Why You Can’t Apply Textbook Knowledge to Real Problems
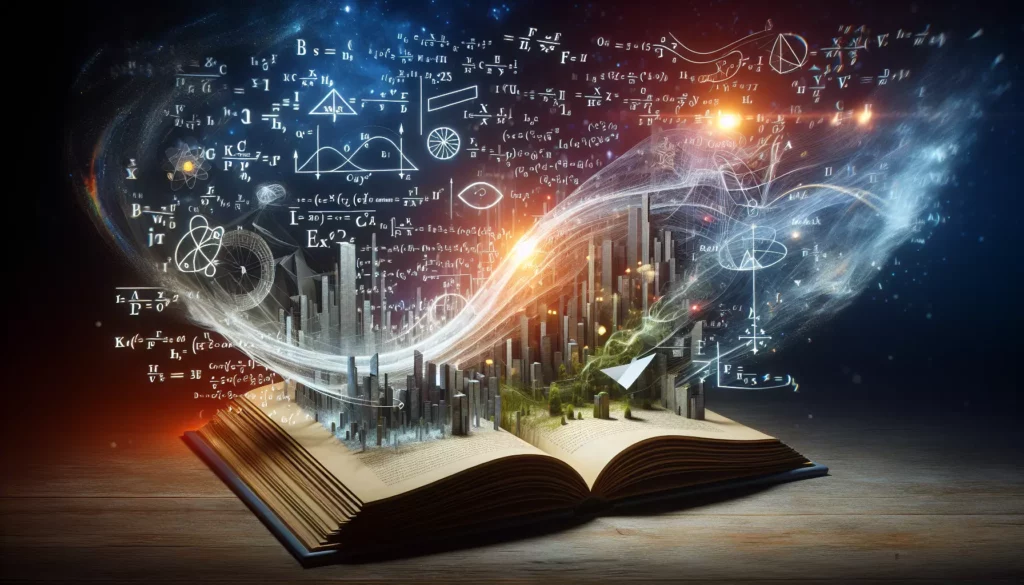
Have you ever found yourself confidently approaching a programming problem, armed with textbook knowledge, only to hit a wall when trying to implement a solution? You’re not alone. The gap between theoretical understanding and practical application is a challenge that plagues many aspiring developers.
In this article, we’ll explore why textbook knowledge often falls short when tackling real world programming challenges, and what you can do to bridge this critical gap in your development journey.
The Theory Practice Gap in Programming
Textbooks are essential for learning fundamental concepts and principles of programming. They provide structured knowledge, clear explanations of algorithms, and well defined examples. However, when you step into the real world of software development, you quickly realize that textbook solutions rarely map perfectly to the messy, complex problems you encounter.
What Textbooks Teach vs. What Real Problems Demand
Textbook World | Real World |
---|---|
Clean, well defined problems | Ambiguous, evolving requirements |
Ideal conditions and constraints | Unpredictable limitations and edge cases |
Simplified examples | Complex, interconnected systems |
Focus on algorithmic correctness | Balance between correctness, performance, maintainability, and user experience |
Single, “correct” solution | Multiple viable approaches with different tradeoffs |
Let’s look at five key reasons why textbook knowledge often proves insufficient when facing real world programming challenges.
1. Real Problems Are Ambiguous and Ill Defined
Textbooks present problems with clear parameters, constraints, and objectives. The famous “FizzBuzz” problem, for instance, has explicit rules that leave little room for interpretation. But in the real world, problem definitions are rarely so neat.
The Reality of Problem Definition
In professional settings, requirements often come as vague descriptions that might change midway through development. You might be asked to “optimize the user experience” or “make the system more scalable” without precise metrics or boundaries.
Consider this common scenario: A product manager requests a feature to “allow users to filter their content.” This seemingly simple requirement raises numerous questions:
- What content types need filtering?
- What filter criteria should be available?
- Should filters be combinable?
- How should the UI represent these filters?
- What performance constraints exist?
Textbooks don’t prepare you for the iterative process of requirement clarification that’s fundamental to real world development.
Example: Textbook Sorting vs. Real World Sorting
In textbooks, sorting problems typically involve arranging a list of numbers in ascending or descending order. The focus is on understanding algorithms like QuickSort, MergeSort, or Bubble Sort.
In real applications, sorting might involve:
- Multi column sorting with different priorities
- Localization aware string sorting that respects different languages’ alphabetical orders
- Custom business logic (e.g., placing premium customers first regardless of other sorting criteria)
- Pagination and its interaction with sorting operations
- Performance considerations for datasets too large to fit in memory
These complexities require adapting and combining textbook knowledge rather than directly applying it.
2. Scale and Performance Constraints Change Everything
Textbook examples typically operate at a scale that allows you to ignore performance considerations. They might present an O(n²) algorithm alongside an O(n log n) alternative, but rarely delve into the practical implications when n reaches millions or billions.
When Big O Notation Meets Reality
While Big O notation is invaluable for understanding algorithmic efficiency, real world applications require more nuanced considerations:
- Constant factors that Big O ignores can be critical in practice
- Memory usage may be more constraining than CPU time
- I/O operations and network latency often dominate performance concerns
- Cache efficiency can make theoretically “slower” algorithms faster in practice
Example: Finding Duplicates
Let’s look at the problem of finding duplicates in a collection:
// Textbook approach for finding duplicates
function findDuplicates(array) {
const seen = new Set();
const duplicates = new Set();
for (const item of array) {
if (seen.has(item)) {
duplicates.add(item);
} else {
seen.add(item);
}
}
return [...duplicates];
}
This works perfectly for textbook examples with small arrays of primitive values. But in the real world, you might face:
- Arrays with millions of elements that won’t fit in memory
- Objects that require custom equality comparison
- Distributed datasets across multiple servers
- Streaming data where you can’t hold the entire collection
These scenarios require completely different approaches, such as probabilistic data structures like Bloom filters, database-specific solutions, or distributed algorithms.
3. Systems Integration Challenges
Textbooks excel at teaching isolated concepts and algorithms. However, real world programming involves integrating multiple systems, libraries, and frameworks that weren’t necessarily designed to work together.
The Integration Reality
Modern software development rarely involves building everything from scratch. Instead, you’ll find yourself:
- Working with legacy systems that can’t be easily modified
- Integrating third party APIs with inconsistent interfaces
- Dealing with versioning conflicts between dependencies
- Managing asynchronous communication between components
- Handling different data formats and conversion between them
Example: Data Processing Pipeline
Consider building a data processing pipeline. A textbook might teach you about sorting algorithms, filtering techniques, and data transformations as separate concepts. But a real world pipeline might involve:
- Extracting data from a REST API that rate-limits requests
- Transforming inconsistent JSON structures into a standardized format
- Enriching records with data from a GraphQL service
- Handling validation failures gracefully
- Writing results to a database while managing transaction boundaries
- Implementing retry logic for failed operations
- Monitoring and logging throughout the process
Each step introduces integration challenges that go far beyond textbook algorithms.
The API Documentation Gap
Even when documentation exists, it often describes ideal usage patterns rather than addressing common integration challenges. You’ll rarely find guidance on:
- How to handle API rate limiting effectively
- Strategies for dealing with inconsistent responses
- Managing authentication token lifecycles
- Graceful degradation when services are unavailable
These patterns must be learned through experience or by studying real world codebases.
4. Error Handling and Edge Cases Dominate Real Code
Examine any production quality code, and you’ll notice that error handling often constitutes a significant portion of the codebase. Textbooks, focused on conveying core concepts, typically minimize or omit error handling entirely.
The 80/20 Rule of Programming
A common observation in software development is that you’ll spend 20% of your time implementing the main functionality and 80% handling edge cases, errors, and exceptional conditions.
Textbook examples might look like this:
function divide(a, b) {
return a / b;
}
While production code needs to consider:
function divide(a, b) {
// Input validation
if (typeof a !== 'number' || typeof b !== 'number') {
throw new TypeError('Both arguments must be numbers');
}
// Edge case handling
if (b === 0) {
throw new Error('Division by zero is not allowed');
}
// Special cases
if (!isFinite(a) || !isFinite(b)) {
// Handle infinity or NaN inputs
return Number.NaN;
}
// Actual operation
const result = a / b;
// Result validation
if (result === 0 && a !== 0) {
console.warn('Result underflow: returned zero for non-zero input');
}
return result;
}
The contrast is stark. The real world version contains significantly more code dedicated to handling edge cases than to performing the actual division operation.
The Unpredictability Factor
Real world applications must be resilient against:
- Invalid or malicious user inputs
- Network failures and timeouts
- Resource constraints (memory, disk space, etc.)
- Concurrent access issues
- External service disruptions
Textbooks rarely convey the defensive mindset required to anticipate and handle these scenarios gracefully.
5. Maintenance and Evolution Over Time
Textbooks present algorithms and data structures as static entities, but real world code is constantly evolving. Today’s elegant solution might become tomorrow’s maintenance nightmare as requirements change and systems grow.
The Long Term View
Production code must consider:
- Readability and maintainability for future developers
- Extensibility to accommodate changing requirements
- Technical debt management
- Backward compatibility constraints
- Documentation needs
These considerations often lead to solutions that look quite different from textbook examples, with additional abstraction layers, design patterns, and architectural choices that might seem unnecessary when viewing the problem in isolation.
Example: Simple CRUD Operation vs. Production Implementation
A textbook might present a basic create operation as:
function createUser(userData) {
database.insert('users', userData);
return userData;
}
A production implementation might look more like:
async function createUser(userData, options = {}) {
// Input validation and sanitization
const validatedData = validateUserData(userData);
// Enrichment with system-generated fields
const enrichedData = {
...validatedData,
createdAt: new Date(),
updatedAt: new Date(),
status: 'active',
id: generateUniqueId()
};
// Pre-save hooks (e.g., password hashing)
await runPreSaveHooks(enrichedData);
// Transaction management
const transaction = options.transaction || await database.beginTransaction();
try {
// Actual database operation
const result = await database.insert('users', enrichedData, { transaction });
// Related operations (e.g., creating default settings)
await createDefaultUserSettings(enrichedData.id, { transaction });
// Commit if we started the transaction
if (!options.transaction) {
await transaction.commit();
}
// Post-save operations (non-transactional)
await sendWelcomeEmail(enrichedData);
publishAuditEvent('user.created', enrichedData);
return result;
} catch (error) {
// Rollback if we started the transaction
if (!options.transaction) {
await transaction.rollback();
}
// Error handling and logging
logger.error('Failed to create user', { error, userData });
// Error classification and transformation
if (error.code === 'DUPLICATE_ENTRY') {
throw new ConflictError('User with this email already exists');
}
throw new DatabaseError('Failed to create user', { cause: error });
}
}
The production version accounts for validation, transactions, error handling, logging, event publishing, and more. It’s designed to be robust, maintainable, and integrated with the broader system.
Bridging the Gap: From Textbook Knowledge to Practical Skills
Recognizing the limitations of textbook knowledge is the first step toward becoming a more effective developer. Here are strategies to help bridge the gap between theory and practice:
1. Build Real Projects
Nothing bridges the theory practice gap like building actual projects that solve real problems. Start with small applications and gradually increase complexity. Focus on projects that:
- Involve data persistence
- Require user authentication
- Integrate with external APIs
- Handle asynchronous operations
- Need responsive user interfaces
Personal projects allow you to experience the full development lifecycle and encounter the messy realities that textbooks simplify away.
2. Study Open Source Codebases
Examining well maintained open source projects provides invaluable insights into how experienced developers structure code for real world use. Look for:
- How they handle error conditions
- Implementation of design patterns
- Testing strategies
- Documentation approaches
- Performance optimizations
Start with smaller libraries in your area of interest before tackling larger frameworks. Tools like GitHub’s code search can help you find implementations of specific algorithms or patterns.
3. Pair Program with Experienced Developers
Working alongside seasoned developers provides exposure to practical wisdom that’s rarely captured in textbooks. You’ll learn:
- Debugging techniques
- Decision making processes
- Shortcut identification (knowing when to use libraries vs. building custom solutions)
- Code review priorities
Look for opportunities through mentorship programs, coding meetups, or collaborative open source projects if you don’t have access to experienced colleagues.
4. Practice Deliberate Problem Solving
Rather than jumping straight to coding solutions, develop a systematic approach to problem solving:
- Clarify requirements: Identify ambiguities and ask questions to define boundaries
- Consider constraints: Understand performance needs, compatibility requirements, and other limitations
- Brainstorm approaches: Generate multiple potential solutions
- Evaluate tradeoffs: Compare approaches based on multiple criteria, not just algorithmic efficiency
- Plan for errors: Identify what could go wrong and how to handle it
- Test thoroughly: Consider edge cases and unexpected inputs
This structured approach helps bridge textbook knowledge to practical application by forcing you to consider the messy realities of real world problems.
5. Learn System Design Principles
While algorithms and data structures are important, system design skills are crucial for tackling larger problems. Study:
- Architectural patterns (microservices, event-driven architecture, etc.)
- Distributed systems concepts (consistency models, failure modes, etc.)
- Database design and query optimization
- API design principles
- Scalability patterns
Resources like system design interview books and courses can provide structured introductions to these topics, even if you’re not preparing for interviews.
Case Study: Implementing a Search Feature
Let’s examine how a seemingly simple feature—implementing search functionality—differs between textbook approaches and real world implementation.
Textbook Approach
A textbook might present search as an exercise in string matching algorithms:
function search(items, query) {
return items.filter(item =>
item.toLowerCase().includes(query.toLowerCase())
);
}
The focus would be on understanding string comparison, perhaps introducing more sophisticated algorithms like Boyer-Moore or Knuth-Morris-Pratt for efficiency.
Real World Implementation
In contrast, a production search feature might involve:
1. User Experience Considerations
- Typeahead suggestions as users type
- Highlighting matched portions of results
- Sorting results by relevance
- Filtering options to narrow results
- Pagination for large result sets
2. Performance Optimizations
- Debouncing input to reduce unnecessary searches
- Implementing caching for recent queries
- Using specialized search engines like Elasticsearch
- Creating database indexes to speed up queries
- Implementing denormalized data structures for search efficiency
3. Search Quality Improvements
- Handling misspellings with fuzzy matching
- Supporting synonyms and related terms
- Implementing stemming to match word variations
- Weighting fields differently (e.g., title matches more important than description matches)
- Incorporating user behavior data to improve relevance
4. Operational Concerns
- Monitoring search performance and error rates
- Tracking popular searches for optimization
- Implementing rate limiting to prevent abuse
- Handling partial system failures gracefully
A simplified real world implementation might look something like:
// Client-side implementation with debouncing
let searchTimeout;
function handleSearchInput(event) {
const query = event.target.value;
// Clear previous timeout
clearTimeout(searchTimeout);
// Set loading state
setSearchState({ isLoading: true });
// Debounce input to avoid excessive requests
searchTimeout = setTimeout(() => {
performSearch(query);
}, 300);
}
async function performSearch(query) {
try {
// Check cache first
const cachedResults = searchCache.get(query);
if (cachedResults) {
setSearchState({
results: cachedResults,
isLoading: false,
error: null
});
return;
}
// Construct request with parameters
const params = new URLSearchParams({
q: query,
page: currentPage,
limit: resultsPerPage,
filters: JSON.stringify(activeFilters)
});
// Perform API request
const response = await fetch(`/api/search?${params}`);
// Handle non-200 responses
if (!response.ok) {
throw new Error(`Search failed: ${response.status}`);
}
const data = await response.json();
// Cache results
searchCache.set(query, data.results);
// Update UI
setSearchState({
results: data.results,
totalResults: data.total,
isLoading: false,
error: null
});
// Log analytics
logSearchAnalytics(query, data.total);
} catch (error) {
console.error('Search error:', error);
setSearchState({
isLoading: false,
error: 'An error occurred while searching. Please try again.'
});
}
}
This implementation addresses debouncing, caching, error handling, and analytics—concerns that rarely appear in textbook examples but are essential in production code.
The Value of Textbook Knowledge
Despite the limitations we’ve discussed, textbook knowledge remains valuable. It provides:
- Foundational understanding: Core algorithms and data structures form the building blocks of more complex solutions
- Common vocabulary: Technical terms and concepts enable efficient communication with other developers
- Problem solving frameworks: Analytical approaches to breaking down complex problems
- Performance analysis tools: Techniques like Big O notation for evaluating algorithmic efficiency
The key is recognizing that textbook knowledge is a starting point, not the destination. It provides tools for your toolbox, but knowing when and how to apply those tools comes with experience.
Conclusion: Embracing the Complexity
The gap between textbook knowledge and real world application isn’t a flaw in educational materials but rather a reflection of the inherent complexity of software development. Textbooks necessarily simplify concepts to make them teachable, while real world problems embrace complexity in all its messy glory.
Becoming an effective developer means learning to navigate this complexity rather than being overwhelmed by it. It requires developing judgment about which aspects of a problem are most important, which solutions best address the specific context, and what tradeoffs are acceptable.
Remember that every experienced developer has faced the same challenges. The path from textbook knowledge to practical expertise isn’t about abandoning what you’ve learned but about enriching it with context, experience, and a deeper understanding of the environments in which code operates.
By building real projects, studying existing codebases, collaborating with experienced developers, and practicing deliberate problem solving, you can bridge the gap and develop the practical skills that transform theoretical knowledge into effective solutions.
The journey from textbook to real world isn’t easy, but it’s incredibly rewarding. Each challenge you overcome builds not just technical skills but also confidence in your ability to tackle whatever problems come next. And in a field as dynamic as software development, that adaptability may be the most valuable skill of all.
Further Resources
Books That Bridge Theory and Practice
- “Clean Code” by Robert C. Martin
- “Designing Data-Intensive Applications” by Martin Kleppmann
- “Pragmatic Programmer” by Andrew Hunt and David Thomas
- “System Design Interview” by Alex Xu
- “Working Effectively with Legacy Code” by Michael Feathers
Online Learning Platforms
- AlgoCademy – For algorithmic thinking and practical coding skills
- GitHub – For studying real world codebases
- Stack Overflow – For seeing how developers solve practical problems
- LeetCode – For practicing algorithmic problem solving
- HackerRank – For coding challenges that build practical skills
Communities for Practical Learning
- Local coding meetups
- Open source project communities
- Language specific forums and Discord servers
- Professional development communities like Dev.to
Remember that the most effective learning combines theory with practice. As you continue your programming journey, seek opportunities to apply what you learn to real problems, and you’ll steadily bridge the gap between textbook knowledge and practical expertise.