes correct behavior:
// Example of a test-driven approach
function testFindMax() {
// Test normal case
assert(findMax([1, 3, 5, 2, 4]) === 5);
// Test negative numbers
assert(findMax([-1, -3, -5]) === -1);
// Test single element
assert(findMax([42]) === 42);
// Test empty array
try {
findMax([]);
assert(false); // Should not reach here
} catch (error) {
assert(error.message === "Array cannot be empty");
}
console.log("All tests passed!");
}
When all tests pass, you have concrete evidence that your solution meets the specified requirements.
Apply the 80/20 Rule
The Pareto principle, or 80/20 rule, suggests that 80% of the value comes from 20% of the effort. Applied to coding, this means:
- Focus first on core functionality and common cases
- Recognize diminishing returns in optimization
- Be strategic about which edge cases warrant handling
By prioritizing the most impactful aspects of your solution, you can achieve a “good enough” state more efficiently.
Seek External Validation
Sometimes, the best way to determine if your solution is good enough is to get feedback from others:
- Code reviews from peers or mentors
- User testing for application features
- Performance benchmarking against established solutions
- Automated code quality tools
External perspectives can highlight blind spots in your evaluation and provide confidence that your solution meets acceptable standards.
Use Concrete Metrics
Whenever possible, use quantifiable metrics to evaluate your solution:
- Execution time on representative inputs
- Memory usage
- Code coverage percentage
- Static analysis scores
These objective measures provide a clearer picture of solution quality than subjective assessment alone.
Case Study: Evaluating a Search Algorithm Solution
Let’s apply these principles to a common interview problem: implementing a binary search algorithm. Here’s a typical implementation:
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (arr[mid] === target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Target not found
}
Is this solution good enough? Let’s evaluate:
Correctness Assessment
The solution correctly implements binary search with O(log n) time complexity, which is optimal for this problem. However, there are several considerations:
- The solution assumes the array is already sorted
- It doesn’t validate that the input is an array
- The calculation of mid could cause integer overflow in languages like Java (though not in JavaScript)
Edge Case Analysis
We should test the solution with various edge cases:
- Empty array
- Array with a single element
- Target at the beginning or end of the array
- Target not present in the array
- Array with duplicate elements
Optimization Considerations
While the time complexity is optimal, we could improve the solution by:
- Adding input validation
- Fixing the potential integer overflow issue
- Making it more robust for different types of comparable elements
An improved version might look like:
function binarySearch(arr, target) {
// Input validation
if (!Array.isArray(arr)) {
throw new Error("Input must be an array");
}
let left = 0;
let right = arr.length - 1;
while (left <= right) {
// Avoid integer overflow
const mid = left + Math.floor((right - left) / 2);
if (arr[mid] === target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Target not found
}
Contextual Evaluation
Whether this solution is “good enough” depends on the context:
- For a coding interview: The improved version demonstrates attention to detail and edge cases, which would likely impress interviewers
- For production code: You might want additional robustness, such as type checking or handling for custom comparison functions
- For an educational example: The simpler version might be preferable to illustrate the core concept without distractions
The Role of Experience in Recognizing “Good Enough”
Pattern Recognition
Experienced developers build a mental library of patterns and anti-patterns that help them recognize when a solution is adequate or problematic. This pattern recognition operates almost subconsciously, allowing seasoned programmers to quickly identify:
- Potential performance bottlenecks
- Maintainability issues
- Common edge cases
- Architectural red flags
This intuitive understanding develops through years of writing, reviewing, and maintaining code across various contexts.
Learning from Past Mistakes
Nothing teaches the meaning of “good enough” like experiencing the consequences of solutions that weren’t. Developers who have:
- Debugged production failures at 2 AM
- Maintained legacy codebases with poor design
- Scaled systems beyond their initial design parameters
- Fixed security vulnerabilities in existing code
These experiences create a visceral understanding of what constitutes an adequate solution in different contexts.
Mentorship and Knowledge Transfer
Mentorship accelerates the development of this judgment. By working with experienced developers, newcomers can absorb wisdom about:
- Which optimizations matter in practice
- How to balance competing concerns
- When to stop refining a solution
- What level of quality is appropriate for different situations
This knowledge transfer helps bridge the gap between theoretical understanding and practical judgment.
Embracing Uncertainty as Part of the Process
The Iterative Nature of Software Development
Software development is inherently iterative. Rather than viewing “good enough” as a final state, consider it a checkpoint in an ongoing process of improvement. This perspective allows you to:
- Ship working solutions that meet current needs
- Gather feedback from real-world usage
- Make informed improvements based on actual requirements
- Balance theoretical ideals with practical constraints
By embracing this iterative approach, you can make peace with the uncertainty of “good enough” and focus on continuous improvement.
Balancing Perfectionism with Pragmatism
Finding a healthy balance between perfectionism and pragmatism is essential for productive development. This balance involves:
- Recognizing when you’re in the zone of diminishing returns
- Understanding the real-world impact of further optimizations
- Being honest about the constraints of time, resources, and requirements
- Accepting that all code represents tradeoffs
This balanced approach allows you to create solutions that are good enough for their intended purpose without falling into the trap of endless optimization.
Conclusion: Developing Your Personal “Good Enough” Compass
The ability to recognize when a solution is good enough is a skill that develops over time through a combination of technical knowledge, practical experience, and self-awareness. While there’s no universal definition of “good enough,” you can develop your personal compass by:
- Clearly defining success criteria for each project or problem
- Seeking feedback from peers and mentors
- Reflecting on past successes and failures
- Considering the specific context and constraints of each situation
- Using objective metrics whenever possible
- Recognizing the signs of diminishing returns
Remember that the goal isn’t perfection but appropriateness. A solution that meets its requirements, performs efficiently within its constraints, and can be maintained by its team is often good enough, even if theoretical improvements are possible.
By developing this judgment, you’ll not only become a more effective developer but also experience greater satisfaction in your work, knowing that you’re making rational decisions about when to ship, when to optimize, and when to move on to the next challenge.
The uncertainty about whether your solution is good enough never completely disappears, even for the most experienced developers. But with time and practice, you’ll learn to navigate this uncertainty with confidence, using it as a tool for growth rather than a source of anxiety.