Why You Can’t Think of Edge Cases During Interviews (And How to Fix It)
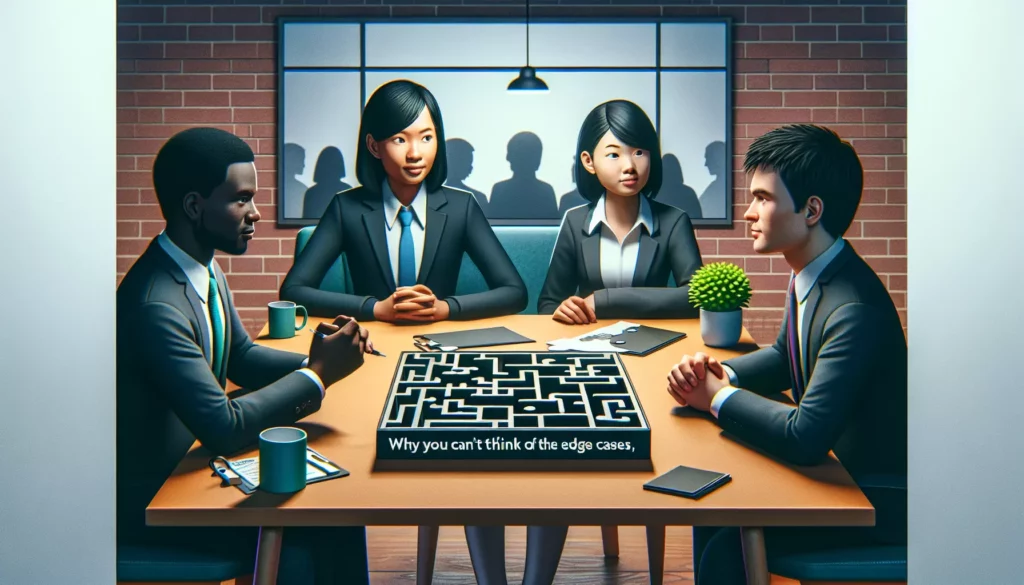
Technical interviews can be nerve-wracking experiences. You’re sitting across from an interviewer (or staring at them through a Zoom call), trying to solve a complex coding problem while articulating your thought process clearly. Then it happens: you confidently present your solution, only for the interviewer to ask, “What about this edge case?” Suddenly, your carefully constructed solution crumbles.
Sound familiar?
Edge cases are those pesky, often overlooked scenarios that exist at the extremes of your problem space. They’re the empty arrays, the negative inputs, the duplicate values, and the boundary conditions that can break an otherwise sound algorithm. And for some reason, they have a knack for hiding until the worst possible moment: your interview.
In this comprehensive guide, we’ll explore why identifying edge cases during high-pressure interviews is so challenging and, more importantly, how you can develop a systematic approach to catching them before your interviewer does.
Table of Contents
- Why Edge Cases Matter in Technical Interviews
- The Cognitive Science Behind Missing Edge Cases
- Common Edge Cases You Should Always Check
- A Framework for Identifying Edge Cases
- Practice Strategies to Improve Edge Case Detection
- What to Do When You Miss an Edge Case During an Interview
- Real-World Examples: Before and After Edge Case Analysis
- Conclusion: Making Edge Case Detection Second Nature
Why Edge Cases Matter in Technical Interviews
Before diving into strategies, let’s understand why edge cases are so crucial in the interview context:
They Demonstrate Thoroughness
When you proactively identify edge cases, you signal to the interviewer that you’re meticulous and detail-oriented. These are qualities that translate directly to writing robust production code.
They Reveal Problem-Solving Depth
Anyone can solve the happy path. It’s how you handle the edge cases that demonstrates your problem-solving depth and experience.
They Simulate Real-World Coding
In real-world software development, edge cases are often where bugs hide. By focusing on them during interviews, companies assess your ability to write resilient code.
A senior engineer at Google once told me, “I don’t hire based on whether candidates can solve the problem perfectly the first time. I hire based on how they handle the unexpected edge cases I throw at them.”
The Cognitive Science Behind Missing Edge Cases
Understanding why our brains often fail to catch edge cases is the first step toward developing strategies to overcome this limitation.
Cognitive Load Theory
During an interview, your working memory is juggling multiple tasks:
- Understanding the problem statement
- Developing an algorithm
- Translating that algorithm into code
- Maintaining awareness of time constraints
- Communicating your thought process clearly
With so much cognitive load, it’s easy for edge cases to slip through the cracks. Your brain prioritizes the core solution over the exceptions.
Confirmation Bias
Once you’ve developed a solution, you tend to test it with examples that confirm it works rather than seeking cases that might break it. This confirmation bias is a natural human tendency but can be detrimental in technical problem-solving.
Pressure-Induced Tunnel Vision
Under stress, your brain narrows its focus (a phenomenon called “perceptual narrowing”). This evolutionary response helps in some dangerous situations but hinders comprehensive thinking needed for edge case detection.
Research from the Journal of Experimental Psychology suggests that performance anxiety can reduce working memory capacity by up to 30%. That’s a significant handicap when you need to think holistically about a problem.
Common Edge Cases You Should Always Check
While edge cases vary by problem type, some categories are so common that you should develop a habit of checking them automatically:
Input-Related Edge Cases
- Empty inputs: Empty strings, arrays, lists, trees, graphs
- Single-element inputs: What happens when there’s just one item?
- Extremely large inputs: Will your solution scale with very large inputs?
- Invalid inputs: What if the input isn’t in the expected format?
Numeric Edge Cases
- Zero: Often a special case in mathematical operations
- Negative numbers: Especially in problems assuming positive inputs
- Minimum/maximum values: Potential integer overflow/underflow
- Floating-point precision: Equality comparisons with floats
Data Structure-Specific Edge Cases
- Arrays/Lists: Empty, single element, duplicates, sorted vs. unsorted
- Trees: Empty, single node, unbalanced, degenerate (essentially a linked list)
- Graphs: Disconnected, cycles, self-loops, directed vs. undirected
- Strings: Empty, single character, all same characters, case sensitivity
Algorithm-Specific Edge Cases
- Binary Search: Even vs. odd number of elements, target not in array
- Recursion: Base cases, stack overflow with deep recursion
- Dynamic Programming: Boundary conditions for your DP table
- Greedy Algorithms: Counterexamples where greedy approach fails
A Framework for Identifying Edge Cases
Now that we understand why edge cases matter and why they’re easy to miss, let’s develop a systematic framework for identifying them during interviews.
The ACTIVE Framework
I’ve developed what I call the ACTIVE framework for edge case detection:
A – Analyze the input domain
Before diving into code, explicitly define the range and types of inputs your solution needs to handle:
- What data types are involved?
- What are the constraints on input values?
- Are there any implicit assumptions in the problem statement?
C – Consider corner cases
Identify the extremes of your input domain:
- What happens at the minimum/maximum values?
- What about empty or minimal inputs?
- Are there special values (like zero) that might behave differently?
T – Test with diverse examples
Generate test cases that cover different scenarios:
- The happy path (normal expected behavior)
- Boundary conditions
- Invalid or unexpected inputs
- Examples that test each branch of your algorithm
I – Iterate through your solution
Mentally trace through your algorithm with your test cases:
- Walk through each step of your solution
- Pay attention to loop conditions, especially off-by-one errors
- Check division operations for potential divide-by-zero errors
V – Verify against constraints
Ensure your solution meets all the stated and implied constraints:
- Time complexity requirements
- Space complexity limitations
- Any special requirements mentioned in the problem
E – Explicitly state assumptions
Verbalize any assumptions you’re making:
- “I’m assuming all inputs will be valid integers.”
- “I’m assuming the input array contains at least one element.”
- “I’m assuming we don’t need to handle duplicate values.”
By explicitly stating your assumptions, you either confirm them with the interviewer or identify potential edge cases you need to handle.
Applying the ACTIVE Framework: A Practical Example
Let’s apply this framework to a common interview problem: finding the maximum subarray sum (Kadane’s algorithm).
Problem Statement:
Given an array of integers, find the contiguous subarray with the largest sum.
A – Analyze the input domain:
- Input is an array of integers
- Integers can be positive, negative, or zero
- Array length can vary
C – Consider corner cases:
- Empty array
- Array with a single element
- Array with all negative numbers
- Array with all zeros
T – Test with diverse examples:
- Normal case: [1, -2, 3, 4, -1, 2, -5, 4] (expected output: 8)
- All negative: [-2, -3, -1, -5] (expected output: -1)
- Single element: [5] (expected output: 5)
- Empty array: [] (undefined behavior, need to clarify)
I – Iterate through your solution:
function maxSubarraySum(nums) {
if (nums.length === 0) return 0; // Edge case: empty array
let currentSum = nums[0];
let maxSum = nums[0];
for (let i = 1; i < nums.length; i++) {
// The key insight of Kadane's algorithm
currentSum = Math.max(nums[i], currentSum + nums[i]);
maxSum = Math.max(maxSum, currentSum);
}
return maxSum;
}
V – Verify against constraints:
- Time complexity: O(n) – a single pass through the array
- Space complexity: O(1) – using only two variables regardless of input size
E – Explicitly state assumptions:
- “I’m assuming we should return 0 for an empty array, but I’d like to confirm that.”
- “For arrays with all negative numbers, I’m returning the single largest negative number as the maximum sum.”
By following this framework, we’ve identified several edge cases that might have been missed with a more casual approach.
Practice Strategies to Improve Edge Case Detection
Like any skill, identifying edge cases improves with deliberate practice. Here are effective strategies to build this muscle:
The “Break My Code” Game
After solving a problem, challenge yourself (or a study partner) to find inputs that would break your solution. This reverses the typical problem-solving approach and trains you to think adversarially about your own code.
Edge Case Journaling
Keep a log of edge cases that you miss during practice. Review this journal regularly to identify patterns in your blind spots. Over time, you’ll develop an intuition for your personal edge case weaknesses.
Test-Driven Development Practice
Before writing your solution, write test cases that cover various scenarios, including edge cases. This forces you to think about potential issues before you’re invested in a particular implementation.
The “What If” Exercise
After reading a problem statement, spend 2-3 minutes asking “what if” questions:
- What if the input is empty?
- What if there are duplicates?
- What if the values are extremely large or small?
Write down these questions and their answers before starting your solution.
Mock Interviews with Edge Case Focus
When practicing with mock interviews, ask your interviewer to specifically probe for edge cases. Alternatively, when you’re the interviewer, make identifying edge cases a key evaluation criterion.
The Five-Minute Review
After implementing your solution but before declaring it complete, spend five minutes specifically reviewing for edge cases. Use a checklist based on the common categories we discussed earlier.
What to Do When You Miss an Edge Case During an Interview
Despite your best preparation, you might still miss edge cases during an actual interview. How you handle this situation can significantly impact the interviewer’s perception:
Acknowledge Gracefully
When an interviewer points out an edge case you missed, acknowledge it directly: “You’re absolutely right. I didn’t consider that case. Let me think about how to handle it.”
Analyze, Don’t Panic
Take a moment to understand why the edge case breaks your solution. Rushing to fix it without understanding might lead to more issues.
Think Aloud
Share your thought process as you adapt your solution: “To handle this empty array case, I need to add a check at the beginning of my function…”
Reflect on Prevention
Briefly mention how you could have caught this edge case earlier: “In the future, I should explicitly check for empty collections as part of my initial analysis.”
Update Your Testing Strategy
After adjusting your solution, propose additional test cases to verify your fix and check for similar edge cases.
Sample Dialogue
Interviewer: “What happens if the input array is empty?”
Poor Response: “Oh, I didn’t think of that. Let me just add a check real quick…” (Shows panic and lack of systematic thinking)
Better Response: “That’s an excellent point. I didn’t explicitly handle the empty array case. In this context, I think we should return 0 as there’s no subarray to sum. Let me add that check at the beginning of my function and then walk through a test case to verify the behavior.”
Real-World Examples: Before and After Edge Case Analysis
Let’s examine some common interview problems before and after applying systematic edge case analysis:
Example 1: Binary Search
Initial Implementation:
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
After Edge Case Analysis:
function binarySearch(arr, target) {
// Edge case: empty array
if (arr.length === 0) return -1;
let left = 0;
let right = arr.length - 1;
while (left <= right) {
// Prevent integer overflow for large arrays
let mid = left + Math.floor((right - left) / 2);
if (arr[mid] === target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
Key improvements:
- Added explicit check for empty array
- Modified mid-point calculation to prevent integer overflow
Example 2: String Reversal
Initial Implementation:
function reverseString(str) {
return str.split('').reverse().join('');
}
After Edge Case Analysis:
function reverseString(str) {
// Edge case: null or undefined input
if (str === null || str === undefined) {
return '';
}
// Edge case: non-string input
if (typeof str !== 'string') {
str = String(str);
}
return str.split('').reverse().join('');
}
Key improvements:
- Added handling for null/undefined inputs
- Added conversion for non-string inputs
Example 3: Finding a Cycle in a Linked List
Initial Implementation:
function hasCycle(head) {
let slow = head;
let fast = head;
while (fast && fast.next) {
slow = slow.next;
fast = fast.next.next;
if (slow === fast) {
return true;
}
}
return false;
}
After Edge Case Analysis:
function hasCycle(head) {
// Edge case: empty list or single node
if (!head || !head.next) {
return false;
}
let slow = head;
let fast = head;
while (fast && fast.next) {
slow = slow.next;
fast = fast.next.next;
if (slow === fast) {
return true;
}
}
return false;
}
Key improvements:
- Added explicit check for empty list
- Added check for single-node list (which cannot have a cycle)
Conclusion: Making Edge Case Detection Second Nature
Edge case detection is not just a technical skill; it’s a mindset. The best engineers don’t treat edge cases as an afterthought—they integrate edge case thinking into their problem-solving process from the beginning.
By understanding the cognitive challenges, learning common patterns, applying a systematic framework, and practicing deliberately, you can transform edge case detection from a weakness into a strength in your technical interviews.
Remember that interviewers are often more impressed by candidates who can identify and handle edge cases proactively than by those who produce a perfect solution only for the happy path. In fact, many interviewers deliberately leave edge cases unspecified to see if candidates will ask the right questions.
The next time you find yourself in a technical interview, take a deep breath, apply the ACTIVE framework, and approach edge cases with confidence. With practice, what once felt like an interviewer’s “gotcha” moment will become your opportunity to shine.
Key Takeaways
- Edge cases matter because they demonstrate thoroughness, problem-solving depth, and real-world coding ability.
- We miss edge cases due to cognitive load, confirmation bias, and pressure-induced tunnel vision.
- Common edge cases include empty inputs, extreme values, and data structure-specific scenarios.
- The ACTIVE framework provides a systematic approach to identifying edge cases.
- Practice strategies like the “Break My Code” game and edge case journaling can help build your edge case detection muscle.
- When you miss an edge case during an interview, acknowledge it gracefully and demonstrate your ability to adapt.
With these strategies in your toolkit, you’ll be well-equipped to tackle the edge cases that might otherwise catch you off guard. Happy interviewing!