Why You Can’t Visualize Program Flow (And How to Change That)
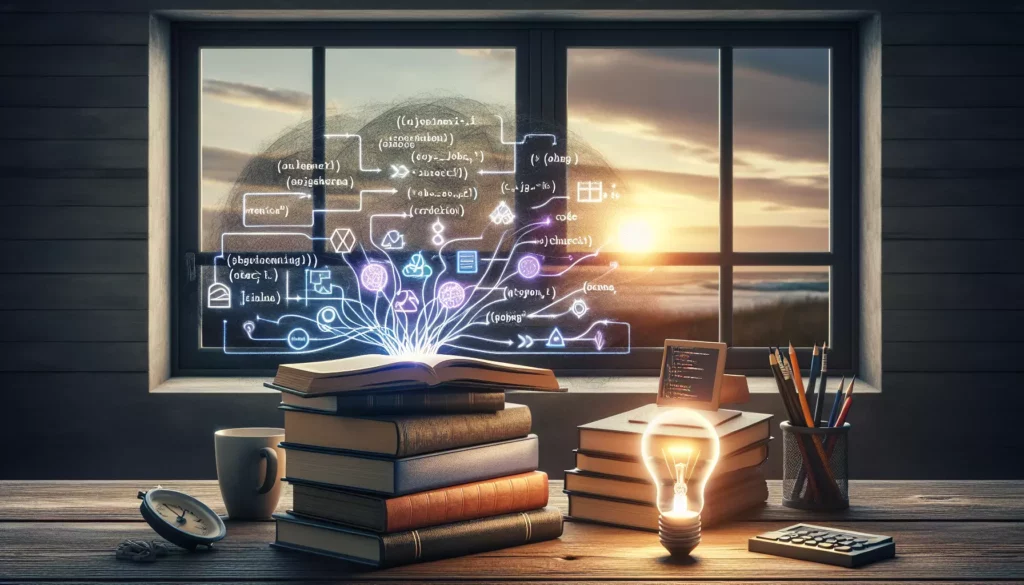
Have you ever found yourself staring at code, trying to mentally trace how it executes, only to lose track halfway through? You’re not alone. One of the most challenging aspects of programming is visualizing program flow—understanding how control moves through your code, how variables change, and how functions interact.
This mental hurdle affects everyone from beginners writing their first loops to experienced developers debugging complex systems. The reality is that our brains aren’t naturally wired to track the abstract, multi-dimensional nature of program execution.
In this article, we’ll explore why visualizing program flow is so difficult, the cognitive limitations we face, and most importantly, practical strategies to overcome these challenges and become more effective programmers.
The Invisible Nature of Program Execution
When you write code, you’re creating something inherently invisible. Unlike physical engineering where you can see a bridge being built or a circuit being connected, software execution happens in the abstract realm of memory addresses and CPU instructions.
Consider this simple Python function:
def process_data(numbers):
result = 0
for num in numbers:
if num > 0:
result += num
return result
When this code runs, there’s nothing to physically observe. You can’t “see” the loop iterating, the condition being checked, or the value of result
changing. The execution happens silently within the computer, leaving us to imagine what’s happening.
This invisibility creates a fundamental challenge: we’re trying to reason about processes we can’t directly perceive with our senses. It’s like trying to understand wind patterns without being able to see the air moving.
The Cognitive Limits of Mental Simulation
Our brains have impressive capabilities, but they also have significant limitations when it comes to simulating program execution:
Working Memory Constraints
Psychological research has established that humans typically can hold only about 4-7 items in working memory at once. Yet, understanding even a moderately complex function might require tracking:
- Multiple variable values
- The current execution point
- The call stack
- Conditional branches that might be taken
- Loop iteration state
Take this seemingly straightforward recursive function:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
Try mentally tracing fibonacci(5)
. You’ll quickly find yourself struggling to keep track of all the recursive calls, return values, and where you are in the execution tree. This isn’t because you lack intelligence or programming skill—it’s because the task exceeds normal human working memory capacity.
Non-Linear Execution
Human thinking tends to be linear, but program execution often isn’t. Code jumps around through:
- Function calls
- Conditionals and branches
- Exceptions
- Callbacks and event handlers
- Asynchronous operations
Our brains struggle to maintain context when execution suddenly jumps to a different part of the program and then needs to return to the original context.
State Explosion
As programs grow in complexity, the number of possible states grows exponentially. Even a small program with 10 boolean variables theoretically has 2^10 (1,024) possible states. No human can mentally simulate all these possibilities.
This state explosion makes it nearly impossible to mentally predict all the ways your code might behave, especially when dealing with user input, network conditions, or other external factors.
The Abstraction Paradox
Programming languages are built on layers of abstraction to make coding more manageable. While these abstractions make writing code easier, they can actually make understanding execution flow harder.
Consider this JavaScript Promise chain:
fetchUserData(userId)
.then(userData => fetchUserPosts(userData.id))
.then(posts => filterRecentPosts(posts))
.then(recentPosts => renderPosts(recentPosts))
.catch(error => handleError(error));
The clean, readable syntax masks a complex execution model involving the event loop, asynchronous operations, and callback queues. The apparent simplicity of the code doesn’t match the complexity of what’s happening under the hood.
Similarly, high-level languages shield us from memory management, pointer arithmetic, and other low-level concerns, but this means we often don’t have a clear mental model of what’s actually happening when our code runs.
The Problem with Text as a Medium
Code is traditionally written as text, but text is a one-dimensional medium trying to represent multi-dimensional processes:
- Time (sequence of operations)
- State (changing values)
- Relationships (how components interact)
- Conditions (branching possibilities)
Text forces us to read linearly, but program execution is rarely linear. Functions defined at the bottom of a file might be called from the top. A class might inherit behavior from multiple parent classes defined elsewhere.
Additionally, text doesn’t naturally show state changes. In a text-based medium, we can only see one version of the code at a time, not how variables evolve throughout execution.
Overcoming the Visualization Challenge
Now that we understand why visualizing program flow is difficult, let’s explore practical strategies to overcome these limitations and improve our mental models.
1. Leverage External Cognitive Tools
The most effective way to overcome working memory limitations is to offload some of the cognitive burden to external tools:
Debuggers: Your Program’s Microscope
Modern debuggers allow you to:
- Step through code line by line
- Inspect variable values at each step
- Set breakpoints at critical junctures
- Visualize the call stack
By using a debugger, you transform the invisible execution process into a visible, controllable experience. Instead of imagining what happens, you can observe it directly.
For example, stepping through our earlier recursive Fibonacci function with a debugger would let you see each recursive call being made and returned, making the execution tree concrete rather than abstract.
Visualization Tools
Specialized visualization tools can represent program execution graphically:
- Python Tutor (pythontutor.com) visualizes code execution step-by-step
- UML diagrams illustrate relationships between components
- Sequence diagrams show interactions between objects over time
- Flame graphs visualize call stacks and performance
These tools convert abstract processes into visual representations that align better with how our brains process information.
Logging and Print Statements
Even simple print statements can dramatically improve your understanding of program flow:
def process_data(numbers):
print(f"Starting with numbers: {numbers}")
result = 0
for i, num in enumerate(numbers):
print(f"Iteration {i}, checking number {num}")
if num > 0:
result += num
print(f" Added {num}, result is now {result}")
print(f"Final result: {result}")
return result
This technique creates a narrative of the program’s execution that you can follow, turning the invisible visible.
2. Develop Mental Models Through Chunking
Expert programmers overcome working memory limitations through chunking—grouping low-level details into higher-level concepts that take up less mental space.
Recognize Common Patterns
Instead of tracking each line of a for-loop, recognize it as “iterating through a collection.” Instead of mentally stepping through a sorting algorithm, recognize it as “sorting the data.”
Learning to identify these patterns lets you reason about code at a higher level, reducing cognitive load:
// Instead of thinking about each step
for (let i = 0; i < array.length; i++) {
result += array[i];
}
// Recognize this as "sum all elements in the array"
Build Your Programming Vocabulary
Learn to recognize and name common programming idioms and design patterns. When you see the Observer pattern or a map-reduce operation, you can think in terms of these higher-level abstractions rather than the individual lines of code.
This is similar to how chess masters don’t see individual pieces but recognize board positions and strategies as coherent wholes.
3. Use Visual Metaphors and Analogies
Our brains are naturally good at spatial reasoning and physical metaphors. Leverage this by creating visual analogies for programming concepts:
The Stack as a Physical Stack
Imagine function calls as plates stacked on top of each other. Each new function adds a plate; each return removes one. This physical metaphor makes the abstract concept of a call stack more intuitive.
Variables as Containers
Visualize variables as labeled boxes holding values. Assignment operations put new values in the boxes; reading variables looks at what’s inside.
Pointers as Arrows
In languages with references or pointers, imagine them as arrows pointing from one object to another. This makes concepts like reference passing and object mutation easier to track.
State Machines as Maps
For complex state-dependent behavior, imagine a map with different regions representing states and paths representing transitions. This spatial metaphor can help reason about state changes more effectively.
4. Decompose Complex Problems
Break down complex program flows into smaller, more manageable pieces that fit within working memory constraints:
Function Decomposition
Instead of tracing through a 100-line function, break it into 5-10 line functions with clear purposes. This allows you to reason about each piece independently before combining them.
// Hard to mentally trace
function processUserData(userData) {
// 100 lines of complex logic
}
// Easier to understand
function processUserData(userData) {
const validatedData = validateUserInput(userData);
const enrichedData = addDefaultValues(validatedData);
const normalizedData = normalizeFields(enrichedData);
return saveToDatabase(normalizedData);
}
State Isolation
Separate your program into components with well-defined state boundaries. This allows you to reason about each component’s state independently rather than tracking the entire program state at once.
Incremental Understanding
When faced with complex code, don’t try to understand everything at once. Start with the high-level structure, then progressively dive into specific components as needed. This “just-in-time” understanding approach prevents cognitive overload.
Practical Techniques for Different Programming Paradigms
Different programming paradigms present unique visualization challenges. Here are strategies tailored to common approaches:
Imperative Programming
Imperative code focuses on sequences of statements that change program state:
- State tables: Track variable values in a table, updating after each statement
- Control flow diagrams: Draw flowcharts showing branches and loops
- Code annotation: Add comments explaining state changes at critical points
int calculateTotal(int[] prices, float taxRate) {
int subtotal = 0; // State: subtotal = 0
// Loop through each price
for (int price : prices) {
subtotal += price; // State: subtotal increases with each price
}
// Apply tax
float total = subtotal * (1 + taxRate); // State: calculate final total
return Math.round(total); // Return rounded total
}
Object-Oriented Programming
OOP involves interactions between objects with their own state and behavior:
- Object diagrams: Visualize objects as boxes with properties and relationships
- Sequence diagrams: Trace method calls between objects
- State tracking by object: Focus on one object’s state changes at a time
When debugging OOP code, focus on the responsibilities of each class and the messages passed between objects rather than trying to track the entire system state.
Functional Programming
Functional programming emphasizes immutable data and function composition:
- Data transformation diagrams: Show how data flows through transformation pipelines
- Function composition trees: Visualize nested function calls as trees
- Value tracing: Track how values (not variables) transform through each function
// Visualize this as a pipeline of transformations
const result = data
.filter(item => item.active)
.map(item => item.value)
.reduce((sum, value) => sum + value, 0);
Asynchronous Programming
Asynchronous code presents special challenges due to its non-linear execution:
- Timeline diagrams: Draw parallel timelines for different asynchronous operations
- Event queue visualization: Mentally model the event loop and callback queue
- State snapshots: Capture the program state at key points like before/after async operations
For complex async code, consider using promises or async/await to make the flow more linear and easier to reason about.
Learning Strategies for Better Program Visualization
Improving your ability to visualize program flow is a skill that develops over time. Here are strategies to accelerate this learning:
Deliberate Practice with Feedback
Regular, focused practice with immediate feedback is key to developing any complex skill:
- Trace code by hand: Manually trace through small code examples, writing down variable values and execution paths
- Verify with tools: Check your manual traces against debugger output to identify misunderstandings
- Progressive complexity: Start with simple loops and conditionals, then gradually work up to recursion, complex data structures, and asynchronous code
Study Well-Written Code
Examining high-quality code helps you internalize good patterns:
- Read open-source projects known for code quality
- Study how experienced developers structure their code for clarity
- Notice how they break down complex operations into understandable pieces
Explain Code to Others
Teaching forces you to clarify your own understanding:
- Practice explaining how code works to colleagues or even to an imaginary audience
- Write blog posts explaining complex programming concepts
- Participate in code reviews, which require you to understand and explain program flow
The “rubber duck debugging” technique—explaining your code line by line to an inanimate object—works because it forces you to articulate your understanding explicitly.
Develop System-Specific Mental Models
For frameworks and environments you use regularly, develop specialized mental models:
- Understand the React component lifecycle or the Django request/response cycle
- Learn how the JavaScript event loop processes tasks and microtasks
- Visualize how database transactions work in your ORM
These domain-specific models make it easier to reason about code in those particular environments.
Tools That Enhance Program Visualization
Beyond debuggers, numerous tools can help make program execution more visible:
Specialized Debuggers and Visualizers
- Python Tutor: Visualizes code execution in Python, JavaScript, Ruby, and more
- Recursion Visualizer: Shows the call tree for recursive functions
- JavaScript Visualizer: Illustrates scope, closures, and execution context
- Data Structure Visualizers: Show how trees, graphs, and other structures change during operations
Logging and Monitoring
- Structured logging: Creates machine-parseable logs that can be analyzed and visualized
- Log visualization tools: Convert logs into timeline views and state transitions
- Application Performance Monitoring (APM): Shows real-time execution data and bottlenecks
Interactive Development Environments
- Notebooks: Jupyter, Observable, and similar environments allow code and output to exist side by side
- REPL environments: Provide immediate feedback as you experiment with code
- Time-travel debuggers: Let you move backward and forward through execution history
Static Analysis Tools
- Call graph generators: Show the relationships between functions
- Control flow analyzers: Visualize all possible execution paths
- Type checkers: Verify data flow consistency before runtime
The Future of Program Visualization
As programming evolves, new approaches to visualization are emerging:
Visual Programming
Visual programming environments like Scratch, Unreal Engine’s Blueprints, or Node-RED represent program flow directly as connected blocks or nodes. These environments make control flow explicit and visible, reducing the need for mental simulation.
Live Programming Environments
Tools like Bret Victor’s “Inventing on Principle” demo or the Light Table IDE show real-time execution alongside code, allowing programmers to see the effects of changes immediately. This tight feedback loop makes program behavior more tangible.
AR/VR Visualization
Emerging research explores using augmented and virtual reality to create three-dimensional visualizations of program execution, allowing programmers to “walk through” their code and see data transformations in immersive environments.
AI-Assisted Understanding
AI tools are beginning to help explain code behavior, generate visualizations automatically, and predict potential issues based on learned patterns from millions of codebases.
Conclusion: From Invisible to Visible
The challenge of visualizing program flow stems from fundamental limitations in human cognition combined with the invisible, abstract nature of program execution. However, with the right strategies and tools, we can make the invisible visible.
By leveraging external tools, building strong mental models, using visual metaphors, and practicing deliberately, you can develop the ability to “see” how your code executes—even when it’s not directly observable.
Remember that even experienced programmers don’t try to keep entire programs in their heads at once. They use a combination of tools, abstractions, and focused attention to understand the parts they need when they need them.
As you develop your visualization skills, you’ll find that debugging becomes more intuitive, design decisions become clearer, and your overall programming effectiveness increases. The code won’t just be text on a screen—it will be a dynamic process you can follow, understand, and control.
What visualization techniques have you found most helpful in your programming journey? Which tools make program flow clearer for you? The quest to make the abstract concrete is ongoing, and sharing our approaches helps everyone improve.