Why You Can’t Start Coding: Breaking the Blank Page Barrier
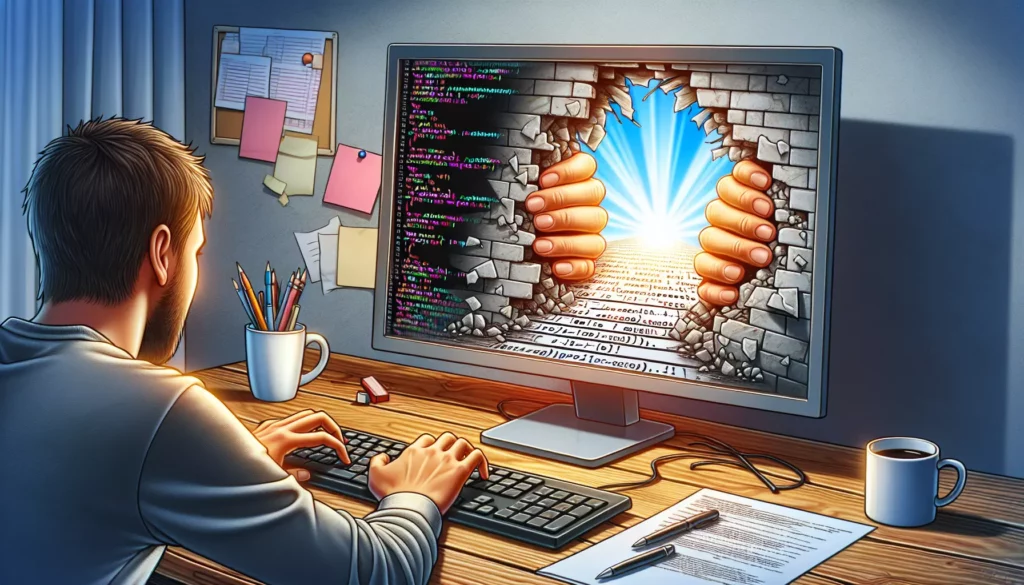
The Blank Screen Phenomenon: Why Starting to Code Feels So Intimidating
You’ve decided to learn programming. You’ve read tutorials, watched videos, maybe even completed some guided exercises. Now you sit down to create your first independent project and… nothing happens. Your mind goes blank, your fingers freeze above the keyboard, and that empty text editor seems to mock you with its blinking cursor.
If this scenario sounds familiar, you’re experiencing what many developers call the “blank screen phenomenon” – that paralyzing moment when you need to write code from scratch, and you simply don’t know where to begin.
This struggle is incredibly common, affecting everyone from complete beginners to experienced professionals tackling unfamiliar problems. In this article, we’ll explore why starting from scratch is so difficult, what’s happening in your brain when you face this challenge, and most importantly, practical strategies to overcome this hurdle in your coding journey.
The Psychology Behind Coding Paralysis
Before diving into solutions, it’s worth understanding the psychological barriers that make starting from scratch so difficult. This isn’t just about technical knowledge – it’s about how our brains process complex tasks.
Cognitive Overload: Too Many Moving Parts
Programming requires juggling multiple concepts simultaneously. When starting a project from scratch, you need to:
- Define the problem scope
- Design the solution architecture
- Choose appropriate data structures
- Plan the program flow
- Remember syntax details
- Anticipate edge cases
- Structure your code for readability and maintenance
This creates what psychologists call “cognitive overload” – when your working memory is bombarded with too many demands at once. Your brain essentially short-circuits, making it difficult to make even simple decisions about where to begin.
Analysis Paralysis: The Paradox of Choice
Modern programming offers countless ways to solve any given problem. Which language should you use? Which framework? Which design pattern? What’s the “right way” to structure your code?
Psychologist Barry Schwartz calls this the “paradox of choice” – when too many options lead to decision paralysis rather than freedom. In coding, this manifests as hours spent researching the “perfect” approach instead of writing actual code.
The Intimidation of the Blank Canvas
Artists have long discussed the terror of the blank canvas – that intimidating empty space where anything is possible, but nothing yet exists. Programmers face the same psychological barrier with empty text editors. Without constraints or starting points, the infinite possibilities can feel overwhelming rather than liberating.
Fear of Failure and Perfectionism
Many developers, especially beginners, struggle with perfectionism. There’s an unspoken expectation that code should be elegant, efficient, and error-free from the start. This perfectionism creates performance anxiety that can prevent you from writing even a single line of code.
The fear of writing “bad code” becomes so paralyzing that you write no code at all – a form of self-sabotage that prevents any progress.
The Tutorial Trap: Why Learning Doesn’t Always Translate to Doing
One of the most frustrating experiences for new programmers is completing dozens of tutorials, only to find they still can’t code independently. This disconnect between learning and application has several causes:
Passive vs. Active Learning
Following tutorials often involves passive learning – watching someone else solve problems or copying existing code. While you might understand each step as you follow along, this doesn’t develop the active problem-solving skills needed to write code from scratch.
It’s similar to the difference between watching someone play piano and learning to play yourself. You might intellectually understand what they’re doing, but that doesn’t mean your fingers can reproduce it.
The Illusion of Competence
Tutorials create what psychologists call an “illusion of competence” – you recognize the concepts and think “I understand this!” But recognition is much easier than recall. When faced with a blank editor, you need to recall information from memory without prompts, which is significantly more difficult.
Tutorial Projects Are Pre-Scoped
In tutorials, someone else has already:
- Defined the exact problem to solve
- Broken it down into manageable steps
- Determined which tools and approaches to use
- Eliminated all unnecessary complexity
Real-world coding rarely comes with such clear boundaries. When creating something from scratch, you must handle all this ambiguity yourself – a skill that tutorials don’t typically teach.
The Knowledge Gap: Syntax vs. Problem-Solving
Many coding education resources focus heavily on syntax and language features, but spend less time on the crucial skill of problem decomposition – breaking complex problems into solvable parts.
The Missing Mental Models
Experienced developers rely on mental models and patterns they’ve built over years of practice. These frameworks help them organize their thoughts and approach problems systematically. Beginners often lack these mental scaffolds, making it difficult to know where to begin or how to structure their thinking.
The Planning Deficit
Coding isn’t just typing – it requires substantial planning before you write a single line. Many beginners struggle because they try to code before they’ve fully understood the problem or planned their approach. Without a roadmap, it’s easy to get lost in implementation details.
Studies of professional programmers show they typically spend significant time thinking and planning before coding. This “invisible” part of programming is rarely taught explicitly but is crucial for starting projects successfully.
Practical Strategies to Overcome Coding Blank Page Syndrome
Now that we understand the challenges, let’s explore practical strategies to overcome the struggle of starting from scratch. These approaches can help whether you’re a complete beginner or an experienced developer facing an unfamiliar problem.
Start With Pseudocode and Comments
Instead of diving straight into implementation, begin by writing pseudocode – plain language descriptions of what your program needs to do. This reduces cognitive load by separating the “what” from the “how.”
For example, if building a simple to-do app, you might start with:
// 1. Create a function to add new tasks
// 2. Create a function to mark tasks as complete
// 3. Create a function to delete tasks
// 4. Create a function to display all tasks
// 5. Set up event listeners for user interactions
This approach gives you a skeleton to flesh out, making the blank page less intimidating.
The Divide and Conquer Method
Break your project into the smallest possible components. Don’t think about building an entire e-commerce site – focus on creating just the product listing component, then just the shopping cart, and so on.
Computer scientists call this “decomposition” – solving complex problems by breaking them into simpler sub-problems. Each small piece becomes manageable, and you can gradually connect them to form your complete solution.
Build a Minimum Viable Product (MVP)
Start with the simplest possible version of your idea that could work. For a calculator app, maybe begin with just addition functionality. For a blog, start with displaying static posts before adding user authentication or comments.
This approach reduces complexity and gives you quick wins to build momentum. Once the core functionality works, you can iteratively add features.
Use the Rubber Duck Debugging Technique
Sometimes the block comes from not fully understanding the problem. Try explaining your project out loud as if teaching someone else (or an actual rubber duck on your desk). The act of verbalization often clarifies your thinking and reveals the logical next steps.
This technique works because it forces you to articulate the problem in clear, simple terms – often revealing gaps in your understanding or obvious solutions you hadn’t considered.
Timeboxed Experimentation
Set a timer for 25 minutes and commit to just experimenting with code during that time. Give yourself permission to write “bad” or incomplete code – the goal is simply to start somewhere and learn through doing.
This technique, inspired by the Pomodoro method, helps overcome perfectionism by creating a safe space for imperfect attempts. Often, once you start typing, ideas begin to flow more naturally.
Scaffolding Your Learning: Bridging Tutorials and Original Coding
To bridge the gap between following tutorials and coding from scratch, you need intermediate stepping stones. Here are effective scaffolding techniques:
The “Extend and Modify” Approach
Instead of starting from zero, begin with a tutorial project but then extend it with your own features. This provides structure while still requiring original problem-solving.
For example, if you’ve completed a tutorial for a basic weather app, try adding:
- A 5-day forecast feature
- The ability to save favorite locations
- A different visual theme
These modifications require you to understand the existing code and apply your knowledge in new ways.
Code Reading and Reverse Engineering
Before writing your own code, spend time reading and analyzing well-written code from open-source projects. Try to understand how experienced developers structure their solutions, then apply similar patterns to your own projects.
The ability to read code effectively is as important as writing it, yet it’s often overlooked in programming education.
Guided Projects with Decreasing Support
Look for learning resources that gradually remove training wheels. The best coding courses start with heavily guided projects, then slowly reduce support until you’re solving problems independently.
If you can’t find such resources, create this progression yourself by:
- Following a tutorial exactly
- Building a similar project while referencing the tutorial
- Building a related project without looking at the tutorial
The Copy-Then-Create Method
Manually type out existing code examples (don’t copy-paste), then close the reference and try to recreate it from memory. This builds muscle memory for syntax while helping you internalize patterns and approaches.
Musicians and artists have used this technique for centuries – copying masters’ work as a way to develop their own skills before creating original compositions.
Essential Planning Techniques for Coding Projects
One of the biggest reasons developers struggle to start coding is insufficient planning. These techniques will help you create a roadmap before writing code:
User Stories and Acceptance Criteria
Before thinking about code, define exactly what your program needs to do from a user’s perspective. User stories follow a simple format:
“As a [type of user], I want [some goal] so that [some reason].”
For example: “As a student, I want to track assignment deadlines so that I don’t miss submissions.”
For each user story, define acceptance criteria – specific conditions that must be met for the feature to be considered complete.
Flowcharts and Diagrams
Visual representations of your program flow can clarify your thinking before you write any code. Tools like draw.io, Lucidchart, or even pen and paper let you map out how different parts of your program will interact.
These diagrams become invaluable references when you finally start coding, giving you a clear path to follow.
Data Structure Planning
Before implementing functionality, decide how you’ll represent and store your data. For example, if building a contact management app, you might plan:
// Contact data structure
contact = {
id: uniqueIdentifier,
name: string,
email: string,
phone: string,
category: string,
dateAdded: dateObject
}
// Collections
contacts = [contact1, contact2, ...]
categories = ["Family", "Work", "Friends", ...]
This planning helps you visualize how information will flow through your application.
Component Hierarchy (for UI Applications)
For frontend applications, sketch the component structure before coding. This helps you identify reusable elements and understand how data should flow between components.
For example, a social media feed might be broken down into:
- FeedContainer
- PostList
- PostItem
- PostHeader
- PostContent
- PostInteractions
- CommentSection
- PostItem
- SidebarRecommendations
- PostList
Templates and Boilerplates: When to Use Them
Starting with templates or boilerplate code can be an effective way to overcome the blank page problem, but it comes with both benefits and potential pitfalls.
The Benefits of Starting Templates
Templates provide structure and best practices out of the box, helping you focus on your unique implementation rather than standard setup tasks. They’re especially useful for:
- Complex project structures (like full-stack applications)
- Standard architecture patterns
- Configuration-heavy frameworks
- Projects with specific security or performance requirements
For example, using Create React App saves you from the complexity of webpack configuration, letting you focus on component development instead.
When Templates Become a Crutch
However, relying too heavily on templates can prevent you from learning crucial fundamentals. If you never set up a project from scratch, you might miss understanding:
- How different parts of your application connect
- Why certain configuration choices matter
- How to troubleshoot infrastructure issues
- Which components are essential vs. optional
Finding the Right Balance
A good approach is to use templates selectively while still building some projects from scratch:
- For learning: Build simple projects without templates to understand fundamentals
- For practice: Occasionally set up projects manually even when templates exist
- For production: Use well-maintained templates for efficiency and best practices
When using templates, take time to understand what they provide rather than treating them as magic. Read through the generated code and documentation to learn why certain choices were made.
Building Your Coding Starter Kit
Over time, experienced developers build their own collection of patterns, snippets, and approaches they reuse across projects. You can accelerate this process by intentionally creating your personal “starter kit.”
Create a Code Snippet Library
Start collecting useful code snippets you find yourself using repeatedly. Most code editors support snippet libraries that let you insert common patterns with a few keystrokes.
For example, you might save snippets for:
- Setting up event listeners
- Implementing common data structure operations
- Handling form validation
- Making API requests
Develop Personal Templates
Create your own minimal starter templates for different types of projects. Unlike third-party boilerplates, these should be simple enough that you understand every line but comprehensive enough to save you repetitive setup.
For example, a personal vanilla JavaScript project template might include:
- Basic HTML structure
- CSS reset
- Module loading setup
- Simple folder structure
Build a Reference Architecture Document
Document patterns and approaches you find effective for different scenarios. This becomes your personal programming playbook, helping you make decisions more quickly when starting new projects.
Your reference might include notes on:
- Preferred ways to structure different types of applications
- Decision trees for choosing technologies
- Common pitfalls and how to avoid them
- Performance optimization strategies
Overcoming Emotional and Psychological Barriers
The technical strategies above are essential, but addressing the emotional aspects of coding struggles is equally important.
Embracing the “Ugly First Draft”
Writers have long embraced the concept of the “ugly first draft” – giving yourself permission to write poorly at first, knowing you can refine later. Apply this same principle to coding.
Your first implementation doesn’t need to be elegant, optimized, or even complete. Its only job is to exist as a starting point you can improve. As the saying goes, “You can’t edit a blank page.”
Reframing Failure as Learning
Every error message, bug, and design mistake is valuable feedback, not evidence of incompetence. Experienced developers encounter problems constantly – they just have more practice solving them.
Keep an “error journal” documenting problems you encounter and how you solved them. Over time, you’ll see how much you’re learning through these challenges.
Building a Support System
Programming in isolation makes starting from scratch much harder. Connect with other learners through:
- Local meetup groups
- Online communities like Dev.to, Reddit’s r/learnprogramming, or Discord servers
- Pair programming sessions
- Code review exchanges
Sharing your struggles normalizes the experience and provides encouragement when you’re stuck.
Tracking Your Progress
It’s easy to focus on how much you don’t know and forget how far you’ve come. Keep a learning journal or portfolio that documents your projects, even the simple ones.
Reviewing this record when you feel stuck reminds you that you’ve overcome similar challenges before and can do so again.
Real-World Examples: From Blank Page to Working Code
Let’s walk through a practical example of starting a project from scratch, applying the principles discussed above.
Example Project: A Simple Task Timer
Imagine we want to build a web application that lets users time how long they spend on different tasks.
Step 1: Define User Stories
Let’s start by defining what our application needs to do:
- As a user, I want to create named tasks I can time
- As a user, I want to start, pause, and reset timers for each task
- As a user, I want to see a log of time spent on each task
- As a user, I want my tasks and times to persist when I close the browser
Step 2: Sketch the Interface
Before coding, we’d sketch a simple interface showing:
- A form to create new tasks
- A list of tasks with timer controls
- A time log section
Step 3: Plan Data Structures
We need to decide how to represent our data:
// Task object
task = {
id: uniqueString,
name: string,
timeSpent: number (milliseconds),
isRunning: boolean,
timeEntries: [
{
start: timestamp,
end: timestamp,
duration: number
}
]
}
// Application state
appState = {
tasks: [task1, task2, ...],
activeTaskId: string or null
}
Step 4: Write Pseudocode for Core Functions
Next, we outline the main functions we’ll need:
// Task management
function createTask(name) {
// Create new task object
// Add to tasks array
// Update storage
// Update UI
}
function deleteTask(id) {
// Remove task from array
// Update storage
// Update UI
}
// Timer functionality
function startTimer(taskId) {
// Set task as running
// Record start time
// Start interval to update elapsed time
// Update UI
}
function pauseTimer(taskId) {
// Set task as not running
// Calculate duration
// Add to time entries
// Clear interval
// Update storage
// Update UI
}
function resetTimer(taskId) {
// Reset time spent
// Clear time entries
// Update storage
// Update UI
}
// Storage
function saveToLocalStorage() {
// Convert state to JSON
// Save to localStorage
}
function loadFromLocalStorage() {
// Read from localStorage
// Parse JSON
// Update state
// Update UI
}
Step 5: Start with HTML Structure
Now we can begin actual coding with a basic HTML structure:
<div class="app-container">
<h2>Task Timer</h2>
<form id="task-form">
<input type="text" id="task-input" placeholder="What are you working on?">
<button type="submit">Add Task</button>
</form>
<div id="tasks-container">
<!-- Tasks will be added here dynamically -->
</div>
<div id="time-log">
<h3>Time Log</h3>
<div id="log-entries">
<!-- Log entries will be added here dynamically -->
</div>
</div>
</div>
Step 6: Implement One Feature at a Time
With this foundation, we’d implement features incrementally, starting with the simplest (like adding a task) and gradually adding complexity (like the timer functionality).
This approach breaks down the intimidating blank page into manageable steps, each building on the last.
Advanced Techniques for Experienced Developers
Even experienced developers struggle with starting new projects, especially in unfamiliar domains. Here are strategies for advancing beyond the intermediate level:
Test-Driven Development (TDD)
TDD flips the traditional coding process by writing tests before implementation. This approach provides several benefits for starting from scratch:
- Tests act as specifications, clarifying what your code needs to do
- You focus on one small piece of functionality at a time
- The red-green-refactor cycle creates a clear workflow
- You build confidence through passing tests
While TDD has a learning curve, it provides valuable structure when facing complex projects.
Domain-Driven Design (DDD)
For larger applications, DDD principles help you model complex domains before worrying about implementation details. Key practices include:
- Developing a ubiquitous language for your domain
- Identifying bounded contexts and their relationships
- Modeling entities, value objects, and aggregates
This approach shifts focus from “how to code” to “what problem am I solving,” often making the starting point clearer.
Architecture Decision Records (ADRs)
When starting complex projects, document your architectural decisions and their rationales. This practice:
- Forces you to articulate your reasoning
- Creates a reference for future decisions
- Helps identify gaps in your thinking
- Provides context for team members
ADRs are especially valuable when working on teams or on projects you’ll maintain long-term.
When to Ask for Help (and How to Do It Effectively)
Knowing when and how to seek help is a crucial skill for overcoming coding blocks.
The Right Time to Ask for Help
Follow the “15-minute rule”: If you’re completely stuck after 15 minutes of focused troubleshooting, it’s time to consider seeking help. This timeframe is:
- Long enough to push yourself to solve problems independently
- Short enough to prevent wasting hours on simple issues
However, this doesn’t mean immediately asking others. First, try:
- Searching for similar problems online
- Checking documentation
- Taking a short break to reset your thinking
Crafting Effective Questions
When you do need to ask for help, whether on Stack Overflow, Discord, or from a mentor, follow these principles:
- Describe what you’re trying to accomplish (the goal, not just the problem)
- Show what you’ve already tried and researched
- Include minimal, reproducible examples of your code
- Be specific about the error or unexpected behavior
- Format your question for readability
Well-formed questions are more likely to receive helpful answers and demonstrate respect for others’ time.
Learning from Answers
When you receive help, treat it as a learning opportunity, not just a solution:
- Ask follow-up questions about why the solution works
- Document what you learned for future reference
- Try to apply the principle to similar problems
- Express gratitude to those who helped you
Conclusion: Embracing the Journey from Blank Page to Working Code
The struggle to start coding from scratch isn’t a sign of inadequacy – it’s a normal part of the development process that affects programmers at all levels. The blank page challenge combines technical hurdles with psychological barriers, creating a uniquely difficult moment in the coding journey.
By understanding the root causes of this struggle and applying structured approaches to overcome it, you can transform the intimidating blank editor into an opportunity for growth and creativity. Remember that every experienced developer has faced this same challenge countless times.
The strategies we’ve explored – from pseudocode and planning techniques to psychological shifts and scaffolding approaches – provide a toolkit you can apply whenever you face the blank page problem. Over time, starting from scratch will become less intimidating as you build confidence in your ability to break down problems and implement solutions step by step.
Perhaps most importantly, recognize that coding is not about writing perfect code on the first try. It’s an iterative process of exploration, experimentation, and refinement. Give yourself permission to start with imperfect code, knowing you can improve it once it exists.
The blank page may always offer a moment of hesitation, but with practice and the right approach, it will transform from an obstacle into the exciting first step of bringing your ideas to life through code.