Why You Can’t See the Forest for the Trees in Programming
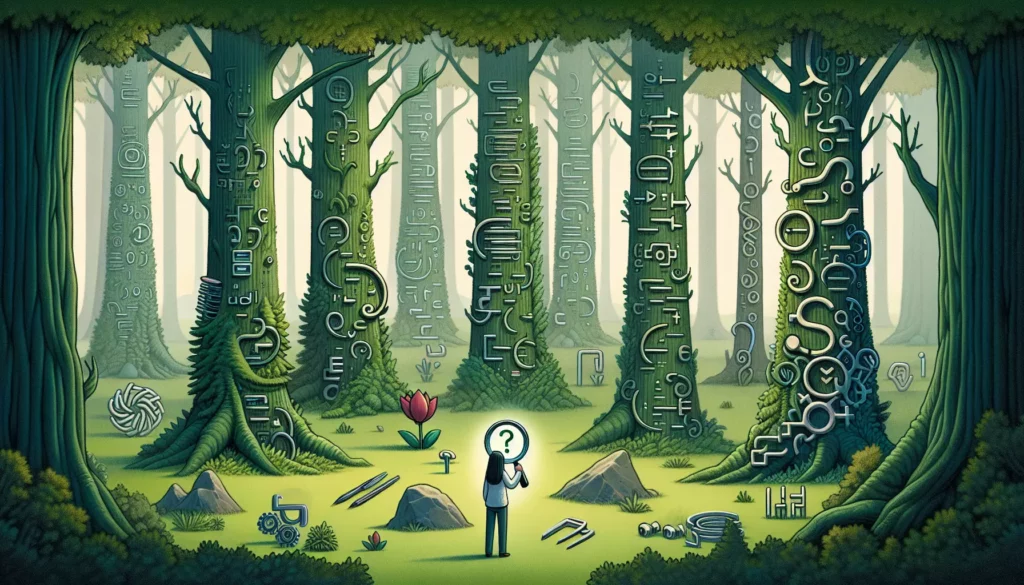
Programming is a fascinating journey filled with countless details, intricate logic, and complex systems. As developers, we often find ourselves deeply immersed in the minutiae of our code, focusing intently on individual lines, functions, or modules. This laser focus is sometimes necessary but can lead to a common phenomenon known as “not seeing the forest for the trees.” In the programming world, this means becoming so engrossed in technical details that we lose sight of the bigger picture.
This article explores why programmers often struggle with this perspective issue, how it impacts our work, and practical strategies to maintain a balanced view that embraces both detailed implementation and broader architectural vision.
Understanding the “Forest vs. Trees” Problem in Programming
The idiom “can’t see the forest for the trees” perfectly captures a challenge that plagues many programmers, from novices to seasoned professionals. Let’s break down what this means in a coding context:
The Trees: Code-Level Details
In programming, the “trees” represent the individual components of our work:
- Individual lines of code
- Function implementations
- Specific algorithms
- Syntax details
- Local optimizations
- Debugging specific issues
The Forest: The Bigger Picture
The “forest” represents the larger context and purpose:
- Overall system architecture
- User experience and needs
- Business goals and requirements
- Long term maintainability
- Cross functional impacts
- Technical debt considerations
When we fixate too much on the trees, we risk creating solutions that are technically impressive but miss the mark in terms of overall effectiveness, maintainability, or alignment with actual needs.
Why Programmers Get Lost in the Details
There are several reasons why programmers tend to focus excessively on code-level details at the expense of the bigger picture:
1. The Nature of Programming Education
Most programming education starts with syntax and small, isolated problems. We learn to write functions before we learn system design. This bottom-up approach is practical but can establish a pattern of thinking that prioritizes implementation details over system thinking.
Consider how most coding tutorials begin:
// A typical first programming lesson
function helloWorld() {
console.log("Hello, World!");
}
helloWorld();
This focus on small, discrete units of code is necessary for learning, but it can create a mindset that doesn’t naturally zoom out to consider broader contexts.
2. The Satisfaction of Solving Concrete Problems
There’s an immediate dopamine hit that comes from fixing a bug or optimizing a function. The feedback loop is tight and rewarding. By contrast, architectural improvements often don’t provide the same immediate gratification.
When we optimize a sorting algorithm and see execution time drop from 500ms to 50ms, we get immediate validation. The benefits of good architecture might not be apparent for months or years.
3. Technical Depth as Identity
Many programmers take pride in their technical depth and specialized knowledge. This expertise is valuable but can sometimes lead to overemphasis on technical elegance rather than practical effectiveness.
For example, a developer might implement a complex caching mechanism using advanced data structures when a simple solution would meet all actual requirements with less complexity.
4. Cognitive Load and Context Switching
Holding an entire system in your head is mentally taxing. It’s often easier to focus on one component at a time, especially when working on complex systems.
As systems grow in complexity, the cognitive effort required to maintain a holistic view increases exponentially. It becomes tempting to narrow focus as a coping mechanism.
The Cost of Missing the Forest
When programmers become too fixated on implementation details without sufficient attention to the broader context, several problems can emerge:
1. Overengineered Solutions
Without a clear view of actual requirements and constraints, developers might build unnecessarily complex solutions. This is sometimes called “gold plating” or “architecture astronautics.”
Consider a developer tasked with creating a simple contact form who implements a complex state management system, custom validation library, and abstracted API layer when a much simpler solution would suffice.
2. Misaligned Priorities
Focus on technical details can lead to spending time optimizing aspects of the system that don’t meaningfully impact user experience or business outcomes.
For instance, a team might spend weeks optimizing database queries to save milliseconds in a process where users are perfectly content with the current performance, while neglecting features that would provide actual business value.
3. Maintainability Issues
Code written with a narrow focus often creates maintainability problems. What seems clever or efficient at the function level might create complexity at the system level.
// Clever but context-free implementation
const processData = data =>
Object.entries(data).reduce((acc, [k, v]) =>
({...acc, [k]: typeof v === "object" ? processData(v) : v * 2}), {});
This function might be efficient, but without context or documentation, future developers (including your future self) will struggle to understand its purpose and constraints.
4. Poor Integration with Other Systems
Focusing too narrowly can result in components that don’t integrate well with other parts of the system or with external systems.
For example, a developer might create a beautifully optimized authentication system that doesn’t properly interface with the company’s existing single sign-on infrastructure.
5. Resistance to Change
Developers who are too invested in the details of their implementation often resist changes that would require significant rework, even when those changes would better serve the overall system goals.
This attachment to code can lead to the “sunk cost fallacy” in technical decision making, where teams continue down suboptimal paths because they’ve already invested significantly in the current approach.
Case Study: The Login System Rewrite
Let’s consider a hypothetical but realistic scenario that illustrates this problem:
A software team was tasked with updating their application’s login system. The lead developer, known for their security expertise, decided to implement a sophisticated authentication system with multiple layers of encryption, complex password policies, and an intricate token management system.
The implementation was technically impressive. The code was clean, well-tested, and followed security best practices. However, several problems emerged:
- The new system increased login time from 1 second to 3 seconds
- Users found the password requirements confusing and frustrating
- The team spent 3 months on the implementation when the roadmap had allocated 3 weeks
- Integration with mobile apps required significant additional work not initially scoped
- The complexity made it difficult for other team members to understand and maintain
What went wrong? The developer focused on the trees (security implementation details) at the expense of the forest (user experience, project timeline, system integration, and maintainability). While security was important, the solution didn’t balance all the necessary considerations.
A more balanced approach might have involved:
- Analyzing actual security threats and requirements
- Setting clear performance budgets for the authentication process
- Conducting user testing on password policies
- Considering integration requirements from the beginning
- Balancing security with usability and development constraints
Finding Balance: Strategies for Seeing Both Forest and Trees
Maintaining perspective is a skill that can be developed. Here are strategies to help programmers maintain both detailed and holistic views of their work:
1. Start with the Why
Before diving into implementation, ensure you understand the purpose of what you’re building. Ask questions like:
- Who will use this feature and what problem does it solve for them?
- How does this component fit into the larger system?
- What are the actual requirements versus nice-to-haves?
- What constraints (time, resources, compatibility) should influence the solution?
Documenting these considerations before writing code can serve as a valuable reference point when you find yourself deep in implementation details.
2. Design Before You Code
Resist the urge to jump straight into coding. Spend time on design, even for smaller features. This doesn’t necessarily mean formal UML diagrams, but could be as simple as:
- Sketching the component structure on paper
- Writing pseudocode that outlines the approach
- Creating a simple API contract for how components will interact
- Listing potential edge cases and how they’ll be handled
This planning phase creates a map that helps maintain orientation when you’re deep in the details of implementation.
3. Regular Zoom Out Sessions
Schedule deliberate times to step back from the details and reassess the broader context. This could be:
- A personal review at the end of each day
- Weekly architecture discussions with the team
- Milestone-based evaluations of how implementation aligns with goals
During these sessions, ask questions like “Is this approach still aligned with our overall goals?” and “Have we discovered anything that should change our approach?”
4. Seek Diverse Perspectives
Different team members often have different natural tendencies toward detail or big-picture thinking. Leverage this diversity through:
- Code reviews that address both implementation details and architectural fit
- Cross-functional discussions with product managers, designers, and other stakeholders
- Pairing sessions between detail-oriented and big-picture developers
These interactions can highlight blind spots in your thinking and broaden your perspective.
5. Use Appropriate Abstractions
Good abstractions allow you to manage complexity by hiding appropriate details while exposing the important concepts. When designing systems:
- Create clear boundaries between components
- Define intuitive interfaces that express intent
- Use naming that reflects domain concepts rather than implementation details
Consider this example of an abstraction that balances detail and context:
// Instead of exposing implementation details
function calculateTaxForOrder(order, taxRates, exemptions, discounts) {
// Complex tax calculation logic
}
// Create an abstraction that handles details but communicates intent
class TaxCalculator {
constructor(taxConfiguration) {
this.config = taxConfiguration;
}
calculateTaxForOrder(order) {
// Complex tax calculation logic hidden inside
}
}
The second approach hides implementation details while clearly communicating the purpose and responsibility of the component.
6. Document Context, Not Just Code
Comments and documentation should explain not just how code works, but why it exists and what role it plays. Consider these two documentation approaches:
// Detail-focused documentation
/**
* Iterates through the array using quicksort algorithm
* with pivot selection optimization for performance.
* O(n log n) average case complexity.
*/
function sortItems(items) {
// Implementation
}
Versus:
/**
* Sorts items for display in the user dashboard.
*
* Uses quicksort for performance as user dashboards typically
* display 50-100 items and need to render in <200ms.
*
* Related components:
* - UserDashboard: Consumes this sorted data
* - ItemDataFetcher: Provides the raw data to be sorted
*/
function sortItems(items) {
// Implementation
}
The second example provides context that helps maintain the connection between implementation details and system purpose.
Balancing Act: When to Focus on Trees vs. Forest
Different situations call for different levels of focus. Here’s a guide for when to zoom in versus zoom out:
When to Focus on the Trees (Details)
- Performance Optimization: When optimizing critical paths, the details matter tremendously.
- Security Implementation: Security vulnerabilities often hide in implementation details.
- Debugging: Tracking down bugs usually requires diving deep into specific code paths.
- API Design: The specifics of parameter names, types, and return values create the contract other code will depend on.
When to Focus on the Forest (Big Picture)
- Initial Planning: When starting a new project or feature, the broader context should drive decisions.
- Architectural Reviews: Evaluating how components fit together requires a system-level view.
- Prioritization: Deciding what to build next should be driven by overall goals, not implementation preferences.
- Technical Debt Assessment: Understanding which areas need refactoring requires evaluating their impact on the system as a whole.
The Ideal: Fluid Movement Between Perspectives
The most effective developers can move fluidly between detailed and holistic perspectives, choosing the right level of focus for each situation and regularly validating one against the other.
This skill develops with experience but can be deliberately practiced by:
- Regularly asking “How does this detail impact the overall system?”
- When making architectural decisions, considering “What implementation challenges might this create?”
- After focusing on details for a period, deliberately stepping back to reassess context
Tools and Practices That Help Maintain Perspective
Several development practices and tools can help maintain a balanced perspective:
1. Domain-Driven Design (DDD)
DDD practices help align code structure with business concepts, making it easier to maintain connection between implementation and purpose:
- Ubiquitous language ensures code terminology matches business terminology
- Bounded contexts provide clear boundaries for different parts of the system
- Aggregates and entities model real-world concepts rather than technical constructs
2. Agile Development Practices
Several agile practices specifically help maintain perspective:
- User Stories: Frame work in terms of user needs rather than technical implementation
- Sprint Reviews: Regularly demonstrate working software to stakeholders to validate direction
- Retrospectives: Reflect on both technical implementation and process effectiveness
3. Architecture Decision Records (ADRs)
ADRs document the context, considerations, and rationale behind significant technical decisions. They help teams:
- Remember why certain approaches were chosen
- Understand the constraints and requirements that shaped solutions
- Evaluate when changing circumstances might warrant revisiting decisions
4. Visualization Tools
Tools that visualize system structure can help maintain awareness of the bigger picture:
- Architecture diagrams showing component relationships
- Dependency graphs revealing coupling between modules
- User journey maps connecting technical components to user experiences
5. Metrics and Monitoring
Well-designed metrics connect implementation details to system-level outcomes:
- Performance dashboards showing the impact of code changes on system behavior
- User-centered metrics that reveal how technical decisions affect user experience
- Business metrics that connect technical work to organizational goals
Learning to See Both Forest and Trees: A Developmental Journey
The ability to maintain perspective is not binary but develops over time. Here’s how this skill tends to evolve through a programmer’s career:
The Beginner: Lost in the Trees
New programmers typically focus almost entirely on making their code work. They’re learning syntax, fighting with compilers, and celebrating when things run without errors. At this stage, the challenge of writing working code consumes most cognitive resources.
For beginners, it’s appropriate to focus primarily on the trees. The forest will come into view gradually as basic coding skills become more automatic.
The Intermediate Developer: Glimpsing the Forest
As developers gain experience, they begin to recognize patterns and understand how components interact. They start to appreciate concepts like separation of concerns, loose coupling, and the impacts of their design choices.
At this stage, developers benefit from deliberately practicing perspective shifts, perhaps with the guidance of more experienced team members who can point out broader implications of design decisions.
The Experienced Developer: Navigating Between Views
Seasoned developers have learned to move fluidly between detailed implementation and system-level thinking. They can dive deep into algorithm optimization while maintaining awareness of how those optimizations affect the overall system.
These developers often serve as bridges between different specialties, helping translate between detailed technical concerns and broader business or user needs.
The Technical Leader: Guiding Others Through the Forest
Those in technical leadership roles must not only maintain their own perspective but help others develop this skill. They create processes, documentation, and mentoring approaches that help team members connect their daily work to larger purposes.
Effective technical leaders create environments where both trees and forest receive appropriate attention, and where the connections between them are made explicit.
Real-World Examples of Forest vs. Trees Thinking
Let’s examine some real-world scenarios that illustrate the difference between detail-focused and context-aware approaches:
Example 1: Database Query Optimization
Trees-focused approach: A developer notices a slow query and spends days optimizing it, adding indexes, rewriting joins, and refactoring the SQL to achieve a 95% performance improvement.
Forest-aware approach: A developer notices a slow query, but before diving into optimization, investigates how frequently the query runs, who depends on its results, and whether caching or denormalization might be more effective solutions given the overall system architecture and usage patterns.
Example 2: Feature Implementation
Trees-focused approach: A developer receives requirements for a new notification system and immediately begins implementing a sophisticated pub/sub architecture with multiple delivery channels, prioritization algorithms, and a custom scheduling system.
Forest-aware approach: A developer receives the same requirements but first investigates how notifications fit into the user experience, what volume and types of notifications are anticipated, and whether existing services might be leveraged before determining the appropriate implementation complexity.
Example 3: Code Refactoring
Trees-focused approach: A developer encounters legacy code and embarks on a massive refactoring to apply the latest patterns and practices, converting everything to use modern language features and restructuring the codebase.
Forest-aware approach: A developer encounters the same legacy code but analyzes which parts are most frequently changed, which cause the most bugs, and which impact critical user journeys, then applies targeted refactoring to provide the greatest system-level benefit with controlled risk.
Conclusion: Cultivating Perspective in Programming
The ability to see both the forest and the trees in programming isn’t just a nice-to-have skill—it’s increasingly essential in a world of complex, interconnected systems. Developers who can navigate between detailed implementation and system-level thinking deliver more effective solutions, collaborate better with diverse stakeholders, and find greater satisfaction in their work by understanding how their code serves larger purposes.
Cultivating this perspective is a career-long journey that involves:
- Developing awareness of your natural tendencies toward detail or big-picture thinking
- Deliberately practicing the perspective that doesn’t come naturally to you
- Using tools and practices that help connect implementation details to system purpose
- Creating documentation that captures both how code works and why it exists
- Building teams that balance and value both detail-oriented and big-picture thinkers
By developing the ability to see both forest and trees, developers can create solutions that are not only technically sound but truly effective in serving user needs and business goals. In doing so, they transform from code writers to problem solvers, capable of leveraging technology to create meaningful impact.
The next time you find yourself deep in a coding session, take a moment to look up from your screen and ask: “Am I seeing the whole forest, or just the tree in front of me?” That simple practice of perspective-shifting might just lead to better solutions and more fulfilling work.