Why You Can’t Design Solutions Before Coding: The Reality of Software Development
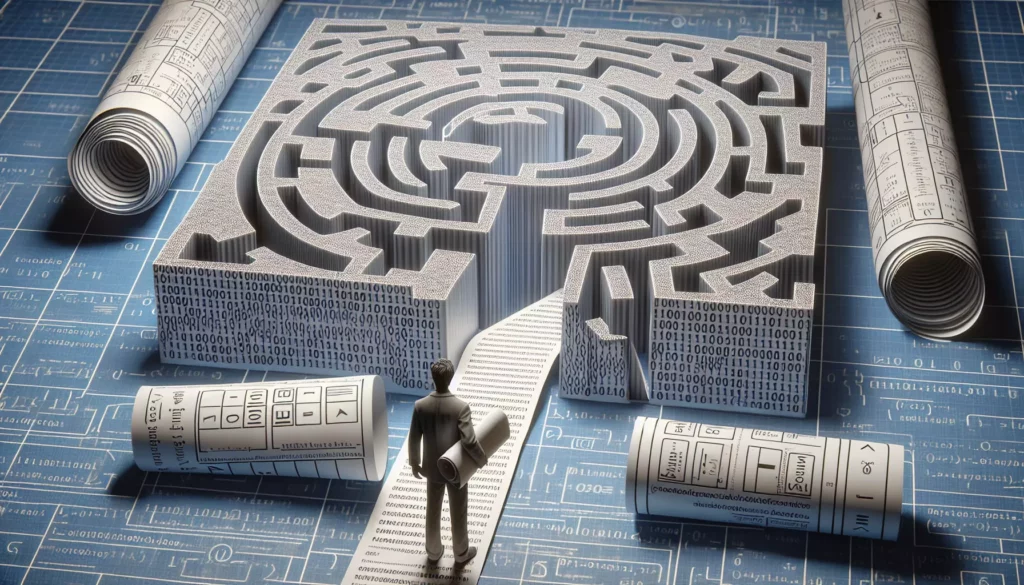
In the world of software development, there’s a commonly held belief that proper design should always precede coding. This waterfall-like approach suggests that developers should meticulously plan every aspect of their solution before writing a single line of code. While this sounds logical in theory, the reality of modern software development tells a different story. Let’s explore why the “design first, code later” paradigm often falls short and what approaches work better in practice.
The Traditional Design-First Approach
Traditional software development methodologies emphasized extensive upfront design. The process typically looked something like this:
- Gather all requirements
- Create detailed design documents
- Plan the entire architecture
- Write the code according to the design
- Test the implementation
- Deploy the solution
This approach assumes several things:
- All requirements can be known in advance
- The problem domain is well understood
- The solution can be fully visualized before implementation
- Changes will be minimal once coding begins
But in reality, these assumptions rarely hold true, especially in today’s fast-paced, complex software landscape.
Why Complete Upfront Design Often Fails
1. Incomplete Understanding of the Problem Domain
When starting a new project or feature, developers rarely have a complete understanding of the problem domain. Even experienced developers working in familiar territories discover nuances and edge cases during implementation that weren’t apparent during the design phase.
Consider trying to design a solution for a natural language processing algorithm without first experimenting with different approaches. The complexity of language makes it nearly impossible to anticipate all the challenges you’ll face without getting your hands dirty with code.
2. Requirements Are Fluid, Not Fixed
In modern software development, requirements change constantly. Market conditions shift, user feedback arrives, and business priorities evolve. A rigid upfront design becomes outdated almost as soon as it’s completed.
For example, imagine spending weeks designing a detailed solution for a mobile app, only to learn that a key feature needs to be completely rethought based on new competitor analysis or user research. Your carefully crafted design might now be partially or wholly irrelevant.
3. Design in Abstraction Has Limitations
Thinking about code in the abstract is fundamentally different from actually writing it. When we design without coding, we miss the technical constraints and opportunities that only become apparent during implementation.
For instance, you might design a database schema that looks perfect on paper, but when you start implementing it, you realize that certain queries would be inefficient or that your chosen database technology has limitations you hadn’t considered.
4. Emergence of Better Solutions Through Coding
The act of coding itself often reveals better solutions than what was conceived during the design phase. As developers work through the implementation details, they discover more elegant approaches, optimizations, and simplifications that weren’t obvious earlier.
This is similar to how writing helps clarify thinking. Many writers don’t know exactly what they want to say until they start writing. Similarly, developers often don’t know the best way to solve a problem until they start coding.
The Cognitive Limitations of Upfront Design
Working Memory Constraints
Human working memory can typically hold only 7±2 items at once. Complex software systems involve hundreds or thousands of interacting components. It’s cognitively impossible to hold all these relationships in mind simultaneously when designing a solution.
When coding, we don’t need to keep everything in working memory because we can see the concrete implementation in front of us. The code itself becomes an extension of our thinking process, allowing us to offload cognitive burden.
The Illusion of Completeness
When creating design documents, there’s a tendency to believe we’ve covered all bases. This is often an illusion. Our brains are excellent at providing a sense of understanding even when that understanding is incomplete. Psychologists call this the “illusion of explanatory depth” — we think we understand something thoroughly until we’re asked to explain it in detail.
Coding forces us to be explicit and precise, revealing gaps in our understanding that weren’t apparent during the design phase.
Real-World Example: Algorithm Design
Let’s consider a concrete example familiar to many programmers: designing an algorithm to solve a complex problem.
Imagine you’re tasked with creating an efficient algorithm for finding the shortest path between two points in a complex network. You might start by thinking about Dijkstra’s algorithm or A* search. You sketch out the high-level approach on a whiteboard, feeling confident in your design.
However, once you start coding, you encounter several issues:
- The data structure you planned to use is inefficient for the specific operations needed
- Edge cases emerge that your design didn’t account for
- The memory requirements are higher than anticipated
- A particular optimization becomes obvious only when you see the code in action
These insights would have been extremely difficult, if not impossible, to discover during the design phase alone.
The Alternative: Iterative and Exploratory Approaches
If complete upfront design isn’t the answer, what is? Modern software development has evolved several approaches that acknowledge the limitations of pure design-first thinking:
1. Prototyping and Spike Solutions
Instead of designing the entire solution upfront, create quick, throwaway code (spikes) to explore the problem space. This allows you to learn about technical constraints and validate assumptions before committing to a design.
For example, before designing a full authentication system, you might write a simple prototype to test different OAuth providers and understand their quirks and limitations.
2. Test-Driven Development (TDD)
TDD flips the traditional design-code-test sequence by starting with tests. You write a failing test, implement just enough code to make it pass, then refactor. This approach allows the design to emerge organically through small, verifiable increments.
// Example of TDD approach for a simple sum function
// 1. Write the test first
test('sum adds two numbers correctly', () => {
expect(sum(2, 3)).toBe(5);
});
// 2. Implement the minimal code to pass the test
function sum(a, b) {
return a + b;
}
// 3. Refactor if needed (not necessary in this simple example)
TDD helps ensure that your design remains grounded in actual requirements rather than abstract speculation.
3. Iterative Development and Agile Methodologies
Agile methodologies embrace the idea that requirements will change and that the best designs emerge through iteration. Rather than designing everything upfront, you design, implement, and refine in small cycles, learning and adapting as you go.
This approach acknowledges that some of the most important insights come during implementation and user feedback, not during initial design.
4. Domain-Driven Design with Bounded Contexts
Domain-Driven Design (DDD) recognizes that large systems can’t be designed as a unified whole. Instead, it advocates for identifying bounded contexts — coherent subdomains of the larger problem — and designing them independently.
This approach reduces complexity by allowing you to focus on smaller, more manageable pieces rather than attempting to design the entire system at once.
Finding the Right Balance: Design Enough, Then Code
The solution isn’t to abandon design entirely but to find the right balance between design and implementation. Here’s a more balanced approach:
1. Design at the Right Level of Abstraction
Focus high-level design on architecture, major components, and interfaces between systems. Don’t try to design every class, method, or function before coding.
For example, you might decide on a microservices architecture and define the boundaries and communication patterns between services, but leave the internal implementation details of each service to emerge during coding.
2. Time-Box Design Activities
Set a limit on how much time you’ll spend on upfront design. This prevents analysis paralysis and acknowledges that diminishing returns set in after a certain point.
A good rule of thumb: when you start debating minor details that could easily change during implementation, it’s time to start coding.
3. Design with Flexibility in Mind
Rather than trying to design the perfect solution upfront, design for change. Create loosely coupled components with well-defined interfaces that can evolve independently.
// Example of designing for flexibility using dependency injection
// Instead of tightly coupling to a specific implementation:
class UserService {
constructor() {
this.repository = new MySQLUserRepository(); // Tightly coupled
}
}
// Design for flexibility:
class UserService {
constructor(userRepository) { // Dependency injection
this.repository = userRepository;
}
}
// Now we can easily change the implementation without modifying UserService
const service = new UserService(new MongoDBUserRepository());
4. Embrace Continuous Refactoring
Accept that your initial implementation won’t be perfect and that refactoring is a natural part of the development process. As your understanding of the problem deepens through coding, continuously improve your design.
This approach aligns with the reality that software development is more about discovery than construction.
Real-World Success Stories: When Less Design Led to Better Outcomes
Amazon’s Two-Pizza Teams
Amazon famously organizes its development teams to be small enough that they can be fed with two pizzas. These small teams don’t create exhaustive designs before coding. Instead, they work iteratively, releasing minimal viable products and improving based on real customer feedback.
This approach has allowed Amazon to innovate rapidly across a wide range of products and services, from e-commerce to cloud computing.
Google’s Gmail Development
Gmail began as a small prototype built by Paul Buchheit, who famously said, “If I had been required to produce a detailed spec first, it would have been much harder to build Gmail.”
Instead of extensive upfront design, Buchheit built working code quickly, demonstrated it to colleagues, and iterated based on feedback. This exploratory approach led to innovative features like conversation threading and generous storage that might not have emerged from a design-first process.
Spotify’s Squad Model
Spotify’s engineering culture emphasizes autonomy and rapid iteration over comprehensive upfront design. Their squad model allows small, cross-functional teams to experiment and implement solutions without being constrained by rigid design requirements.
This approach has enabled Spotify to continuously evolve its product in response to user needs and market changes.
When More Upfront Design Makes Sense
While this article argues against complete upfront design, there are situations where more extensive design is beneficial:
1. Safety-Critical Systems
For software controlling medical devices, aircraft, or nuclear facilities, the cost of failure is extremely high. In these cases, more rigorous upfront design and formal verification may be necessary.
2. Highly Distributed Teams
When teams are large and distributed across multiple locations or time zones, more detailed design documentation can help ensure everyone shares a common understanding.
3. Contractual Requirements
Some projects, particularly in government or enterprise contexts, have contractual obligations that require detailed specifications before implementation begins.
4. Stable, Well-Understood Domains
In domains that are stable and well-understood, where requirements change infrequently, more upfront design may be appropriate.
Even in these cases, however, some degree of iterative development and flexibility is usually beneficial.
Practical Tips for Effective Design in Modern Development
1. Use Lightweight Design Artifacts
Instead of comprehensive design documents, use lightweight artifacts that can be easily updated:
- CRC (Class-Responsibility-Collaboration) cards
- Whiteboard sketches (captured as photos)
- Simple UML diagrams focusing on key relationships
- Architecture Decision Records (ADRs)
2. Design in Code When Possible
For many aspects of design, the code itself can serve as the primary design artifact:
- Create interfaces or protocols that define the contract between components
- Implement skeleton classes with method signatures but minimal implementation
- Use comments to outline the intended behavior before filling in the details
// Design in code: defining an interface before implementation
interface PaymentProcessor {
boolean processPayment(Order order, PaymentMethod method);
boolean refundPayment(String transactionId);
PaymentStatus checkStatus(String transactionId);
}
// Now different implementations can be created as needed
class StripePaymentProcessor implements PaymentProcessor {
// Implementation details go here
}
3. Leverage Feature Flags and Canary Releases
Design your system to support experimentation through feature flags and gradual rollouts. This allows you to test design decisions with real users before fully committing to them.
// Example of using a feature flag
if (featureFlags.isEnabled("new-recommendation-algorithm")) {
recommendations = newRecommendationAlgorithm.getRecommendations(user);
} else {
recommendations = currentRecommendationAlgorithm.getRecommendations(user);
}
4. Practice Continuous Design Review
Rather than treating design as a one-time activity, incorporate design reviews throughout the development process:
- Pair programming sessions that focus on design decisions
- Regular architecture review meetings
- Code reviews that evaluate design choices, not just implementation details
Learning to Think While Coding: Skills for Modern Developers
The ability to design effectively while coding is a skill that can be developed. Here are some practices that can help:
1. Develop Incremental Thinking
Train yourself to break problems down into small, manageable pieces that can be implemented and tested independently. This reduces cognitive load and allows your design to evolve naturally.
2. Practice Reflective Coding
Regularly step back from your code to reflect on what you’ve learned and how it might influence your overall approach. Ask questions like:
- What assumptions did I make that turned out to be incorrect?
- What patterns are emerging in my implementation?
- How might these insights affect other parts of the system?
3. Build a Mental Library of Patterns
Familiarize yourself with common design patterns and architectural styles. This gives you a vocabulary for thinking about design and a set of proven solutions to common problems.
However, avoid forcing patterns where they don’t fit. Let the appropriate patterns emerge from your understanding of the specific problem.
4. Cultivate Technical Empathy
Develop the ability to see code from different perspectives — maintenance, performance, security, usability. This helps you make better design decisions as you code.
The Role of AI and Modern Tools in Design and Coding
Modern development tools, including AI assistants, are changing the relationship between design and coding:
1. AI-Assisted Coding
Tools like GitHub Copilot and ChatGPT can generate implementation details based on high-level descriptions, allowing developers to focus more on conceptual design while the AI handles routine coding tasks.
2. Interactive Visualization Tools
Tools that visualize code structure and dependencies in real-time help developers understand the emerging design of their system as they code.
3. Automated Refactoring
Modern IDEs offer powerful refactoring tools that make it easier to evolve your design as your understanding deepens, reducing the need for perfect upfront design.
4. Simulation and Modeling
Advanced tools allow developers to simulate and test designs before full implementation, bridging the gap between design and coding.
Conclusion: Embracing the Reality of Software Development
The notion that you can or should fully design a solution before coding is a myth that doesn’t align with how software development actually works. The most successful approaches recognize that:
- Design and implementation are intertwined, not sequential activities
- The act of coding itself provides essential insights that inform better design
- Human cognitive limitations make complete upfront design impractical for complex systems
- Iterative approaches that allow design to emerge through coding lead to better outcomes
Rather than clinging to the comforting but unrealistic idea of perfect upfront design, embrace the messy reality of software development. Design enough to start, code to learn, and let your solution evolve through continuous refinement.
This approach not only produces better software but also makes the development process more engaging and responsive to real-world needs. As you gain experience, you’ll develop the judgment to know how much design is enough before diving into code, finding the sweet spot between planning and discovery for each unique situation.
Remember: in software development, coding isn’t just the implementation of design — it’s an integral part of the design process itself.
Further Learning Resources
If you’re interested in exploring this topic further, here are some valuable resources:
- “Clean Code” by Robert C. Martin — While emphasizing good design, Martin acknowledges the iterative nature of software development.
- “Domain-Driven Design” by Eric Evans — Explores how to create effective designs that evolve with your understanding of the problem domain.
- “Refactoring” by Martin Fowler — Demonstrates how to improve design incrementally through systematic code changes.
- “The Pragmatic Programmer” by Andrew Hunt and David Thomas — Offers practical advice on balancing design and implementation.
- AlgoCademy’s interactive tutorials — Practice algorithmic thinking and problem-solving with guidance that helps you develop the ability to design while coding.
By developing a balanced approach to design and coding, you’ll become a more effective developer, capable of creating solutions that are both well-structured and adaptable to changing requirements.