Why You Can’t Learn From Other People’s Solutions
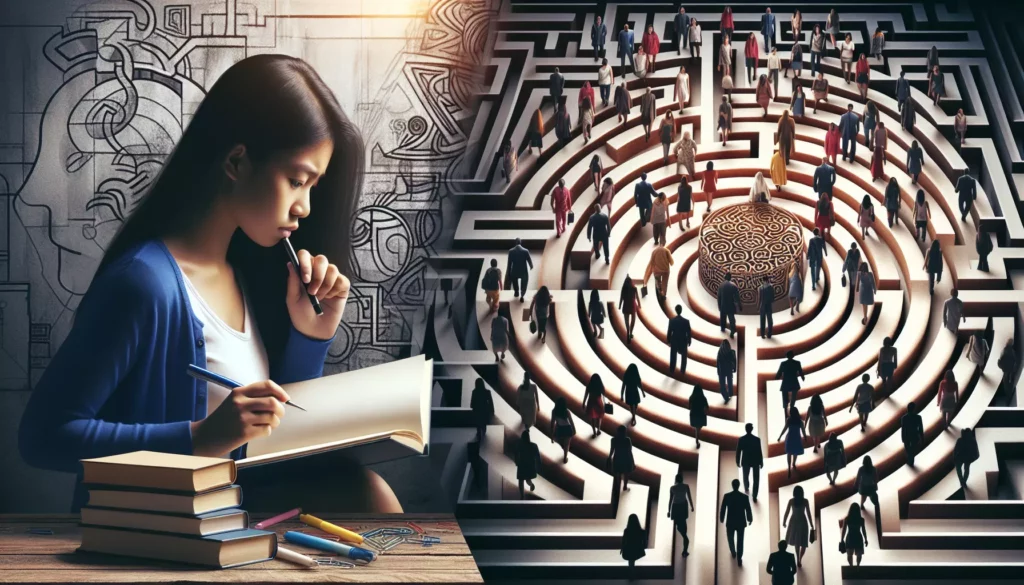
TL;DR: Why You Can’t Learn From Other People’s Solutions
Looking at solutions when you’re stuck creates an “illusion of competence” where you think you understand more than you actually do. Reading code uses recognition memory, but solving problems requires recall memory and deeper mental models. Productive struggle is essential for building lasting programming skills – when you work through challenges, your brain forms stronger neural connections. Instead of peeking at solutions, use structured hints, time-box your struggles, explain problems out loud, or review just enough to get unstuck. The most effective approach combines independent problem-solving with spaced repetition. While studying solutions has its place (after solving independently or learning new concepts), true algorithmic mastery comes from developing your own problem-solving abilities through persistent practice.
In the world of programming and problem solving, there’s a temptation that almost every learner faces: looking at someone else’s solution when you’re stuck. It seems logical. After all, isn’t that how we learn many things in life? By observing others who have already mastered the skill?
But when it comes to algorithmic problem solving and coding challenges, this approach often backfires in subtle yet significant ways. At AlgoCademy, we’ve observed thousands of learners on their journey from beginner programmers to successful technical interview candidates at top tech companies. What we’ve consistently found is that those who truly excel develop their skills through struggle, not shortcuts.
In this article, we’ll explore why simply studying other people’s solutions doesn’t lead to the deep learning and problem solving abilities needed to become an exceptional programmer. We’ll also provide alternative approaches that actually work for building lasting skills.
The False Promise of Learning by Solution
Let’s start with a scenario that might sound familiar: You’re working on a challenging algorithm problem. After 30 minutes of struggle, you feel stuck. Your instinct kicks in: “Let me just see how others solved this.”
You find a clean, elegant solution online. It makes perfect sense as you read it. You understand each line. You even explain it to yourself. “Wow, that was so simple! I get it now,” you think.
But here’s the crucial question: Two weeks later, when faced with a similar but slightly different problem, will you be able to solve it independently?
For most people, the answer is no. And understanding why this happens is key to improving how you learn.
The Illusion of Competence
When you read someone else’s solution, you experience what psychologists call the “illusion of competence.” This cognitive bias tricks your brain into thinking you understand the material more deeply than you actually do.
It’s like watching a professional chef prepare a complex dish. Everything looks straightforward as you observe. “I could do that,” you think. But when you’re alone in your kitchen with the same ingredients, you discover there were dozens of small decisions and techniques that weren’t obvious from just watching.
Programming works the same way. Reading code activates different neural pathways than producing code. Understanding someone else’s solution engages your recognition memory, which is much easier to activate than the recall memory and problem solving abilities needed to create a solution from scratch.
The Missing Mental Models
When you jump straight to a solution, you miss building the mental models that are essential for problem solving. These models are the frameworks your brain constructs to understand and navigate complex domains.
Consider a classic algorithmic challenge like finding all permutations of a string. A concise recursive solution might look elegant and obvious:
function permutations(str) {
if (str.length <= 1) return [str];
const result = [];
for (let i = 0; i < str.length; i++) {
const current = str[i];
const remaining = str.slice(0, i) + str.slice(i + 1);
for (const perm of permutations(remaining)) {
result.push(current + perm);
}
}
return result;
}
Reading this solution might make perfect sense. But developing the mental model that leads to this approach—understanding how to break a permutation problem into smaller subproblems, recognizing the recursive pattern, visualizing the call stack—these crucial mental structures only form through the struggle of attempting to solve the problem yourself.
The Crucial Role of Productive Struggle
Cognitive science research consistently shows that learning happens most effectively during periods of productive struggle. When you grapple with a problem just beyond your current abilities, your brain forms stronger neural connections.
This process, sometimes called “desirable difficulty,” is what transforms information from short-term understanding into long-term learning. It’s the difference between recognizing a solution and being able to produce one.
Think about how children learn to walk. They don’t watch walking tutorials and then immediately stride confidently across the room. They fall repeatedly, adjust, struggle, and gradually build the neural pathways needed for this complex motor skill.
Programming problem solving works similarly. The moments of confusion, the wrong approaches, the debugging—these aren’t wasted time. They’re essential components of building robust mental models.
The Technical Interview Reality
If you’re preparing for technical interviews at major tech companies, the stakes for genuine learning are even higher. In these high-pressure situations, the illusion of competence quickly dissolves.
Consider this scenario: You’ve “studied” dozens of LeetCode problems by reading solutions. During your interview at a top tech company, the interviewer presents a problem that seems familiar. You try to recall the solution you read, but under pressure, you realize you don’t truly understand the underlying principles. You struggle to adapt the memorized approach to this slightly different context.
Contrast this with someone who solved fewer problems but wrestled with each one independently. They’ve built robust mental models that allow them to approach novel problems with confidence, even under pressure.
Solution Reading vs. Solution Construction
The core issue is the fundamental difference between solution reading and solution construction:
Solution Reading | Solution Construction |
---|---|
Passive | Active |
Recognition memory | Recall memory |
Follows a predetermined path | Explores multiple paths |
Skips dead ends | Learns from dead ends |
Appears efficient | Appears inefficient but builds stronger foundations |
This distinction explains why so many programmers can read and understand complex code but struggle to produce solutions independently. They’ve optimized for the wrong skill.
The Hidden Cost of Solution Peeking
Beyond the illusion of competence, there’s another subtle cost to regularly checking solutions: it trains you to give up too easily.
Every time you hit a roadblock and immediately look at a solution, you’re reinforcing a pattern of behavior that says, “When things get difficult, seek the answer externally rather than persisting.”
Over time, this habit lowers your frustration tolerance and problem solving stamina—qualities that are essential for tackling complex programming challenges in the real world, where there might not be a solution to peek at.
Professional software engineers often spend days working through difficult problems. The ability to persist through confusion and uncertainty is as valuable as technical knowledge itself.
When Learning Plateaus: A Common Pattern
Many programmers experience a peculiar learning plateau that stems directly from solution-checking habits. Their progress follows a predictable pattern:
- Initial rapid progress through basic concepts
- Increasing challenge as problems become more complex
- Growing reliance on looking at solutions when stuck
- Apparent continued progress (completing more problems)
- A sudden plateau when faced with novel problems that require independent thinking
This plateau is particularly frustrating because it often comes after investing significant time in study. The programmer has “completed” many problems but hasn’t developed the independent problem solving ability they need.
The Memorization Trap
When you frequently read solutions before solving problems yourself, you may fall into the “memorization trap”—attempting to memorize solutions to specific problem types rather than understanding the underlying principles.
This approach might seem to work initially, especially for common problem patterns. You might think, “This is a sliding window problem, so I’ll apply the sliding window template I memorized.”
But algorithmic problem solving isn’t about memorizing templates. It’s about developing the ability to analyze problems, recognize patterns, and construct appropriate solutions from first principles.
Real-world programming and technical interviews rarely present problems that exactly match templates. They test your ability to adapt and combine concepts, which memorization doesn’t prepare you for.
Better Alternatives to Solution Peeking
If looking at solutions isn’t effective, what should you do when you’re stuck? Here are more productive approaches:
1. Structured Hints Instead of Complete Solutions
Rather than jumping to a complete solution, seek out or create a system of progressive hints. These guide your thinking without giving away the entire approach.
Good hints might include:
- What data structure would be most appropriate here?
- Can you break this problem into smaller subproblems?
- Is there a specific algorithm pattern that applies?
This approach keeps you in the driver’s seat of problem solving while providing just enough guidance to move forward.
2. Time-Boxing Your Struggle
Set a reasonable time limit for struggling with a problem before seeking help. This might be 30 minutes for simpler problems or several hours for complex ones.
During this time, fully commit to solving the problem independently. Document your approaches, even the failed ones. This practice builds problem solving stamina while ensuring you don’t get permanently stuck.
3. Rubber Duck Debugging
Sometimes, the best way past an obstacle is to explain your problem to someone else—or something else. The practice of explaining a problem out loud, even to an inanimate object like a rubber duck, often reveals insights you missed while keeping the solution process active rather than passive.
Try articulating:
- What you’re trying to accomplish
- The approaches you’ve tried
- Why you think they didn’t work
- What you’re considering trying next
This verbalization process often uncovers logical gaps or new perspectives.
4. Partial Solution Review
If you must look at a solution, try reviewing only enough to get unstuck on your specific obstacle, then return to solving the problem independently.
For example, if you’re struggling with how to efficiently check for duplicates in an array, you might look up just that specific technique rather than a complete solution to your broader problem.
5. Post-Solution Reflection
After completing a problem (whether with or without help), take time to reflect on the solution process. Ask yourself:
- What was the key insight needed to solve this problem?
- What patterns or techniques were used that I could apply elsewhere?
- Where specifically did I get stuck, and why?
- How could I approach a similar problem differently next time?
This reflection transforms even solution-assisted problems into learning opportunities.
The Spaced Repetition Approach
One of the most effective techniques for building lasting problem solving skills combines the principles above with spaced repetition. Here’s how it works:
- Attempt to solve a problem completely independently.
- If you succeed, schedule to revisit a similar problem in 1-2 weeks.
- If you get stuck, use the structured hint approach, only looking at a solution as a last resort.
- Regardless of whether you solved it independently, schedule to solve the exact same problem again in 2-3 days, without referring to any notes or previous solutions.
- If you struggle again, repeat the process. If you solve it easily, schedule a more challenging variation for a week later.
This approach leverages the psychological spacing effect, which shows that information learned and recalled across multiple sessions with gaps between them forms stronger, more durable neural pathways.
Real Examples: The Difference in Approaches
Let’s examine how these different learning approaches play out with a common interview problem: finding the longest substring without repeating characters.
Approach 1: Solution Peeking
A learner reads this solution:
function lengthOfLongestSubstring(s) {
let maxLength = 0;
let start = 0;
const charMap = new Map();
for (let end = 0; end < s.length; end++) {
const currentChar = s[end];
if (charMap.has(currentChar) && charMap.get(currentChar) >= start) {
start = charMap.get(currentChar) + 1;
}
charMap.set(currentChar, end);
maxLength = Math.max(maxLength, end - start + 1);
}
return maxLength;
}
They understand it conceptually: “We use a sliding window approach with a hashmap to track character positions. When we find a duplicate, we move the start pointer past the previous occurrence.”
But two weeks later, when asked to solve a similar problem about finding the longest substring with at most K distinct characters, they struggle to adapt the approach because they didn’t develop the underlying mental model.
Approach 2: Structured Learning
A different learner tackles the same problem:
- They spend 20 minutes trying different approaches, including a naive solution that checks all substrings (which is too slow).
- They get stuck and use a hint: “Could you solve this in a single pass through the string?”
- After more thought, they realize they need to track character positions and only need to consider substrings ending at the current position.
- They develop a sliding window approach with a hashmap, working through several edge cases.
- They revisit the problem a week later, reconstructing the solution from their understanding of the pattern.
When later faced with the K-distinct characters problem, they recognize it as a variation of the sliding window pattern they’ve internalized and can adapt their approach accordingly.
Common Objections and Responses
Let’s address some common objections to the “struggle first” approach:
“But I learn better by example!”
While examples are valuable for learning, there’s a crucial difference between using examples to understand concepts and skipping the problem solving process entirely. The most effective approach often involves:
- Learning conceptual fundamentals with examples
- Attempting problems independently
- Reviewing different solution approaches after you’ve developed your own
This sequence preserves the cognitive benefits of struggle while still leveraging the power of examples.
“I don’t have time to spend hours on each problem!”
It’s true that time is limited, but consider this: spending three hours deeply engaging with one problem often provides more lasting learning than skimming through solutions to five problems in the same time.
Quality trumps quantity in algorithmic learning. A smaller number of thoroughly understood problems creates more transferable skills than a larger number of superficially “completed” ones.
“Some problems are just too difficult for my current level!”
This is valid. Not every problem is appropriate for independent solving at every skill level. The key is finding problems within your “zone of proximal development”—challenging enough to promote growth but not so difficult that productive struggle turns into unproductive frustration.
If a problem is truly beyond your current capabilities, look for simpler related problems to build the necessary foundational skills.
When It IS Appropriate to Study Solutions
While we’ve emphasized the limitations of solution-reading as a primary learning method, there are legitimate situations where studying others’ solutions is valuable:
1. After Solving a Problem Independently
Once you’ve successfully solved a problem on your own, reviewing alternative solutions can expose you to more elegant or efficient approaches. This comparative analysis deepens your understanding without short-circuiting the problem solving process.
2. Learning Entirely New Concepts
When encountering algorithm patterns or data structures you’ve never seen before (like dynamic programming for a beginner), studying example solutions alongside explanations can provide necessary context before you attempt similar problems.
3. Breaking Through Persistent Roadblocks
If you’ve made multiple genuine attempts at a problem over several sessions and remain completely stuck, reviewing a partial solution or high-level approach may be necessary to continue making progress.
4. Reverse Engineering for Pattern Recognition
Occasionally studying solutions to identify common patterns across problem types can help you recognize these patterns in future problems. The key is to study the pattern abstractly rather than memorizing specific implementations.
Building a Sustainable Learning Practice
Based on the principles we’ve discussed, here’s a framework for building a sustainable practice that develops genuine problem solving abilities:
1. Establish Clear Time Boundaries
Decide in advance how long you’ll work on a problem before seeking hints or solutions. This prevents both premature solution-checking and unproductive frustration.
2. Document Your Problem Solving Process
Keep a journal of your approaches, including:
- Your initial understanding of the problem
- Approaches you considered and why
- Specific obstacles you encountered
- Insights that led to breakthroughs
This documentation helps reinforce learning and reveals patterns in your problem solving process over time.
3. Build a Personal Hint System
Create or collect graduated hints for problem types you’re studying. These might range from gentle nudges (“Consider using a hash table”) to more specific guidance (“Try tracking the last position of each character”).
4. Implement Deliberate Review
Schedule regular review sessions where you revisit problems you’ve previously solved. Attempt to solve them from scratch, then compare your new solution to your previous one.
5. Join a Learning Community
Connect with other learners who share your commitment to deep learning. Explaining your approaches to others and seeing how they tackle problems expands your thinking without short-circuiting the problem solving process.
The Path to Algorithmic Mastery
Becoming proficient at algorithmic problem solving isn’t about accumulating knowledge of solutions. It’s about developing the mental models and problem solving frameworks that allow you to approach novel challenges with confidence.
The most successful engineers we’ve worked with at AlgoCademy share a common trait: they embrace the productive struggle of problem solving rather than seeking shortcuts. They understand that the moments of confusion and breakthrough are precisely where the most valuable learning happens.
As you continue your learning journey, remember that your goal isn’t just to solve problems—it’s to become a problem solver. And that transformation happens through the challenges you overcome, not the solutions you consume.
Practical Next Steps
If you’re convinced of the value of productive struggle but aren’t sure how to implement it in your learning routine, here are concrete next steps:
- Choose a problem slightly above your current level. Set a timer for 30 minutes and commit to working on it without looking at hints or solutions during that time.
- Write down every approach you try, even the ones that don’t work. Document why you think they failed.
- If you solve it, write an explanation of your solution as if teaching it to someone else. Then look at alternative solutions to compare approaches.
- If you don’t solve it after your time limit, write down specific questions or points of confusion. Seek out targeted hints rather than complete solutions.
- Schedule a time to revisit the exact same problem in 2-3 days, attempting it from scratch.
This deliberate practice approach might feel slower initially, but the depth of understanding and problem solving ability you’ll develop will serve you far better in the long run than collecting superficial exposure to many solutions.
Conclusion
The path to programming mastery is not through consuming other people’s solutions, but through developing your own problem solving abilities through persistent, thoughtful practice.
While it might be tempting to take shortcuts when learning algorithms and data structures, the research is clear: productive struggle is not wasted time—it’s the essence of effective learning. By embracing this process, you build not just knowledge of specific solutions, but the far more valuable ability to solve novel problems independently.
Remember that your goal in studying algorithms isn’t just to pass interviews or complete assignments—it’s to develop thinking patterns that will serve you throughout your programming career. And those patterns emerge most strongly through the challenges you overcome yourself, not the solutions you borrow from others.
The next time you feel stuck on a problem and your finger hovers over that “View Solution” button, ask yourself: Am I looking for understanding, or am I looking for an answer? The difference between these two intentions might just determine the trajectory of your growth as a programmer.