Why You Can’t Break Big Problems Into Smaller Ones: The Myth of Problem Decomposition
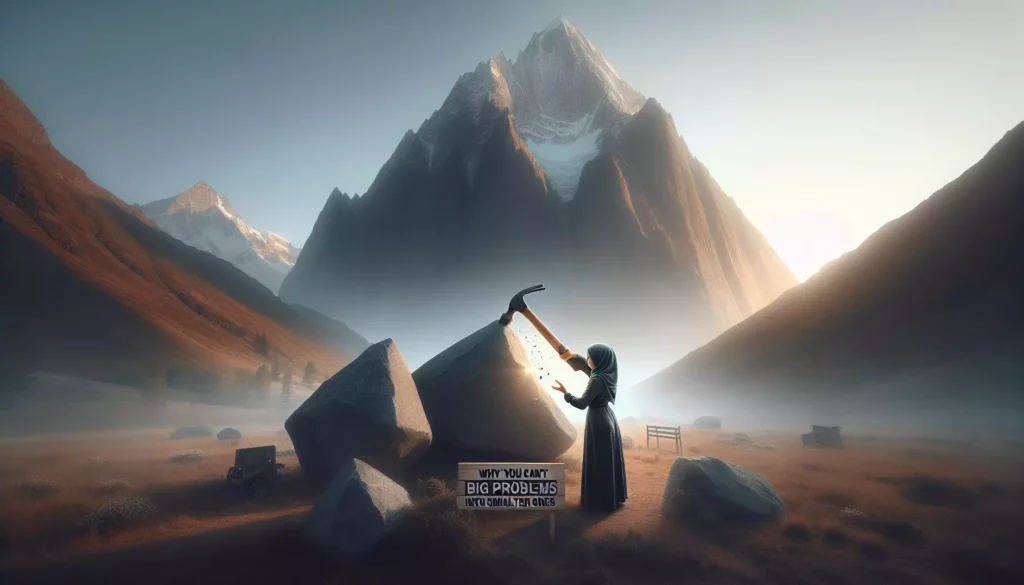
In the world of programming and algorithm design, a piece of advice you’ll frequently encounter is: “Break big problems into smaller ones.” This approach, often called decomposition, is presented as a universal solution for tackling complex challenges. But what if I told you that this approach doesn’t always work? What if some problems simply resist our attempts to neatly divide them into manageable chunks?
Today, we’re going to explore why the popular “divide and conquer” mentality has limitations, when it falls short, and what alternative approaches might serve you better when facing truly complex programming challenges.
The Conventional Wisdom on Problem Decomposition
Before we challenge this widely accepted practice, let’s understand what problem decomposition actually means in programming.
Problem decomposition is the process of breaking down a large, complex problem into smaller, more manageable subproblems. The idea is that these smaller components are easier to understand, solve, and test individually. Once solved, they can be recombined to address the original problem.
For example, if you’re building a social media application, you might break it down into user authentication, feed generation, post creation, and notification systems. Each of these components can be further divided into smaller tasks, creating a hierarchy of increasingly specific problems to solve.
This approach has been the backbone of software engineering methodologies for decades, and for good reason. It has enabled teams to divide work efficiently, create modular code, and manage complexity in many scenarios.
When Decomposition Fails: The Indivisible Problem
Despite its popularity and usefulness in many contexts, problem decomposition isn’t a universal solution. Some problems are fundamentally indivisible or lose their essential characteristics when broken apart.
Emergent Complexity
Some systems exhibit what’s called “emergent behavior”—properties that arise from the interactions between components rather than from the components themselves. In such cases, breaking the problem down means losing sight of the very interactions that define the system.
Consider natural language processing. Understanding individual words and grammar rules is relatively straightforward, but comprehending the meaning of a sentence requires understanding how words interact in context. The meaning emerges from the relationships between words, not just from the words themselves.
Similarly, in machine learning, the effectiveness of a model often emerges from the complex interplay of various components, hyperparameters, and the specific characteristics of the data. You can’t optimize each aspect in isolation and expect the whole system to work optimally.
The Interdependence Challenge
Some problems involve components that are so tightly coupled that separating them creates artificial boundaries that hinder rather than help the solution process.
For instance, in designing a real-time multiplayer game, you can’t completely separate the networking code from the game state management. Decisions in one area profoundly affect the other, and optimizing them separately might lead to incompatible approaches.
Another example is database schema design. While you can theoretically separate different aspects of your data model, in practice, decisions about normalization, indexing, and query patterns are deeply interconnected. Optimizing one aspect without considering the others can lead to serious performance issues.
The NP-Hard Reality
In computational complexity theory, there exists a class of problems known as NP-hard problems. These problems are notoriously difficult because they don’t lend themselves to efficient divide-and-conquer approaches.
The traveling salesman problem (TSP) is a classic example. The goal is to find the shortest possible route that visits each city exactly once and returns to the origin city. You might think you could break this down by solving optimal paths between pairs of cities, but that approach doesn’t work. The optimal solution depends on considering the entire set of cities and their relationships simultaneously.
Other examples include graph coloring, the knapsack problem, and many optimization problems that appear in scheduling, routing, and resource allocation contexts. These problems often require holistic approaches rather than decomposition.
The Hidden Costs of Forced Decomposition
When we attempt to decompose problems that resist decomposition, we often introduce new challenges and inefficiencies.
Integration Complexity
Breaking a problem into parts means those parts will eventually need to be integrated. When the subproblems aren’t naturally separable, this integration phase can become more complex than the original problem.
For example, in building a recommendation system, you might separately optimize for accuracy, diversity, and novelty of recommendations. However, when you try to combine these optimizations, you may find they work at cross-purposes, requiring complex trade-offs that weren’t apparent when working on each dimension separately.
Lost Optimization Opportunities
Some problems have global optimization characteristics that are lost when broken down. The optimal solution to the whole problem may be very different from combining optimal solutions to subproblems.
Consider compiler optimization. If you separately optimize different parts of the compilation process (parsing, type checking, code generation), you might miss opportunities for cross-stage optimizations that could significantly improve performance.
Increased Cognitive Load
Counterintuitively, decomposition can sometimes increase cognitive load rather than reduce it. This happens when the mental effort required to track relationships between subproblems exceeds the complexity of thinking about the problem holistically.
In designing a complex user interface, breaking down the problem into separate components like layout, styling, and interaction can sometimes make it harder to ensure a cohesive, intuitive user experience. The designer must constantly switch contexts and mentally reassemble the pieces to evaluate the overall effect.
Real-World Examples of Indivisible Problems
Let’s look at some concrete programming scenarios where decomposition approaches often fall short.
Concurrent Systems Design
Designing systems with significant concurrency requirements often resists clean decomposition. Issues like race conditions, deadlocks, and livelocks emerge from the interactions between components rather than from the components themselves.
For example, consider implementing a lock-free data structure like a concurrent hash map. You can’t simply break this down into “implement hash map” and “add concurrency” as separate tasks. The concurrency considerations fundamentally change how the data structure needs to be designed from the ground up.
Distributed Systems
Distributed systems present unique challenges that often can’t be decomposed. Problems like consistency, partition tolerance, and failure detection are system-wide concerns that cut across component boundaries.
The CAP theorem (Consistency, Availability, Partition tolerance) illustrates this well. You can’t optimize for all three properties simultaneously, and decisions about trade-offs need to be made at the system level, not at the component level.
Genetic Algorithms and Evolutionary Computing
When working with genetic algorithms or other evolutionary approaches, the fitness function and selection mechanisms often need to consider the solution as a whole. Breaking the problem down might destroy the very patterns that evolutionary processes need to discover.
For instance, in designing a neural network architecture using evolutionary algorithms, you can’t separately evolve different parts of the network and then combine them. The fitness of any particular structure depends on how all parts work together.
Cryptographic Systems
Security in cryptographic systems is a holistic property. A system is only as secure as its weakest link, which means security considerations can’t be fully decomposed into separate concerns.
You might design a perfect encryption algorithm, but if the key management or the implementation has flaws, the entire system becomes vulnerable. Security requires thinking about the system as a whole, including how components interact and what attack vectors might emerge from those interactions.
Alternative Approaches for Complex Problems
If traditional decomposition isn’t always the answer, what alternatives do we have for tackling complex problems? Here are some approaches that often work better for indivisible challenges:
Holistic Design Thinking
Instead of breaking the problem down immediately, spend more time understanding it as a whole. This might involve:
- Creating comprehensive mental models of the problem space
- Identifying core principles and constraints that apply across the entire problem
- Developing a high-level architecture that acknowledges interdependencies
For example, when designing a new programming language, you might start by defining its core philosophy and principles rather than immediately breaking it down into lexer, parser, and runtime components.
Iterative Refinement
Rather than breaking a problem into parallel components, consider approaching it through successive refinement:
- Start with a simplified but complete version of the solution
- Gradually add complexity while maintaining a working system
- Continuously refactor to keep the design coherent
This approach is particularly useful for user interfaces and API design, where the cohesiveness of the final product is critical.
Simulation and Modeling
For problems with emergent properties, simulation can be more effective than decomposition:
- Build simplified models of the entire system
- Run simulations to observe how different components interact
- Use insights from simulations to guide your design decisions
This approach is commonly used in game development, where gameplay mechanics often can’t be evaluated in isolation.
Pattern Recognition
Some problems are better approached by recognizing patterns rather than breaking them down:
- Study similar problems and their solutions
- Look for recurring patterns and principles
- Adapt existing patterns to your specific context
For instance, when designing distributed systems, understanding patterns like CQRS (Command Query Responsibility Segregation) or Event Sourcing can provide more guidance than trying to decompose the problem from first principles.
Constraint-Based Thinking
Instead of breaking down the problem, focus on identifying and managing constraints:
- Enumerate all the constraints the solution must satisfy
- Explore the solution space defined by these constraints
- Look for ways to relax or redefine constraints when necessary
This approach works well for optimization problems like scheduling or resource allocation, where the challenge often lies in finding solutions that satisfy multiple competing constraints.
Finding Balance: When to Decompose and When Not To
The key insight isn’t that decomposition is wrong, but that it’s not universally applicable. Skilled problem solvers need to recognize when to decompose and when to use alternative approaches.
Signs That Decomposition Might Not Be Ideal
Consider alternative approaches when you observe these warning signs:
- Subproblems have significant overlap or circular dependencies
- Integration of subproblems seems more complex than the original problem
- The problem involves emergent properties or system-wide optimization
- You’re dealing with known NP-hard problems or complex trade-offs
- Previous attempts to solve similar problems through decomposition have failed
A Hybrid Approach
In practice, many complex problems benefit from a hybrid approach:
- Start with holistic understanding and high-level design
- Decompose only where natural boundaries exist
- Maintain awareness of cross-cutting concerns and interdependencies
- Use iterative development to refine the solution as a whole
For example, when building a machine learning system, you might decompose the data pipeline and model training infrastructure, but approach the model architecture and feature engineering more holistically.
Case Study: Building a Chess Engine
Let’s explore a concrete example: building a chess engine. This is a complex problem that illustrates the limitations of pure decomposition.
A naive decomposition might break this down into:
- Board representation
- Move generation
- Position evaluation
- Search algorithm
While this division seems logical, it misses crucial interdependencies:
The efficiency of your search algorithm depends heavily on your board representation. For instance, using bitboards allows for faster move generation, which affects how deep your search can go.
Your evaluation function needs to consider the same factors your search algorithm prioritizes. If they’re optimized separately, the engine might make suboptimal moves.
Time management (deciding how long to think on each move) interacts with search depth, evaluation accuracy, and position complexity. It can’t be separated from these concerns.
A more effective approach might be:
- Start with a minimal but complete engine that plays legal chess
- Iteratively improve the engine while measuring overall playing strength
- Make design decisions based on how they affect the complete system
- Use patterns from successful chess engines while understanding why they work
This approach acknowledges the holistic nature of chess engine design while still providing a path forward through incremental improvement.
Programming Paradigms and Indivisible Problems
Different programming paradigms offer varying tools for handling problems that resist decomposition.
Functional Programming
Functional programming often handles certain types of complexity well through:
- Higher-order functions that allow for powerful abstractions
- Immutability, which can simplify reasoning about system state
- Monads and other patterns for managing effects and context
For problems involving complex transformations or where state management is challenging, functional approaches can provide elegant solutions without requiring traditional decomposition.
Object-Oriented Programming
Object-oriented programming offers different tools:
- Encapsulation to manage complexity within cohesive units
- Inheritance and composition for code reuse and abstraction
- Design patterns that address common integration challenges
OOP can be particularly effective when the problem domain has natural entities with clear responsibilities, even if those entities have complex interactions.
Reactive Programming
For problems involving complex event flows and asynchronous behavior:
- Data flow modeling instead of control flow
- Event streams and operators for managing complex interactions
- Declarative specification of relationships between events
Reactive approaches often handle certain types of indivisible problems better by focusing on the relationships between events rather than trying to decompose the system into separate components.
Learning to Recognize and Handle Indivisible Problems
Developing the ability to recognize and effectively approach indivisible problems is an advanced skill that comes with experience. Here are some ways to cultivate this skill:
Study System Thinking
System thinking focuses on understanding how parts of a system interact and how systems interact with their environments. Resources on system dynamics, complexity theory, and emergent behavior can provide valuable mental models for approaching indivisible problems.
Analyze Failed Projects
When you encounter projects that struggled despite seemingly good decomposition, analyze what went wrong. Were there hidden interdependencies? Did integration challenges overwhelm the benefits of modularization? Learning from failures can help you recognize similar patterns in future projects.
Practice Holistic Problem Solving
Challenge yourself with problems known to resist decomposition, such as:
- Designing concurrent data structures
- Optimizing complex algorithms with multiple competing objectives
- Creating robust distributed systems
- Developing AI systems with emergent behavior
Through practice, you’ll develop intuition about when decomposition is helpful and when it might hinder progress.
Learn Multiple Paradigms
Different programming paradigms provide different tools for managing complexity. By becoming proficient in multiple paradigms (functional, object-oriented, reactive, etc.), you’ll have more options when facing problems that don’t fit neatly into a decomposition approach.
Code Example: The Traveling Salesman Problem
Let’s look at a concrete example of an indivisible problem: the Traveling Salesman Problem (TSP). This classic NP-hard problem illustrates why some challenges resist decomposition.
Here’s a naive attempt to solve TSP by decomposing it into finding the shortest paths between pairs of cities:
function solveTSPByDecomposition(cities) {
let route = [cities[0]];
let currentCity = cities[0];
let remainingCities = cities.slice(1);
while (remainingCities.length > 0) {
// Find the nearest unvisited city
let nearestCity = findNearestCity(currentCity, remainingCities);
route.push(nearestCity);
currentCity = nearestCity;
remainingCities = remainingCities.filter(city => city !== nearestCity);
}
// Return to starting city
route.push(cities[0]);
return route;
}
function findNearestCity(currentCity, cities) {
let nearestCity = null;
let shortestDistance = Infinity;
for (let city of cities) {
let distance = calculateDistance(currentCity, city);
if (distance < shortestDistance) {
shortestDistance = distance;
nearestCity = city;
}
}
return nearestCity;
}
function calculateDistance(city1, city2) {
// Calculate Euclidean distance between two cities
return Math.sqrt(
Math.pow(city2.x - city1.x, 2) +
Math.pow(city2.y - city1.y, 2)
);
}
This approach (known as the nearest neighbor algorithm) seems reasonable: we’re breaking down the problem into a series of “find the nearest unvisited city” subproblems. However, this algorithm often produces suboptimal routes because it makes locally optimal choices without considering the global picture.
A more effective approach might use simulated annealing, which considers the route as a whole:
function solveTSPSimulatedAnnealing(cities, initialTemperature = 100, coolingRate = 0.995) {
// Generate initial solution
let currentSolution = generateRandomRoute(cities);
let bestSolution = [...currentSolution];
let currentEnergy = calculateRouteLength(currentSolution);
let bestEnergy = currentEnergy;
let temperature = initialTemperature;
while (temperature > 0.1) {
// Generate a neighboring solution
let newSolution = generateNeighbor(currentSolution);
let newEnergy = calculateRouteLength(newSolution);
// Decide whether to accept the new solution
if (acceptanceProbability(currentEnergy, newEnergy, temperature) > Math.random()) {
currentSolution = newSolution;
currentEnergy = newEnergy;
if (currentEnergy < bestEnergy) {
bestSolution = [...currentSolution];
bestEnergy = currentEnergy;
}
}
// Cool the system
temperature *= coolingRate;
}
return bestSolution;
}
function generateRandomRoute(cities) {
let route = [...cities];
// Fisher-Yates shuffle
for (let i = route.length - 1; i > 0; i--) {
const j = Math.floor(Math.random() * (i + 1));
[route[i], route[j]] = [route[j], route[i]];
}
return route;
}
function generateNeighbor(route) {
let newRoute = [...route];
// Swap two random cities
let i = Math.floor(Math.random() * route.length);
let j = Math.floor(Math.random() * route.length);
[newRoute[i], newRoute[j]] = [newRoute[j], newRoute[i]];
return newRoute;
}
function calculateRouteLength(route) {
let length = 0;
for (let i = 0; i < route.length - 1; i++) {
length += calculateDistance(route[i], route[i + 1]);
}
// Add distance from last city back to first city
length += calculateDistance(route[route.length - 1], route[0]);
return length;
}
function acceptanceProbability(currentEnergy, newEnergy, temperature) {
// If the new solution is better, accept it
if (newEnergy < currentEnergy) {
return 1.0;
}
// If the new solution is worse, accept it with a probability
return Math.exp((currentEnergy - newEnergy) / temperature);
}
This approach treats the route as a whole, making probabilistic decisions based on the overall route length rather than trying to optimize individual segments. It’s more effective because it acknowledges the indivisible nature of the TSP.
Conclusion: Embracing Complexity
The advice to “break big problems into smaller ones” is valuable in many contexts, but it’s not a universal solution. Some problems resist decomposition due to emergent properties, tight coupling between components, or inherent mathematical complexity.
Recognizing when a problem might be indivisible is an important skill for any programmer or algorithm designer. It prevents you from forcing inappropriate decomposition approaches that might actually increase complexity rather than reduce it.
When facing potentially indivisible problems, consider alternative approaches:
- Holistic design thinking
- Iterative refinement
- Simulation and modeling
- Pattern recognition
- Constraint-based thinking
These approaches often lead to more elegant and effective solutions for complex challenges.
Remember that the goal isn’t to avoid decomposition entirely, but to use it judiciously, recognizing its limitations. The most skilled problem solvers know when to break problems down and when to approach them as integrated wholes.
By expanding your problem-solving toolkit beyond simple decomposition, you’ll be better equipped to tackle the truly challenging problems in programming, algorithm design, and software engineering. These are often the problems that are most interesting, impactful, and rewarding to solve.
So the next time someone tells you to “just break it down into smaller problems,” consider whether that’s really the best approach. Sometimes, embracing the complexity and addressing the problem holistically is the path to a more elegant and effective solution.