Why You Can’t Debug Your Code During Technical Interviews
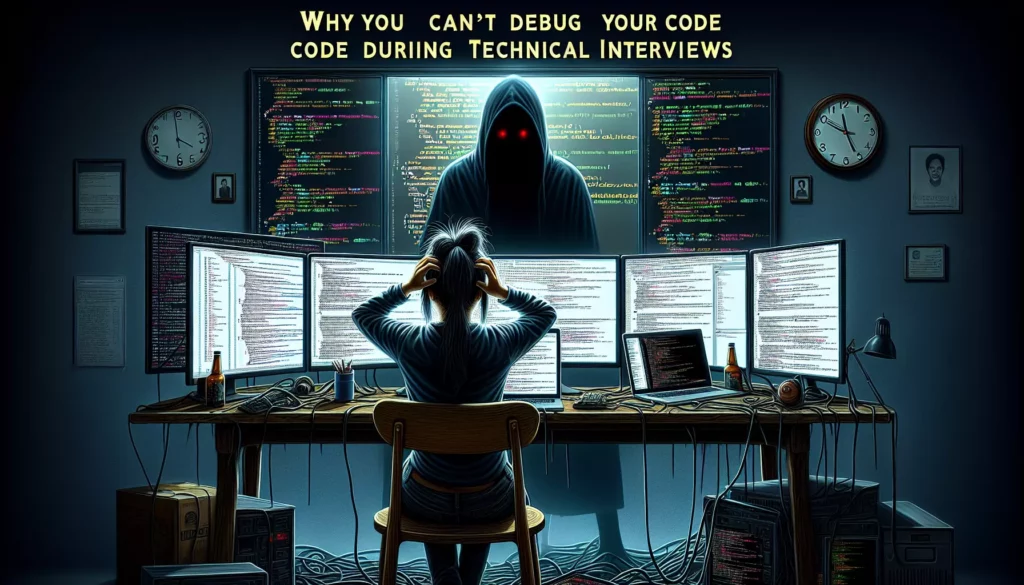
If you’ve ever been through a technical interview, you might have had this frustrating experience: you’re coding on a whiteboard or in a shared editor, you write what you think is a solid solution, but there’s a bug. Instinctively, you reach for the “Run” button or try to add a print statement—only to remember that you can’t actually execute the code.
This situation is incredibly common, and it highlights one of the most challenging aspects of technical interviews: you have to write correct code without the debugging tools you normally rely on.
The Reality of Technical Interviews
Technical interviews, especially at major tech companies like Google, Amazon, Facebook (Meta), Apple, and Netflix (often collectively referred to as FAANG), typically involve coding challenges that must be solved without executing the code. Whether you’re writing on a whiteboard, a shared document, or even a specialized interview platform, the expectation is that you’ll produce a working solution without the ability to run tests or use a debugger.
This approach creates a unique challenge that many developers struggle with. After all, in our day to day work, we constantly rely on debugging tools, print statements, and test runs to verify our code works as expected.
Why Interviews Are Designed This Way
You might be wondering: “Why do companies interview this way? This isn’t how real coding works!” While this is a valid concern, there are several reasons for this approach:
1. Testing Your Understanding of Algorithms and Data Structures
When you can’t rely on trial and error through debugging, you’re forced to think more deeply about the problem. Interviewers want to see that you understand fundamental concepts well enough to implement them correctly on the first try.
2. Evaluating Your Mental Model
Being able to trace through code mentally is an important skill. It demonstrates that you can build accurate mental models of how your code executes, which is crucial for designing complex systems.
3. Assessing Communication Skills
Technical interviews are as much about communication as they are about coding. By explaining your thought process and talking through potential bugs, you demonstrate your ability to collaborate with team members.
4. Simulating Pressure Environments
While not necessarily intentional, the pressure of solving problems without debugging mimics high pressure situations that can occur in real work environments. Companies want to see how you perform under stress.
Common Debugging Challenges in Interviews
Let’s look at some of the specific challenges you might face when you can’t debug during an interview:
Edge Cases Are Hard to Spot
Without running your code, it’s easy to miss edge cases. Consider this seemingly simple function to find the maximum value in an array:
function findMax(arr) {
let max = 0;
for (let i = 0; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
This looks correct at first glance, but what if the array contains only negative numbers? The function would incorrectly return 0. If you could run the code, you’d quickly catch this, but in an interview setting, you need to spot this issue through careful analysis.
Off by One Errors
These errors are notoriously difficult to catch without running code. Consider a binary search implementation:
function binarySearch(arr, target) {
let left = 0;
let right = arr.length;
while (left < right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid;
}
}
return -1;
}
Did you notice the issue? The initial value of right
should be arr.length - 1
, not arr.length
. These off by one errors are easy to make and hard to spot without executing the code.
Logical Errors in Complex Algorithms
When implementing more complex algorithms like graph traversals or dynamic programming solutions, logical errors can be particularly challenging to identify without step by step execution.
Strategies to Succeed Without Debugging
Despite these challenges, there are effective strategies you can use to succeed in technical interviews without relying on debugging:
1. Trace Your Code Manually
The most important skill to develop is the ability to trace through your code manually, line by line, using specific examples. This is essentially what a debugger does, but you’ll be doing it on paper or in your head.
Let’s practice with a simple example. Consider this function that’s supposed to reverse a string:
function reverseString(str) {
let result = "";
for (let i = 0; i < str.length; i++) {
result = str[i] + result;
}
return result;
}
To trace this manually, pick a simple input like “hello” and walk through each step:
- Initialize
result
to an empty string - i = 0:
result = "h" + "" = "h"
- i = 1:
result = "e" + "h" = "eh"
- i = 2:
result = "l" + "eh" = "leh"
- i = 3:
result = "l" + "leh" = "lleh"
- i = 4:
result = "o" + "lleh" = "olleh"
- Return “olleh”
This confirms our function works correctly. By practicing this skill, you’ll get faster at spotting issues without needing to run the code.
2. Test With Multiple Examples
Don’t just test your solution with one example. Consider multiple cases, including:
- A typical case (average sized input with normal values)
- Edge cases (empty arrays, single element arrays, etc.)
- Boundary cases (minimum and maximum values)
- Invalid inputs (if applicable)
For example, if you wrote a function to find the median of an array, you should manually test it with:
- An array with an odd number of elements: [1, 3, 5]
- An array with an even number of elements: [1, 3, 5, 7]
- An empty array: []
- An array with one element: [42]
3. Use Visual Aids
When solving complex problems, especially those involving data structures like trees or graphs, drawing out the structure and how your algorithm traverses it can be incredibly helpful.
For example, if you’re implementing a tree traversal algorithm, draw a small tree and trace through your algorithm step by step, noting the order in which nodes are visited.
4. Implement Helper Functions
Breaking down complex problems into smaller helper functions can make your code easier to reason about and less prone to bugs. It also makes manual tracing more manageable since you can verify each helper function independently.
5. Verbalize Your Thought Process
Talking through your code as you write it forces you to think more carefully about each step. It also helps the interviewer understand your approach and might lead them to give you hints if you’re heading in the wrong direction.
For example, you might say: “I’m initializing the result to an empty string. Then I’ll iterate through each character of the input string. For each character, I’ll add it to the beginning of the result string, which will effectively reverse the order.”
Common Mistakes to Avoid
Even with the best strategies, there are common pitfalls that candidates often fall into during technical interviews:
1. Not Considering Edge Cases
Always consider what happens with empty inputs, single elements, or extremely large inputs. These are common sources of bugs that interviewers specifically look for.
2. Rushing to Write Code
Without the safety net of debugging, rushing to write code is risky. Take time to think through your approach thoroughly before coding. A well thought out solution is less likely to contain bugs.
3. Ignoring Time and Space Complexity
Even if your solution works, it might not be efficient enough. Always analyze and discuss the time and space complexity of your solution, and consider if there are more efficient approaches.
4. Not Testing Your Solution
After writing your code, manually test it with at least one example. This shows the interviewer that you’re careful and thorough, and it gives you a chance to catch any obvious bugs.
5. Being Too Attached to Your First Approach
If you realize your approach has issues, be willing to pivot. It’s better to acknowledge a problem and switch strategies than to continue down a problematic path.
Real Interview Example: Finding a Cycle in a Linked List
Let’s walk through a complete example of how to approach a common interview problem without debugging. The problem is to determine if a linked list contains a cycle.
Step 1: Understand the Problem
First, make sure you understand what constitutes a cycle in a linked list. A cycle exists if, starting from any node, following the next pointers eventually leads back to a previously visited node.
Step 2: Discuss Approaches
Before coding, discuss possible approaches:
“One approach is to use a hash set to keep track of nodes we’ve seen. As we traverse the list, we check if each node is already in our set. If it is, we’ve found a cycle. If we reach the end of the list, there’s no cycle.”
“Another approach is the Floyd’s Tortoise and Hare algorithm, which uses two pointers moving at different speeds. If there’s a cycle, the fast pointer will eventually catch up to the slow pointer.”
Step 3: Choose an Approach and Plan
“I’ll implement the Floyd’s Tortoise and Hare algorithm since it uses O(1) space complexity compared to O(n) for the hash set approach.”
Step 4: Write the Code
function hasCycle(head) {
if (!head || !head.next) {
return false;
}
let slow = head;
let fast = head;
while (fast && fast.next) {
slow = slow.next;
fast = fast.next.next;
if (slow === fast) {
return true;
}
}
return false;
}
Step 5: Trace Through an Example
“Let me trace through this with an example. Consider a linked list: 1 → 2 → 3 → 4 → 2 (pointing back to the second node, creating a cycle).”
Initial state:
- slow = node with value 1
- fast = node with value 1
First iteration:
- slow moves to node with value 2
- fast moves to node with value 3
- slow !== fast, continue
Second iteration:
- slow moves to node with value 3
- fast moves to node with value 2 (after moving through 4)
- slow !== fast, continue
Third iteration:
- slow moves to node with value 4
- fast moves to node with value 4 (after moving through 3)
- slow === fast, return true
“The algorithm correctly identifies the cycle in the linked list.”
Step 6: Consider Edge Cases
“Let me check some edge cases:
- Empty list: The function returns false at the beginning check, which is correct.
- Single node: Also returns false at the beginning, unless the node points to itself.
- No cycle: The fast pointer will reach the end of the list, and the function will return false.
Step 7: Analyze Time and Space Complexity
“The time complexity is O(n) where n is the number of nodes in the linked list. In the worst case, the slow pointer needs to traverse the non cyclic part of the list. The space complexity is O(1) since we only use two pointers regardless of the list size.”
Preparing for No Debug Interviews
Now that you understand the challenges and strategies, how can you prepare effectively for technical interviews where debugging isn’t an option?
1. Practice Manual Tracing
Make it a habit to trace through your code manually before running it. This builds the mental muscle needed for interviews. Start with simple algorithms and gradually increase complexity.
2. Solve Problems on Paper First
Before coding, work through problems on paper or a whiteboard. This mimics the interview environment and forces you to think carefully about your solution.
3. Conduct Mock Interviews
Practice with a friend or use a platform that offers mock interviews. The experience of explaining your code and approach to another person is invaluable.
4. Review Common Algorithms and Data Structures
Make sure you understand fundamental algorithms and data structures well enough to implement them without reference. This includes:
- Sorting algorithms (merge sort, quicksort)
- Search algorithms (binary search, depth first search, breadth first search)
- Data structures (linked lists, trees, graphs, hash tables)
- Dynamic programming approaches
- String manipulation techniques
5. Study Common Patterns and Techniques
Familiarize yourself with common problem solving patterns like:
- Two pointers
- Sliding window
- Binary search
- Backtracking
- Divide and conquer
Recognizing these patterns can help you approach new problems more systematically.
Tools That Can Help
While the goal is to become proficient without debugging tools, there are resources that can help you prepare:
1. Visualization Tools
Websites like VisuAlgo allow you to visualize algorithms and data structures, which can help build better mental models.
2. Interactive Coding Platforms
Platforms like LeetCode, HackerRank, and AlgoCademy offer coding challenges similar to those in technical interviews. While they allow you to run your code, you can practice by first solving problems on paper before testing your solution.
3. Interview Preparation Resources
Books like “Cracking the Coding Interview” provide not just problems but also insights into the interview process and common pitfalls.
4. Code Review Tools
Tools that analyze code quality can help you develop habits that lead to cleaner, less error prone code. This translates to fewer bugs in interview settings.
The Silver Lining: Benefits of No Debug Interviews
While not being able to debug during interviews is challenging, there are some unexpected benefits:
1. It Makes You a Better Programmer
Learning to write correct code without relying on a debugger improves your overall programming skills. You’ll develop more careful coding habits and better attention to detail.
2. It Improves Your Problem Solving
When you can’t rely on trial and error, you’re forced to think more deeply about problems and solutions. This builds stronger analytical skills.
3. It Enhances Communication Skills
The need to explain your approach and code clearly strengthens your technical communication, which is valuable in any development role.
Conclusion
Technical interviews without debugging are a unique challenge that requires specific preparation and skills. By developing your ability to trace code manually, test with multiple examples, use visual aids, and communicate your thought process clearly, you can succeed even without the debugging tools you rely on in your day to day work.
Remember that the goal of these interviews isn’t just to find candidates who can write bug free code on the first try—it’s to identify those who have a deep understanding of algorithms and data structures, can think systematically about problems, and can communicate their thoughts effectively.
While it might seem artificial to code without debugging tools, the skills you develop in preparing for these interviews will make you a stronger programmer overall. So embrace the challenge, practice diligently, and approach your next technical interview with confidence.
And if you do encounter a bug during your interview, don’t panic! Use it as an opportunity to demonstrate your problem solving skills by methodically analyzing your code, identifying the issue, and proposing a fix. Sometimes, how you handle bugs can be just as impressive to interviewers as writing perfect code on the first try.