Why You Can’t Identify What Makes a Good Solution
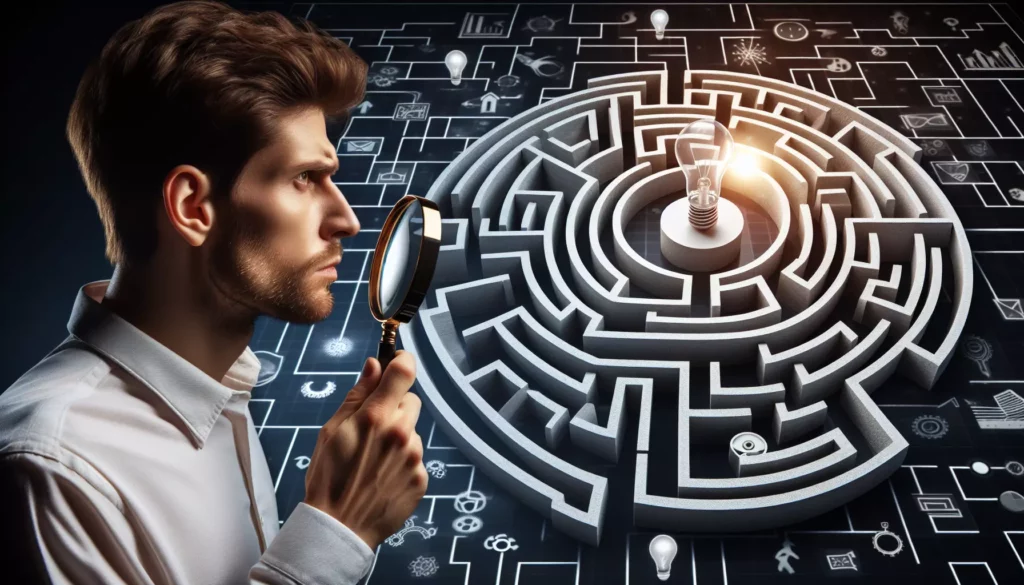
In the world of programming and algorithmic problem-solving, understanding what constitutes a “good” solution is often more challenging than finding the solution itself. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at major tech companies, the ability to evaluate and improve your solutions is a crucial skill that many struggle to develop.
At AlgoCademy, we’ve observed thousands of learners tackle coding challenges, and we’ve noticed a consistent pattern: many programmers can eventually solve a problem, but far fewer can articulate why their solution is good—or identify how it could be better. This disconnect isn’t just an academic concern; it directly impacts your growth as a developer and your performance in technical interviews.
The Evaluation Gap in Programming Education
Most coding education focuses heavily on getting to a working solution. You’re taught syntax, algorithms, and data structures with the primary goal of solving problems correctly. While correctness is certainly essential, it’s just one dimension of solution quality. This narrow focus creates what we call the “evaluation gap”—the inability to comprehensively assess your own code beyond basic functionality.
Consider a common scenario: You solve a coding problem and your solution passes all test cases. Success, right? Not necessarily. What if your solution:
- Runs exponentially slower than an optimal approach?
- Uses significantly more memory than necessary?
- Contains redundant logic that makes maintenance difficult?
- Lacks readability, making it challenging for others (or your future self) to understand?
- Fails to handle edge cases not covered in the test suite?
Without the skills to evaluate these aspects, you might believe you’ve mastered a concept when you’ve only scratched the surface of what makes a truly good solution.
The Multidimensional Nature of Code Quality
What makes identifying good solutions so challenging is that code quality isn’t a single-dimensional measure—it’s multifaceted and often involves tradeoffs. Let’s explore the key dimensions:
1. Correctness
At the most basic level, a solution must be correct—it should produce the expected output for all valid inputs. However, correctness extends beyond just the happy path:
- Does your solution handle edge cases correctly?
- Does it validate inputs appropriately?
- Does it handle error conditions gracefully?
Many programmers focus exclusively on correctness while neglecting other crucial aspects of solution quality.
2. Time Complexity
Time complexity measures how the execution time of your algorithm grows as the input size increases. An efficient algorithm might run in O(n log n) time, while a less efficient one might run in O(n²). The difference becomes dramatic with large inputs:
- For n = 1,000: O(n log n) performs ~10,000 operations, while O(n²) performs ~1,000,000 operations
- For n = 1,000,000: O(n log n) performs ~20,000,000 operations, while O(n²) performs a trillion operations
Many programmers struggle to analyze time complexity accurately or optimize their solutions for better performance.
3. Space Complexity
Space complexity measures the amount of memory your solution requires. While modern computers have abundant memory, inefficient space usage can still lead to problems, especially in resource-constrained environments or with very large datasets.
Consider two solutions to the same problem:
- Solution A: O(n) time complexity, O(n) space complexity
- Solution B: O(n log n) time complexity, O(1) space complexity
Which is better? It depends on your constraints and priorities—a tradeoff that many programmers struggle to evaluate.
4. Readability and Maintainability
Code is read far more often than it’s written. A solution that’s difficult to understand will create problems for future maintainers (including yourself). Good solutions balance efficiency with readability through:
- Clear variable and function names
- Appropriate comments for complex logic
- Consistent formatting and style
- Modular design with single-responsibility functions
Many programmers undervalue readability, especially when focused on optimization or when under time pressure (like in coding interviews).
5. Extensibility and Flexibility
How easily can your solution adapt to changing requirements? Good solutions anticipate potential changes and are structured to accommodate them without complete rewrites.
For example, if you’re writing a sorting function, can it easily be modified to sort in descending order or to sort by different criteria? These considerations reflect the flexibility of your solution.
Common Barriers to Solution Evaluation
Now that we understand the multidimensional nature of solution quality, let’s explore why many programmers struggle to evaluate their own code effectively:
1. The Curse of Knowledge
Once you’ve solved a problem, you understand both the problem and your solution intimately. This makes it difficult to step back and evaluate your code objectively. You might miss unclear variable names or convoluted logic because you already know what the code is supposed to do.
This psychological phenomenon, known as the “curse of knowledge,” blinds you to potential improvements in your own code.
2. Lack of Comparative Experience
To recognize what makes a solution “good,” you need exposure to a range of solutions—from poor to excellent. Without this comparative experience, you lack benchmarks for evaluation.
Many self-taught programmers or those with limited peer review experience haven’t seen enough code variety to develop strong evaluation skills.
3. Overemphasis on Getting to “Working”
The relief of finally getting your code to work creates a powerful psychological incentive to stop there. The effort required to refine a working solution often feels disproportionate to the benefit, especially when you’re eager to move on to the next challenge.
This “good enough” mindset inhibits the development of solution evaluation skills.
4. Insufficient Understanding of Algorithms and Data Structures
Without a solid grasp of fundamental algorithms and data structures, it’s difficult to recognize when your solution could leverage more efficient approaches. For example, if you’re unfamiliar with hash tables, you might not realize that your O(n²) lookup operation could be reduced to O(1).
This knowledge gap limits your ability to identify optimization opportunities.
5. Limited Feedback Loops
Learning to evaluate solutions effectively requires feedback—ideally from more experienced programmers who can point out aspects you’ve missed. Without these feedback loops, misconceptions persist and evaluation skills stagnate.
Many programmers, especially those learning independently, lack access to quality feedback on their code.
The Cost of Poor Evaluation Skills
Failing to develop solution evaluation skills carries significant costs throughout your programming career:
In Learning and Skill Development
When you can’t evaluate your solutions effectively, your learning becomes inefficient. You might:
- Repeatedly implement suboptimal patterns without realizing it
- Miss opportunities to learn more elegant or efficient approaches
- Develop false confidence in skills that aren’t as strong as you believe
- Plateau in your growth due to limited self-critique
In Technical Interviews
Technical interviews at companies like Google, Amazon, or Meta (formerly Facebook) explicitly test your ability to evaluate and improve solutions. Interviewers expect you to:
- Analyze the time and space complexity of your approach
- Identify tradeoffs between different solutions
- Recognize and articulate the strengths and weaknesses of your code
- Iteratively improve your initial solution
Without strong evaluation skills, you might implement a working solution but fail to demonstrate the critical thinking that interviewers seek.
In Professional Development
In professional settings, code quality directly impacts:
- System performance and scalability
- Maintenance costs and technical debt
- Team collaboration and knowledge sharing
- Your reputation and advancement opportunities
Developers who consistently deliver well-designed, efficient, and maintainable code are valued far more highly than those who simply get things working.
Developing Solution Evaluation Skills
Now that we understand the challenges, how can you develop stronger solution evaluation skills? Here are practical strategies:
1. Study Multiple Solutions to the Same Problem
One of the most effective ways to develop evaluation skills is to compare different approaches to the same problem. This builds your mental library of patterns and tradeoffs.
For example, consider these three approaches to finding duplicates in an array:
// Approach 1: Nested loops (O(n²) time, O(1) space)
function findDuplicates1(arr) {
let duplicates = [];
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] === arr[j] && !duplicates.includes(arr[i])) {
duplicates.push(arr[i]);
}
}
}
return duplicates;
}
// Approach 2: Sorting first (O(n log n) time, O(1) space if sorted in-place)
function findDuplicates2(arr) {
let duplicates = [];
let sortedArr = [...arr].sort((a, b) => a - b);
for (let i = 0; i < sortedArr.length - 1; i++) {
if (sortedArr[i] === sortedArr[i + 1] &&
(i === 0 || sortedArr[i] !== sortedArr[i - 1])) {
duplicates.push(sortedArr[i]);
}
}
return duplicates;
}
// Approach 3: Using a hash set (O(n) time, O(n) space)
function findDuplicates3(arr) {
let seen = new Set();
let duplicates = new Set();
for (let num of arr) {
if (seen.has(num)) {
duplicates.add(num);
} else {
seen.add(num);
}
}
return [...duplicates];
}
By comparing these solutions, you can see the explicit tradeoffs between time complexity, space complexity, and code simplicity.
2. Practice Analyzing Time and Space Complexity
Complexity analysis is a foundational skill for solution evaluation. Practice analyzing the time and space complexity of both your solutions and others’.
Start with simple examples and work your way up to more complex algorithms. Ask yourself:
- How does the execution time grow as the input size increases?
- What’s the worst-case scenario for this algorithm?
- How much extra memory does this solution require?
Tools like algorithm visualizers can help build intuition about how different algorithms perform.
3. Implement the Same Solution in Multiple Ways
Challenge yourself to solve problems using different approaches:
- First, focus on getting a working solution
- Then, optimize for time complexity
- Next, optimize for space complexity
- Finally, optimize for readability and maintainability
This exercise helps you understand the tradeoffs involved in different optimization priorities.
4. Seek and Embrace Code Reviews
Code reviews are invaluable for developing evaluation skills. They provide external perspectives that highlight blind spots in your own evaluation.
Actively seek reviews from more experienced developers, and approach feedback with curiosity rather than defensiveness. Ask specific questions like:
- “How would you improve the efficiency of this function?”
- “Is there a clearer way to express this logic?”
- “What edge cases am I missing?”
Online communities like Stack Overflow’s Code Review, GitHub, or programming Discord servers can provide review opportunities if you don’t have access to experienced colleagues.
5. Review and Refactor Old Code
Revisiting your old code after time has passed provides a fresh perspective. You’ll often be surprised by what you notice when the code isn’t fresh in your mind.
Set a calendar reminder to review solutions you wrote months ago, and challenge yourself to improve them. This practice helps overcome the curse of knowledge and builds self-evaluation skills.
6. Use Objective Metrics and Tools
Leverage tools that provide objective measurements of code quality:
- Profilers to measure actual performance
- Linters to identify potential issues
- Static analysis tools to detect code smells
- Complexity analyzers to quantify cognitive complexity
These tools can highlight issues you might miss in manual review and provide concrete metrics for improvement.
Case Study: Evolving a Solution
Let’s examine how a solution might evolve through increasingly sophisticated evaluation. We’ll use the classic problem of finding the longest substring without repeating characters.
Initial Solution
A beginner might write:
function lengthOfLongestSubstring(s) {
let maxLength = 0;
for (let i = 0; i < s.length; i++) {
let currentChars = new Set();
for (let j = i; j < s.length; j++) {
if (currentChars.has(s[j])) {
break;
}
currentChars.add(s[j]);
maxLength = Math.max(maxLength, currentChars.size);
}
}
return maxLength;
}
This solution works correctly, but it has O(n²) time complexity because it potentially examines each character multiple times.
Intermediate Evaluation
With some algorithmic knowledge, you might recognize that we can optimize using a sliding window approach:
function lengthOfLongestSubstring(s) {
let maxLength = 0;
let start = 0;
let charMap = {};
for (let end = 0; end < s.length; end++) {
const currentChar = s[end];
if (charMap[currentChar] !== undefined && charMap[currentChar] >= start) {
start = charMap[currentChar] + 1;
}
charMap[currentChar] = end;
maxLength = Math.max(maxLength, end - start + 1);
}
return maxLength;
}
This solution improves time complexity to O(n) by using a sliding window and a hash map to track character positions.
Advanced Evaluation
An experienced developer might further refine the solution for readability and maintainability:
function lengthOfLongestSubstring(s) {
if (!s) return 0;
let maxLength = 0;
let windowStart = 0;
const lastSeen = new Map();
for (let windowEnd = 0; windowEnd < s.length; windowEnd++) {
const currentChar = s[windowEnd];
// If we've seen this character before and it's within our current window
if (lastSeen.has(currentChar) && lastSeen.get(currentChar) >= windowStart) {
// Move window start to just after the previous occurrence
windowStart = lastSeen.get(currentChar) + 1;
}
// Update last seen position of current character
lastSeen.set(currentChar, windowEnd);
// Update max length if current window is larger
const currentWindowLength = windowEnd - windowStart + 1;
maxLength = Math.max(maxLength, currentWindowLength);
}
return maxLength;
}
This solution maintains the O(n) time complexity but adds:
- Descriptive variable names that clarify the sliding window concept
- Comments explaining the logic
- Input validation
- A more semantically appropriate Map instead of an object
- Extracted the window length calculation for clarity
The evolution of this solution demonstrates how increasingly sophisticated evaluation leads to improvements in efficiency, readability, and robustness.
The Role of Algorithmic Patterns
Recognizing common algorithmic patterns is crucial for solution evaluation. When you can identify that a problem fits a known pattern, you can compare your solution to established best practices for that pattern.
Key patterns to study include:
1. Sliding Window
Useful for problems involving subarrays or substrings where you need to find a contiguous sequence with certain properties. Examples include:
- Finding the longest substring without repeating characters
- Finding the minimum subarray with a sum greater than a value
- Finding the maximum sum subarray of a fixed size
2. Two Pointers
Effective for problems where you need to find pairs or process arrays from both ends. Examples include:
- Finding pairs that sum to a target value
- Removing duplicates from a sorted array
- Reversing arrays or strings
3. Fast and Slow Pointers
Useful for cycle detection or finding midpoints. Examples include:
- Detecting cycles in linked lists
- Finding the middle element of a linked list
- Determining if a number is a palindrome
4. Merge Intervals
Helpful for problems involving intervals or ranges. Examples include:
- Merging overlapping intervals
- Finding meeting room requirements
- Finding free time slots in calendars
5. Dynamic Programming
Essential for optimization problems with overlapping subproblems. Examples include:
- Finding the longest common subsequence
- Calculating the minimum edit distance between strings
- Solving knapsack problems
By studying these patterns, you develop a mental framework for evaluating whether your solution aligns with established approaches for similar problems.
Beyond Technical Evaluation: The Human Factor
While we’ve focused primarily on technical aspects of solution evaluation, it’s important to recognize that code exists in a human context. A truly comprehensive evaluation considers:
Team Understanding
Will your teammates be able to understand and maintain this code? Even a technically optimal solution can be problematic if it’s too complex for your team’s context.
Business Requirements
Does the solution meet the actual business needs? Sometimes a simpler, less optimal solution is preferable if it satisfies requirements and can be delivered more quickly.
Future Adaptability
How will this solution evolve as requirements change? Code that’s difficult to modify can become a liability, even if it’s currently efficient.
Learning Value
For educational contexts, sometimes a more verbose or explicit solution has greater learning value than a highly optimized one.
The Journey to Evaluation Mastery
Developing strong solution evaluation skills is a journey that parallels your growth as a programmer. As you progress, your evaluation will evolve through several stages:
Beginner: Correctness Focus
At this stage, you’re primarily concerned with whether your code works for the test cases. Your evaluation is binary: it either works or it doesn’t.
Intermediate: Efficiency Awareness
You begin to recognize inefficient patterns and can analyze basic time and space complexity. You start to consider tradeoffs between different approaches.
Advanced: Comprehensive Evaluation
You can evaluate solutions across multiple dimensions simultaneously, weighing tradeoffs and selecting approaches based on context. You can articulate why certain solutions are preferable in specific situations.
Expert: Intuitive Optimization
You develop an intuition for optimal solutions and can quickly identify improvements. Your evaluation becomes more nuanced, considering subtle factors like cache performance, readability implications, and maintenance costs.
Remember that this journey takes time and deliberate practice. Even experienced developers continue to refine their evaluation skills throughout their careers.
Conclusion: Bridging the Evaluation Gap
The ability to identify what makes a good solution is a distinguishing characteristic of exceptional programmers. It’s what separates those who merely solve problems from those who solve them elegantly, efficiently, and maintainably.
At AlgoCademy, we believe that bridging the evaluation gap is essential for becoming a truly proficient programmer. By developing your solution evaluation skills, you’ll:
- Learn more effectively from each problem you solve
- Perform better in technical interviews
- Contribute more valuable code in professional settings
- Develop deeper insight into algorithmic patterns and tradeoffs
The next time you solve a coding problem, challenge yourself to go beyond “it works.” Ask yourself:
- How efficient is this solution?
- How readable and maintainable is it?
- What tradeoffs am I making?
- How could I improve it further?
These questions—and your growing ability to answer them thoughtfully—will accelerate your journey toward programming mastery.
Remember, the goal isn’t perfectionism; it’s developing the awareness and skills to make informed decisions about your code. With practice and persistence, you’ll find yourself not just writing solutions, but crafting them with intention and insight.