Why You Can’t Connect Different Programming Concepts (And How to Fix It)
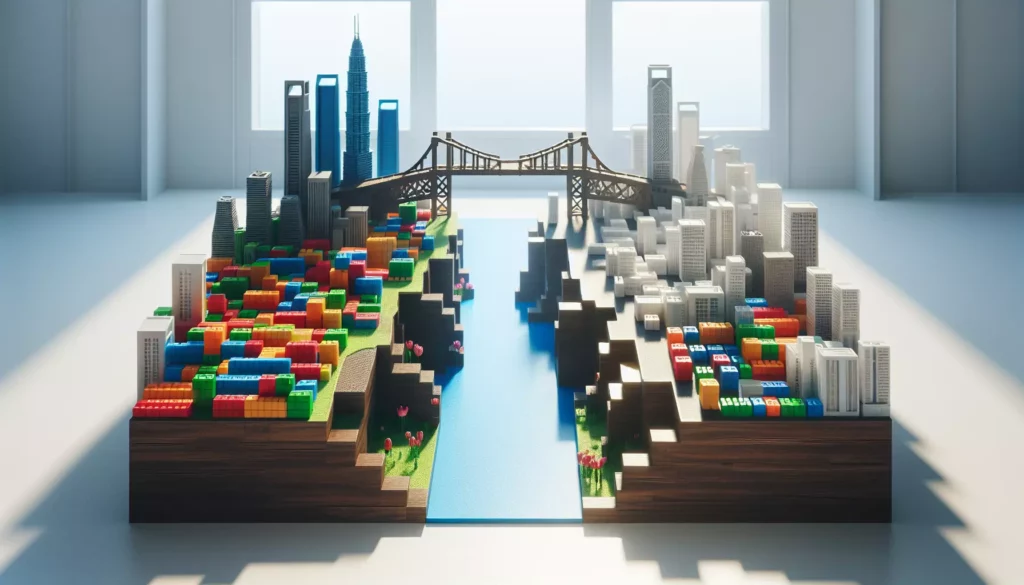
Learning to program is like building a complex puzzle. Each concept is a piece that should connect with others to create a complete picture. Yet many learners struggle to see how variables relate to functions, how data structures support algorithms, or how object-oriented principles apply to real-world problems.
This disconnection between programming concepts isn’t just frustrating—it’s one of the biggest barriers to becoming a proficient developer. When concepts remain isolated in your mind, writing cohesive code becomes nearly impossible, and preparing for technical interviews at companies like Google, Amazon, or Meta feels overwhelming.
In this comprehensive guide, we’ll explore why connecting programming concepts is challenging and provide practical strategies to build a unified understanding that will transform how you code.
The Fragmented Learning Problem
If you’re struggling to connect programming concepts, you’re not alone. Most coding education is inherently fragmented, teaching topics in isolation without emphasizing their relationships. Let’s examine why this happens and how it affects your progress.
Why Traditional Learning Methods Create Disconnection
Traditional programming education often follows a linear, topic-by-topic approach. You might spend a week learning variables, another on loops, and another on functions. This sequential learning creates artificial boundaries between related concepts.
Consider these common learning scenarios:
- You understand arrays and functions separately but struggle to implement a function that processes array data
- You know the syntax for classes but can’t design a coherent object-oriented system
- You’ve memorized sorting algorithms but don’t know when to apply each one in real problems
This fragmentation happens because:
- Tutorial isolation: Most tutorials and courses teach concepts in separate modules without showing their interconnections
- Syntax focus: Excessive emphasis on syntax rather than conceptual understanding
- Lack of context: Learning programming constructs without understanding their purpose in larger systems
- Missing mental models: Not developing frameworks that show how concepts relate to each other
The Impact of Disconnected Knowledge
When programming concepts remain disconnected in your mind, several problems emerge:
- Code inefficiency: Writing unnecessarily complex or redundant code because you can’t see simpler approaches that combine concepts
- Debugging difficulties: Struggling to fix bugs because you don’t understand how different parts of your code interact
- Limited problem-solving: Inability to break down new problems because you can’t identify which concepts apply
- Interview anxiety: Freezing during technical interviews when questions require connecting multiple concepts
- Imposter syndrome: Feeling like you don’t really understand programming despite knowing individual concepts
Alex, a self-taught programmer, shared his experience: “I knew how to write functions, use arrays, and implement basic algorithms. But when I tried building my first real project, everything fell apart. I couldn’t see how these pieces were supposed to work together. It was like knowing all the chess pieces but not understanding the game.”
The Core Connections You’re Missing
Before we explore solutions, let’s identify the essential connections between programming concepts that many learners miss. Understanding these relationships creates the foundation for integrated programming knowledge.
Data Structures and Algorithms
Perhaps the most fundamental connection is between data structures and algorithms. Every algorithm operates on data structured in specific ways, and choosing the right data structure can make an algorithm efficient or inefficient.
For example:
- Binary search requires an ordered array or tree structure
- Graph algorithms need adjacency lists or matrices to represent connections
- Hash-based algorithms depend on hash tables for O(1) lookups
Understanding that algorithms and data structures are two sides of the same coin helps you select the right tools for each problem.
Functions and Data Flow
Functions aren’t just code containers—they define how data flows through your program. The connections between functions create the architecture of your application.
Missing connections include:
- How function parameters and return values create data pipelines
- How function composition enables complex operations from simple components
- How recursion creates elegant solutions by having functions call themselves
Consider this simple example of function composition:
function getUserData(userId) {
const userData = fetchFromDatabase(userId);
const enrichedData = addUserPreferences(userData);
return formatForDisplay(enrichedData);
}
This function connects three operations into a coherent data flow—something that’s difficult to conceptualize if you view functions as isolated units.
Object-Oriented Programming and Real-World Modeling
Many learners memorize OOP principles (encapsulation, inheritance, polymorphism) without understanding their purpose: modeling real-world relationships in code.
The connections often missed include:
- How class hierarchies mirror real-world categorization (e.g., Vehicle → Car → SportsCar)
- How encapsulation relates to information hiding in real systems
- How interfaces define contracts similar to real-world agreements
When you understand these connections, OOP becomes intuitive rather than a set of abstract rules.
Asynchronous Programming and Event Flow
Asynchronous concepts like callbacks, promises, and async/await often remain mysterious because learners don’t connect them to the event-driven nature of modern applications.
Key connections include:
- How asynchronous operations mirror real-world waiting (e.g., waiting for a response)
- How promises represent future values, similar to real-world IOUs
- How event listeners connect user actions to program responses
Language Features and Computational Thinking
Programming languages provide features that express computational thinking patterns. Without this connection, language features seem arbitrary.
Important connections include:
- How loops implement iteration patterns in computational thinking
- How conditionals represent decision trees and branching logic
- How variables correspond to the concept of state in computing
Why Your Brain Resists Connected Learning
Understanding why your brain naturally resists forming these connections can help you overcome these tendencies.
Cognitive Load Theory and Programming
According to cognitive load theory, our working memory has limited capacity. Learning programming involves significant cognitive load because:
- Intrinsic load: Programming concepts are inherently complex
- Extraneous load: Syntax, development environments, and tools add additional complexity
- Germane load: Forming connections between concepts requires mental effort
When your brain is overwhelmed with remembering syntax (extraneous load) and understanding individual concepts (intrinsic load), it has little capacity left for making connections (germane load).
The Curse of Knowledge Compartmentalization
Our brains naturally compartmentalize knowledge for efficient storage and retrieval. This evolutionary adaptation helps us focus on one thing at a time but creates challenges for programming, which requires integrated thinking.
Dr. Barbara Oakley, author of “Learning How to Learn,” explains: “When we first learn something, our brain stores it as an isolated chunk. Connecting these chunks requires deliberate practice and exposure to examples that bridge concepts.”
The Illusion of Understanding
Many learners suffer from what psychologists call the “illusion of understanding”—believing they understand a concept because they recognize it, even if they can’t apply it in new contexts or connect it to other ideas.
For example, you might recognize a binary search algorithm when you see it but fail to recognize when it’s the appropriate solution to a new problem. This illusion prevents you from seeking deeper connections between concepts.
Strategies for Building Connected Programming Knowledge
Now that we understand the problem, let’s explore practical strategies to build a connected understanding of programming concepts.
1. Practice Concept Mapping
Concept mapping is a powerful technique for visualizing relationships between ideas. Create maps that explicitly show how programming concepts connect.
How to implement:
- Draw a central concept (e.g., “Arrays”)
- Branch out to related concepts (e.g., “Loops,” “Memory,” “Algorithms”)
- Add cross-connections between branches with labeled relationships
- Regularly review and expand your maps as you learn new concepts
For example, a concept map for arrays might connect to loops (for iteration), memory (for storage understanding), and algorithms (for operations like searching and sorting).
2. Build Projects That Integrate Multiple Concepts
Projects force you to connect concepts in practical ways. Choose projects that deliberately combine multiple programming areas.
Effective project ideas:
- Data dashboard: Combines data structures, algorithms, asynchronous programming, and UI concepts
- Task management application: Integrates OOP, event handling, state management, and storage
- API-based service: Connects networking, data parsing, error handling, and asynchronous programming
When building projects, explicitly document how different concepts interact in your implementation. This reflection enhances connection-building.
3. Teach Concepts to Others
Teaching forces you to articulate connections between concepts. The Feynman Technique—explaining complex ideas in simple terms—is particularly effective for programming.
How to apply the Feynman Technique:
- Choose a programming concept you want to understand better
- Explain it as if teaching a beginner, using simple language and examples
- Identify gaps in your explanation where connections are missing
- Review and refine your understanding to fill those gaps
You can teach through blog posts, study groups, or even explaining to a rubber duck (a common programming practice where explaining a problem often reveals the solution).
4. Study Code That Connects Concepts
Reading well-written code exposes you to how experts connect programming concepts. Look for codebases that exemplify good integration of multiple programming principles.
Where to find good code examples:
- Open-source projects with good documentation (e.g., React, Vue.js, Flask)
- Books like “Clean Code” by Robert C. Martin that emphasize well-structured code
- Code review platforms like GitHub where you can see discussions about code connections
When studying code, ask questions like:
- Why did the developer choose this data structure for this algorithm?
- How do the functions in this module communicate with each other?
- How does this code handle the flow of data through the system?
5. Use Analogies and Mental Models
Analogies connect programming concepts to familiar real-world situations, making abstract ideas concrete and relatable.
Useful programming analogies:
- Variables as labeled boxes: Variables store values like boxes store items
- Functions as recipes: They take ingredients (parameters), follow steps (statements), and produce a dish (return value)
- Classes as blueprints: They define the structure for creating objects, like blueprints for buildings
- Recursion as nested Russian dolls: Each function call opens a new context within the previous one
Develop your own analogies that make sense to you based on your interests and experiences.
6. Practice Interleaved Learning
Interleaved learning involves mixing different but related concepts during study sessions, rather than focusing on one topic at a time. This approach naturally builds connections between ideas.
How to implement interleaved learning:
- Instead of studying arrays for a week, then algorithms the next week, mix them together
- Practice problems that combine multiple concepts in each study session
- Revisit previously learned concepts when studying new ones, explicitly noting connections
Research shows that interleaved learning improves long-term retention and transfer of knowledge, even though it feels more difficult in the moment.
7. Use Visualization Tools
Visualization tools help you see how code works, making abstract connections concrete. They’re particularly useful for understanding data structures, algorithms, and program flow.
Recommended visualization tools:
- Python Tutor for visualizing code execution step by step
- Algorithm visualizers like VisuAlgo for seeing data structures in action
- Diagramming tools like draw.io for creating system architecture diagrams
Seeing how data moves through your program or how an algorithm traverses a data structure builds intuitive understanding of their connection.
Building a Connected Learning Path
Now let’s apply these strategies to create a coherent learning path that naturally builds connections between programming concepts.
Phase 1: Foundational Connections
Start by connecting the most fundamental programming concepts:
- Variables and Data Types → Control Flow
- Practice: Write programs that use different data types to control program flow
- Project: Create a simple calculator that handles different operations based on input
- Functions → Data Structures
- Practice: Write functions that create, modify, and transform basic data structures
- Project: Build a contact management system using functions and arrays/objects
- Algorithms → Problem Solving
- Practice: Implement basic algorithms like search and sort, focusing on the problem-solving approach
- Project: Create a program that solves a real problem using algorithmic thinking
During this phase, create concept maps that visualize these connections and use analogies to reinforce understanding.
Phase 2: Intermediate Connections
Build on your foundation by connecting more complex concepts:
- Object-Oriented Programming → Real-World Modeling
- Practice: Design classes that model familiar systems (e.g., a library, a store)
- Project: Build a simulation that uses inheritance and polymorphism to model a dynamic system
- Asynchronous Programming → Event-Driven Systems
- Practice: Implement callbacks, promises, and async/await in different scenarios
- Project: Create a web application that handles multiple asynchronous operations
- Data Structures → Algorithm Efficiency
- Practice: Implement the same algorithm using different data structures and compare performance
- Project: Build a program that requires selecting appropriate data structures for efficiency
During this phase, start teaching concepts to others and studying well-written code that demonstrates these connections.
Phase 3: Advanced Integration
At this stage, focus on integrating multiple concepts in complex systems:
- System Architecture → Design Patterns
- Practice: Implement common design patterns and understand their use cases
- Project: Refactor an existing project using appropriate design patterns
- Full-Stack Development → End-to-End Data Flow
- Practice: Build features that require understanding data flow from database to UI
- Project: Create a full-stack application with complex data transformations
- Technical Interview Preparation → Holistic Problem Solving
- Practice: Solve problems that require combining multiple concepts
- Project: Implement a complex algorithm or system from scratch, explaining all connections
During this phase, use visualization tools to understand complex systems and practice interleaved learning to reinforce connections between all the concepts you’ve learned.
Overcoming Common Obstacles to Connected Learning
Even with the right strategies, you may encounter obstacles to building connected programming knowledge. Here’s how to overcome them.
Tutorial Hell and Passive Learning
The problem: Endlessly watching tutorials without applying concepts creates the illusion of understanding without building true connections.
Solution: Implement the “50/50 rule”—spend 50% of your learning time consuming content and 50% actively applying it. After learning a concept, immediately implement it in a way that connects to previously learned concepts.
Overwhelm and Analysis Paralysis
The problem: Trying to connect too many concepts at once leads to cognitive overload and inaction.
Solution: Use the “concept triplet” approach—focus on connecting just three related concepts at a time. Master these connections before moving on to new concept groups.
For example, focus on connecting:
- Arrays + Loops + Functions
- Objects + Methods + Inheritance
- Promises + Async/Await + Error Handling
Forgetting Previously Learned Concepts
The problem: As you learn new programming concepts, earlier ones fade, making connections difficult to maintain.
Solution: Implement spaced repetition with connection focus. Use tools like Anki to create flashcards that explicitly test connections between concepts, not just individual ideas.
Example flashcard questions:
- “How would you use a hash map to optimize this array-based algorithm?”
- “Explain how promises improve upon callbacks for asynchronous operations”
- “How does inheritance support the DRY (Don’t Repeat Yourself) principle?”
Isolation and Lack of Feedback
The problem: Learning alone makes it difficult to validate your understanding of concept connections.
Solution: Join or create a “concept connection” study group where members explain relationships between concepts to each other. Use platforms like:
- Discord or Slack programming communities
- Local meetup groups
- Pair programming sessions focused on code review
How Connected Knowledge Transforms Technical Interviews
Connected programming knowledge is particularly valuable during technical interviews at companies like Google, Amazon, and Meta. Here’s how it transforms your interview performance:
Problem Decomposition Abilities
When you understand how concepts connect, you can break down complex interview problems into familiar patterns. Instead of seeing each problem as entirely new, you recognize components that connect to your existing knowledge.
For example, a question about finding the shortest path in a weighted graph connects to:
- Graph representation (adjacency lists/matrices)
- Shortest path algorithms (Dijkstra’s, Bellman-Ford)
- Priority queues for efficient implementation
- Edge case handling for disconnected graphs
With connected knowledge, you naturally consider all these aspects rather than focusing narrowly on just the algorithm.
Flexible Solution Approaches
Connected knowledge enables you to pivot between different solution approaches when your initial strategy hits a roadblock—a common scenario in interviews.
For instance, if your recursive solution is causing stack overflow issues, connected knowledge helps you seamlessly transform it to an iterative approach using a stack data structure.
Improved Communication Skills
Interviewers evaluate not just your solution but how you explain your thinking. Connected knowledge enables you to articulate relationships between concepts clearly.
Instead of saying, “I’ll use a hash map here,” you can explain, “I’m choosing a hash map because we need O(1) lookups to optimize the inner loop of this algorithm, which would otherwise make this an O(n²) solution.”
Real-World Application Awareness
Top companies want developers who understand how technical solutions apply to business problems. Connected knowledge helps you explain not just how your code works but why it’s appropriate for the specific context.
For example, you might explain why caching (connecting system architecture, memory management, and performance optimization) would be crucial for a particular feature at scale.
The Journey to Connected Programming Mastery
Building connected programming knowledge is not a destination but a continuous journey. Here’s what this journey looks like as you progress:
Beginner: Concept Recognition
At this stage, you recognize individual concepts but see them as separate tools. Your code works but often takes roundabout approaches because you don’t see the connections that would enable more elegant solutions.
Signs you’re at this stage:
- You can write working code by following examples
- You struggle when requirements differ from examples you’ve seen
- Your projects tend to be direct implementations of tutorials
Intermediate: Concept Application
Now you’re beginning to see connections between related concepts and can apply them in familiar contexts. Your code is more efficient because you recognize when to use specific combinations of techniques.
Signs you’re at this stage:
- You can solve problems that require combining 2-3 concepts
- You recognize common patterns in code and can implement them
- Your projects show original thinking but may still lack cohesive architecture
Advanced: Concept Integration
At this level, you see programming as an integrated whole rather than separate concepts. You can fluidly move between different areas of programming and select the optimal approach for each situation.
Signs you’re at this stage:
- You can design complex systems with appropriate architecture
- You intuitively select the right combination of techniques for new problems
- Your projects demonstrate thoughtful integration of multiple programming paradigms
Expert: Concept Innovation
At the expert level, you not only connect existing concepts but create new connections, leading to innovative approaches. You understand the underlying principles so well that you can adapt and extend them to novel situations.
Signs you’re at this stage:
- You develop new patterns that elegantly solve complex problems
- You can teach others how to build connected programming knowledge
- Your work influences how others approach programming challenges
Conclusion: From Fragmentation to Fluency
The inability to connect programming concepts is not a personal failing but a natural consequence of how programming is typically taught and how our brains process information. By understanding these challenges and implementing the strategies outlined in this guide, you can transform fragmented knowledge into connected understanding.
Remember that building these connections takes time and deliberate practice. Be patient with yourself and celebrate small victories when you recognize a new connection between concepts.
As your understanding becomes more integrated, you’ll experience several transformations:
- Writing code will feel more like a creative expression than a mechanical process
- Problem-solving will become more intuitive as you naturally draw on interconnected knowledge
- Learning new concepts will be easier as you have a framework to connect them to existing understanding
- Technical interviews will become opportunities to demonstrate your holistic understanding rather than stress-inducing tests
The journey from fragmented knowledge to connected mastery is what separates casual coders from professional developers. By committing to this journey, you’re not just learning to code—you’re learning to think like a programmer.
Start today by selecting one connection between concepts you’ve struggled with and applying the strategies in this guide. Share your experience with others and continue building your connected programming knowledge one relationship at a time.
Your future as a developer depends not just on what you know, but on how all that knowledge connects in your mind.