Why You Can’t Translate Your Thoughts Into Code: Understanding The Gap Between Thinking And Programming
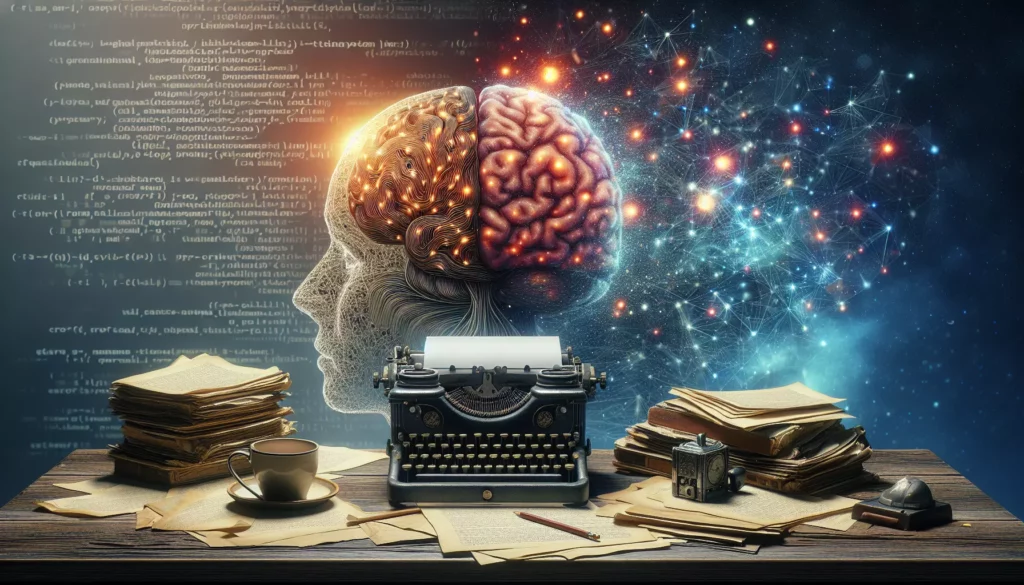
Have you ever had a brilliant idea for an app or a website, only to find yourself staring at a blank code editor, unable to translate your vision into working code? If so, you’re not alone. Many aspiring programmers and even experienced developers experience this disconnect between having a clear idea in their minds and actually implementing it through code.
This phenomenon is so common that it has become a universal experience in the programming community. The ability to think logically about a problem is one thing, but transforming those thoughts into functional code is an entirely different skill set.
The Thought-to-Code Gap: What’s Really Happening?
When we think about a solution to a problem, our brains operate in an abstract, high-level manner. We visualize outcomes, make intuitive leaps, and process information in parallel. Programming, on the other hand, requires precise, sequential instructions that follow strict syntactical rules.
Let’s break down the key factors that contribute to this gap:
1. Natural Language vs. Programming Language
Human thoughts occur in natural language, which is inherently ambiguous, contextual, and flexible. Programming languages, by contrast, are formal systems with rigid syntax and semantics.
For example, you might think: “I want to display a list of users sorted by their age.” This makes perfect sense to a human, but a computer needs explicit instructions:
const sortedUsers = users.sort((a, b) => a.age - b.age);
userList.innerHTML = sortedUsers.map(user => `<li>${user.name}, ${user.age}</li>`).join('');
Notice how much more detailed and structured the code is compared to the simple thought.
2. Implicit vs. Explicit Knowledge
When we think about solutions, we rely heavily on implicit knowledge and assumptions. We might skip steps that seem obvious or take shortcuts in our reasoning.
Computers, however, require every single step to be explicitly defined. Nothing is assumed or inferred. This means you have to fill in all the gaps that your brain automatically bridges when thinking about a problem.
3. The Curse of Knowledge
As humans, we suffer from what psychologists call “the curse of knowledge” — once we understand something, it’s difficult to imagine not understanding it. This makes it challenging to break down our intuitive understanding into the atomic steps needed for programming.
When you think, “I’ll just create a login system,” your mind glosses over hundreds of implementation details that you’ll need to explicitly code: form validation, password hashing, session management, security considerations, error handling, and more.
Common Manifestations of the Thought-to-Code Gap
This gap manifests in several ways throughout the programming journey:
The Beginner’s Paralysis
For beginners, the gap often appears as complete paralysis. You understand conceptually what variables, loops, and functions are, but when faced with an actual problem, you struggle to determine which programming constructs to use and how to organize them.
Consider this simple problem: “Calculate the average of a list of numbers.”
A beginner might understand the mathematical concept (sum all numbers and divide by count) but struggle with how to implement a loop, how to accumulate values, and how to handle edge cases like an empty list.
The Intermediate Plateau
Intermediate programmers often hit a plateau where they can implement individual features but struggle with system architecture. They can write functions and classes but have difficulty designing how these components should interact in a larger application.
You might be able to code a user authentication function and a data retrieval function separately, but connecting them into a coherent system with proper error handling and state management becomes challenging.
The Expert’s Abstraction Gap
Even expert programmers face this gap when working with new paradigms or at very high levels of abstraction. An experienced object-oriented programmer might struggle when first attempting functional programming, or a backend developer might find it difficult to think in terms of reactive frontend frameworks.
Why Programming Is More Than Just Logical Thinking
A common misconception is that programming is purely about logical thinking. While logic is fundamental, programming involves several other cognitive skills:
Mental Model Building
Effective programmers build detailed mental models of how their code works. They can visualize data flowing through functions, how objects interact, and how state changes over time.
This skill doesn’t come naturally; it develops through practice and experience. When you can’t translate your thoughts to code, it’s often because your mental model of programming concepts isn’t fully formed.
Pattern Recognition
Experienced programmers recognize patterns in problems and know which programming patterns to apply as solutions. This pattern-matching ability significantly reduces the cognitive load of programming.
When you’re not familiar with these patterns, each problem feels unique and overwhelming, making the translation from thought to code much harder.
Procedural Thinking
Programming requires breaking down tasks into precise, sequential steps. This procedural thinking is different from how we naturally solve problems, which often involves intuitive leaps and parallel processing.
Consider how you might give directions to a friend versus how you would program a GPS app. The level of detail and precision required is vastly different.
Practical Strategies to Bridge the Gap
Now that we understand why translating thoughts to code is challenging, let’s explore strategies to improve this skill:
1. Practice Pseudocode as an Intermediate Step
Pseudocode serves as a bridge between natural language and programming language. It allows you to express your solution in a structured way without worrying about syntax.
For example, before coding a sorting algorithm, write it out in pseudocode:
FUNCTION bubbleSort(array)
FOR i = 0 TO length of array - 1
FOR j = 0 TO length of array - i - 1
IF array[j] > array[j+1] THEN
SWAP array[j] AND array[j+1]
END IF
END FOR
END FOR
RETURN array
END FUNCTION
This helps clarify your thinking before you tackle the syntax of a specific programming language.
2. Break Problems Down Into Smaller Subproblems
Large problems are overwhelming and difficult to translate directly into code. Break them down into smaller, manageable subproblems.
For instance, if you’re building a todo app, break it down into:
- Creating the UI components
- Adding new todo items
- Marking items as complete
- Filtering the todo list
- Storing todos in local storage
Tackle each subproblem individually, then integrate them.
3. Start With Data Structures
Often, the hardest part of programming is deciding how to represent your data. Before writing functions or algorithms, define your data structures clearly.
For example, if building a user management system, start by defining what a user object looks like:
const user = {
id: 1,
username: 'johndoe',
email: 'john@example.com',
role: 'admin',
lastLogin: new Date('2023-10-15')
};
Once you know how your data is structured, the operations on that data become clearer.
4. Learn Design Patterns and Common Algorithms
Design patterns are proven solutions to common programming problems. Learning these patterns gives you templates to apply to similar problems.
For instance, if you need to notify multiple parts of your application when data changes, you might use the Observer pattern:
class Subject {
constructor() {
this.observers = [];
}
addObserver(observer) {
this.observers.push(observer);
}
notifyObservers(data) {
this.observers.forEach(observer => observer.update(data));
}
}
The more patterns you know, the easier it becomes to map your thoughts to established coding solutions.
5. Use Visual Aids and Diagrams
Visual representations can help bridge the gap between abstract thoughts and concrete code. Before coding a complex system, sketch out its components and how they interact.
Tools like flowcharts, UML diagrams, or even simple boxes and arrows on paper can clarify your thinking and provide a roadmap for implementation.
6. Read and Analyze Quality Code
Reading well-written code exposes you to how experienced developers structure their solutions. Pay attention to how they break down problems, name variables, and organize functions.
Open-source projects are excellent resources for this. Find projects related to your interests and study how they’re implemented.
7. Verbalize Your Thought Process
Explaining your approach out loud, even to yourself, forces you to articulate your thinking more precisely. This technique, known as “rubber duck debugging,” often reveals gaps in your reasoning.
As you verbalize each step, you’ll naturally break down complex ideas into simpler, more implementable parts.
Common Roadblocks and How to Overcome Them
Even with these strategies, certain roadblocks commonly impede the thought-to-code translation process:
Analysis Paralysis
When faced with multiple possible implementations, you might become paralyzed by indecision.
Solution: Embrace the principle of “make it work, make it right, make it fast.” Start with a simple implementation that works, then refine it. Remember that perfect is the enemy of done.
Syntax Frustration
Getting hung up on syntax details can derail your progress and disconnect you from your original thought process.
Solution: Use reference materials liberally. Keep documentation open while coding, and don’t hesitate to look up syntax. Modern IDEs with autocompletion and linting also help reduce syntax errors.
The Blank Screen Problem
Starting from nothing is often the hardest part. The empty editor can be intimidating.
Solution: Begin with comments outlining your approach, then gradually replace them with code. Alternatively, start with a template or boilerplate that provides basic structure.
// 1. Define the user class
// TODO: Add properties for name, email, etc.
// 2. Implement authentication method
// TODO: Add password validation
// 3. Create user profile view
// TODO: Design UI components
Scope Creep
As you code, you might think of additional features or edge cases, expanding the scope beyond your initial thought.
Solution: Maintain a separate list for new ideas and enhancements. Focus on implementing your original thought first, then revisit the list for iterations.
The Role of Experience in Bridging the Gap
There’s no shortcut around experience when it comes to bridging the thought-to-code gap. With time and practice, several things happen:
Chunking
Experienced programmers “chunk” code patterns in their minds. Instead of thinking about individual lines, they think in terms of higher-level constructs.
A beginner might laboriously construct a loop to process array elements:
for (let i = 0; i < array.length; i++) {
const item = array[i];
// Process item
}
An experienced developer might simply think "map over the array" and write:
array.map(item => /* Process item */)
This chunking reduces cognitive load and makes translation more fluid.
Intuition Development
With experience, you develop intuition about which approaches are likely to work and which might cause problems. This intuition guides your thought-to-code translation process.
You'll start to sense when a recursive solution might lead to stack overflow problems or when a particular data structure might become a performance bottleneck.
Language Fluency
Just as with natural languages, fluency in programming languages comes with practice. Eventually, you'll think partially in the programming language itself, reducing the translation overhead.
Learning from Failures: The Iteration Process
Perhaps the most important aspect of improving your thought-to-code translation is embracing failure as part of the learning process.
When your initial implementation doesn't match your mental model, don't get discouraged. Instead:
- Analyze the gap: What aspect of your thought didn't translate correctly?
- Refine your mental model: Update your understanding based on what you learned.
- Iterate: Try again with your refined understanding.
This iterative process gradually aligns your thinking with how code actually works.
Case Study: From Thought to Working Application
Let's walk through a concrete example of translating a thought into code, highlighting the challenges and strategies along the way.
Initial Thought: "I want to build a simple weather app that shows the current temperature and conditions for a user's location."
This seems straightforward, but let's see how it unfolds when we try to implement it:
Step 1: Break Down the Problem
The app needs to:
- Get the user's location
- Fetch weather data from an API
- Display the data in a user-friendly way
- Handle errors and edge cases
Step 2: Define Data Structures
What does our weather data look like?
const weatherData = {
location: {
city: 'New York',
country: 'US'
},
current: {
temperature: 72,
condition: 'Sunny',
humidity: 65,
windSpeed: 5
},
forecast: [
// Daily forecasts
]
};
Step 3: Pseudocode the Main Functionality
FUNCTION getWeatherForLocation()
TRY
coordinates = GET user's geolocation
weatherData = FETCH weather from API using coordinates
UPDATE UI with weatherData
CATCH error
SHOW error message to user
END TRY
END FUNCTION
WHEN page loads
CALL getWeatherForLocation
END WHEN
Step 4: Implement in Actual Code
Now we translate our pseudocode to JavaScript:
async function getWeatherForLocation() {
try {
// Get user's location
const position = await new Promise((resolve, reject) => {
navigator.geolocation.getCurrentPosition(resolve, reject);
});
const { latitude, longitude } = position.coords;
// Fetch weather data
const response = await fetch(
`https://api.weatherservice.com/data?lat=${latitude}&lon=${longitude}&units=imperial`
);
if (!response.ok) {
throw new Error('Weather data not available');
}
const weatherData = await response.json();
// Update UI
document.getElementById('location').textContent =
`${weatherData.location.city}, ${weatherData.location.country}`;
document.getElementById('temperature').textContent =
`${weatherData.current.temperature}°F`;
document.getElementById('condition').textContent =
weatherData.current.condition;
document.getElementById('condition-icon').src =
getIconForCondition(weatherData.current.condition);
} catch (error) {
document.getElementById('weather-container').classList.add('hidden');
document.getElementById('error-message').textContent =
'Unable to get weather information. ' + error.message;
document.getElementById('error-container').classList.remove('hidden');
}
}
function getIconForCondition(condition) {
// Map condition strings to icon paths
const iconMap = {
'Sunny': 'icons/sunny.svg',
'Cloudy': 'icons/cloudy.svg',
// More conditions...
};
return iconMap[condition] || 'icons/unknown.svg';
}
// Initialize when page loads
document.addEventListener('DOMContentLoaded', getWeatherForLocation);
Step 5: Identify Gaps and Refine
Notice how our implementation revealed details we didn't initially consider:
- We needed to handle promises and async operations
- We had to add error handling for API responses
- We created a helper function for mapping conditions to icons
- We needed to consider the DOM structure for updating the UI
This example illustrates how even a seemingly simple idea expands significantly when translated to code. Each step of the process forced us to think more concretely and address details that were implicit in our initial thought.
The Role of Tools and AI in Bridging the Gap
Modern development environments and AI tools can help bridge the thought-to-code gap:
Integrated Development Environments (IDEs)
IDEs provide code completion, syntax highlighting, and error detection that reduce the cognitive load of translating thoughts to code. Features like refactoring tools and code snippets also help express common patterns quickly.
AI Coding Assistants
Tools like GitHub Copilot, ChatGPT, and other AI coding assistants can generate code from natural language descriptions, helping bridge the gap between thoughts and implementation. While these tools aren't perfect, they can provide a starting point that you can refine.
For example, you might describe a function in a comment:
// Function to validate an email address using regex
And an AI assistant might generate:
function validateEmail(email) {
const regex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return regex.test(email);
}
Visual Programming Tools
Visual programming environments like Scratch, Node-RED, or even no-code platforms can provide a more intuitive way to express logic, especially for beginners or for specific domains like data flow or UI design.
The Continual Journey of Improvement
Becoming proficient at translating thoughts to code is not a destination but a journey. Even senior developers with decades of experience continue to refine this skill as they encounter new problems and paradigms.
The good news is that this skill follows a positive feedback loop: The more you practice translating thoughts to code, the better your mental models become, which in turn makes future translations easier.
Progressive Challenges
To improve systematically, challenge yourself with increasingly complex problems:
- Start with algorithmic problems that have clear inputs and outputs
- Move to feature implementation within existing codebases
- Progress to designing components that interact with other parts of a system
- Ultimately tackle system architecture where you design entire applications
Each level builds upon the skills of the previous one and expands your ability to translate more complex thoughts into working code.
Conclusion: Embracing the Gap as Part of the Journey
The gap between thoughts and code is not a deficiency but an inherent characteristic of programming. Understanding this gap and developing strategies to bridge it is an essential part of growing as a programmer.
Remember that every programmer, from beginners to experts at top tech companies, faces this challenge. The difference lies in the tools, strategies, and experience they bring to bear on the problem.
So the next time you find yourself staring at a blank editor, unable to translate your brilliant idea into code, don't be discouraged. Instead:
- Acknowledge the natural gap between thoughts and code
- Break down your idea into smaller, manageable pieces
- Use pseudocode to bridge natural and programming languages
- Focus on data structures before algorithms
- Leverage design patterns and existing solutions
- Embrace the iterative process of refinement
With practice and persistence, the gap will narrow, and you'll find yourself moving more fluidly between the realm of ideas and the world of working code. The journey may be challenging, but it's also immensely rewarding as you gain the power to bring your ideas to life through programming.
Remember that programming is ultimately a creative endeavor, and the thought-to-code translation is where much of that creativity happens. By mastering this skill, you don't just become a better coder; you become a more effective creator and problem solver in the digital age.