Why You Can’t Break Down Problems in Front of Interviewers: A Comprehensive Guide
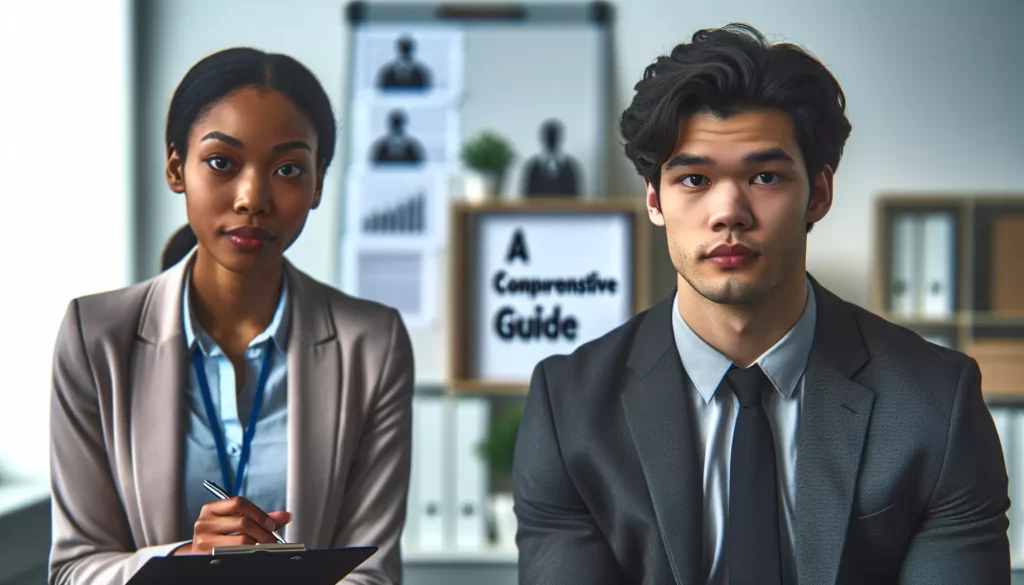
Have you ever found yourself freezing up during a technical interview? That moment when the interviewer presents a coding problem, and despite all your preparation, your mind goes blank? You’re not alone. This phenomenon is incredibly common, even among experienced developers, and understanding why it happens is the first step to overcoming it.
In this comprehensive guide, we’ll explore the psychological, cognitive, and practical reasons why breaking down problems during interviews can be challenging. More importantly, we’ll provide actionable strategies to help you overcome these obstacles and showcase your true problem solving abilities when it matters most.
Table of Contents
- Understanding the Problem: Why We Freeze Up
- Cognitive Overload During Interviews
- The Fear Factor: Performance Anxiety
- Lack of Structured Problem Solving Practice
- Unrealistic Expectations
- Effective Strategies for Breaking Down Problems
- A Framework for Problem Solving
- The Art of Thinking Aloud
- Practice Techniques That Actually Work
- Case Studies: Before and After
- The Interviewer’s Perspective
- Conclusion: From Freezing to Flowing
Understanding the Problem: Why We Freeze Up
To solve any problem, we must first understand it. The same applies to the meta-problem of why we struggle to break down coding challenges during interviews.
Technical interviews create a unique environment where multiple factors converge to make problem solving particularly difficult:
- Time pressure (typically 45-60 minutes per problem)
- Being observed and evaluated in real time
- The high stakes nature of job interviews
- Unfamiliar problems requiring on the spot analysis
- The need to communicate your thought process while solving
This combination creates what psychologists call “evaluation apprehension” — the anxiety that comes from knowing your performance is being judged. This anxiety triggers our brain’s threat response system, which can literally impair cognitive function.
Research in cognitive psychology shows that working memory — the mental workspace we use for problem solving — becomes significantly compromised under stress. When our brain detects a threat (in this case, the possibility of failure or judgment), it redirects resources to more primitive functions associated with the fight-or-flight response.
Cognitive Overload During Interviews
During a typical coding interview, your brain is juggling multiple cognitive tasks simultaneously:
- Understanding the problem statement
- Recalling relevant algorithms and data structures
- Developing a solution strategy
- Translating that strategy into code
- Analyzing time and space complexity
- Communicating your thought process clearly
- Managing your anxiety and self-presentation
Each of these tasks consumes cognitive resources, and when combined, they can easily exceed our working memory capacity. This is what cognitive scientists call “cognitive load,” and when it exceeds our capacity, our problem solving abilities deteriorate dramatically.
Consider this real interview scenario: You’re asked to solve a graph traversal problem. You need to:
- Recall how BFS and DFS work
- Determine which approach is more suitable
- Code the solution without reference materials
- Explain your reasoning to the interviewer
- Handle edge cases
- Analyze the time complexity
All while managing your nervousness and the ticking clock. It’s no wonder even experienced developers can struggle in this situation!
The Fear Factor: Performance Anxiety
Performance anxiety in technical interviews goes beyond general nervousness. It’s a specific form of anxiety that directly impacts cognitive function. This anxiety manifests in several ways:
Impostor Syndrome
Many developers, even highly skilled ones, suffer from impostor syndrome — the feeling that they’re not as competent as others perceive them to be. This can be particularly acute during interviews when you’re being explicitly evaluated.
Thoughts like “I should know this” or “A real developer would solve this easily” create additional cognitive load that distracts from the actual problem solving task.
Fear of Negative Judgment
The fear of being negatively judged by the interviewer can lead to what psychologists call “cognitive distraction.” Instead of focusing fully on the problem, part of your mental resources are dedicated to worrying about how you’re being perceived.
This self-consciousness creates a meta-cognitive loop where you’re thinking about your thinking, which further reduces available cognitive resources for actual problem solving.
The Spotlight Effect
Psychologists have identified what they call the “spotlight effect” — our tendency to overestimate how much others notice our mistakes or flaws. During an interview, this effect is amplified, making us hyper-aware of every hesitation or misstep.
This heightened self-awareness can create a debilitating feedback loop: you become nervous about appearing nervous, which makes you more nervous, and so on.
Lack of Structured Problem Solving Practice
Many developers prepare for technical interviews by solving coding challenges on platforms like LeetCode, HackerRank, or CodeSignal. While this practice is valuable, it often lacks a crucial element: the structured approach to breaking down problems.
When practicing alone, developers often:
- Jump straight to coding without fully analyzing the problem
- Skip the step of verbalizing their thought process
- Rely on pattern recognition rather than methodical problem decomposition
- Focus on getting the correct answer rather than the approach
This creates a significant gap between practice and the actual interview experience, where methodical problem breakdown and clear communication are just as important as arriving at the correct solution.
Additionally, when practicing alone, there’s no external pressure or observation, meaning you’re not building the mental resilience needed for high-pressure situations.
Unrealistic Expectations
Many candidates enter interviews with unrealistic expectations that further compound the difficulty:
The Expectation of Immediate Insight
There’s a common misconception that skilled developers should have immediate insights into problem solutions. This expectation creates additional pressure when you don’t see the solution right away.
In reality, professional problem solving is often messy, iterative, and involves false starts. Experienced developers know this, but interview candidates often hold themselves to an unrealistic standard of immediate comprehension.
The Perfectionism Trap
Many developers fall into the trap of perfectionism during interviews, believing they need to produce a flawless, optimized solution on the first attempt. This perfectionism can be paralyzing, preventing you from starting with a simple approach and iteratively improving it.
The expectation that you should arrive at the optimal solution immediately adds unnecessary pressure that impedes the natural problem solving process.
Misunderstanding the Evaluation Criteria
Candidates often believe they’re being evaluated solely on whether they solve the problem correctly and optimally. This misunderstanding leads to anxiety about the solution rather than focusing on demonstrating a sound problem solving process.
In reality, interviewers are often more interested in how you approach problems, communicate your thinking, and respond to hints than whether you immediately arrive at the optimal solution.
Effective Strategies for Breaking Down Problems
Now that we understand the challenges, let’s explore proven strategies to overcome them. These approaches can help you systematically break down problems even under the pressure of an interview situation.
Develop a Consistent Methodology
Having a consistent, practiced methodology for approaching problems provides a safety net when anxiety strikes. It gives you a clear path to follow even when you’re feeling overwhelmed.
A basic problem solving framework might include:
- Understand: Clarify the problem and requirements
- Plan: Formulate a high-level approach before coding
- Execute: Implement your solution step by step
- Verify: Test your solution with examples and edge cases
By internalizing this framework through practice, you create a reliable process that can carry you through even when you’re feeling anxious or uncertain.
The Power of External Representation
One of the most effective ways to reduce cognitive load is to use external representations of the problem. This means getting the problem out of your head and onto the whiteboard or paper.
Research in cognitive science shows that external representations significantly expand our problem solving capabilities by offloading memory requirements and making relationships more visible.
Practical approaches include:
- Diagrams: Visualize data structures or algorithm flow
- Tables: Track variable states or algorithm progress
- Pseudocode: Outline your approach before coding
- Examples: Work through concrete instances of the problem
These external aids not only help you think more clearly but also communicate your thought process to the interviewer.
Start with Brute Force
Many candidates freeze because they’re searching for the optimal solution immediately. A more effective approach is to start with a brute force solution, even if it’s inefficient.
Benefits of this approach include:
- It gets you moving instead of being paralyzed
- It demonstrates to the interviewer that you can at least solve the problem
- It often reveals insights that lead to optimizations
- It provides a baseline for discussing time and space complexity
Starting with “I’ll first consider a simple approach…” relieves the pressure of finding the perfect solution immediately and creates a foundation for improvement.
A Framework for Problem Solving
Let’s explore a more detailed framework that you can apply to virtually any coding interview problem:
1. Clarify the Problem (2-3 minutes)
Before diving into solutions, ensure you fully understand what’s being asked:
- Restate the problem in your own words
- Ask about input constraints and edge cases
- Clarify expected outputs
- Determine if there are performance requirements
Example dialogue: “So, if I understand correctly, I need to design an algorithm that finds the kth largest element in an unsorted array. Before I start, I’d like to clarify a few things: Can the array contain duplicates? What should I return if k is larger than the array size?”
2. Work Through Examples (3-5 minutes)
Examples serve multiple purposes: they verify your understanding, help you identify patterns, and provide test cases for your solution.
- Start with the example provided (if any)
- Create your own examples, including edge cases
- Trace through these examples manually
Verbalize your process: “Let me work through a simple example. If we have the array [3, 1, 5, 2, 4] and k=2, we’d first sort it to [1, 2, 3, 4, 5], and then the 2nd largest element would be 4.”
3. Outline a High-Level Approach (3-5 minutes)
Before writing any code, describe your approach at a high level:
- Identify the key algorithms or data structures you’ll use
- Break the problem into smaller sub-problems
- Consider multiple approaches if possible
Example: “I can think of a few approaches to this problem. The simplest would be to sort the array and return the kth element from the end, which would be O(n log n). Another approach would be to use a min-heap of size k, which would give us O(n log k) time complexity. Let me explore both options…”
4. Analyze Complexity Before Coding (2-3 minutes)
Before diving into code, analyze the time and space complexity of your approach:
- Identify the dominant operations
- Calculate best, average, and worst-case scenarios
- Consider if optimizations are needed
This demonstrates algorithmic thinking and may save you from implementing an approach that doesn’t meet requirements.
5. Code the Solution (10-15 minutes)
Now that you have a clear plan, implement your solution:
- Write clean, readable code
- Use meaningful variable names
- Comment on non-obvious parts
- Explain key steps as you code
Example: “I’ll implement the heap-based approach. First, I’ll create a min-heap and populate it with the first k elements…”
6. Test Your Solution (5-7 minutes)
Once your code is written, test it thoroughly:
- Trace through your examples step by step
- Check edge cases explicitly
- Look for off-by-one errors or boundary conditions
- Verify that your solution handles all requirements
Example: “Let me trace through our example: [3, 1, 5, 2, 4] with k=2. First, we build a min-heap with [3, 1, 5]…”
7. Optimize If Needed (3-5 minutes)
If time permits, discuss potential optimizations:
- Identify bottlenecks in your current solution
- Consider alternative algorithms or data structures
- Discuss trade-offs between different approaches
This demonstrates depth of knowledge and the ability to think beyond the initial solution.
The Art of Thinking Aloud
One of the most challenging aspects of technical interviews is the expectation to verbalize your thought process. This “thinking aloud” doesn’t come naturally to most developers, who are accustomed to solving problems silently.
Why Verbalization is Crucial
From the interviewer’s perspective, your verbalization provides a window into your thought process. It helps them understand:
- How you approach problems
- Your reasoning behind specific decisions
- Your ability to communicate technical concepts
- How you might collaborate with team members
Without this verbalization, the interviewer can only judge the final result, not the journey that led there.
Structured Verbalization Techniques
Effective verbalization follows a structure that makes your thinking clear and accessible:
- Signal transitions: “Now that I understand the problem, I’ll consider some approaches…”
- Explain reasoning: “I’m choosing a hash map here because we need O(1) lookup time…”
- Acknowledge trade-offs: “This approach uses more memory but gives us better time complexity…”
- Think meta: “Let me step back and consider if there’s a pattern I’m missing…”
These verbal signposts help the interviewer follow your reasoning and demonstrate your metacognitive awareness.
Managing Silence
There will inevitably be moments when you need to think silently. This is perfectly acceptable, but needs to be managed properly:
- Signal when you need thinking time: “I’d like to take a moment to think about this…”
- Provide updates during longer silences: “I’m considering whether we could use dynamic programming here…”
- Return to verbalization once you have direction: “Okay, I think I see a way forward…”
These techniques transform potentially awkward silences into productive thinking time that the interviewer can understand and respect.
Practice Techniques That Actually Work
To overcome the challenges of problem breakdown during interviews, you need deliberate practice that simulates the interview environment as closely as possible.
Mock Interviews with Feedback
The most effective practice involves realistic mock interviews with feedback:
- Find a peer, mentor, or professional service for mock interviews
- Request specific feedback on your problem breakdown approach
- Record sessions to review your communication patterns
- Gradually increase difficulty to build confidence
This type of practice helps normalize the experience of solving problems under observation, reducing anxiety during actual interviews.
The Rubber Duck Technique
When practice partners aren’t available, the “rubber duck debugging” technique can be adapted for interview preparation:
- Set up a recording device or simply place a rubber duck (or any object) on your desk
- Verbalize your entire problem solving process as if explaining to the duck
- Force yourself to articulate each step of your thinking
- Review recordings to identify areas for improvement
This technique helps develop the habit of verbalization and can reveal gaps in your reasoning that might not be apparent when solving problems silently.
Timeboxed Problem Solving
To simulate the time pressure of interviews, practice with strict time constraints:
- Set a timer for 30-45 minutes per problem
- Allocate specific time blocks for each phase of your framework
- Practice recovering when you get stuck
- Analyze how your performance changes under time pressure
This practice builds time awareness and helps you develop strategies for managing pressure during actual interviews.
Deliberate Practice of Problem Types
Not all problem solving skills transfer equally. Practice should focus on developing general problem decomposition skills:
- Practice categorizing problems by underlying patterns
- Focus on problems that require multiple approaches
- Deliberately practice problems that initially confuse you
- Review and refactor your solutions after solving
This type of practice builds the mental flexibility needed to tackle novel problems during interviews.
Case Studies: Before and After
To illustrate the impact of effective problem breakdown, let’s examine two approaches to the same interview problem:
The Problem: Word Break
Given a string s and a dictionary of strings wordDict, determine if s can be segmented into a space-separated sequence of one or more dictionary words.
Ineffective Approach (Before)
Candidate: [Reads problem] Okay, so I need to check if the string can be split into words from the dictionary.
[Long silence as candidate thinks]
Candidate: I think I can use recursion here. Let me try coding...
[Starts writing code immediately without explanation]
def wordBreak(s, wordDict):
if not s:
return True
for i in range(1, len(s) + 1):
if s[:i] in wordDict and wordBreak(s[i:], wordDict):
return True
return False
[Tests on a simple example]
Candidate: Wait, this might not be efficient enough. I think there's a lot of repeated work...
[Gets stuck trying to optimize without clear direction]
Effective Approach (After)
Candidate: Let me make sure I understand the problem correctly. I have a string s and a dictionary of words. I need to determine if s can be broken down into a sequence of words from the dictionary. Is that correct?
Interviewer: Yes, that's right.
Candidate: Let me work through an example to clarify. If s = "leetcode" and wordDict = ["leet", "code"], then the answer would be true because "leetcode" can be broken into "leet" + "code". If s = "catsandog" and wordDict = ["cats", "dog", "sand", "and", "cat"], then the answer would be false because we can't form the complete string using the dictionary words.
Candidate: Before diving into code, let me think about approaches. The naive approach would be to try all possible ways to split the string and check if each part is in the dictionary. This is essentially a search problem.
Candidate: [Draws a decision tree on the whiteboard] At each position, I have two choices: either the substring from the start to the current position is a valid word, in which case I continue from the next position, or it's not, in which case I need to extend the substring.
Candidate: This suggests a recursive approach, but I'm concerned about efficiency. If I naively implement recursion, I might end up recalculating the same subproblems multiple times.
Candidate: Let me think... This sounds like a good candidate for dynamic programming. I can define DP[i] as whether the substring s[0:i] can be segmented into dictionary words. Then DP[i] is true if there exists a j < i such that DP[j] is true and s[j:i] is in the dictionary.
[Proceeds to implement the DP solution with clear explanation of each step]
The key differences in the effective approach include:
- Clarifying the problem with examples
- Thinking about the problem structure before coding
- Identifying potential inefficiencies proactively
- Using external representations (the decision tree)
- Recognizing the DP pattern and explaining the recurrence relation
- Maintaining clear communication throughout
The Interviewer’s Perspective
Understanding what interviewers are actually looking for can help alleviate anxiety and focus your efforts more effectively.
What Interviewers Actually Evaluate
Contrary to popular belief, most interviewers aren’t primarily interested in whether you immediately know the optimal solution. They’re evaluating:
- Problem solving approach: Do you have a systematic method for tackling problems?
- Communication skills: Can you clearly articulate your thinking?
- Technical fundamentals: Do you understand core CS concepts?
- Adaptability: Can you incorporate feedback and hints?
- Code quality: Do you write clean, maintainable code?
A candidate who methodically works through a problem, communicating clearly even if they don’t reach the optimal solution, often rates higher than someone who jumps to the answer without explanation.
Red Flags vs. Green Flags
From an interviewer’s perspective, these behaviors send strong signals:
Red Flags:
- Diving into code without understanding the problem
- Long silences without explanation
- Resistance to hints or feedback
- Giving up when facing obstacles
- Making assumptions without verification
Green Flags:
- Clarifying the problem before solving
- Discussing multiple approaches and trade-offs
- Communicating clearly about challenges
- Testing code thoroughly
- Building on interviewer hints constructively
By focusing on displaying these positive behaviors, you can make a strong impression even when facing difficult problems.
The Hidden Evaluation Criteria
Beyond technical skills, interviewers are often assessing factors they may not explicitly mention:
- Collaboration potential: Would other team members enjoy working with you?
- Learning agility: How quickly can you incorporate new information?
- Grace under pressure: How do you handle stress and uncertainty?
- Intellectual curiosity: Do you show interest in understanding problems deeply?
These “soft skills” often become tiebreakers between technically competent candidates.
Conclusion: From Freezing to Flowing
The ability to break down problems effectively during interviews is not an innate talent but a learnable skill. By understanding the psychological and cognitive challenges involved, developing a structured framework, and practicing deliberately, you can transform from freezing under pressure to flowing through problems methodically.
Remember these key takeaways:
- Preparation is process-oriented: Focus on internalizing a problem solving framework rather than memorizing solutions
- Communication is crucial: Practice verbalizing your thought process until it becomes second nature
- External representation reduces cognitive load: Use diagrams, examples, and pseudocode to extend your working memory
- Incremental progress beats perfectionism: Start with brute force and improve rather than freezing in search of the perfect solution
- Interviewers evaluate process over perfection: Demonstrating a sound approach often matters more than reaching the optimal solution
By applying these principles, you can overcome the common challenge of freezing during technical interviews and demonstrate your true problem solving abilities when it matters most.
The next time you face a challenging interview problem, remember that the goal isn’t to appear brilliant by immediately seeing the solution. The goal is to demonstrate that you have a reliable process for methodically breaking down and solving complex problems — a skill that’s far more valuable in real-world software development than the ability to produce instant solutions.
With practice and the right mindset, you can transform the interview from an anxiety-inducing test into an opportunity to showcase your structured thinking and problem solving capabilities.