Why You Can’t Explain Your Approach While Coding: Overcoming the Communication Gap
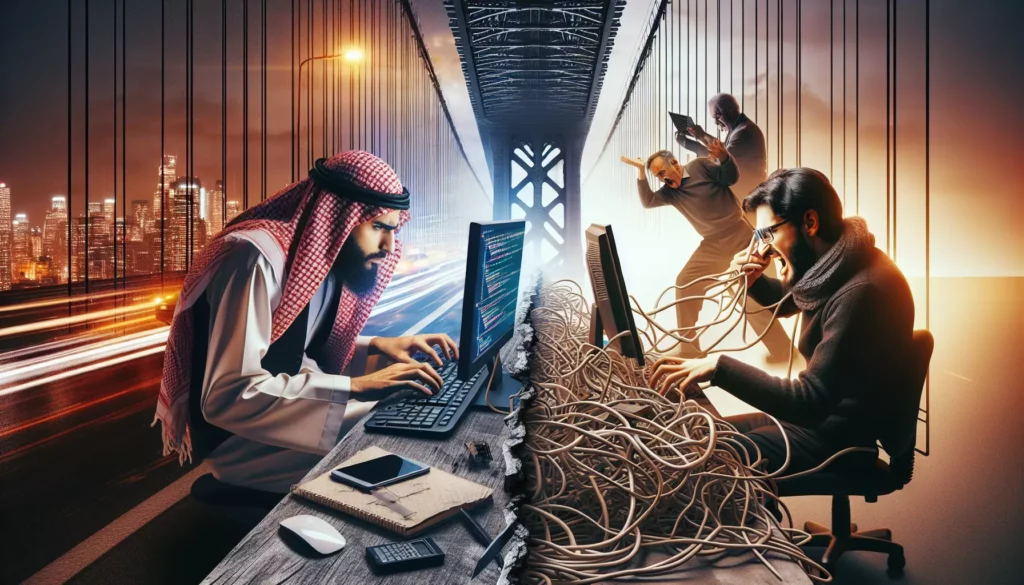
Have you ever found yourself staring at a problem, knowing exactly how to solve it in code, but struggling to articulate your approach when asked? Or perhaps you’ve been in a technical interview where your mind goes blank when trying to explain your thought process, even though your fingers are itching to just start typing the solution?
This disconnect between doing and explaining is a common challenge among programmers at all levels. Today, we’re diving deep into why this happens and how you can bridge this communication gap to become not just a better coder, but a more effective communicator of your technical knowledge.
The Silent Programmer Phenomenon
Programming is often portrayed as a solitary activity—a lone developer typing away furiously in a dimly lit room, speaking only in code. While this stereotype is exaggerated, there’s some truth to the idea that many programmers develop their skills in relative isolation. This can lead to what I call the “Silent Programmer Phenomenon”—being fluent in code but struggling with verbal explanations.
When you’re coding alone, you’re engaging in a dialogue with the computer, not with other humans. The computer doesn’t need you to explain your reasoning; it only cares if your syntax is correct and your logic produces the expected output. This creates a habit of thinking in code rather than in explanatory language.
The Cognitive Science Behind the Struggle
The difficulty in explaining your coding approach isn’t just about communication skills—it’s rooted in cognitive science. Several factors contribute to this challenge:
1. Different Brain Regions for Doing vs. Explaining
Research in neuroscience suggests that the parts of your brain engaged when solving a problem are different from those used when explaining that solution. Coding activates procedural memory and problem-solving circuits, while explanation requires verbal processing areas and metacognitive awareness.
When you write code, you’re primarily using the dorsolateral prefrontal cortex for logical reasoning and the motor cortex for typing. But explaining requires engagement of Broca’s area and other language centers, creating a cognitive switch that can feel jarring.
2. Implicit vs. Explicit Knowledge
Much of your coding knowledge becomes implicit over time—you know how to do something without consciously thinking about each step. This is similar to how experienced drivers don’t think about the individual actions of driving; they just drive.
Psychologist Michael Polanyi described this as “tacit knowledge” or “we know more than we can tell.” Your fingers might automatically type a for-loop or implement a binary search algorithm, but articulating why you chose that approach requires converting implicit knowledge into explicit knowledge—a challenging cognitive task.
3. The Curse of Knowledge
Once you understand something well, it becomes difficult to remember what it was like not to understand it. This cognitive bias, known as the “curse of knowledge,” makes it hard to explain concepts at an appropriate level for listeners who don’t share your background knowledge.
For experienced programmers, certain concepts become so obvious that they skip explaining crucial steps or assumptions when describing their approach, leaving listeners confused.
Real-World Implications
The inability to clearly explain your coding approach has significant consequences in professional settings:
Technical Interviews
Technical interviews at companies like Google, Amazon, and other tech giants typically require candidates to not only solve coding problems but to think aloud and explain their approach. Many capable programmers fail these interviews not because they can’t solve the problems, but because they can’t effectively communicate their thought process.
Consider this common feedback from interviewers: “The candidate found the correct solution but didn’t adequately explain their thinking. We’re concerned about how they would collaborate with team members.”
Team Collaboration
In collaborative environments, code that can’t be explained creates technical debt. If you implement a clever algorithm but can’t explain how it works, your team will struggle to maintain or modify it later. As the saying goes, “Code is read more often than it is written.”
Teaching and Mentoring
If you aspire to become a technical lead, mentor junior developers, or teach programming, the ability to explain concepts clearly becomes essential. Your technical knowledge has limited value if you can’t transfer it to others.
Common Scenarios Where Explanation Fails
Let’s examine some typical situations where programmers struggle to explain their approach:
The Algorithm Intuition Gap
You might intuitively know that a particular algorithm is the right choice for a problem, but struggle to articulate why. For instance, you might instantly recognize that a problem calls for dynamic programming, but fumble when asked to explain how you reached that conclusion.
Consider this common dialogue:
Interviewer: “Why did you choose dynamic programming for this problem?”
Programmer: “Um, because it has overlapping subproblems… and, uh, optimal substructure… and… can I just show you the code?”
The Code First, Explain Later Trap
Many programmers prefer to write code first and explain afterward. While this approach works for some problems, it often leads to disjointed explanations and gives the impression that you’re solving by trial and error rather than through structured thinking.
The Terminology Freeze
Sometimes you understand a concept perfectly but forget the formal terminology. You might implement a breadth-first search algorithm correctly but blank on the name “breadth-first search” when explaining your approach.
The Psychological Barriers
Beyond cognitive factors, psychological elements also contribute to the difficulty in explaining code:
Performance Anxiety
Explaining your approach, especially in high-pressure situations like job interviews, triggers performance anxiety. This anxiety consumes cognitive resources that would otherwise be available for clear articulation of your thoughts.
Impostor Syndrome
Many programmers suffer from impostor syndrome—the feeling that they’re not as competent as others perceive them to be. This can lead to overthinking explanations or doubting your approach even when it’s correct.
Fear of Judgment
Programming culture sometimes glorifies clever, concise solutions. The fear that your approach might be judged as inefficient or inelegant can make you hesitant to explain it fully.
Strategies to Improve Your Explanation Skills
Now that we understand the problem, let’s explore practical strategies to enhance your ability to explain your coding approach:
1. Practice Thinking Aloud
Make it a habit to verbalize your thought process while coding, even when working alone. This builds the neural pathways needed to translate your implicit coding knowledge into explicit verbal explanations.
Exercise: Solve a problem on a platform like LeetCode, but record yourself explaining each step as you code. Review the recording to identify gaps in your explanation.
2. Develop a Structured Explanation Framework
Create a mental template for explaining solutions. One effective framework is:
- Problem understanding: Restate the problem and clarify constraints
- Approach selection: Explain why you chose a particular approach
- Algorithm outline: Provide a high-level description of your algorithm
- Implementation details: Dive into specific code structures
- Complexity analysis: Discuss time and space complexity
By following this structure consistently, explanation becomes more automatic and less cognitively demanding.
3. Master the Art of Analogies
Complex algorithms and data structures often become clearer when explained through analogies. Develop a repertoire of analogies for common programming concepts.
For example, instead of giving a technical definition of recursion, you might say: “Recursion is like looking up a word in a dictionary, only to find that the definition contains another word you don’t know, so you have to look that up too, and so on, until you reach a word you understand. Then you work your way back up to understand the original word.”
4. Visualize Before Verbalizing
Many programming concepts are inherently visual. Before explaining an algorithm, sketch it out or visualize its execution in your mind. This activates different cognitive pathways and can make verbal explanation easier.
Tools like diagrams, flowcharts, or even simple pen-and-paper illustrations can bridge the gap between your understanding and your explanation.
5. Simulate Teaching to Different Audiences
Practice explaining the same concept to imaginary audiences with different levels of technical knowledge—a fellow senior developer, a junior programmer, and a non-technical person. This exercise helps you identify the core concepts versus the technical details and improves your ability to adjust explanations on the fly.
Practical Examples: Before and After
Let’s see how these strategies might transform explanations for common coding problems:
Example 1: Explaining a Binary Search Implementation
Before (Unclear Explanation):
“I’m using binary search here. So I set low and high pointers and find the middle. Then I check if the target is at the middle. If not, I move the pointers and repeat until I find it or the pointers cross.”
After (Clear Explanation):
“I’ve chosen binary search for this problem because we’re working with a sorted array, which allows us to eliminate half of the remaining elements with each comparison, giving us logarithmic time complexity.
Let me walk through my approach: I’ll maintain two pointers, ‘low’ and ‘high’, initially pointing to the first and last elements of the array. In each iteration, I calculate the middle position and compare the element there with our target value.
If they match, we’ve found our answer. If the middle element is greater than our target, we know our target must be in the left half, so we move the ‘high’ pointer to middle-1. Conversely, if the middle element is less than our target, we focus on the right half by moving ‘low’ to middle+1.
This process continues until either we find the target or the ‘low’ pointer exceeds the ‘high’ pointer, indicating the target isn’t present in the array.
The time complexity is O(log n) because we divide the search space in half with each iteration, and the space complexity is O(1) as we only use a constant amount of extra space regardless of input size.”
Example 2: Explaining a Dynamic Programming Solution
Before (Unclear Explanation):
“This is a classic DP problem. I’ll create a table and fill it using the recurrence relation. The answer will be in the bottom-right cell.”
After (Clear Explanation):
“I recognized this as a dynamic programming problem because it has two key properties: overlapping subproblems (we would recalculate the same scenarios multiple times in a naive approach) and optimal substructure (the optimal solution can be constructed from optimal solutions of its subproblems).
Let me explain my thought process: First, I’ll define what each cell in my DP table represents—dp[i][j] will store the minimum cost to reach position (i,j) from the starting point.
For the base case, dp[0][0] is just the cost of the starting cell itself. For cells in the first row, we can only reach them by moving right from previous cells, and similarly, for cells in the first column, we can only reach them by moving down.
For all other cells, we have two possible ways to reach them: from above or from the left. The recurrence relation is:
dp[i][j] = grid[i][j] + min(dp[i-1][j], dp[i][j-1])
After filling the entire table bottom-up, dp[m-1][n-1] will contain our answer—the minimum cost to reach the bottom-right cell.
This approach has O(m*n) time complexity because we fill each cell in the m×n table exactly once, and O(m*n) space complexity for storing the DP table, though we could optimize this to O(n) by only keeping track of the previous row.”
Bridging Code and Communication in Technical Interviews
Technical interviews particularly highlight the gap between coding ability and explanation skills. Here are specific strategies for this high-pressure context:
1. Clarify the Problem First
Before diving into solutions, demonstrate your understanding of the problem by restating it and asking clarifying questions. This not only ensures you’re solving the right problem but also gives you time to organize your thoughts.
Example questions to ask:
- “What are the constraints on the input size?”
- “Should I handle edge cases like empty arrays or negative values?”
- “Can I assume the input is always valid?”
2. Think Aloud, But Strategically
Interviewers want to follow your thought process, but this doesn’t mean verbalizing every fleeting idea. Structure your thinking aloud into clear phases:
- Consider multiple approaches first (“I see three potential approaches to this problem…”)
- Evaluate each approach briefly (“The first approach would be O(n²) which might be too slow…”)
- Commit to one approach and explain why (“I’ll implement the sliding window approach because…”)
- Outline your algorithm before coding (“First, I’ll initialize two pointers…”)
3. Use the STAR Method for System Design
For system design questions, adapt the STAR method (Situation, Task, Action, Result) to structure your explanation:
- Situation: Define the problem scope and constraints
- Task: Break down the key components needed
- Action: Explain your design decisions and tradeoffs
- Result: Discuss how your solution meets the requirements and how it would scale
Code Documentation as Explanation Practice
Writing good documentation is excellent practice for explaining code. It forces you to articulate your thought process in a clear, structured way. Here’s an example of well-documented code:
/**
* Finds the longest substring without repeating characters in a given string.
* Uses the sliding window technique to achieve O(n) time complexity.
*
* @param {string} s - The input string
* @return {number} - Length of the longest substring without repeating characters
*/
function lengthOfLongestSubstring(s) {
// Edge case: empty string
if (s.length === 0) return 0;
// Map to store the last seen index of each character
const charMap = new Map();
// Initialize variables to track window and result
let left = 0;
let maxLength = 0;
// Iterate through the string with right pointer
for (let right = 0; right < s.length; right++) {
const currentChar = s[right];
// If we've seen this character before and it's within our current window
if (charMap.has(currentChar) && charMap.get(currentChar) >= left) {
// Move left pointer to position after the last occurrence
left = charMap.get(currentChar) + 1;
}
// Update the last seen position of current character
charMap.set(currentChar, right);
// Update max length if current window is larger
maxLength = Math.max(maxLength, right - left + 1);
}
return maxLength;
}
Notice how the comments explain not just what the code does, but why certain approaches were chosen and how the algorithm works conceptually.
Teaching Others: The Ultimate Test
Richard Feynman, the renowned physicist, popularized a technique for deeply understanding concepts: explain them as if you’re teaching a beginner. This “Feynman Technique” is particularly valuable for programmers:
- Choose a concept (e.g., recursion, dynamic programming)
- Explain it in simple terms, as if to a complete beginner
- Identify gaps in your explanation where you struggle or resort to jargon
- Go back to the source material to improve your understanding
- Simplify and use analogies until your explanation is clear and concise
Volunteering to teach or mentor junior developers, contributing to documentation, or writing technical blog posts are all practical ways to apply this technique.
The Role of Code Reviews in Improving Explanation Skills
Code reviews provide a structured opportunity to practice explaining your code. When submitting code for review, don’t just wait for feedback—proactively explain your approach in the pull request description.
A good pull request explanation includes:
- The problem being solved
- Your approach and why you chose it
- Any alternative approaches considered
- Specific areas where you’d like feedback
Similarly, when reviewing others’ code, ask clarifying questions that prompt them to explain their thinking. This creates a culture where articulating the reasoning behind code becomes normalized.
Building a Personal Library of Explanations
Create your own repository of clear explanations for common algorithms and data structures. When you successfully explain a concept in a way that resonates with others, document that explanation for future use.
This library becomes particularly valuable when preparing for interviews or when mentoring others. Over time, you’ll develop a personal style of explanation that works for you and your audience.
Leveraging Visualization Tools
Sometimes, words alone aren’t enough. Visualization tools can bridge the gap between your understanding and your explanation:
- Algorithm visualizers like VisuAlgo or Algorithm Visualizer help demonstrate how algorithms work step-by-step
- Diagramming tools like draw.io or Excalidraw allow you to create visual representations of data structures
- Code execution visualizers like Python Tutor show how code executes line by line, making it easier to explain program flow
Including visual aids in your explanations can dramatically improve comprehension, especially for complex concepts.
The Connection Between Writing and Explaining
Writing and verbal explanation share many cognitive processes. Improving your technical writing skills often translates to better verbal explanations.
Practice writing explanations of your code or solutions to programming problems. Focus on clarity, logical flow, and avoiding unnecessary jargon. Have peers review your writing and provide feedback.
Over time, this practice builds the mental muscles needed for clear verbal communication about code.
Overcoming the Terminology Barrier
Forgetting technical terms during explanations is common and can derail your confidence. Develop strategies to work around terminology gaps:
- Keep a glossary of terms you frequently forget
- Practice using proper terminology in your everyday coding discussions
- When you forget a term, describe the concept instead of freezing (“I’m using that algorithm where you repeatedly divide the search space in half” instead of blanking when you forget “binary search”)
Remember that understanding concepts is more important than remembering names. A clear explanation using simple language is better than silence while searching for the perfect term.
Cultural and Language Considerations
For non-native English speakers in predominantly English-speaking tech environments, explaining code presents additional challenges. If this applies to you:
- Build a vocabulary of technical terms and practice using them in context
- Practice explaining concepts in English specifically, even if it feels uncomfortable at first
- Consider joining communities like Toastmasters that focus on public speaking skills
- Remember that technical knowledge is valuable regardless of language fluency
Similarly, be mindful of cultural differences in communication styles. Some cultures value concise, direct explanations, while others prefer more context and background information.
Conclusion: The Journey from Coder to Communicator
The gap between coding ability and explanation skills is not fixed—it’s a spectrum that you can move along with deliberate practice. As you progress in your career, the ability to clearly articulate your technical thoughts becomes increasingly valuable, often differentiating senior engineers from junior ones.
Remember that explaining code is a skill separate from writing code. It requires different cognitive processes and practice. By understanding the psychological and cognitive barriers to clear explanation and implementing the strategies outlined in this article, you can become not just a better programmer, but a more effective communicator of complex technical ideas.
The next time you find yourself struggling to explain your approach while coding, remember that this is a common challenge with specific solutions. With practice and the right strategies, you can bridge the gap between knowing and explaining, unlocking new opportunities for collaboration, leadership, and growth in your programming career.
What strategies have you found helpful for explaining your code? Share your experiences and tips in the comments below!