Why You Can’t Measure Your Own Improvement in Programming
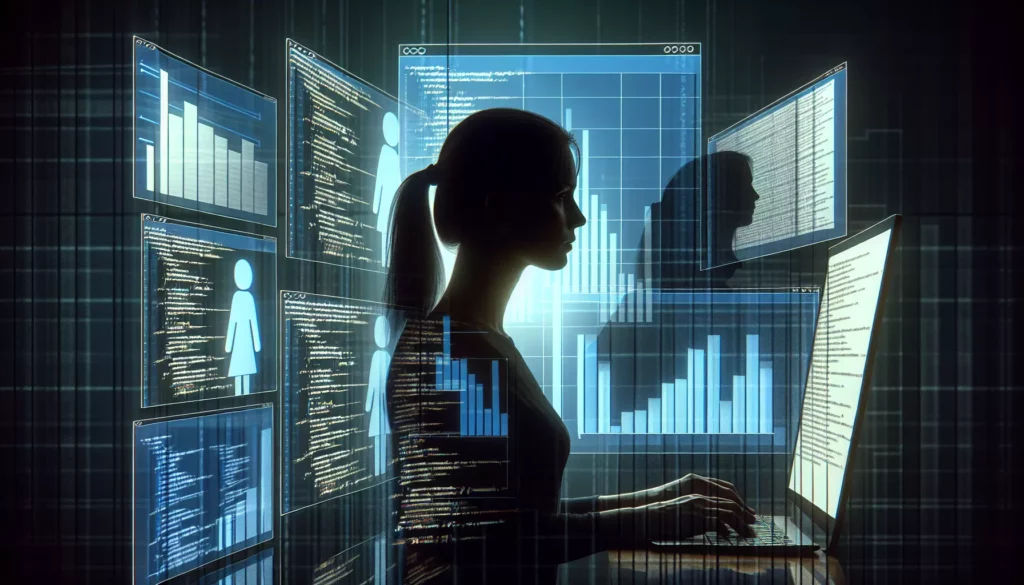
Learning to code is a journey filled with excitement, frustration, breakthroughs, and countless hours staring at error messages. If you’re on this path, you’ve likely wondered at some point: “Am I actually getting better at this?” It’s a question that plagues beginners and experienced developers alike, and the answer is more complex than you might think.
In this article, we’ll explore why measuring your own programming improvement is surprisingly difficult, what obstacles stand in your way, and what strategies you can employ to gauge your progress more effectively.
The Illusion of Measurement
When we learn new skills, we naturally want to track our progress. In many domains, this tracking is straightforward. If you’re learning to play basketball, you can count how many free throws you make out of 100 attempts. If you’re learning a language, you can measure how many words you understand in a conversation.
Programming seems like it should be similarly quantifiable. After all, it’s a technical discipline built on logic and precision. Shouldn’t our improvement be equally precise and measurable?
Unfortunately, no. And here’s why.
The Dunning-Kruger Effect in Programming
The Dunning-Kruger effect is a cognitive bias where people with limited knowledge in a domain overestimate their abilities. This effect is particularly pronounced in programming.
When you first learn to code, everything seems magical. You write a few lines of HTML and CSS, and suddenly you have a webpage! You create your first “Hello, World!” program, and it feels like you’re on the path to becoming the next tech visionary.
This initial confidence is often followed by a steep drop when you encounter your first real challenge. Perhaps it’s debugging a complex issue, understanding recursion, or implementing an algorithm efficiently. Suddenly, the vastness of what you don’t know becomes apparent.
This cognitive bias makes self-assessment particularly tricky. When you’re a beginner, you lack the knowledge to know what you don’t know. When you’re more advanced, you become acutely aware of your limitations and may underestimate your abilities.
The Moving Target Problem
Programming is not a static field. It’s constantly evolving, with new languages, frameworks, libraries, and best practices emerging regularly. This creates what we might call “the moving target problem.”
Imagine you’ve spent months mastering JavaScript and its ecosystem. You feel confident with React, know your way around Node.js, and can handle complex state management. Then suddenly, a new framework emerges that promises to solve all the problems of its predecessors.
Now you face a dilemma: Do you stick with what you know, potentially becoming outdated? Or do you pivot to learn this new technology, essentially starting over on the learning curve?
This constant evolution makes it difficult to establish a stable baseline against which to measure your progress. It’s like trying to measure your height while standing on a boat in rough seas.
The Complexity of Programming Tasks
Another challenge in measuring programming improvement is the vast difference in complexity between tasks. Programming encompasses everything from simple scripts to complex distributed systems, from frontend animations to low-level memory management.
Consider these two scenarios:
- Last year, it took you a week to build a simple todo app with React.
- This year, it took you a month to build a full-stack application with authentication, database integration, and real-time updates.
Are you four times slower now? Of course not. The second task is exponentially more complex than the first. But without context, raw metrics like “time to complete” can be misleading.
This complexity gradient makes linear measurement nearly impossible. It’s not like tracking how fast you can run a mile, where the task remains constant.
The Problem of Invisible Progress
Much of your growth as a programmer happens beneath the surface. It’s not always reflected in the code you write or the projects you complete.
For instance, you might spend weeks learning about design patterns. This knowledge doesn’t immediately translate into faster coding or more features. Instead, it gradually influences how you structure your code, making it more maintainable and extensible over time.
Similarly, hours spent debugging a particularly tricky issue might seem like wasted time. But the troubleshooting skills and system understanding you gain are invaluable, even if they don’t result in new lines of code.
This invisible progress is difficult to measure but often represents the most significant growth in your journey.
The Subjective Nature of Code Quality
What makes code “good” is surprisingly subjective. Is it efficiency? Readability? Maintainability? Elegance? The answer depends on context, team preferences, and specific requirements.
Consider these two implementations of a function to find the maximum value in an array:
// Implementation 1
function findMax(arr) {
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
// Implementation 2
function findMax(arr) {
return Math.max(...arr);
}
The second implementation is shorter and more elegant. But is it better? What if the array is very large, causing a stack overflow with the spread operator? What if readability for junior developers is a priority?
The subjective nature of code quality makes it difficult to establish objective metrics for improvement.
The Plateau Phenomenon
Learning to code often follows a pattern of rapid initial progress followed by plateaus where improvement seems to stall. These plateaus can last for months or even years and can be deeply discouraging.
What makes these plateaus particularly challenging is that they often occur just before significant breakthroughs. You might spend months struggling with a concept like recursion or asynchronous programming, feeling like you’re making no progress, only to have it suddenly “click” in a moment of insight.
During these plateaus, traditional metrics of progress may show no improvement, even though important neural connections are forming beneath the surface.
The Isolation Factor
Many programmers, especially those learning independently or working in small teams, lack regular exposure to different skill levels. Without this context, it’s difficult to gauge where you stand in the broader landscape.
This isolation can lead to distorted self-perception. You might think your code is exceptional because it’s the best you’ve seen, or you might believe you’re falling behind because you compare yourself to experts on GitHub or Stack Overflow.
The lack of a representative sample makes it hard to calibrate your self-assessment.
Better Ways to Track Your Programming Progress
Given these challenges, how can you more effectively gauge your improvement as a programmer? Here are some strategies that go beyond simplistic metrics:
1. Focus on Capabilities, Not Knowledge
Instead of measuring what you know, track what you can build. Can you now create applications that were beyond your abilities six months ago? Can you solve problems that would have stumped you last year?
This capabilities-based approach provides a more practical measure of your growth than trying to quantify knowledge acquisition.
2. Maintain a Programming Journal
Keep a log of challenges you face, how you solve them, and how long they take. Over time, this journal becomes a valuable record of your journey and provides concrete evidence of your progress.
For example, you might note: “April 15: Spent 3 hours debugging an infinite loop in my recursion function. Finally realized I wasn’t incrementing my counter in the base case.”
Six months later, you might write: “October 20: Implemented a recursive solution for traversing a binary tree. Took about 30 minutes, including debugging.”
These entries tell a story of growth that raw metrics could never capture.
3. Embrace Code Reviews
Whether through formal processes at work, open-source contributions, or peer learning groups, code reviews provide invaluable external perspective on your code quality.
Pay attention to how the feedback changes over time. Are reviewers focusing on higher-level concerns rather than basic syntax issues? This evolution in feedback often indicates significant growth.
4. Build a Portfolio of Solved Problems
Platforms like LeetCode, HackerRank, and AlgoCademy offer standardized programming challenges. By regularly solving these problems and tracking your solutions, you can observe how your approach to problem-solving evolves.
Review solutions you wrote months or years ago. You’ll often be surprised by how much clearer, more efficient, or more elegant your current solutions are.
5. Teach Others
Teaching is one of the most effective ways to gauge your understanding. When you can explain a concept clearly to someone else, it demonstrates a level of mastery that goes beyond mere familiarity.
Consider starting a blog, creating tutorials, or mentoring less experienced programmers. The questions you receive and your ability to answer them will highlight both your strengths and areas for growth.
6. Track Your “Time to Solution”
For comparable tasks, monitor how long it takes you to implement solutions. While this metric has limitations due to varying complexity, it can provide useful insights when the context is similar.
For instance, if implementing authentication features used to take you a full day but now takes only a couple of hours, that’s a meaningful indicator of growth.
7. Measure Your Debugging Efficiency
Debugging skills are a critical component of programming expertise. Track how quickly you can identify and fix bugs, particularly in unfamiliar codebases.
As you grow, you’ll likely notice that you develop more systematic approaches to debugging and can narrow down the source of issues more efficiently.
The Role of External Validation
While self-assessment has its limitations, external validation can provide valuable benchmarks for your progress. Here are some forms of external validation to consider:
Technical Interviews
Love them or hate them, technical interviews provide standardized challenges that test your problem-solving abilities, algorithmic thinking, and code quality. Success in these interviews, especially at companies known for rigorous hiring processes, can be a meaningful indicator of growth.
Professional Certifications
While certifications have varying levels of respect in the industry, some provide legitimate validation of specific skills. Cloud certifications from AWS, Azure, or Google Cloud, for example, test practical knowledge that’s relevant in professional settings.
Peer Recognition
Recognition from respected colleagues or the broader community can be a powerful affirmation of your growth. This might come in the form of GitHub stars on your projects, upvotes on your Stack Overflow answers, or simply colleagues coming to you for help with difficult problems.
Professional Advancement
Career progression, whether through promotions, job changes, or increasing responsibilities, often reflects growing technical capabilities. While not a pure measure of programming skill, it represents a real-world validation of your value as a developer.
The Importance of Specific Goals
Measuring general “improvement” in programming is challenging partly because it’s too vague. Setting specific, achievable goals makes progress more tangible and measurable.
For example, instead of “become a better JavaScript developer,” you might aim to:
- Implement a small project using the Proxy API
- Refactor an existing application to use TypeScript
- Contribute to an open-source JavaScript library
- Build a custom React hook that solves a specific problem
These concrete goals provide clear milestones and make it easier to recognize when you’ve made progress.
Comparing Against Yourself, Not Others
One of the most destructive habits in measuring programming improvement is comparing yourself to others, especially those with different backgrounds, experiences, and resources.
The only fair comparison is to your past self. Are you more capable than you were six months ago? Can you build things that your past self would have found challenging?
This self-referential approach to measurement is both more accurate and more motivating than external comparisons.
The Expertise Paradox
Interestingly, as you become more advanced as a programmer, it often becomes harder to feel like you’re improving. This is sometimes called the “expertise paradox.”
When you’re a beginner, progress is rapid and visible. You go from not being able to write a for loop to building simple applications in a matter of weeks. But as you advance, improvements become more subtle and specialized.
An expert JavaScript developer might spend months deepening their understanding of the event loop or optimizing garbage collection patterns. These improvements are real and valuable but don’t provide the same dopamine hit as those early wins.
Recognizing this paradox can help you appreciate the more nuanced growth that comes with advancing expertise.
The Impact of Learning Environment
Your learning environment significantly affects both your rate of improvement and your ability to measure it. Consider these different contexts:
Self-Taught Learning
If you’re learning independently, you have the freedom to explore topics that interest you but may lack structured feedback. In this environment, creating artificial benchmarks becomes crucial.
Platforms like AlgoCademy can provide this structure through guided lessons, interactive challenges, and AI-powered feedback on your code. These tools offer objective measures of progress that self-taught programmers might otherwise lack.
Bootcamp or Formal Education
In structured educational environments, you benefit from a curriculum designed to build skills progressively and instructors who can assess your work. The built-in assessments and projects provide natural checkpoints for measuring growth.
However, these environments sometimes emphasize breadth over depth, potentially creating gaps in fundamental understanding that become apparent only later in your career.
Professional Development
In a professional setting, improvement is often measured by your increasing value to the organization. Can you take on more complex tasks? Are you spending less time on implementation? Are you helping others solve problems?
These practical measures often matter more than theoretical knowledge or algorithmic prowess, though the specific metrics vary widely by company and role.
The Role of Deliberate Practice
Research on expertise development consistently highlights the importance of deliberate practice—focused, challenging work that targets specific weaknesses and incorporates immediate feedback.
In programming, deliberate practice might involve:
- Solving algorithmic challenges slightly beyond your current abilities
- Implementing the same functionality using different approaches or paradigms
- Rewriting existing code to improve its performance, readability, or maintainability
- Building projects that force you to use unfamiliar technologies or techniques
The key is that the practice must be challenging enough to push your boundaries but not so difficult that it becomes demoralizing.
Tracking your engagement in deliberate practice can be a more reliable indicator of long-term growth than measuring immediate outcomes.
The Hierarchy of Programming Skills
Programming improvement isn’t one-dimensional. It encompasses a hierarchy of skills that develop at different rates:
Syntax and Language Features
At the most basic level, you need to understand the syntax and features of your programming languages. This knowledge is relatively easy to acquire and measure through simple coding exercises.
Algorithmic Thinking
Beyond syntax, you need to develop the ability to break down problems and design efficient algorithms. This skill is more challenging to measure but can be assessed through algorithmic challenges and problem-solving exercises.
System Design
At a higher level, programming involves designing systems that are scalable, maintainable, and resilient. This skill is difficult to measure objectively but becomes evident in the success of larger projects and collaborations.
Domain Expertise
The most advanced programmers combine technical skills with deep domain knowledge, allowing them to create solutions that address complex real-world problems. This expertise is highly contextual and often visible only to others working in the same domain.
Recognizing which level of this hierarchy you’re currently focusing on can help you set appropriate expectations for measurement and progress.
The Role of Feedback Loops
Effective learning requires tight feedback loops—mechanisms that quickly tell you whether you’re on the right track. In programming, these feedback loops take many forms:
- Compiler errors and runtime exceptions
- Unit tests and automated checks
- Code reviews and peer feedback
- User interaction with your applications
- Performance metrics and monitoring
The quality and speed of these feedback loops significantly impact your rate of improvement. A development environment with robust testing, quick builds, and regular code reviews will accelerate your growth compared to one where feedback is delayed or absent.
Assessing the quality of your feedback loops can provide indirect insight into your potential for improvement, even when direct measurement is challenging.
Embracing the Uncertainty
After exploring all these challenges and strategies, we come to an important conclusion: perfect measurement of programming improvement is impossible. And that’s okay.
Programming is as much an art as it is a science. Like artists, programmers develop an aesthetic sense, a personal style, and an intuitive understanding that defies quantification.
Rather than obsessing over precise metrics, embrace the uncertainty of the journey. Focus on the joy of creation, the satisfaction of solving problems, and the continuous expansion of your capabilities.
Trust that if you consistently engage with challenging problems, seek feedback, and reflect on your work, improvement will happen naturally, even if you can’t always measure it precisely.
Conclusion
Measuring your programming improvement is inherently difficult due to cognitive biases, the moving target nature of the field, the complexity gradient of tasks, invisible progress, subjective quality standards, learning plateaus, and isolation from context.
Rather than relying on simplistic metrics, focus on tracking capabilities, maintaining a programming journal, seeking code reviews, building a portfolio of solved problems, teaching others, and monitoring your efficiency in comparable tasks.
Remember that the most meaningful comparison is to your past self, not to others with different circumstances. Set specific goals that provide concrete milestones, and recognize that as you advance, progress becomes more nuanced and specialized.
Finally, embrace the uncertainty inherent in the learning process. Programming is a lifelong journey of growth and discovery, not a destination with a clear finish line.
By adopting these more sophisticated approaches to tracking your progress, you’ll develop a more accurate understanding of your growth as a programmer and maintain the motivation to continue improving, even when the path forward isn’t always clear.
What strategies have you found effective for measuring your programming improvement? Share your experiences in the comments below!