Why You Can’t Write Code Without Examples: The Essential Role of Learning by Example
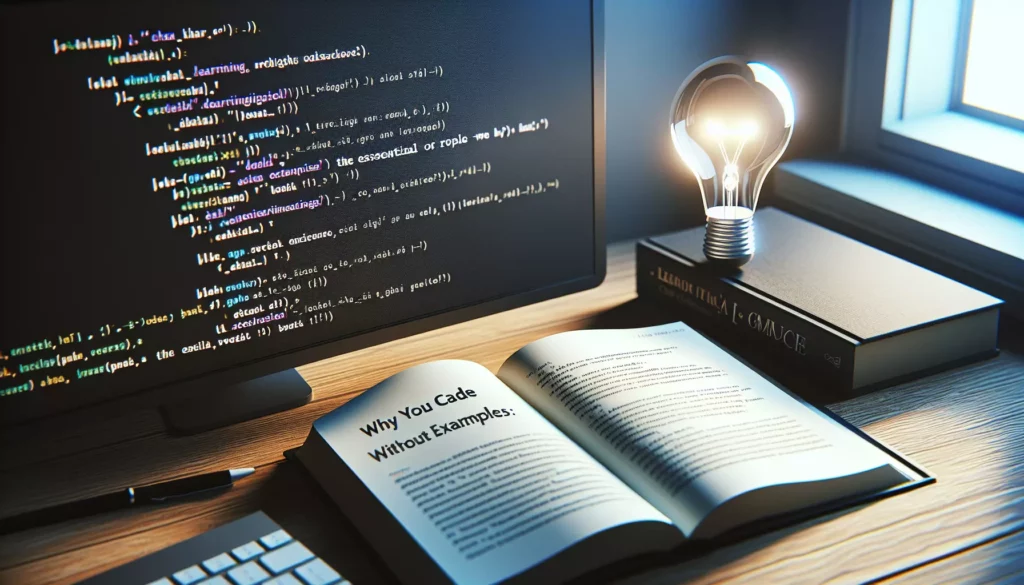
In the world of programming and software development, one truth stands out among the rest: you simply cannot become proficient at coding without studying examples. Whether you’re a complete beginner taking your first steps into the world of programming or an experienced developer tackling a new language or framework, examples serve as the bridge between abstract concepts and practical implementation.
At AlgoCademy, we’ve observed thousands of students in their coding journey, and the pattern is unmistakable. Those who immerse themselves in well crafted examples progress faster, develop stronger problem solving skills, and ultimately become more capable programmers. Let’s explore why examples are not just helpful but essential to coding education.
The Science Behind Learning Through Examples
Learning to code is fundamentally different from many other types of learning. When you write code, you’re not just memorizing facts; you’re developing a new way of thinking. Cognitive science research supports the idea that example based learning is particularly effective for coding education.
Cognitive Load Theory and Programming
According to cognitive load theory, our working memory has limited capacity. When learning to code, beginners must simultaneously grasp:
- Syntax rules of the programming language
- Programming concepts and structures
- Problem solving approaches
- Debugging strategies
- The specific problem domain they’re working in
Without examples, this cognitive load quickly becomes overwhelming. Examples break down complex tasks into manageable chunks that our brains can process more effectively.
Research from the University of Michigan found that students who studied worked examples before attempting to solve programming problems outperformed those who jumped straight into problem solving. The example first group not only completed tasks faster but also developed more accurate mental models of how programming works.
Schema Building in Programming
Examples help programmers build what cognitive scientists call “schemas” or mental frameworks for understanding code. When you examine multiple examples of loops, conditionals, or data structures, your brain begins to recognize patterns and create generalizable knowledge structures.
These schemas become the foundation for problem solving. An experienced programmer can look at a new problem and think, “This looks similar to that sorting algorithm I studied” or “I can adapt that authentication example I worked through last week.”
How Examples Bridge Theory and Practice
Programming textbooks and documentation often explain concepts in abstract terms. While this theoretical knowledge is important, it’s insufficient for developing practical coding skills.
Examples Demonstrate Practical Implementation
Consider a beginner learning about arrays. They might read: “An array is a data structure that stores a collection of elements in contiguous memory locations, accessible by index.”
While technically correct, this definition does little to help someone actually use arrays. Compare that to seeing this example:
// Creating and using an array in JavaScript
let fruits = ['apple', 'banana', 'orange'];
// Accessing elements
console.log(fruits[0]); // Outputs: apple
// Adding an element
fruits.push('grape');
console.log(fruits); // Outputs: ['apple', 'banana', 'orange', 'grape']
// Iterating through an array
for (let i = 0; i < fruits.length; i++) {
console.log(`Fruit ${i+1}: ${fruits[i]}`);
}
This example shows not just what an array is, but how to create one, access its elements, modify it, and iterate through it. The abstract concept suddenly becomes concrete and usable.
Examples Reveal Hidden Assumptions
Documentation often contains hidden assumptions that are only revealed through examples. For instance, a function description might not explicitly mention that a parameter must be in a specific format or that certain edge cases produce unexpected results.
Well crafted examples expose these nuances by showing:
- Expected inputs and outputs
- Common edge cases
- Error handling approaches
- Typical usage patterns
The Four Levels of Example Based Learning
At AlgoCademy, we've identified four progressive levels of learning through examples that help coders develop from novices to experts:
1. Reading and Understanding Examples
The journey begins with reading code examples and understanding how they work. This might involve:
- Following code execution line by line
- Understanding how variables change throughout the program
- Identifying key programming constructs used
- Recognizing the purpose of each section of code
For beginners, even this level requires significant effort. Consider this simple Python example:
def calculate_average(numbers):
if not numbers:
return 0
return sum(numbers) / len(numbers)
scores = [85, 92, 78, 90, 88]
average = calculate_average(scores)
print(f"The average score is: {average}")
A beginner needs to understand function definitions, conditional statements, list operations, and string formatting just to follow this short example.
2. Modifying Existing Examples
The next level involves taking existing examples and modifying them to solve slightly different problems. This might include:
- Changing variable values to see how output changes
- Adding new features to an existing program
- Fixing bugs in sample code
- Adapting an algorithm to work with different data types
For instance, a learner might modify the above average calculation function to also return the minimum and maximum values:
def analyze_numbers(numbers):
if not numbers:
return {'average': 0, 'min': None, 'max': None}
return {
'average': sum(numbers) / len(numbers),
'min': min(numbers),
'max': max(numbers)
}
scores = [85, 92, 78, 90, 88]
results = analyze_numbers(scores)
print(f"Analysis: Average={results['average']}, Min={results['min']}, Max={results['max']}")
This modification requires understanding the original code plus knowledge of dictionaries and additional built in functions.
3. Combining Multiple Examples
As skills advance, programmers learn to combine patterns and techniques from multiple examples to solve more complex problems.
Imagine a learner has previously studied examples of:
- Reading data from files
- Calculating statistics on numerical data
- Creating simple visualizations
They might combine these skills to create a program that reads test scores from a CSV file, calculates various statistics, and generates a visual report:
import csv
import matplotlib.pyplot as plt
def read_scores_from_file(filename):
scores = []
with open(filename, 'r') as file:
reader = csv.reader(file)
next(reader) # Skip header row
for row in reader:
scores.append(int(row[1])) # Assume second column contains scores
return scores
def analyze_scores(scores):
return {
'average': sum(scores) / len(scores),
'min': min(scores),
'max': max(scores),
'passing': sum(1 for score in scores if score >= 60),
'failing': sum(1 for score in scores if score < 60)
}
def visualize_results(scores, analysis):
plt.figure(figsize=(10, 6))
# Create histogram of scores
plt.subplot(1, 2, 1)
plt.hist(scores, bins=10, color='skyblue')
plt.title('Distribution of Scores')
plt.xlabel('Score')
plt.ylabel('Frequency')
# Create pie chart of passing/failing
plt.subplot(1, 2, 2)
plt.pie([analysis['passing'], analysis['failing']],
labels=['Passing', 'Failing'],
autopct='%1.1f%%',
colors=['green', 'red'])
plt.title('Pass/Fail Rate')
plt.tight_layout()
plt.savefig('score_analysis.png')
plt.show()
# Main program
scores = read_scores_from_file('class_scores.csv')
analysis = analyze_scores(scores)
print(f"Class Statistics:")
print(f"Average: {analysis['average']:.2f}")
print(f"Range: {analysis['min']} to {analysis['max']}")
print(f"Passing: {analysis['passing']} students")
print(f"Failing: {analysis['failing']} students")
visualize_results(scores, analysis)
This more complex program demonstrates how multiple example based skills can combine to create something more sophisticated.
4. Creating Novel Solutions
The highest level of example based learning is the ability to create entirely new solutions by applying patterns and principles from previously studied examples to novel problems.
At this stage, programmers don't just copy examples; they internalize the underlying principles and can apply them creatively. The examples have become part of their problem solving toolkit.
Common Misconceptions About Learning From Examples
Despite the clear benefits, some misconceptions about learning from examples persist in programming education:
Misconception 1: "Studying Examples Is Just Copying"
Some believe that looking at examples promotes mere copying rather than understanding. However, effective example based learning involves active engagement:
- Tracing code execution mentally
- Predicting outputs before running code
- Analyzing why certain approaches were chosen
- Identifying the principles that make the code work
When done properly, studying examples is not passive copying but active learning that builds deep understanding.
Misconception 2: "Real Programmers Figure Things Out From Scratch"
There's a harmful myth that "real programmers" should be able to figure everything out from first principles, without looking at examples. This couldn't be further from the truth.
Professional developers constantly refer to examples, whether from documentation, Stack Overflow, or their own previous work. In fact, the ability to find, evaluate, and adapt examples is a critical professional skill.
Google's own research on developer productivity found that their most effective engineers regularly leverage existing code examples rather than reinventing solutions.
Misconception 3: "Examples Only Help Beginners"
While beginners benefit enormously from examples, even the most experienced developers rely on them when:
- Learning new languages or frameworks
- Working with unfamiliar APIs
- Implementing complex algorithms
- Optimizing performance
- Following best practices in new domains
The difference is that experienced developers can extract principles from examples more quickly and apply them more flexibly.
How Top Tech Companies Use Examples in Their Engineering Culture
Leading technology companies recognize the value of examples in maintaining high quality code and onboarding new engineers efficiently.
Google's Code Examples and Style Guides
Google maintains extensive internal documentation with canonical examples of how to implement common patterns across their codebase. Their style guides for various languages include numerous examples of both recommended and discouraged practices.
New Google engineers are explicitly encouraged to find and study examples before writing new code, ensuring consistency and quality.
Amazon's Working Backwards Approach
Amazon's famous "working backwards" product development process often begins with example implementations. Engineers might create sample code that demonstrates how a new API would be used before actually building it, ensuring the final product will meet real world needs.
Microsoft's Documentation Strategy
Microsoft has transformed its developer documentation in recent years to be heavily example centric. Their docs now feature:
- Interactive code examples that can be run directly in the browser
- Multiple examples showing different use cases for each feature
- Complete sample applications demonstrating end to end solutions
This approach has significantly improved developer adoption of Microsoft technologies.
What Makes a Good Code Example?
Not all examples are created equal. At AlgoCademy, we've identified several characteristics that make code examples particularly effective for learning:
Clarity and Readability
Good examples prioritize clarity over cleverness. They use:
- Descriptive variable and function names
- Consistent formatting and style
- Appropriate comments explaining the "why" behind complex operations
- Simple approaches where possible
Compare these two examples that accomplish the same task:
// Unclear example
function f(a) {
return a.filter(x => x % 2).map(x => x * x).reduce((a, b) => a + b, 0);
}
// Clear example
function sumOfSquaredOddNumbers(numbers) {
// Filter to keep only odd numbers
const oddNumbers = numbers.filter(number => number % 2 !== 0);
// Square each odd number
const squaredOddNumbers = oddNumbers.map(number => number * number);
// Sum all the squared values
const sum = squaredOddNumbers.reduce((total, current) => total + current, 0);
return sum;
}
While the first example is more concise, the second is far more valuable for learning.
Progression from Simple to Complex
The best examples start simple and progressively add complexity. For instance, when teaching API requests, an effective sequence might be:
- Making a basic GET request to a public API
- Adding error handling
- Incorporating query parameters
- Adding authentication
- Implementing more complex patterns like pagination or rate limiting
Each example builds on the previous one, allowing learners to understand each new concept without being overwhelmed.
Real World Relevance
Examples that solve realistic problems are more engaging and better prepare learners for actual development work. Instead of generic examples like calculating factorials, effective learning materials might show:
- How to validate user input in a registration form
- Processing data from a CSV file to generate a report
- Building a simple API that interacts with a database
- Creating a responsive UI component
Multiple Approaches to the Same Problem
Showing different ways to solve the same problem helps learners understand tradeoffs and develop flexibility. For instance, when teaching how to find the maximum value in an array, you might show:
// Approach 1: Using a for loop
function findMaxWithLoop(numbers) {
if (numbers.length === 0) return null;
let max = numbers[0];
for (let i = 1; i < numbers.length; i++) {
if (numbers[i] > max) {
max = numbers[i];
}
}
return max;
}
// Approach 2: Using Math.max with spread syntax
function findMaxWithMathMax(numbers) {
if (numbers.length === 0) return null;
return Math.max(...numbers);
}
// Approach 3: Using array reduce
function findMaxWithReduce(numbers) {
if (numbers.length === 0) return null;
return numbers.reduce((max, current) =>
current > max ? current : max, numbers[0]);
}
Each approach illustrates different programming concepts and has different performance characteristics.
How AlgoCademy Uses Examples to Accelerate Learning
At AlgoCademy, we've developed a comprehensive approach to example based learning that helps students progress from beginner to interview ready more efficiently.
Progressive Example Sequences
Our curriculum organizes examples into carefully sequenced learning paths. Each concept is introduced with:
- Introductory examples that demonstrate the core concept in isolation
- Expanded examples showing common variations and use cases
- Applied examples that use the concept in realistic scenarios
- Integration examples showing how the concept works with other techniques
This progressive approach ensures that students build a solid foundation before tackling more complex implementations.
Interactive Example Exploration
Our platform allows students to interact with examples in multiple ways:
- Running examples with different inputs to observe behavior
- Stepping through code execution line by line
- Visualizing data structures and algorithm operations
- Modifying examples and seeing immediate results
This interactivity transforms passive reading into active learning, significantly improving retention and understanding.
Example Driven Problem Solving
When students face new programming challenges, our AI powered assistant helps them find relevant examples from our extensive library. By showing similar problems with solutions, we help students bridge the gap between what they know and what they're trying to accomplish.
This approach mirrors how professional developers work, teaching not just coding syntax but the crucial skill of adapting existing patterns to new situations.
How to Maximize Learning from Code Examples
Whether you're using AlgoCademy or other resources, these strategies will help you get the most from code examples:
Active Reading Techniques
Instead of passively scanning examples, engage with them:
- Trace variables: Track how each variable changes throughout the program
- Predict outcomes: Before running code, try to predict what it will do
- Ask why: For each line, ask why the programmer made that choice
- Identify patterns: Note recurring structures and techniques
The "Recreate from Memory" Technique
After studying an example:
- Close the original example
- Try to recreate it from memory
- Compare your version with the original
- Note any differences and understand why they matter
This technique forces deeper processing of the example and reveals gaps in your understanding.
Deliberate Variation Practice
Once you understand an example, systematically vary it:
- Change input values to test edge cases
- Modify the algorithm slightly to solve a related problem
- Implement the same functionality in a different way
- Add features or optimizations to the original code
This practice builds flexibility and deepens your understanding of the underlying principles.
Building Your Personal Example Library
Professional developers maintain collections of useful code examples. Start building yours by:
- Saving examples that demonstrate important techniques
- Annotating them with your own notes about key concepts
- Organizing them by topic for easy reference
- Reviewing them periodically to reinforce your learning
Over time, this library becomes an invaluable resource for your programming journey.
From Examples to Expertise: The Journey to Coding Mastery
As you progress in your coding journey, your relationship with examples evolves:
The Beginner Phase: Learning the Basics
When you're just starting, examples provide essential models of correct code. You might:
- Follow tutorials step by step
- Make minor modifications to working code
- Focus on understanding syntax and basic structures
At this stage, examples are like training wheels providing necessary support.
The Intermediate Phase: Building Independence
As your skills grow, you'll use examples differently:
- Consulting examples when facing new challenges
- Combining techniques from multiple examples
- Recognizing patterns across different coding situations
- Adapting examples to fit your specific needs
Examples now serve as references rather than templates.
The Advanced Phase: Creating Your Own Patterns
At advanced levels, your relationship with examples transforms again:
- Evaluating examples critically based on your own expertise
- Identifying optimization opportunities in existing code
- Creating your own examples to teach others
- Developing novel solutions inspired by fundamental patterns
You've internalized so many examples that they've become part of your thinking process.
Conclusion: Embracing the Power of Examples
The evidence is clear: you simply cannot write code effectively without learning from examples. They are not crutches or shortcuts but essential tools in the learning process that even the most experienced developers rely on.
At AlgoCademy, our example centric approach accelerates learning by providing carefully crafted, progressive examples that build both understanding and practical skills. We've seen firsthand how this approach transforms struggling beginners into confident, capable programmers ready to tackle technical interviews at top tech companies.
Whether you're writing your first lines of code or preparing for a senior developer role, embracing the power of examples will make you a more effective programmer. The key is not to avoid examples but to engage with them actively, extracting the principles that make them work and applying those principles to new situations.
Remember: every expert programmer started by studying examples. The path to mastery isn't about avoiding examples; it's about learning how to use them as stepping stones to develop your own problem solving abilities.
So the next time you face a coding challenge, don't hesitate to seek out relevant examples. Study them, understand them, adapt them, and eventually, you'll internalize the patterns they demonstrate. That's not just how coding is taught; it's how it's actually done in the real world of software development.