Why You Can’t Find Bugs in Your Own Code But Can Spot Them in Others’
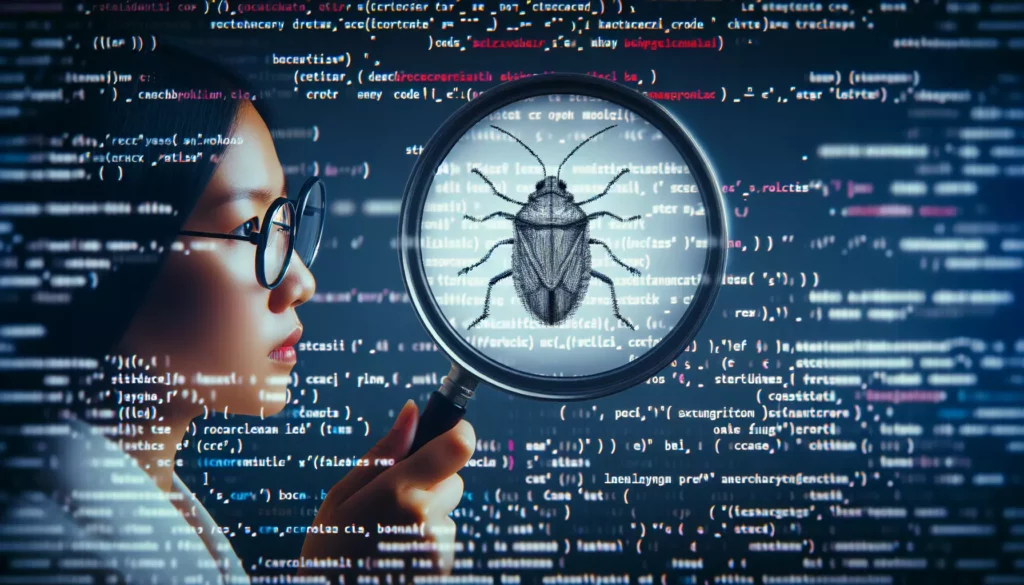
Have you ever spent hours debugging your code, only to have a colleague glance at it and immediately point out the error? Or perhaps you’ve been that colleague, spotting issues in others’ code that they’ve been blind to for days. This phenomenon is remarkably common in programming and has fascinating psychological underpinnings.
As developers, we’ve all experienced the frustration of being unable to find bugs in our own code while seemingly having eagle eyes when reviewing others’ work. This article explores why this happens and provides practical strategies to improve your debugging skills when working with your own code.
The Psychology Behind Code Blindness
Several psychological factors contribute to our inability to spot errors in our own code:
1. The Curse of Knowledge
When you write code, you develop an intimate understanding of how it’s supposed to work. This deep knowledge can actually hinder your ability to see mistakes because you read what you intended to write rather than what you actually wrote.
The curse of knowledge is a cognitive bias where better-informed individuals find it difficult to understand the perspective of less-informed people. In programming, you understand your own intentions so well that your brain automatically fills in gaps or corrects errors as you read your code.
For example, if you meant to write:
if (user.isAuthenticated()) {
allowAccess();
}
But actually wrote:
if (user.isAuthentcated()) {
allowAccess();
}
Your brain might automatically correct the typo in “isAuthentcated” because you know what you intended to write.
2. Inattentional Blindness
This psychological phenomenon occurs when you fail to notice an unexpected object in your field of vision because your attention is focused elsewhere. In programming, you might be so focused on the algorithm’s logic that you miss syntax errors or other issues.
A classic example is when you’re concentrating on implementing complex business logic and completely miss a missing semicolon or bracket that would be immediately obvious to a fresh pair of eyes.
3. Confirmation Bias
We naturally seek information that confirms our existing beliefs. When reviewing your own code, you’re looking for confirmation that it works as intended rather than searching for evidence that it doesn’t. This bias can cause you to overlook bugs that contradict your belief that your code is correct.
For instance, you might run a test case that shows your function working correctly in one scenario and subconsciously avoid testing edge cases that might reveal problems.
4. Mental Fatigue
After spending hours writing and debugging code, mental fatigue sets in. Your ability to spot errors diminishes significantly when you’re tired, leading to overlooked bugs that would be obvious to someone with fresh eyes.
Why We’re Better at Finding Others’ Bugs
The flip side of this phenomenon is equally interesting: why are we so much better at finding bugs in code written by others?
1. Objective Perspective
When examining someone else’s code, you have no preconceived notions about how it should work. You’re reading what’s actually there, not what was intended. This objectivity makes it easier to spot inconsistencies, logical errors, and syntax issues.
2. Different Mental Models
Each programmer has a unique way of thinking about problems. When you review someone else’s code, you bring a different mental model to the table. This alternative perspective often highlights issues that the original programmer couldn’t see because of their particular approach to the problem.
3. Critical Reading vs. Confirmation Reading
When reviewing others’ code, you naturally adopt a more critical mindset. Rather than seeking confirmation that the code works, you’re actively looking for potential issues and questioning assumptions. This critical approach is much more effective for bug detection.
4. Fresh Cognitive Resources
When looking at code for the first time, you bring fresh mental energy to the task. You haven’t depleted your cognitive resources on writing the code, so you have more mental bandwidth available for spotting errors.
Real World Examples of Code Blindness
Let’s examine some common scenarios where programmers often miss bugs in their own code:
The Off By One Error
Consider this classic array iteration bug:
// Intended to process all elements in an array
for (int i = 0; i <= array.length; i++) {
process(array[i]);
}
The bug here is subtle but critical: the loop condition should be i < array.length
rather than i <= array.length
. This will cause an ArrayIndexOutOfBoundsException on the last iteration. The original programmer might miss this because they’re thinking about the processing logic rather than the loop bounds.
The Null Pointer Dereference
Here’s another common oversight:
public void processUser(User user) {
String username = user.getUsername();
if (user != null) {
// Do something with username
}
}
The programmer has checked for null after already dereferencing the user object. Their mental model correctly included a null check, but the implementation order is wrong. Someone else reviewing this code would likely spot the issue immediately.
The Unintended Assignment
if (x = 5) {
// Do something when x equals 5
}
Here, the programmer intended to compare x to 5 using ==
but accidentally used the assignment operator =
instead. This assigns 5 to x and then evaluates to true, causing the conditional block to always execute. The original programmer might miss this because they know they intended a comparison.
Practical Strategies to Find Bugs in Your Own Code
While it’s natural to have blind spots when reviewing your own code, several techniques can help you overcome these limitations:
1. Take a Break
One of the simplest yet most effective strategies is to step away from your code for a while. Even a short break of 15-30 minutes can give your brain enough distance to approach the code with fresher eyes. For complex bugs, consider revisiting the code the next day if possible.
This technique works because it helps clear your working memory and reduces the curse of knowledge effect. When you return to the code, you’re more likely to read what’s actually there rather than what you remember writing.
2. Change Your Environment
Sometimes, physically changing your environment can help you see your code differently:
- Print out your code and review it on paper
- Change your IDE theme or font
- Work in a different location
- Read your code on a different device (tablet, different monitor)
These changes disrupt your brain’s pattern recognition and can help you notice details you previously missed.
3. Explain Your Code to Someone Else (or Something Else)
The “rubber duck debugging” technique involves explaining your code line by line to an inanimate object (traditionally a rubber duck). This forces you to articulate your logic explicitly, often revealing gaps in reasoning or implementation errors.
The act of verbalization engages different parts of your brain and requires you to think about your code from an outsider’s perspective. Even if you don’t have a rubber duck, explaining your code aloud to yourself can be surprisingly effective.
4. Read Your Code Backwards
Reading your code from bottom to top or right to left disrupts your normal reading patterns and helps you focus on the actual syntax rather than the logical flow. This technique is particularly useful for finding syntax errors, typos, and other surface-level bugs.
5. Use a Different Perspective
Try to review your code from different perspectives:
- As a compiler/interpreter: Focus solely on syntax and language rules
- As a security expert: Look for potential vulnerabilities or edge cases
- As a performance engineer: Examine efficiency and resource usage
- As a junior developer: Question every assumption and look for clarity issues
Each perspective will highlight different potential issues in your code.
6. Leverage Code Review Tools
Modern development environments offer numerous tools to help find bugs:
- Linters and static analyzers: Tools like ESLint, PyLint, or SonarQube can automatically detect many common errors and code smells
- IDE inspections: Most IDEs include built-in code analysis features that flag potential issues
- Automated tests: Unit tests, integration tests, and property-based testing can reveal bugs that manual review might miss
These tools provide an objective, rule-based perspective on your code that isn’t affected by the psychological biases we’ve discussed.
7. Use Debugging Techniques Systematically
Rather than randomly inserting print statements or setting breakpoints, adopt a methodical approach to debugging:
- Binary search debugging: Narrow down the problem area by testing the midpoint of your code and determining if the bug occurs before or after that point
- State inspection: Systematically examine the state of all relevant variables at key points in your program
- Trace execution: Step through your code line by line, comparing actual behavior to expected behavior
A systematic approach reduces the chance that your biases will lead you astray during debugging.
8. Pretend You’re Reviewing Someone Else’s Code
Consciously adopt the mindset you would have when reviewing a colleague’s code. Ask yourself:
- What assumptions is this programmer making?
- What edge cases might they have missed?
- How could this code fail?
- Is there a clearer or more efficient way to achieve this goal?
This deliberate perspective shift can help overcome the confirmation bias that normally affects your review of your own code.
Building a Personal Debugging Checklist
Based on your own common mistakes, create a personalized debugging checklist. Review your code against this list before considering it complete. Some items to consider including:
- Off-by-one errors in loops and array access
- Null/undefined checks before object access
- Resource cleanup (closing files, releasing locks)
- Error handling for all operations that could fail
- Edge cases (empty collections, maximum values, etc.)
- Correct operator usage (==, ===, =, etc.)
- Scope issues (variable shadowing, closure pitfalls)
- Race conditions in concurrent code
Over time, refine this list based on the bugs you discover in your code. This creates a personalized safety net for your specific blind spots.
Leveraging Pair Programming and Code Reviews
While this article focuses on finding bugs in your own code, it’s worth acknowledging that pair programming and code reviews are extremely effective debugging practices:
Pair Programming Benefits
When two developers work together at one workstation, they bring complementary perspectives to the code as it’s being written. The navigator (non-typing partner) can often spot issues that the driver (typing partner) misses due to the psychological factors we’ve discussed.
Studies have shown that while pair programming might take more person-hours up front, it typically results in higher-quality code with fewer bugs, potentially saving debugging time later.
Effective Code Reviews
Regular code reviews create a culture where code is viewed from multiple perspectives before being deployed. To get the most from code reviews:
- Review manageable chunks of code (200-400 lines maximum)
- Use a checklist to ensure thorough reviews
- Focus on finding issues, not just confirming correctness
- Encourage reviewers to ask questions when something isn’t clear
- View code reviews as learning opportunities, not criticism
Advanced Debugging Techniques
For particularly challenging bugs, consider these advanced approaches:
Instrumentation and Logging
Adding comprehensive logging can reveal the actual execution path and state changes in your program. Modern logging frameworks make it easy to enable detailed logs during debugging and disable them in production.
logger.debug("Processing user {} with role {}", user.getId(), user.getRole());
try {
result = userService.processUser(user);
logger.debug("Processing completed with result: {}", result);
} catch (Exception e) {
logger.error("Error processing user: {}", e.getMessage(), e);
throw e;
}
Assertions and Invariants
Adding assertions to your code can catch bugs early by verifying that your assumptions hold true during execution:
public void transferFunds(Account source, Account destination, double amount) {
assert amount > 0 : "Transfer amount must be positive";
assert source != null : "Source account cannot be null";
assert destination != null : "Destination account cannot be null";
assert source.getBalance() >= amount : "Insufficient funds";
// Proceed with transfer
double initialTotal = source.getBalance() + destination.getBalance();
source.withdraw(amount);
destination.deposit(amount);
// Verify invariant: total money in the system should remain constant
assert Math.abs((source.getBalance() + destination.getBalance()) - initialTotal) < 0.001 : "Money was created or destroyed";
}
Bisect Debugging
For bugs that appeared after many changes, use a bisect approach (similar to git bisect) to identify which change introduced the bug. This is particularly useful for regression bugs where previously working code now fails.
Comparative Debugging
When you have both working and non-working versions or instances of your code, systematically compare their behavior to isolate the difference that causes the bug.
The Role of Testing in Bug Prevention
While this article focuses on finding bugs after they've been introduced, it's worth emphasizing that comprehensive testing can prevent many bugs from surviving long enough to require debugging:
Test-Driven Development (TDD)
By writing tests before code, you clarify your expectations and create an immediate feedback loop that catches bugs as soon as they're introduced. TDD helps you think through edge cases and failure modes before you write the implementation.
Property-Based Testing
Rather than writing specific test cases, property-based testing frameworks (like QuickCheck or Hypothesis) generate thousands of random inputs to test properties your code should maintain. This approach often finds edge cases you wouldn't think to test manually.
Mutation Testing
Mutation testing tools introduce small changes (mutations) to your code and verify that your tests fail when this happens. If they don't, your tests may not be thorough enough to catch similar bugs in your actual code.
Learning from Your Bugs
Every bug you encounter is a learning opportunity. Keep a "bug journal" documenting:
- What the bug was
- How it manifested
- Why you didn't catch it initially
- How you eventually found it
- What you could do differently next time
Over time, this journal will reveal patterns in your coding blind spots and help you develop personal strategies to overcome them.
Psychological Techniques for Better Debugging
Beyond the practical strategies, certain psychological approaches can improve your debugging abilities:
Mindfulness
Practicing mindfulness can help you stay present and focused when reviewing code, making you less likely to skim over details or make assumptions. Take deliberate, slow breaths and consciously focus your attention on each line of code.
Growth Mindset
View bugs as learning opportunities rather than failures. This mindset shift reduces the defensive reactions that can prevent you from seeing flaws in your own code.
Metacognition
Develop awareness of your own thinking processes while coding and debugging. Ask yourself questions like:
- What assumptions am I making right now?
- Am I rushing because I think this should be simple?
- Have I considered all possible execution paths?
This self-awareness can help you recognize and counteract your cognitive biases.
Conclusion: Embracing the Struggle
The challenge of finding bugs in your own code is a universal experience among programmers, rooted in fundamental aspects of human psychology. Rather than being frustrated by this limitation, embrace it as an inherent part of the software development process.
By understanding the psychological factors at play and implementing structured strategies to counteract them, you can significantly improve your ability to debug your own code. Remember that even the most experienced developers face this challenge, and the best coding environments acknowledge it through practices like pair programming and code reviews.
Ultimately, becoming a better debugger is about developing mental discipline, systematic approaches, and a willingness to see your code through different perspectives. As you apply the techniques discussed in this article, you'll not only find bugs more efficiently but also write more robust code from the start.
The next time you find yourself staring at your code for hours, unable to see a bug that later seems obvious, be kind to yourself. It's not a failure of intelligence or attention, but simply your brain doing what brains do. Take a break, change your approach, and return with fresh eyes—the bug will often reveal itself when you do.
Frequently Asked Questions
Why do experienced programmers still struggle to find bugs in their own code?
Experience doesn't eliminate the cognitive biases that cause code blindness. In fact, expertise can sometimes worsen the curse of knowledge, as experienced programmers have stronger mental models and expectations about how their code should work. However, experienced developers typically have better strategies for overcoming these biases and more effective debugging techniques.
How long should I take a break before reviewing my own code?
Even a short break of 15-30 minutes can be beneficial. For complex problems, waiting overnight can provide maximum mental distance. The ideal duration depends on the complexity of the code and how deeply you've been immersed in it. The goal is to clear your working memory enough that you'll read what's actually there rather than what you remember writing.
Can automated tools replace human code review?
While automated tools like linters and static analyzers are valuable for catching many types of errors, they can't fully replace human review. Automated tools excel at finding syntax errors, potential runtime exceptions, and violations of coding standards. However, they struggle with logical errors, unclear naming, missed requirements, and higher-level design issues that human reviewers can readily identify.
Does this phenomenon occur in other creative fields beyond programming?
Yes, similar effects occur in writing, design, music composition, and other creative fields. Writers often miss typos and logical inconsistencies in their own work that readers immediately notice. The psychological factors—particularly the curse of knowledge and confirmation bias—apply broadly to any field where creators review their own work.