Why You Can’t Learn Programming By Just Reading About It
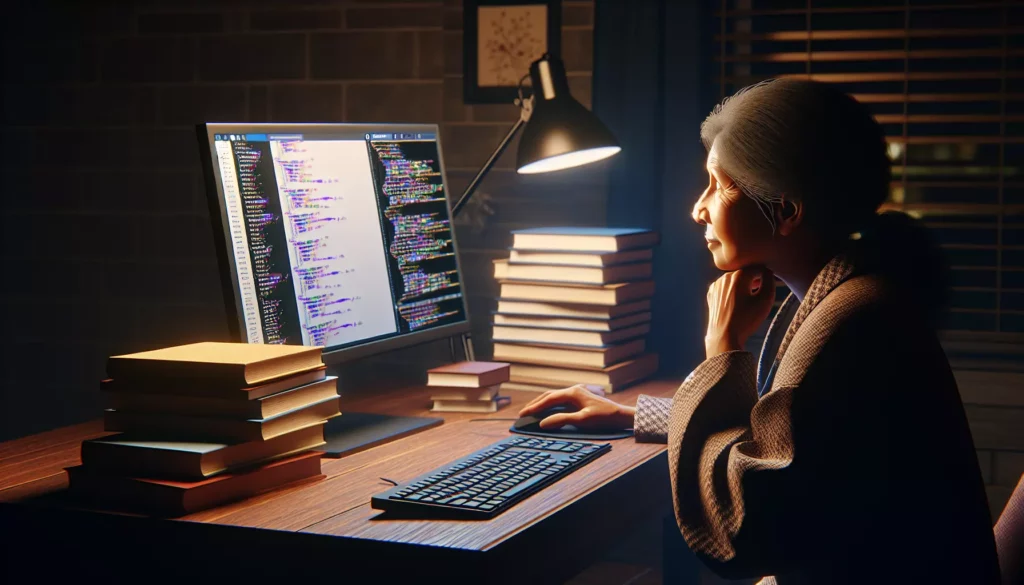
Have you ever tried to learn swimming by reading a book? Or mastering the piano through an article? Programming is no different. Despite the countless books, tutorials, and blog posts available on coding, there’s a fundamental truth that many beginners overlook: you cannot truly learn programming by just reading about it.
In this comprehensive guide, we’ll explore why active practice is essential for programming mastery, the limitations of passive learning, and how to effectively combine reading with hands-on coding experience to become a proficient developer.
The Illusion of Understanding
One of the most dangerous traps for aspiring programmers is what I call the “illusion of understanding.” This happens when you read a programming concept, nod along thinking “this makes sense,” but then struggle to implement it when sitting in front of a blank code editor.
This phenomenon isn’t unique to programming. It’s a well-documented cognitive bias called the “illusion of explanatory depth” – people believe they understand complex systems more thoroughly than they actually do. With programming, this illusion is particularly deceptive.
Why Reading Creates False Confidence
When you read about programming concepts, several things happen:
- The author presents ideas in a logical, pre-organized fashion
- Examples are carefully selected to clearly demonstrate the concept
- Complex edge cases are often simplified or omitted
- The cognitive load of syntax and implementation details is handled for you
All of this creates a smooth learning experience that can mask the true complexity of applying these concepts. You might read about loops, conditionals, or object-oriented programming and think, “I get this!” But understanding the concept is just the beginning.
The Knowledge-Application Gap
There’s a substantial gap between knowing about programming and knowing how to program. This gap manifests in several ways:
Syntax Challenges
Reading code is far easier than writing it from scratch. When reading, you only need to recognize syntax. When writing, you must recall specific syntax rules, which is a more demanding cognitive task.
For example, consider this simple Python function:
def calculate_average(numbers):
if not numbers:
return 0
return sum(numbers) / len(numbers)
Reading this is straightforward. But when writing it yourself, you might struggle with:
- Exact function definition syntax
- How to check for an empty list
- The precise methods to calculate a sum and length
- Proper indentation requirements
Problem-Solving Barriers
Programming is fundamentally about problem-solving. Reading demonstrates solutions, but doesn’t teach you the process of arriving at those solutions independently.
When you read a tutorial, you’re following someone else’s problem-solving path. But real programming requires:
- Breaking down problems into manageable components
- Identifying appropriate algorithms and data structures
- Developing a solution strategy
- Testing and iteratively improving your approach
These skills only develop through practice and experience with varied problems.
Debugging Deficiency
Perhaps the most significant gap is in debugging skills. Reading polished, working code doesn’t prepare you for the reality that most of your programming time will be spent fixing broken code.
Debugging requires:
- Interpreting error messages
- Tracing program execution
- Forming and testing hypotheses about what’s wrong
- Using debugging tools effectively
These crucial skills remain undeveloped if you only read about programming.
The Science Behind Learning to Code
Cognitive science offers valuable insights into why active programming practice is essential for learning.
Building Mental Models
Effective programming requires building accurate mental models of how code executes. These models allow you to simulate program behavior in your mind, predict outcomes, and reason about code.
Research in cognitive science shows that mental models develop through active engagement, not passive consumption. When you write and debug code, you’re constantly refining your mental model based on feedback from the computer.
Procedural vs. Declarative Knowledge
Psychology distinguishes between two types of knowledge:
- Declarative knowledge: Knowing facts and concepts (“what” knowledge)
- Procedural knowledge: Knowing how to perform tasks (“how” knowledge)
Reading builds declarative knowledge about programming. You learn concepts, terminology, and patterns. But programming proficiency requires procedural knowledge – the ability to apply these concepts to solve problems.
Procedural knowledge develops through practice, feedback, and repetition. This is why experienced programmers can write code almost automatically, while beginners must consciously think through each step.
The Role of Deliberate Practice
Psychologist Anders Ericsson’s research on expertise development highlights the importance of “deliberate practice” – focused, challenging practice with immediate feedback. For programming, this means:
- Working on problems slightly beyond your current ability
- Receiving immediate feedback (from compilers, tests, or mentors)
- Reflecting on mistakes and adjusting your approach
- Repeatedly practicing core skills until they become automatic
Simply reading about programming provides none of these elements of deliberate practice.
The Missing Elements in Passive Learning
Beyond the cognitive aspects, several practical elements essential to programming proficiency are missing from passive reading:
Error Recognition and Recovery
Programming involves making mistakes – lots of them. Experienced programmers aren’t those who avoid errors; they’re those who efficiently recognize, diagnose, and fix them.
Reading polished tutorials rarely exposes you to the messy reality of error messages, logical bugs, and unexpected behavior. You don’t develop the resilience and problem-solving strategies needed to overcome these challenges.
Environment Setup and Tool Mastery
A significant part of real-world programming involves setting up and managing development environments, using version control, and mastering various tools. These practical skills are rarely covered in depth in programming books and tutorials.
When you only read about programming, you miss learning how to:
- Configure IDEs and text editors
- Manage packages and dependencies
- Use version control systems like Git
- Deploy and run applications in different environments
Handling Ambiguity and Incomplete Information
Programming tutorials present well-defined problems with clear solutions. Real-world programming is messier, involving ambiguous requirements, incomplete information, and multiple valid approaches.
Only through practice do you develop the judgment to make design decisions, choose between alternative implementations, and work effectively with ambiguity.
Real-World Evidence: The Industry Perspective
The technology industry has long recognized that reading alone is insufficient for developing programming skills.
Hiring Practices
Tech companies assess programming ability through:
- Coding challenges that require writing actual code
- Technical interviews focused on problem-solving
- Portfolio reviews showcasing completed projects
Notice what’s missing: questions about programming books you’ve read or tutorials you’ve followed. Companies know that practical experience is the true indicator of programming ability.
The Bootcamp Revolution
The rise of coding bootcamps further demonstrates the industry’s recognition that hands-on practice is essential. Successful bootcamps focus on:
- Project-based learning
- Pair programming
- Realistic coding challenges
- Building portfolio projects
These programs minimize lecture time in favor of active coding experience, recognizing that skills develop through practice.
Professional Development Patterns
Even experienced programmers recognize the limitations of passive learning. When learning new technologies, skilled developers typically:
- Read just enough to understand core concepts
- Immediately build a small project to apply the knowledge
- Use documentation as a reference while coding
- Join communities to ask questions and share code
This pattern of rapid transition from reading to doing is a hallmark of effective programming skill development.
Finding the Right Balance: How Reading Fits In
Despite my emphasis on practice, reading does play a valuable role in learning to program. The key is understanding when and how to incorporate reading into your learning process.
Reading as Foundation
Reading provides essential conceptual foundations. It helps you:
- Learn programming vocabulary and terminology
- Understand core concepts and principles
- Discover best practices and patterns
- Gain exposure to new ideas and approaches
These foundations are necessary but insufficient for programming proficiency.
The Optimal Learning Loop
Instead of reading extensively before coding, consider this more effective learning loop:
- Read briefly: Learn just enough about a concept to start using it
- Code immediately: Apply the concept in a small, focused example
- Encounter problems: Notice what you don’t understand or can’t implement
- Read strategically: Return to reading with specific questions in mind
- Code again: Apply your improved understanding
- Repeat: Continue this cycle, gradually tackling more complex problems
This approach uses reading as a tool within a practice-centered learning process.
Reading Documentation While Coding
One of the most valuable reading skills for programmers is the ability to effectively use documentation while coding. This includes:
- Quickly finding relevant information in documentation
- Understanding API references and examples
- Extracting just what you need to solve your current problem
This skill develops through practice – specifically, the practice of coding while referencing documentation.
Effective Practice Strategies for Programming
Now that we understand why practice is essential, let’s explore how to practice effectively.
Start Small and Build Incrementally
Begin with tiny programs that do one simple thing. As you gain confidence, gradually increase complexity. For example:
- Write a program that prints “Hello, World!”
- Modify it to ask for the user’s name and say hello to them
- Add validation to handle empty inputs
- Store multiple names and greet each person
This incremental approach builds confidence while gradually expanding your skills.
Practice Deliberate Imitation
One powerful learning technique is to study existing code, then try to recreate it from memory:
- Find a small, well-written code example
- Study it carefully, understanding each line
- Close the example and try to rewrite it from memory
- Compare your version to the original
- Note and learn from the differences
This approach bridges reading and coding, helping you internalize patterns and techniques.
Embrace the Struggle
Learning to program inevitably involves struggling with problems you can’t immediately solve. This struggle is not a sign of failure – it’s a necessary part of the learning process.
When you encounter a difficult problem:
- Try to solve it yourself before looking for solutions
- Break it down into smaller parts
- Experiment with different approaches
- Use debugging tools to understand what’s happening
These moments of struggle create the strongest learning experiences.
Build Projects That Interest You
Personal projects provide motivation and context for your learning. Choose projects that:
- Align with your interests
- Solve a problem you actually have
- Start simple but can grow in complexity
- Expose you to different aspects of programming
Working on meaningful projects sustains motivation through the inevitable challenges of learning to code.
Learning Resources That Promote Active Coding
Not all learning resources are created equal. Some encourage active practice more effectively than others.
Interactive Coding Platforms
Platforms like AlgoCademy, Codecademy, and freeCodeCamp integrate reading and practice, prompting you to write code after introducing each concept. These platforms offer:
- Immediate feedback on your code
- Progressive challenges that build on previous concepts
- A structured path from basics to more advanced topics
- Built-in testing to validate your solutions
These resources help bridge the gap between reading and applying concepts.
Project-Based Tutorials
Look for tutorials that guide you through building complete projects, rather than just explaining concepts. Effective project tutorials:
- Start with a clear goal (what you’ll build)
- Break the process into manageable steps
- Explain concepts as they become relevant
- Encourage modifications and extensions
These tutorials provide context for concepts and demonstrate how they work together in real applications.
Coding Challenges and Exercises
Platforms like LeetCode, HackerRank, and Exercism offer structured coding challenges that:
- Focus on specific programming concepts
- Provide immediate feedback through tests
- Offer increasing levels of difficulty
- Often show multiple solutions after you complete a challenge
Regular practice with these challenges builds problem-solving skills and fluency with programming concepts.
Common Pitfalls and How to Avoid Them
Even when focusing on practice, learners often fall into several common traps.
Tutorial Hell
“Tutorial hell” occurs when learners continually follow tutorials without building independent projects. To escape:
- After completing a tutorial, build something similar without following instructions
- Modify tutorial projects with new features
- Set a ratio (e.g., for every tutorial, build one independent project)
Perfectionism Paralysis
Many beginners avoid practice because they fear writing imperfect code. Remember:
- All programmers write bad code sometimes
- Early projects don’t need to be perfect
- Improving code is easier than creating it from scratch
- Even “bad” code that works is valuable learning
Give yourself permission to write imperfect code as you learn.
Isolation
Programming alone limits your growth. Counter this by:
- Joining programming communities and forums
- Finding study partners or mentors
- Sharing your code for feedback
- Participating in pair programming sessions
Other programmers can provide insights and perspectives that accelerate your learning.
Case Studies: Learning Journeys
Let’s examine how these principles play out in real learning journeys.
The Book Collector
Alex spent six months reading programming books and watching video tutorials. He could explain object-oriented programming, algorithms, and data structures in detail. But when he attempted to build a simple web application, he struggled to write even basic functions.
The issue? Alex had developed theoretical knowledge without practical skills. He had to start with small coding exercises to bridge the gap between knowing and doing.
The Pragmatic Learner
Maya took a different approach. She read just enough about JavaScript to understand basic syntax, then immediately built a simple calculator. The project was challenging and her code wasn’t elegant, but the experience taught her more than weeks of reading would have.
As she encountered specific problems, she consulted documentation and tutorials with focused questions. Each small project built both her confidence and competence.
The Balanced Approach
Carlos developed an effective rhythm: read for 20 minutes, code for 40 minutes, reflect for 10 minutes. This pattern allowed him to learn new concepts while immediately applying them.
He maintained a programming journal where he documented challenges and solutions. Over time, he noticed patterns in his learning and could target specific weaknesses with focused practice.
The Path Forward: Balancing Theory and Practice
The most effective approach to learning programming combines thoughtful reading with abundant practice.
The 20/80 Rule for Programming
Consider adopting a modified 80/20 rule for learning programming:
- Spend 20% of your time reading and consuming content
- Spend 80% of your time writing, debugging, and improving code
This ratio ensures you gain necessary knowledge while developing practical skills.
Creating a Sustainable Learning Practice
Sustainable learning requires:
- Consistent practice (daily is ideal)
- Manageable challenges that stretch but don’t overwhelm you
- A supportive community or learning environment
- Regular reflection on your progress and challenges
The goal is to develop habits that support continuous improvement.
Measuring Real Progress
Instead of measuring progress by books read or tutorials completed, track:
- Problems you can solve independently
- Projects you’ve completed
- Concepts you can apply without reference
- Your comfort with debugging and problem-solving
These metrics better reflect your growing programming capabilities.
Conclusion: The Programming Journey
Learning to program is a journey that requires active participation. Reading about programming is like reading about swimming – it might help you understand the principles, but you’ll never learn to swim until you get in the water.
The most effective approach combines targeted reading with abundant practice. Read to understand concepts, but code to develop skills. Embrace the challenges, learn from mistakes, and gradually build your capabilities through deliberate practice.
Remember that every experienced programmer started as a beginner. What distinguishes those who succeed is not how many books they read, but how much code they wrote, how many problems they solved, and how persistently they practiced their craft.
So close this article, open your code editor, and start writing code. Your programming journey becomes real not when you read about coding, but when you begin to code.
FAQ: Learning Programming Through Practice
How much should I read before starting to code?
Read just enough to understand the basic syntax and concepts needed to write simple programs in your chosen language. This might take a few hours, not days or weeks. Then start coding immediately, referring back to resources as needed.
What if I get stuck while practicing?
Getting stuck is a normal part of learning to program. Try to solve the problem yourself for at least 15-30 minutes. If you remain stuck, look for specific help on that issue, then return to coding. Each obstacle you overcome builds problem-solving skills.
How do I know if I’m making progress?
Track your ability to solve problems independently. Can you build programs that you couldn’t a month ago? Are you spending less time stuck on syntax errors? Do you need to consult documentation less frequently? These are better indicators of progress than pages read or videos watched.
Is it better to deeply understand concepts before practicing?
Deep understanding comes through practice, not before it. Start applying concepts with a basic understanding, and your comprehension will deepen through use. Reading can provide initial clarity, but true understanding develops through application.
How do I balance learning fundamentals with building projects?
Integrate fundamental learning into project work. Choose projects that utilize the concepts you want to learn. When you encounter something you don’t understand, learn just enough about it to implement it in your project, then continue building.