Why You Can’t Handle Unexpected Interview Questions (And How to Fix That)
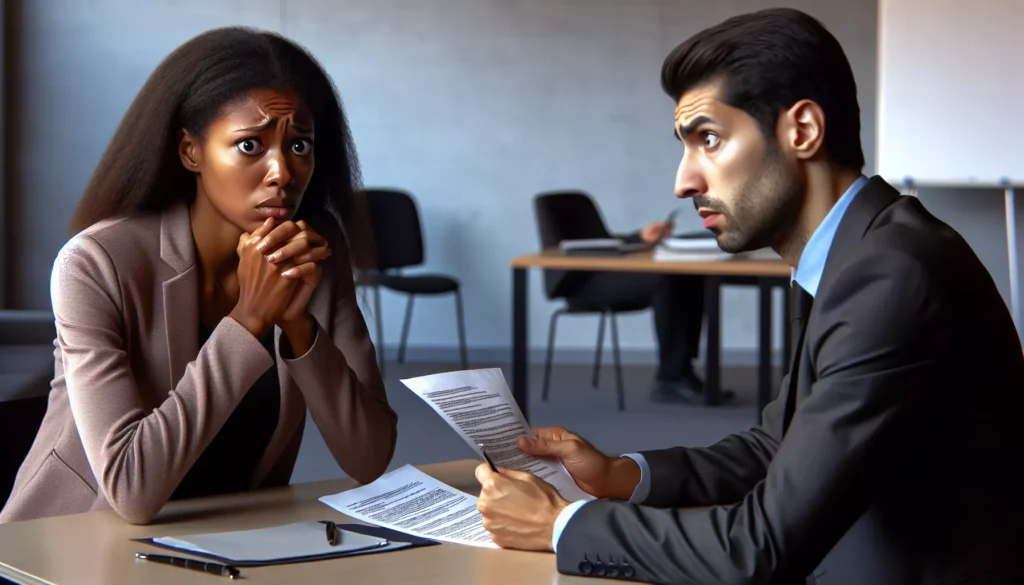
Technical interviews are notorious for throwing candidates curveballs. One moment you’re confidently explaining your approach to a familiar algorithm, and the next you’re staring blankly at a question you’ve never encountered before. Your mind races, palms sweat, and suddenly all your preparation seems inadequate.
If this scenario sounds familiar, you’re not alone. Many candidates struggle with unexpected interview questions, regardless of their technical expertise. This failure to adapt in the moment can cost you opportunities at top tech companies like Google, Amazon, or Meta, where interviewers deliberately design questions to test your problem-solving abilities under pressure.
In this comprehensive guide, we’ll explore why even well-prepared candidates freeze when faced with unfamiliar questions and provide actionable strategies to transform this weakness into a strength. By the end, you’ll have the tools to approach any technical interview with confidence, even when the questions catch you off guard.
The Psychology Behind Interview Freezes
Before we dive into solutions, it’s essential to understand what’s happening in your brain when you encounter an unexpected question. This isn’t just about technical knowledge; it’s about how your mind processes challenges under pressure.
The Panic Response
When faced with an unfamiliar problem during a high-stakes interview, your body often triggers a fight-or-flight response. This biological reaction evolved to help humans survive physical threats, but in a modern interview setting, it manifests as:
- Increased heart rate and rapid breathing
- Difficulty concentrating or thinking clearly
- Tunnel vision that prevents creative thinking
- Working memory impairment
Neuroscientist Dr. Amy Arnsten of Yale University has studied this phenomenon extensively. Her research shows that stress chemicals like cortisol and adrenaline can actually impair the function of your prefrontal cortex—the exact part of your brain responsible for problem-solving and logical thinking.
The Impostor Syndrome Spiral
Beyond the physiological response, many candidates experience an immediate psychological reaction:
“I should know this. Everyone else would know how to solve this. I don’t belong in this field.”
This impostor syndrome spiral compounds the problem. Now you’re not just trying to solve a difficult question; you’re simultaneously battling negative self-talk and anxiety about your capabilities. This cognitive load further diminishes your ability to think clearly and access the knowledge you do have.
The Fixed Mindset Trap
Carol Dweck’s research on mindset provides another piece of the puzzle. Candidates with a fixed mindset tend to view their technical abilities as static traits. When they encounter a question they can’t immediately answer, they interpret it as evidence of their limitations rather than an opportunity to grow.
This mindset leads to avoidance behaviors during interviews: giving up quickly, providing vague answers, or trying to redirect the conversation to more comfortable territory—all of which signal red flags to interviewers.
Common Types of Unexpected Interview Questions
Unexpected questions in technical interviews typically fall into several categories. Recognizing these patterns can help you prepare more effectively.
1. Familiar Problems with Unfamiliar Constraints
Often, interviewers take standard problems and add unexpected constraints to test your adaptability. For example, you might be asked to solve a sorting problem but with severe memory limitations, or implement a common algorithm without using recursion.
Example: “Implement a binary search algorithm without using recursion or any built-in methods.”
2. Domain Knowledge Questions Outside Your Comfort Zone
These questions test your breadth of knowledge across different areas of computer science. A frontend developer might be asked about database optimization, or a mobile developer about network protocols.
Example: “Explain how you would diagnose and fix a slow database query in a production environment.”
3. Real-World Scenario Questions
These questions present messy, ambiguous problems that don’t have textbook solutions. They test your ability to make reasonable assumptions and create practical solutions.
Example: “Design a system to detect fraudulent transactions for an e-commerce platform that processes millions of orders daily.”
4. Conceptual Questions with Practical Applications
These questions test your understanding of theoretical concepts by asking you to apply them in unconventional ways.
Example: “How would you use a hash table to implement a least recently used (LRU) cache?”
5. Questions Designed to Probe Your Limits
Some interviewers deliberately ask increasingly difficult questions until you reach your knowledge boundary. The goal isn’t necessarily for you to solve everything but to see how you handle reaching your limits.
Example: Starting with basic string manipulation, then moving to regular expressions, then to implementing a simple parser, and finally to optimizing a lexer for performance.
Why Traditional Interview Prep Falls Short
Many candidates spend weeks or months preparing for technical interviews, only to find themselves struggling with unexpected questions. Here’s why traditional preparation methods often fall short:
The Memorization Trap
Memorizing solutions to common problems can create a false sense of security. When faced with a variation of these problems, candidates often try to force their memorized solution rather than adapting their approach. This rigid thinking is exactly what interviewers are trying to identify and avoid in potential hires.
As one Google interviewer noted: “I’m not interested in whether a candidate has memorized the optimal solution to a standard problem. I want to see how they think through problems they haven’t encountered before.”
Breadth vs. Depth Imbalance
Many preparation resources focus on depth in a few key areas (like dynamic programming or tree traversal) while neglecting breadth across the full spectrum of computer science concepts. This creates knowledge gaps that unexpected questions can easily expose.
Missing the Meta-Skills
Traditional interview prep often focuses exclusively on technical knowledge while neglecting the meta-skills that help you approach unfamiliar problems:
- How to break down ambiguous problems
- How to communicate your thought process while uncertain
- How to leverage first principles when standard approaches don’t apply
- How to manage stress during high-pressure situations
These meta-skills are often more important than specific technical knowledge when dealing with unexpected questions.
Building a Framework for Handling Any Question
The good news is that you can develop a systematic approach to unexpected interview questions. This framework will help you navigate unfamiliar territory with confidence.
Step 1: Embrace the Clarification Phase
When faced with an unexpected question, your first response should always be clarification—not an immediate attempt at a solution.
Effective clarification serves multiple purposes:
- It gives you time to collect your thoughts
- It ensures you’re solving the right problem
- It demonstrates thoughtfulness to the interviewer
- It might reveal hints about the expected approach
Example clarification questions:
- “Can you tell me more about the constraints on memory usage for this solution?”
- “Should I optimize for read operations or write operations in this scenario?”
- “What scale are we talking about—thousands of users or millions?”
- “Are there any assumptions I should make about the input format?”
A senior engineer at Netflix shared: “I’m always impressed when candidates take the time to fully understand the problem before diving into code. It shows maturity and prevents them from going down rabbit holes.”
Step 2: Break Down the Problem
Once you understand the question, break it down into smaller, more manageable components. This decomposition makes even the most complex problems approachable.
Techniques for effective problem decomposition:
- Identify the core data structures involved: What kind of data are you working with, and how should it be organized?
- Define the key operations: What are the fundamental actions your solution needs to perform?
- Consider edge cases early: What are the boundary conditions or special cases?
- Draw connections to familiar problems: Does this resemble any known problems, even partially?
Verbalize this breakdown to the interviewer. Even if your approach isn’t perfect, demonstrating structured thinking is valuable.
Step 3: Start with a Brute Force Approach
When facing an unfamiliar problem, begin with the simplest solution that will work, regardless of efficiency. This accomplishes several things:
- Establishes a baseline solution that you can refine
- Demonstrates that you can at least solve the problem
- Often reveals insights that lead to more efficient approaches
- Gives you confidence to continue the problem-solving process
A Microsoft interviewer explains: “I’d rather see a candidate implement a naive solution and then improve it than get stuck trying to find the perfect algorithm from the start.”
Step 4: Analyze and Optimize
Once you have a working solution, analyze its performance characteristics:
- What is the time complexity?
- What is the space complexity?
- Where are the bottlenecks?
- What assumptions could be changed to improve performance?
Then, explore optimizations based on your analysis. Even if you don’t immediately see the optimal solution, demonstrating this analytical process shows strong problem-solving skills.
Step 5: Test Your Solution
Always test your solution with examples, including edge cases. This demonstrates thoroughness and attention to detail. Walk through your code step by step, explaining what happens at each point.
Common edge cases to consider:
- Empty inputs
- Single-element inputs
- Very large inputs
- Inputs with duplicates
- Inputs with negative values (for numerical problems)
- Inputs that cause boundary conditions
Practical Strategies for Building Adaptability
The framework above provides a structure for approaching unexpected questions, but you also need to develop the underlying skills that make this approach effective. Here are practical strategies to build your adaptability.
Expand Your Problem-Solving Patterns
Rather than memorizing specific solutions, focus on recognizing and applying problem-solving patterns. When you understand these patterns, you can adapt them to novel situations.
Key problem-solving patterns to master:
- Sliding Window: For problems involving subarrays or substrings
- Two Pointers: For searching, comparing, or merging operations
- Breadth-First and Depth-First Search: For graph and tree traversal
- Binary Search: For efficient searching in sorted collections
- Dynamic Programming: For optimization problems with overlapping subproblems
- Backtracking: For constraint satisfaction and permutation problems
- Greedy Algorithms: For local optimization problems
- Divide and Conquer: For breaking problems into smaller, similar subproblems
Understanding these patterns allows you to approach unfamiliar problems by recognizing similarities to known patterns.
Practice Active Recall, Not Passive Review
Instead of reviewing solutions repeatedly, practice active recall by challenging yourself to solve problems from scratch. This builds the neural pathways needed for real-time problem-solving during interviews.
Implement a spaced repetition system for problems you’ve already solved:
- Solve a problem
- Wait a few days
- Try to solve it again without looking at your previous solution
- Compare your approaches
- Extend the interval before the next attempt
This practice strengthens your ability to reconstruct solutions rather than merely recognize them.
Diversify Your Practice
Many candidates focus exclusively on algorithm problems while neglecting other important areas. Expand your practice to include:
- System design questions: Practice designing scalable architectures for various applications
- Object-oriented design: Design classes and interfaces for real-world scenarios
- Database queries and optimization: Understand indexing, query planning, and normalization
- Operating system concepts: Processes, threads, synchronization, and memory management
- Networking fundamentals: TCP/IP, HTTP, REST, and other protocols
- Language-specific questions: Understand the nuances of your primary programming language
This breadth ensures you’re prepared for questions across the spectrum of software development.
Simulate Interview Conditions
Practice under conditions that mimic real interviews:
- Use a timer to create time pressure
- Code on a whiteboard or in a simple text editor without auto-completion
- Explain your thought process out loud as you solve problems
- Have a friend ask you unexpected follow-up questions
- Practice with distractions present to build focus
These simulations help you build resilience to the stress of actual interviews.
Develop a Growth Mindset
Cultivate a perspective that views challenges as opportunities for growth rather than threats to your self-image. When you encounter a question you can’t solve:
- Acknowledge the gap in your knowledge without judgment
- Be honest about what you don’t know
- Show enthusiasm for learning something new
- Focus on the process rather than the outcome
This mindset transforms interviews from evaluations into learning experiences.
Real-World Examples: Turning Unexpected Questions into Opportunities
Let’s examine how the framework and strategies above can be applied to real unexpected interview questions.
Example 1: The Unexpected Constraint
Question: “Implement a function to find the kth largest element in an array, but you cannot use any sorting algorithm or built-in sort functions.”
Initial reaction: Most candidates would immediately think of sorting the array and returning the kth element from the end, but the constraint prohibits this approach.
Applying the framework:
- Clarification: “Just to confirm, I need to find the kth largest element without sorting. Can I make any assumptions about the input array? Are there duplicates? Any constraints on memory usage?”
- Break down the problem: “I need to find the kth largest element, which means I need some way to track or identify the relative ordering of elements without fully sorting them.”
- Brute force approach: “A simple approach would be to find the maximum element, remove it, and repeat k times. This would be O(n*k) time complexity.”
- Analyze and optimize: “I realize we can use a min-heap of size k to keep track of the k largest elements we’ve seen so far. This would be more efficient with O(n log k) time complexity.”
- Test the solution: Walk through the heap-based approach with a small example array and different values of k.
Sample code:
function findKthLargest(nums, k) {
// Use a min heap to keep track of the k largest elements
const minHeap = [];
for (let num of nums) {
// If the heap is smaller than k, just add the element
if (minHeap.length < k) {
minHeap.push(num);
bubbleUp(minHeap, minHeap.length - 1);
}
// If the current element is larger than the smallest in our heap
else if (num > minHeap[0]) {
minHeap[0] = num;
bubbleDown(minHeap, 0);
}
}
// The root of the min heap will be the kth largest element
return minHeap[0];
}
// Helper functions for heap operations would be implemented here
Alternative approach: “Another approach would be to use the QuickSelect algorithm, which is based on the partitioning step of QuickSort but only recursively processes one partition. This gives us an average case time complexity of O(n).”
Example 2: The Domain Knowledge Question
Question: “Explain how you would diagnose and fix a memory leak in a Node.js application in production.”
Initial reaction: This question might catch you off guard if you’re primarily focused on algorithms and data structures or if your experience with Node.js is limited.
Applying the framework:
- Clarification: “To make sure I understand correctly, we’re dealing with a Node.js application that’s showing signs of memory issues in production. Can you tell me more about the symptoms you’re observing? Is it gradually using more memory over time, or are there sudden spikes?”
- Break down the problem: “Diagnosing a memory leak involves several steps: confirming the leak exists, identifying the source, and implementing a fix. I’ll walk through each of these steps.”
- Start with what you know: “First, I’d confirm the memory leak by monitoring memory usage over time. Node.js applications typically show a sawtooth pattern due to garbage collection, but a leak would show an upward trend in the baseline.”
- Use first principles: “Memory leaks in JavaScript typically occur when references to objects are unintentionally retained, preventing garbage collection. Common causes include event listeners that aren’t removed, closures capturing large objects, or circular references that the garbage collector can’t handle.”
- Outline a systematic approach: “I would use tools like heapdump to capture memory snapshots at different points, then compare them to identify objects that are growing in number. I’d also look at the application code for common patterns that lead to memory leaks.”
Even with limited Node.js experience, this approach demonstrates analytical thinking and problem-solving skills, which are often more valuable to interviewers than specific knowledge.
Example 3: The Ambiguous System Design Question
Question: “Design a URL shortening service like bit.ly.”
Initial reaction: The open-ended nature of this question can be overwhelming, especially if you haven’t specifically prepared for URL shortener design.
Applying the framework:
- Clarification: “I’d like to understand the requirements better. What scale are we designing for? How many URLs do we expect to shorten per day? What’s the expected read-to-write ratio? Any specific requirements around URL customization or analytics?”
- Break down the problem: “At a high level, we need several components: an API for URL shortening, a storage system for mapping short URLs to original URLs, a redirection mechanism, and potentially analytics tracking.”
- Start with a basic design: “Let me sketch a simple architecture with a web server that handles both the shortening API and redirection, connected to a database that stores the URL mappings.”
- Identify key technical challenges: “The main challenges include generating unique short codes efficiently, ensuring high availability for the redirection service, and scaling the database as the number of URLs grows.”
- Refine based on requirements: “Based on the scale we discussed, I’d recommend a distributed database with sharding based on the short code. For the shortening algorithm, we could use a counter-based approach with base62 encoding or a cryptographic hash function.”
By systematically working through the problem and explicitly addressing scale, performance, and reliability, you demonstrate systems thinking even if you hadn’t specifically prepared for this exact question.
Handling Your Mind When It Goes Blank
Despite the best preparation, there may still be moments when your mind goes blank during an interview. Here’s how to recover gracefully:
The STOP Technique
When you feel yourself freezing:
- Stop: Pause and take a deep breath
- Think: Recognize what’s happening (“I’m experiencing interview anxiety”)
- Observe: Notice your physical sensations without judgment
- Proceed: Return to the problem with a clear mind
This mindfulness technique helps interrupt the panic response and regain cognitive control.
Verbalize Your Process
Don’t suffer in silence. Tell the interviewer what you’re experiencing:
“I’m thinking through different approaches here. Let me take a moment to organize my thoughts.”
This transparency is far better than awkward silence and shows emotional intelligence.
Return to First Principles
When stuck, go back to basics:
- What is the problem asking for at its core?
- What are the simplest examples I can think of?
- What basic data structures might be relevant?
- Can I solve a simpler version of this problem first?
This approach often unblocks your thinking by removing unnecessary complexity.
Ask Thoughtful Questions
If you’re truly stuck, ask a question that demonstrates your problem-solving process:
“I’m considering using a hash map to optimize lookups, but I’m not sure if that addresses the constraint about space complexity. Could you clarify if that’s a valid approach?”
This shows you’re engaged with the problem even if you don’t have the complete solution.
Learning from Interview Failures
Even with all these strategies, you will likely encounter questions that stump you. The difference between candidates who ultimately succeed and those who don’t is how they respond to these setbacks.
The Post-Interview Review Process
After every interview, successful or not, conduct a thorough review:
- Document the questions you were asked while they’re fresh in your mind
- Identify knowledge gaps that were exposed during the interview
- Research optimal solutions to questions you struggled with
- Reflect on your emotional responses and how they affected your performance
- Create a focused study plan based on your findings
This process transforms interview failures into valuable learning opportunities.
Building a Personal Question Bank
Maintain a database of questions that have challenged you, along with your solutions and reflections. This personalized resource becomes increasingly valuable as you progress in your interview preparation.
For each question, record:
- The question itself
- Your initial approach
- Where you got stuck
- The optimal solution
- Key insights or patterns
- Similar problems to practice
This database helps you identify recurring patterns in your struggles and track your improvement over time.
Conclusion: From Freezing to Flowing
Handling unexpected interview questions isn’t about knowing everything—it’s about developing a resilient, adaptable approach to problem-solving under pressure. By understanding the psychology behind interview freezes, building a flexible framework for approaching unfamiliar problems, and practicing the right strategies, you can transform your response to these challenges.
Remember that interviewers at top tech companies are often more interested in how you think than what you know. They deliberately design questions to test the boundaries of your knowledge because they want to see how you handle uncertainty—a constant in real-world software development.
The next time you face an unexpected question, rather than panicking, see it as an opportunity to demonstrate your problem-solving process. With practice and the right mindset, you can turn these moments from weaknesses into strengths that set you apart from other candidates.
The journey from freezing to flowing in technical interviews isn’t easy, but it’s a transformation that pays dividends throughout your career—not just in interviews but in every challenging problem you’ll face as a software developer.
So embrace the unexpected. It’s not just the path to interview success; it’s the essence of what makes software development a rewarding and endlessly fascinating field.