Why You Can’t Transform Requirements Into Code
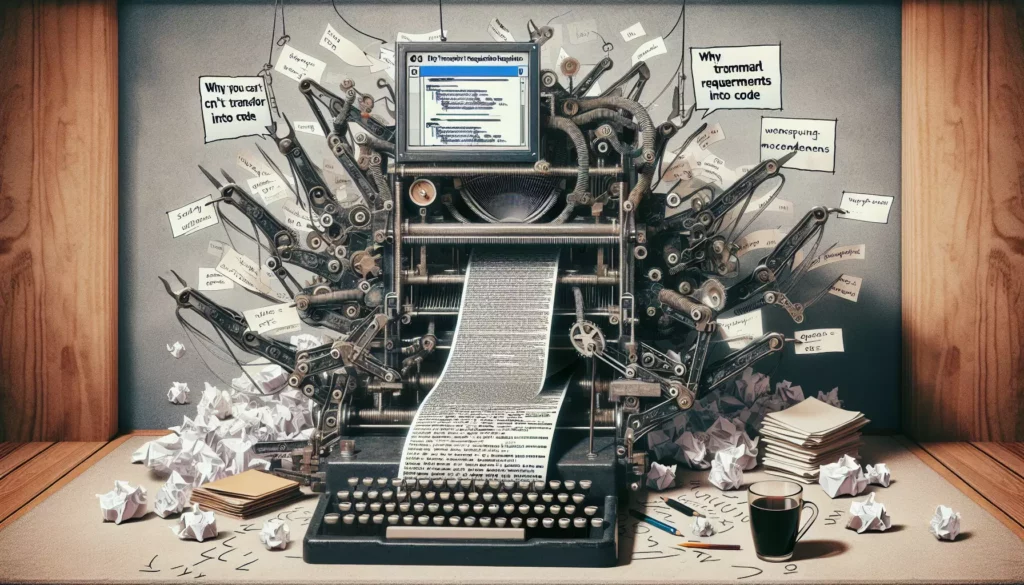
In the world of software development, there’s a persistent myth that programming is simply about translating requirements into code – as if it were a mechanical process of conversion from English (or any human language) to a programming language. This misconception is not only wrong but potentially harmful to both aspiring and experienced developers. In this article, we’ll explore why the transformation from requirements to code is far from straightforward and why understanding this complexity is crucial for becoming a proficient programmer.
The Illusion of Direct Translation
When newcomers to programming first approach coding, they often carry a mental model that suggests coding is like translation: take what the client wants in English and convert it to Java, Python, or another programming language. This view is reinforced by simplistic programming exercises that present clear problems with expected outputs.
However, real world software development rarely works this way. Requirements are often:
- Ambiguous and open to interpretation
- Incomplete, missing critical details
- Contradictory, containing mutually exclusive demands
- Unstated, with assumptions that aren’t explicitly documented
Consider a seemingly simple requirement: “The system should allow users to log in.” This single sentence contains numerous unstated decisions:
- What authentication methods will be supported?
- How will passwords be stored securely?
- What happens after multiple failed login attempts?
- Will there be password recovery options?
- How will user sessions be managed?
Each of these questions represents a decision point that must be resolved before writing a single line of code, and each decision could lead development in different directions.
The Gap Between Requirements and Implementation
Even with perfectly clear requirements, there remains a substantial gap between knowing what to build and knowing how to build it. This gap represents the essence of software engineering as a creative problem solving discipline rather than a mechanical translation process.
Let’s examine why this gap exists:
1. Multiple Valid Solutions
For any given requirement, there are typically multiple valid implementation approaches, each with different trade-offs. A login system could be implemented using:
- JWT (JSON Web Tokens) for stateless authentication
- Session-based authentication with cookies
- OAuth integration with third-party providers
- Biometric authentication for mobile applications
Choosing between these options requires understanding the broader context of the application, security needs, scalability requirements, and user experience considerations.
2. Technical Constraints
Real-world implementation must account for technical constraints that aren’t mentioned in requirements:
- Performance boundaries
- Memory limitations
- Network reliability
- Compatibility with existing systems
- Deployment environment restrictions
A developer must balance the ideal solution against what’s technically feasible within the project’s constraints.
3. Design Decisions
Software development involves countless design decisions that shape the final code but aren’t specified in requirements:
- Code organization and architecture
- Error handling strategies
- Logging and monitoring approaches
- Testing methodologies
- Data structure selection
These decisions significantly impact maintainability, scalability, and robustness of the solution.
Requirements Interpretation: A Creative Process
Interpreting requirements is fundamentally a creative process that involves:
1. Reading Between the Lines
Experienced developers develop the ability to identify unstated requirements and assumptions. They ask questions like:
- “What happens in this edge case?”
- “How should the system behave under failure conditions?”
- “What are the performance expectations?”
This skill of anticipating what’s not explicitly stated comes from experience and domain knowledge.
2. Contextual Understanding
Requirements don’t exist in isolation. They must be understood within the context of:
- The overall business goals
- User needs and behaviors
- Regulatory and compliance requirements
- Technical ecosystem
A requirement to “store user data” takes on very different meanings in a healthcare application versus a simple blog platform.
3. Reconciling Contradictions
Requirements often contain implicit contradictions that must be resolved. For example:
- “The application should be highly secure” vs. “The login process should be simple and quick”
- “The system must handle millions of concurrent users” vs. “The solution should be low-cost”
Finding the right balance between competing concerns is a core skill in software development.
The Implementation Gap: From What to How
Even with perfectly understood requirements, the journey to implementation involves substantial problem-solving:
1. Algorithm Selection
Choosing the right algorithm for a task involves understanding computational complexity, memory usage, and the specific characteristics of your data. For instance, a requirement to “sort the data” might lead to very different implementations depending on:
- The size of the dataset
- Whether the data is nearly sorted already
- Memory constraints
- Whether sorting needs to be stable
An experienced developer might choose quicksort for general-purpose in-memory sorting, merge sort for external sorting of large datasets, or insertion sort for tiny or nearly-sorted collections.
2. Data Structure Design
Selecting appropriate data structures profoundly impacts performance and code clarity. Consider a requirement to “efficiently look up customer records by ID.” This could be implemented using:
- A hash map for O(1) average-case lookups
- A balanced tree for guaranteed O(log n) worst-case performance
- A simple array with binary search if the dataset is small and stable
- A database with appropriate indexing for persistent storage
Each choice carries different implications for memory usage, lookup speed, and implementation complexity.
3. Error Handling Strategy
Requirements rarely specify how errors should be handled in detail. Developers must decide:
- When to use exceptions versus return codes
- How granular error types should be
- What information should be included in error messages
- How errors should be logged and monitored
- Recovery strategies for different failure scenarios
These decisions shape both the internal structure of the code and the user experience when things go wrong.
Code as Design
Software development is fundamentally a design activity. As Jack W. Reeves argued in his seminal essay “What Is Software Design?”, the source code itself is the design document, and the compiled executable is the final product. This perspective highlights why the transformation from requirements to code is not mechanical but creative.
Consider these aspects of code as design:
1. Balancing Competing Concerns
Good code design requires balancing multiple competing concerns:
- Performance vs. readability
- Flexibility vs. simplicity
- Immediate delivery vs. long-term maintainability
- Reusability vs. specialization
There’s rarely a single “correct” balance; the appropriate trade-offs depend on the specific context and constraints of the project.
2. Expressing Intent
Well-designed code clearly expresses the intent behind the implementation. Compare these two code snippets that implement the same requirement of “validate that a string is a valid email address”:
// Approach 1: Regular expression
boolean isValidEmail(String email) {
return email.matches("^[A-Za-z0-9+_.-]+@(.+)$");
}
// Approach 2: Structured validation
boolean isValidEmail(String email) {
if (email == null || email.isEmpty()) {
return false;
}
int atIndex = email.indexOf('@');
if (atIndex <= 0 || atIndex == email.length() - 1) {
return false;
}
String localPart = email.substring(0, atIndex);
String domainPart = email.substring(atIndex + 1);
return isValidLocalPart(localPart) && isValidDomainPart(domainPart);
}
Both implementations satisfy the requirement, but they express different intents and make different trade-offs in terms of readability, maintainability, and thoroughness of validation.
3. Anticipating Change
Good code design anticipates likely changes and makes them easier to implement. This requires judgment about which aspects of the system are likely to change and which will remain stable. Requirements rarely specify this dimension explicitly.
The Reality of Requirements Evolution
The idea that requirements can be fully specified before coding begins is increasingly recognized as unrealistic in modern software development. Requirements evolve for several reasons:
1. Discovery Through Implementation
The act of implementing a feature often reveals nuances and complexities that weren’t apparent during requirements gathering. As developers work through the implementation details, they uncover questions and edge cases that require clarification or additional requirements.
2. User Feedback
Once users interact with a feature, they often provide feedback that leads to refinement of the requirements. This is why agile methodologies emphasize early and frequent delivery of working software – it enables faster feedback loops.
3. Changing Business Needs
Business needs evolve over time in response to market conditions, competitive pressures, and organizational learning. Requirements must evolve accordingly.
This reality of constantly evolving requirements further undermines the notion that programming is simply about translating fixed requirements into code. Instead, it’s an ongoing conversation between possibilities and constraints.
Case Study: A Simple Search Feature
Let’s examine how a seemingly simple requirement expands into complex implementation decisions through a case study.
Initial requirement: “Users should be able to search for products by name.”
This straightforward request immediately raises questions:
- Should the search be exact match or partial match?
- Should it be case-sensitive?
- Should it handle special characters and accents?
- How should results be ranked if multiple products match?
- How many results should be returned at once?
- Should the search support filters or advanced operators?
Let’s say we clarify these and arrive at a more detailed requirement:
“Users should be able to search for products by name using partial, case-insensitive matching. Results should be ranked by relevance, with exact matches appearing first. The search should return up to 20 results at a time, with pagination for additional results.”
Even with this clarification, the implementation involves numerous decisions:
Search Algorithm Selection
Options include:
- Simple SQL LIKE queries (easy to implement but limited functionality)
- Full-text search capabilities of the database
- Dedicated search engines like Elasticsearch or Solr
- Custom in-memory search algorithms
Each option offers different trade-offs in terms of implementation complexity, performance characteristics, and feature richness.
Relevance Ranking
Determining how to rank results by “relevance” is non-trivial. Factors might include:
- Position of the match within the product name
- Completeness of the match
- Product popularity or rating
- Recency of product listing
The algorithm for combining these factors requires careful design and tuning.
Performance Considerations
For a production system, performance becomes critical:
- How will the search perform with millions of products?
- Should results be cached?
- Should the search operate asynchronously for complex queries?
- How will the system handle high concurrent search volume?
These considerations may lead to architectural decisions like implementing search indexes, query optimization, or service-level partitioning.
User Experience Details
The implementation must also consider user experience aspects:
- Should search suggestions appear as the user types?
- How should “no results found” scenarios be handled?
- Should common misspellings be automatically corrected?
- How should the pagination interface work?
Even this relatively simple feature expands into dozens of design decisions that aren’t specified in the original requirement. The final implementation might range from 50 lines of code for a basic solution to thousands of lines for a sophisticated search system.
Learning to Bridge the Gap
If programming isn’t simply about translating requirements to code, how can developers learn to bridge this gap effectively? Here are key strategies:
1. Develop Problem-Solving Skills
At its core, programming is about problem-solving. Developers should practice:
- Breaking complex problems into smaller, manageable parts
- Identifying and challenging assumptions
- Exploring multiple solution approaches before committing
- Learning to recognize patterns in problems and solutions
Platforms like AlgoCademy that focus on algorithmic thinking and problem-solving skills are valuable for developing this foundation.
2. Build Technical Breadth
Knowing a wide range of technical solutions enables developers to select the most appropriate tools for each problem:
- Study diverse algorithms and data structures
- Learn multiple programming paradigms (object-oriented, functional, etc.)
- Explore different architectural patterns
- Understand various technology stacks and their trade-offs
This breadth allows developers to see beyond the immediate solution to consider alternatives that might better address the underlying needs.
3. Cultivate Domain Knowledge
Understanding the business domain enables developers to interpret requirements more effectively:
- Learn the terminology and concepts of the industry
- Understand the workflows and pain points of users
- Recognize unstated assumptions that domain experts take for granted
- Identify common patterns and best practices in the domain
Domain knowledge helps bridge the semantic gap between what stakeholders say and what they actually need.
4. Practice Incremental Development
Embracing incremental development acknowledges the reality that requirements and understanding evolve:
- Start with the simplest implementation that addresses the core need
- Seek early feedback on partial implementations
- Refine solutions based on new insights
- Build flexibility into designs to accommodate likely changes
This approach reduces the risk of building elaborate solutions based on misunderstood requirements.
5. Develop Communication Skills
Effective communication is essential for clarifying requirements:
- Ask probing questions to uncover unstated assumptions
- Restate requirements in your own words to confirm understanding
- Use diagrams, prototypes, and examples to make abstract concepts concrete
- Explain technical constraints and trade-offs in non-technical terms
Strong communication skills help close the gap between what stakeholders envision and what developers can realistically build.
The Value of Experience
Much of the ability to bridge the gap between requirements and code comes from experience. Experienced developers have:
- Encountered similar problems before and know common pitfalls
- Developed an intuition for which approaches are likely to succeed
- Built a mental library of patterns and anti-patterns
- Learned from past mistakes and successes
This experience can’t be fully taught in a classroom or learned from books; it must be acquired through practice, reflection, and mentorship.
However, aspiring developers can accelerate this learning by:
- Working on diverse projects to encounter a wider range of challenges
- Studying open-source codebases to see how others solve problems
- Participating in code reviews to learn from peers
- Reflecting on past projects to identify lessons learned
Embracing the Creative Nature of Programming
Recognizing that programming is not a mechanical translation process but a creative endeavor has important implications:
1. For Educators
Programming education should go beyond syntax and basic algorithms to include:
- Problem-solving strategies
- Design thinking
- Requirements analysis
- Trade-off evaluation
Exercises should include ambiguous or incomplete requirements to develop the skills needed in real-world programming.
2. For Aspiring Developers
Those learning to code should:
- Embrace the ambiguity and creativity inherent in programming
- Focus on building problem-solving skills alongside technical knowledge
- Seek opportunities to work on open-ended projects
- Develop communication skills to clarify requirements
3. For Organizations
Software development processes should acknowledge the creative nature of programming by:
- Allowing time for exploration and prototyping
- Facilitating close collaboration between developers and stakeholders
- Embracing iterative development approaches
- Valuing design and architecture work as essential activities
Conclusion
The notion that programming is simply about translating requirements into code fundamentally misunderstands the nature of software development. In reality, the journey from requirements to working software involves interpretation, design thinking, creative problem-solving, and countless decisions that shape the final solution.
This gap between requirements and implementation isn’t a bug in the software development process – it’s a feature. It’s the space where developers add value through their expertise, creativity, and judgment. It’s where elegant solutions are crafted, technical constraints are navigated, and user needs are truly understood and addressed.
By recognizing and embracing the creative nature of programming, we can better prepare new developers for the challenges they’ll face, design more effective development processes, and ultimately build better software. The transformation from requirements to code isn’t a mechanical process but a creative journey – and that’s what makes programming both challenging and deeply rewarding.
For those learning to code on platforms like AlgoCademy, understanding this reality is crucial. While mastering algorithms and data structures is important, equally vital is developing the problem-solving mindset and design thinking skills that enable you to bridge the gap between what users want and what code can deliver. This holistic approach to programming education will better prepare you for the realities of software development in the professional world.