Why You Can’t Evaluate Solution Trade-offs (And How to Fix It)
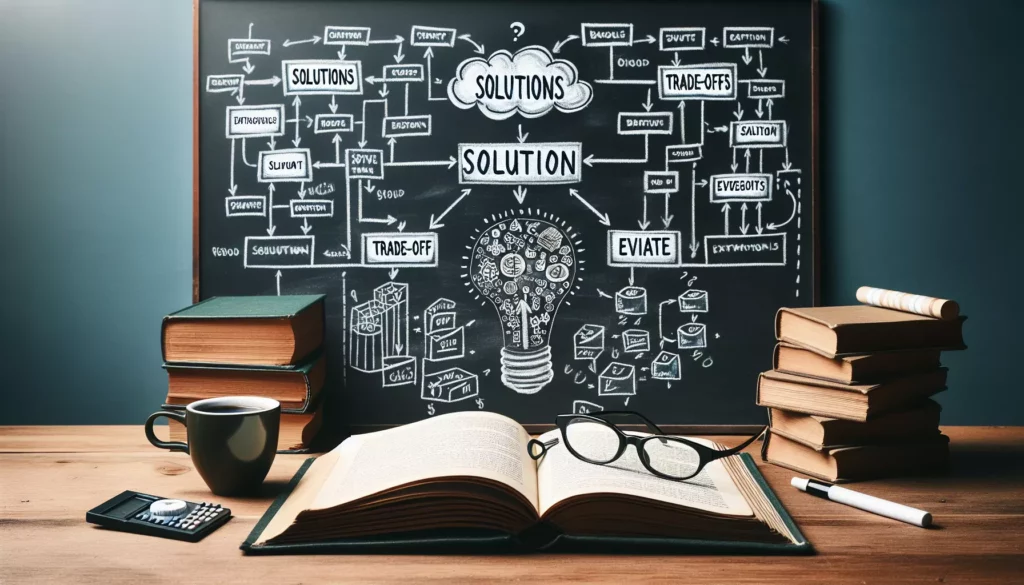
If you’ve ever found yourself stuck between multiple coding solutions, unsure which one to choose, you’re not alone. Many programmers, from beginners to seasoned professionals, struggle with evaluating solution trade-offs effectively.
This critical skill—the ability to weigh the pros and cons of different approaches—often separates good programmers from great ones. Yet it’s rarely taught explicitly in coding education.
In this comprehensive guide, we’ll explore why evaluating solution trade-offs is challenging, why it matters, and most importantly, how to develop this essential skill to level up your programming abilities.
The Hidden Challenge in Programming Education
Programming education typically focuses on syntax, algorithms, and problem-solving techniques. While these fundamentals are essential, they often leave a significant gap: the nuanced decision-making process that experienced developers use when choosing between multiple valid solutions.
Consider this scenario: You’ve just solved a coding problem and your solution works perfectly. But is it the best approach? Could it be more efficient? More readable? More maintainable? These questions reveal the complex landscape of trade-offs that every programmer navigates daily.
Why Trade-off Evaluation Is Difficult
1. The Multidimensional Nature of Code Quality
Code isn’t just about correctness—it exists in a multidimensional space where various qualities matter:
- Time complexity: How execution time scales with input size
- Space complexity: Memory requirements
- Readability: How easily others can understand your code
- Maintainability: How easily the code can be modified
- Scalability: How well the solution adapts to growing demands
- Robustness: How well it handles edge cases and unexpected inputs
Optimizing for one dimension often means compromising on others. For instance, the most time-efficient algorithm might be complex and difficult to maintain, while the most readable solution might not perform well at scale.
2. Context Dependency
There’s rarely a universally “best” solution. The right approach depends heavily on context:
- Is this code for a one-time script or a long-term project?
- Will it run on resource-constrained devices or powerful servers?
- Is it part of a critical path that demands maximum performance?
- Who will maintain this code in the future?
Without clear context, evaluating trade-offs becomes a shot in the dark.
3. Experience Gap
Experienced developers have seen the long-term consequences of various design choices, giving them intuition about trade-offs that beginners simply haven’t developed yet. This experience gap makes it difficult for newer programmers to accurately predict the implications of their design decisions.
4. The Moving Target Problem
Technology evolves rapidly. What constitutes a good trade-off today might be suboptimal tomorrow due to:
- Changes in hardware capabilities
- New language features
- Evolving best practices
- Shifting project requirements
This moving target makes trade-off evaluation a perpetual learning process, even for experts.
The Cost of Poor Trade-off Evaluation
Failing to properly evaluate solution trade-offs can lead to significant problems:
Technical Debt
Choosing solutions without considering long-term implications often results in technical debt. What seems expedient now may become a maintenance nightmare later.
Performance Issues
Prioritizing readability or development speed without considering performance can lead to systems that collapse under real-world loads.
Wasted Resources
Overengineering—optimizing prematurely or implementing unnecessarily complex solutions—wastes development resources and can make code harder to maintain.
Career Limitations
In technical interviews, especially at top tech companies, candidates are explicitly evaluated on their ability to discuss trade-offs. Struggling with this skill can limit career advancement opportunities.
A Framework for Evaluating Solution Trade-offs
Let’s develop a systematic approach to evaluating trade-offs in your coding solutions:
Step 1: Identify Your Evaluation Dimensions
Before comparing solutions, clarify which dimensions matter for your specific context. Common dimensions include:
- Performance metrics: Time complexity, space complexity, actual runtime
- Development metrics: Implementation time, code simplicity
- Maintenance metrics: Readability, modularity, testability
- Operational metrics: Reliability, scalability, security
For each problem, determine which of these dimensions are most important given your context.
Step 2: Analyze Multiple Solutions
For any non-trivial problem, develop at least two different solutions. This forces you to think beyond your first instinct and explore the solution space more thoroughly.
For each solution, analyze how it performs across your identified dimensions. Be specific and quantitative where possible:
- “Solution A has O(n) time complexity but O(n) space complexity”
- “Solution B has O(n log n) time complexity but O(1) space complexity”
- “Solution A uses standard library functions, making it more readable”
- “Solution B requires custom logic but eliminates an extra pass through the data”
Step 3: Consider Context and Constraints
Evaluate your solutions in light of your specific context:
- Hardware constraints: Is memory limited? Is CPU power abundant?
- Scale expectations: Will this handle 10 items or 10 million?
- Team factors: What’s the expertise level of your team?
- Future considerations: How might requirements evolve?
Step 4: Make Explicit Trade-offs
Based on your analysis, make deliberate trade-off decisions and document your reasoning. For example:
“We’re choosing Solution B despite its higher implementation complexity because memory is our primary constraint, and the O(1) space complexity will allow us to scale to larger datasets.”
This explicit reasoning helps both you and others understand why certain decisions were made, making it easier to revisit those decisions if circumstances change.
Practical Examples: Evaluating Trade-offs in Common Problems
Let’s apply our framework to some typical coding problems to see how trade-off evaluation works in practice.
Example 1: Finding Duplicates in an Array
Problem: Given an array of integers, find any duplicate.
Solution A: Using a Hash Set
def find_duplicate_hash(nums):
seen = set()
for num in nums:
if num in seen:
return num
seen.add(num)
return -1 # No duplicate found
Solution B: Sorting First
def find_duplicate_sort(nums):
nums.sort()
for i in range(1, len(nums)):
if nums[i] == nums[i-1]:
return nums[i]
return -1 # No duplicate found
Solution C: Floyd’s Tortoise and Hare (Cycle Detection)
def find_duplicate_floyd(nums):
slow = fast = nums[0]
# Find meeting point
while True:
slow = nums[slow]
fast = nums[nums[fast]]
if slow == fast:
break
# Find cycle entrance
slow = nums[0]
while slow != fast:
slow = nums[slow]
fast = nums[fast]
return slow
Trade-off Analysis:
- Time Complexity:
- Solution A: O(n) with hash set lookups
- Solution B: O(n log n) due to sorting
- Solution C: O(n) using cycle detection
- Space Complexity:
- Solution A: O(n) for the hash set
- Solution B: O(1) if using in-place sort
- Solution C: O(1) using only two pointers
- Readability:
- Solution A: Very readable, straightforward approach
- Solution B: Fairly readable, familiar sorting pattern
- Solution C: Less intuitive, requires understanding cycle detection
Context-Based Decision:
- If memory is constrained but you have time, Solution B is reasonable
- If both memory and optimal time complexity matter, Solution C is best but comes with readability costs
- For most practical scenarios, Solution A offers the best balance of efficiency and readability
- In an interview setting, demonstrating knowledge of all three and discussing trade-offs would be impressive
Example 2: Implementing a Cache
Problem: Implement a cache with get and put operations.
Solution A: Simple Dictionary/Hash Map
class SimpleCache:
def __init__(self):
self.cache = {}
def get(self, key):
return self.cache.get(key, -1)
def put(self, key, value):
self.cache[key] = value
Solution B: LRU Cache with Doubly Linked List
class LRUCache:
class Node:
def __init__(self, key, val):
self.key = key
self.val = val
self.prev = None
self.next = None
def __init__(self, capacity):
self.capacity = capacity
self.cache = {} # key to node mapping
self.head = self.Node(-1, -1) # dummy head
self.tail = self.Node(-1, -1) # dummy tail
self.head.next = self.tail
self.tail.prev = self.head
def _add_node(self, node):
# Always add to head
node.prev = self.head
node.next = self.head.next
self.head.next.prev = node
self.head.next = node
def _remove_node(self, node):
node.prev.next = node.next
node.next.prev = node.prev
def _move_to_head(self, node):
self._remove_node(node)
self._add_node(node)
def _pop_tail(self):
node = self.tail.prev
self._remove_node(node)
return node
def get(self, key):
if key not in self.cache:
return -1
node = self.cache[key]
self._move_to_head(node) # Update as recently used
return node.val
def put(self, key, value):
if key in self.cache:
node = self.cache[key]
node.val = value
self._move_to_head(node)
return
# New key
node = self.Node(key, value)
self.cache[key] = node
self._add_node(node)
# Check capacity
if len(self.cache) > self.capacity:
tail = self._pop_tail()
del self.cache[tail.key]
Trade-off Analysis:
- Time Complexity:
- Solution A: O(1) for both operations
- Solution B: O(1) for both operations
- Space Complexity:
- Solution A: O(n) with no size limit
- Solution B: O(capacity) with enforced limit
- Implementation Complexity:
- Solution A: Very simple, few lines of code
- Solution B: Significantly more complex with linked list maintenance
- Features:
- Solution A: Basic caching only
- Solution B: Includes eviction policy (LRU)
Context-Based Decision:
- For a simple application with known memory limits, Solution A might be sufficient
- For production systems where memory management is critical, Solution B’s eviction policy is essential
- If development time is constrained, starting with Solution A and evolving to B if needed might be pragmatic
Common Pitfalls in Trade-off Evaluation
Even with a framework, certain pitfalls can derail your trade-off evaluation:
1. Premature Optimization
As Donald Knuth famously said, “Premature optimization is the root of all evil.” Overemphasizing performance before it’s proven necessary leads to unnecessarily complex code.
How to avoid it: Start with clear, readable solutions. Optimize only when measurements indicate a need.
2. Analysis Paralysis
Overthinking trade-offs can lead to decision paralysis, where you spend more time analyzing than implementing.
How to avoid it: Set a time limit for analysis. Remember that most decisions aren’t permanent—you can refactor later if needed.
3. False Dichotomies
Thinking in binary terms (“either readable or efficient”) misses creative solutions that might achieve multiple goals.
How to avoid it: Challenge yourself to find solutions that satisfy multiple criteria. Often, a third approach exists that offers a better balance.
4. Recency Bias
Favoring familiar or recently learned techniques regardless of their appropriateness for the current problem.
How to avoid it: Deliberately consider multiple approaches, especially those you haven’t used recently.
5. Ignoring Team Factors
Choosing technically optimal solutions without considering your team’s familiarity with the approach.
How to avoid it: Include team capabilities in your evaluation criteria. The “best” solution is one your team can implement and maintain effectively.
How to Develop Your Trade-off Evaluation Skills
Like any skill, evaluating trade-offs improves with deliberate practice:
1. Solve Problems Multiple Ways
Challenge yourself to solve each coding problem with at least two different approaches. Compare them systematically using the framework above.
2. Study Algorithm Design
Algorithm textbooks often discuss trade-offs explicitly. Study classic algorithms and the design decisions behind them.
3. Code Reviews
Participate in code reviews, focusing not just on correctness but on design choices. Ask questions like “Why did you choose this approach over alternatives?”
4. Read Open Source Code
Examine how experienced developers make trade-offs in real-world projects. Look for comments explaining design decisions.
5. Retrospective Analysis
Revisit your old code and analyze how your design decisions played out. Would you make the same choices today?
6. Deliberate Refactoring
Take working code and refactor it with different priorities (e.g., optimize for readability, then for performance). Compare the results.
7. Mock Interviews with Trade-off Discussions
Practice explaining your solution trade-offs out loud. This verbalization helps clarify your thinking and prepares you for technical interviews.
Trade-off Evaluation in Technical Interviews
At top tech companies, the ability to evaluate trade-offs is explicitly tested during interviews. Interviewers want to see that you:
- Can generate multiple valid solutions
- Understand the pros and cons of each approach
- Can select appropriate solutions based on context
- Communicate your reasoning clearly
When interviewing, follow these steps:
- Start by providing a working solution
- Analyze its time and space complexity
- Suggest alternative approaches
- Compare trade-offs explicitly
- Recommend what you think is best for the given context
For example, rather than just saying “This is an O(n) solution,” say something like:
“This solution has O(n) time complexity and O(n) space complexity. An alternative would be to sort the array first, which would give us O(n log n) time but O(1) extra space. Given that we’re not constrained on memory in this problem, I’d recommend the first approach for its better time complexity and more straightforward implementation.”
Real-world Trade-offs: Beyond Algorithmic Complexity
While algorithmic complexity is important, real-world software development involves broader trade-offs:
Build vs. Buy
Should you implement a solution yourself or use an existing library or service?
Trade-offs include:
- Development time vs. customization needs
- Licensing costs vs. maintenance burden
- External dependencies vs. control
Monolith vs. Microservices
Should your application be structured as a single codebase or as multiple independent services?
Trade-offs include:
- Development simplicity vs. deployment flexibility
- Consistent technology stack vs. service-specific optimizations
- Easier local development vs. better scaling characteristics
Eager vs. Lazy Computation
Should you compute values upfront or on-demand?
Trade-offs include:
- Predictable performance vs. resource efficiency
- Simpler code vs. better average-case performance
- Upfront costs vs. unpredictable latency spikes
Abstraction vs. Concreteness
How generic should your interfaces and implementations be?
Trade-offs include:
- Reusability vs. simplicity
- Future-proofing vs. current needs
- Flexibility vs. performance optimization
Learning to navigate these higher-level trade-offs is essential for senior engineering roles and system design interviews.
The Evolution of Trade-off Evaluation
As you grow as a developer, your approach to trade-offs will evolve:
Beginner: Focused on Correctness
Beginners primarily focus on getting a working solution. Trade-off evaluation is minimal or nonexistent.
Intermediate: Focused on Complexity Analysis
Intermediate developers can analyze time and space complexity and make basic trade-offs between these dimensions.
Advanced: Contextual Optimization
Advanced developers consider a wider range of factors and tailor their solutions to specific contexts and constraints.
Expert: Predictive Trade-offs
Experts can predict how various solutions will perform as requirements evolve, making trade-offs that account for future needs.
Conclusion: Embracing the Art of Trade-offs
Evaluating solution trade-offs isn’t just a technical skill—it’s the essence of engineering. Engineers don’t just build solutions; they build appropriate solutions given specific contexts and constraints.
By developing a systematic approach to trade-off evaluation, you’ll:
- Write more thoughtful, contextually appropriate code
- Make better architectural decisions
- Communicate your reasoning more effectively
- Perform better in technical interviews
- Grow faster as a developer
Remember that there’s rarely a perfect solution—just trade-offs. The goal isn’t to find solutions without downsides, but to choose solutions whose downsides you can live with given your specific context.
As you continue your programming journey, make trade-off evaluation an explicit part of your problem-solving process. Ask not just “Does this work?” but “Is this the right approach for this context?” Your code—and your career—will benefit immensely.
Further Learning Resources
To deepen your understanding of solution trade-offs, explore these resources:
- Books:
- “Clean Code” by Robert C. Martin
- “The Pragmatic Programmer” by Andrew Hunt and David Thomas
- “Designing Data-Intensive Applications” by Martin Kleppmann
- Online Courses:
- Algorithm specializations that emphasize multiple approaches
- System design courses that discuss architectural trade-offs
- Practice Platforms:
- LeetCode discussion forums where multiple solutions are compared
- AlgoCademy’s interactive problem-solving environment
The ability to evaluate trade-offs effectively is what separates programmers who can follow instructions from engineers who can make decisions. Invest in developing this skill, and watch your capabilities—and your career—transform.