Why You Cannot Identify Which Programming Approach Is Best
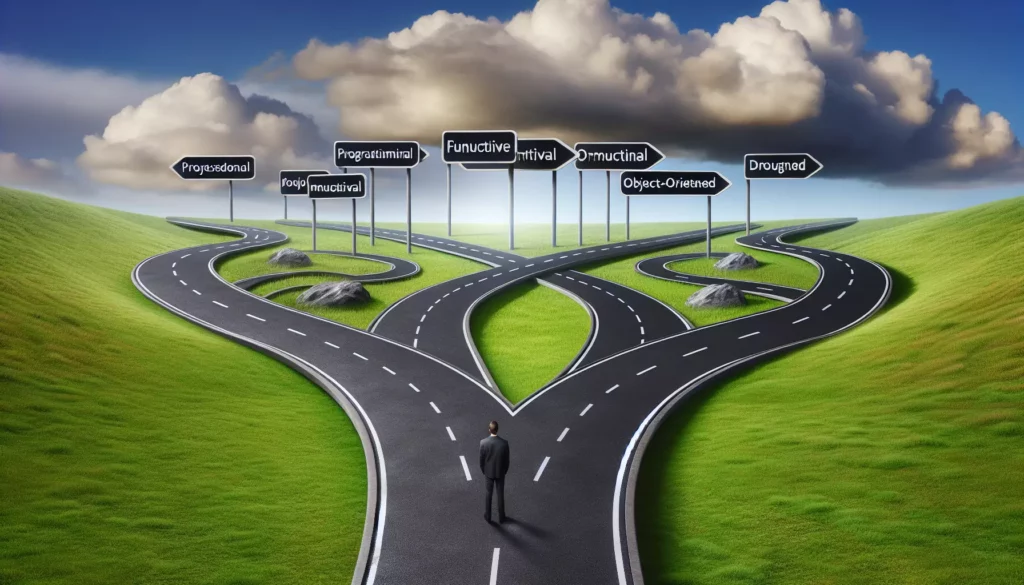
In the world of programming and software development, one of the most common questions beginners ask is, “What is the best programming approach?” Whether it’s choosing between functional and object-oriented programming, deciding on a development methodology, or selecting the right algorithm for a specific problem, the quest for the “best” approach seems never-ending.
However, experienced developers know a secret: there is no universally “best” approach in programming. This reality can be frustrating for newcomers who are looking for clear-cut answers, but understanding why this is the case is a crucial step in your development as a programmer.
The Myth of the Perfect Programming Paradigm
Programming paradigms represent different ways of organizing and structuring code. Object-oriented programming (OOP), functional programming, procedural programming, and declarative programming are just a few examples. Each paradigm has its own philosophy, strengths, and weaknesses.
Many beginners hope to find the “one true paradigm” that will solve all their programming challenges. However, this is a misconception that can limit your growth as a developer.
The Contextual Nature of Programming
The effectiveness of a programming approach depends heavily on context. Factors that influence which approach might work best include:
- The specific problem you’re trying to solve
- The constraints of your environment (hardware, software, time, resources)
- The skills and preferences of your development team
- The requirements for maintenance and scalability
- The characteristics of your target users
For example, functional programming excels at handling complex data transformations with minimal side effects, making it ideal for certain types of applications. However, object-oriented programming might be more intuitive for modeling real-world entities and their interactions.
The Multi-Dimensional Nature of “Best”
When we talk about the “best” approach, we need to ask: best according to what criteria? Different stakeholders and different projects have different priorities:
Performance vs. Readability
Some applications require maximum performance, where every millisecond counts. Others prioritize code readability and maintainability, recognizing that developer time is often more expensive than CPU time.
Consider this simple example of calculating the sum of numbers from 1 to n:
// Iterative approach (potentially more readable for beginners)
function sumNumbers(n) {
let sum = 0;
for (let i = 1; i <= n; i++) {
sum += i;
}
return sum;
}
// Mathematical approach (more efficient)
function sumNumbersMath(n) {
return (n * (n + 1)) / 2;
}
The mathematical approach is significantly faster for large values of n, but the iterative approach might be more intuitive for someone new to programming.
Development Speed vs. Long-term Maintainability
Some projects need to get to market quickly, while others need to be maintained for years or decades. These different requirements might lead to different architectural choices.
A rapid prototype might benefit from a high-level language and framework that allows for quick development, while a system that needs to be maintained for decades might prioritize simplicity, clear documentation, and avoiding dependencies on technologies that might become obsolete.
Memory Efficiency vs. CPU Efficiency
Different algorithms and data structures make different trade-offs between memory usage and computational efficiency. The classic example is dynamic programming, which often trades memory for speed:
// Recursive Fibonacci (CPU intensive, memory efficient)
function fibRecursive(n) {
if (n <= 1) return n;
return fibRecursive(n - 1) + fibRecursive(n - 2);
}
// Dynamic Programming Fibonacci (memory intensive, CPU efficient)
function fibDP(n) {
let memo = [0, 1];
for (let i = 2; i <= n; i++) {
memo[i] = memo[i - 1] + memo[i - 2];
}
return memo[n];
}
For small values of n, the difference might be negligible, but for larger values, the dynamic programming approach is dramatically faster, while using more memory.
The Evolution of Programming Approaches
Programming approaches are not static; they evolve over time as technology advances and as we gain new insights into software development. What was considered “best practice” a decade ago might be viewed differently today.
Historical Perspective
In the early days of computing, when hardware was expensive and limited, efficiency was paramount. Programs were written in assembly language or other low-level languages that gave programmers precise control over the machine.
As hardware became more powerful and software more complex, higher-level languages and paradigms emerged. Structured programming gave way to object-oriented programming, which in turn has seen the rise of functional programming concepts being incorporated into mainstream languages.
Each shift was driven by changing needs and constraints, not because one approach was inherently “better” than another.
Modern Hybrid Approaches
Today’s most successful programming languages and frameworks often incorporate elements from multiple paradigms. JavaScript supports both object-oriented and functional programming styles. Python is multi-paradigm by design. Even traditionally object-oriented languages like Java have added functional programming features.
This hybridization reflects the recognition that different problems benefit from different approaches, and that the ability to choose the right tool for the job is more valuable than dogmatic adherence to a single paradigm.
The Role of Problem Characteristics
The nature of the problem you’re solving often suggests which approach might be most suitable. Different problem domains have different characteristics that lend themselves to different solutions.
Data-Intensive Applications
Applications that process large amounts of data often benefit from functional programming techniques, which make it easier to reason about data transformations and to parallelize operations.
// Functional approach to processing a list of numbers
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map(n => n * 2);
const sum = doubled.reduce((acc, n) => acc + n, 0);
This approach clearly expresses the intent of the code and makes it easy to understand the data flow.
Interactive User Interfaces
User interfaces often benefit from event-driven programming models, where the program responds to user actions. Object-oriented programming can be useful for modeling UI components and their relationships.
// Object-oriented approach to a simple UI component
class Button {
constructor(text, onClick) {
this.text = text;
this.onClick = onClick;
}
render() {
const button = document.createElement("button");
button.textContent = this.text;
button.addEventListener("click", this.onClick);
return button;
}
}
const submitButton = new Button("Submit", () => {
console.log("Form submitted!");
});
document.body.appendChild(submitButton.render());
This approach encapsulates the behavior and state of UI components, making them reusable and maintainable.
Systems Programming
Low-level systems programming, such as operating systems or embedded systems, often requires precise control over memory and resources. Languages like C or Rust, which provide low-level access while still offering some higher-level abstractions, are often preferred in this domain.
// C code for a simple memory allocation
void* allocate_memory(size_t size) {
void* ptr = malloc(size);
if (ptr == NULL) {
// Handle allocation failure
return NULL;
}
// Initialize memory to zero
memset(ptr, 0, size);
return ptr;
}
This approach gives the programmer explicit control over memory allocation and initialization, which is critical in systems programming.
The Impact of Team and Organizational Factors
Programming doesn’t happen in a vacuum. The choice of approach is often influenced by the team and organization in which development takes place.
Team Expertise and Experience
A team with deep expertise in a particular language or paradigm will likely be more productive using that approach, even if another approach might theoretically be “better” for the problem at hand.
For example, a team of experienced Java developers might be more productive using Java and its ecosystem, even for a problem that might be more elegantly solved in a functional language like Haskell, simply because they can leverage their existing knowledge and tools.
Organizational Standards and Legacy Systems
Organizations often have established standards and practices that influence the choice of programming approach. Integration with existing systems can also be a major factor.
If your organization has invested heavily in a particular technology stack, it might make more sense to continue using that stack for new projects, even if another approach might be technically superior in isolation.
The Pragmatic Approach: Embracing Diversity
Given all these factors, the most effective programmers tend to be pragmatic rather than dogmatic. They recognize that different situations call for different approaches, and they develop a diverse toolkit of techniques that they can apply as needed.
The Value of Polyglot Programming
Polyglot programming—the practice of using multiple programming languages—is increasingly common in modern software development. Different languages have different strengths, and using the right language for each part of a system can lead to better overall results.
For example, a web application might use JavaScript for the front-end, Python for data processing, and Go for high-performance microservices. Each language is chosen for its strengths in a particular domain.
Continuous Learning and Adaptation
The field of programming is constantly evolving, with new languages, frameworks, and paradigms emerging regularly. Successful programmers embrace continuous learning and are willing to adapt their approaches as the field evolves.
This doesn’t mean chasing every new trend, but rather staying open to new ideas and being willing to incorporate them when they offer genuine improvements.
Case Studies: Different Approaches for Different Scenarios
Let’s look at some specific scenarios and how different approaches might be applied to each.
Case Study 1: Web Application Development
For a typical web application, you might choose:
- Front-end: React or Vue.js, using a component-based architecture that combines aspects of object-oriented and functional programming
- Back-end: Node.js with Express for a JavaScript-based stack, or Django/Flask for Python, depending on team expertise and specific requirements
- Database: SQL for structured data with clear relationships, or NoSQL for more flexible, document-oriented data
Each of these choices involves trade-offs and depends on factors like the complexity of the application, the expected scale, and the team’s expertise.
Case Study 2: Data Analysis Pipeline
For a data analysis pipeline processing large datasets, you might choose:
- Data Processing: Python with libraries like Pandas and NumPy for their rich ecosystem of data analysis tools
- Parallel Processing: Spark or Dask for distributed computing, using functional programming concepts to parallelize operations
- Visualization: D3.js or Matplotlib for creating interactive or static visualizations
This approach leverages the strengths of different tools for different parts of the pipeline, recognizing that no single tool is optimal for all aspects of data analysis.
Case Study 3: Embedded System
For an embedded system with limited resources, you might choose:
- Core Functionality: C or C++ for low-level control and efficiency
- Architecture: A simple, modular design with clear interfaces between components
- Memory Management: Manual memory management or simple allocation patterns to avoid fragmentation and ensure predictable performance
This approach prioritizes efficiency and reliability over developer convenience, which is appropriate given the constraints of embedded systems.
The Role of Algorithmic Thinking
Regardless of the specific programming paradigm or language you choose, algorithmic thinking remains a fundamental skill. The ability to break down problems, identify patterns, and design efficient solutions is valuable across all approaches.
Problem Decomposition
Breaking a complex problem into smaller, more manageable subproblems is a key skill in programming. This approach works whether you’re using functional, object-oriented, or any other paradigm.
// Problem: Calculate statistics for a dataset
// Decomposition: Split into functions for each statistic
function calculateMean(data) {
const sum = data.reduce((acc, val) => acc + val, 0);
return sum / data.length;
}
function calculateMedian(data) {
const sorted = [...data].sort((a, b) => a - b);
const mid = Math.floor(sorted.length / 2);
return sorted.length % 2 === 0
? (sorted[mid - 1] + sorted[mid]) / 2
: sorted[mid];
}
function calculateMode(data) {
const counts = {};
data.forEach(val => {
counts[val] = (counts[val] || 0) + 1;
});
let mode;
let maxCount = 0;
for (const [val, count] of Object.entries(counts)) {
if (count > maxCount) {
maxCount = count;
mode = Number(val);
}
}
return mode;
}
function calculateStatistics(data) {
return {
mean: calculateMean(data),
median: calculateMedian(data),
mode: calculateMode(data)
};
}
This approach breaks down the problem of calculating statistics into smaller, more manageable functions, each responsible for a specific calculation.
Algorithmic Efficiency
Understanding the efficiency of algorithms in terms of time and space complexity is crucial for writing performant code, regardless of the programming paradigm you use.
For example, the choice between a quick sort and a merge sort might depend on the characteristics of your data and the requirements of your application, not on whether you’re using functional or object-oriented programming.
// Two different sorting algorithms with different characteristics
// Quick sort: In-place, often faster in practice, but worst-case O(n²)
function quickSort(arr, left = 0, right = arr.length - 1) {
if (left >= right) return;
const pivotIndex = partition(arr, left, right);
quickSort(arr, left, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, right);
return arr;
}
function partition(arr, left, right) {
const pivot = arr[right];
let i = left - 1;
for (let j = left; j < right; j++) {
if (arr[j] <= pivot) {
i++;
[arr[i], arr[j]] = [arr[j], arr[i]];
}
}
[arr[i + 1], arr[right]] = [arr[right], arr[i + 1]];
return i + 1;
}
// Merge sort: Stable, guaranteed O(n log n), but requires extra space
function mergeSort(arr) {
if (arr.length <= 1) return arr;
const mid = Math.floor(arr.length / 2);
const left = mergeSort(arr.slice(0, mid));
const right = mergeSort(arr.slice(mid));
return merge(left, right);
}
function merge(left, right) {
const result = [];
let leftIndex = 0;
let rightIndex = 0;
while (leftIndex < left.length && rightIndex < right.length) {
if (left[leftIndex] < right[rightIndex]) {
result.push(left[leftIndex]);
leftIndex++;
} else {
result.push(right[rightIndex]);
rightIndex++;
}
}
return result.concat(left.slice(leftIndex)).concat(right.slice(rightIndex));
}
Both quicksort and mergesort are efficient sorting algorithms, but they have different characteristics that make them suitable for different scenarios.
The Importance of Adaptability
Perhaps the most important skill for a programmer is not mastery of any particular approach, but rather the ability to adapt and choose the right approach for each situation.
Developing a Flexible Mindset
Rather than becoming attached to a single way of doing things, cultivate a flexible mindset that is open to different approaches. Be willing to question your assumptions and to learn from approaches that are different from what you're accustomed to.
This doesn't mean abandoning your expertise or constantly switching between approaches. Rather, it means being aware of the trade-offs involved in different approaches and being willing to choose the one that best fits the current situation.
Building a Diverse Toolkit
As you gain experience as a programmer, aim to build a diverse toolkit of approaches, patterns, and techniques that you can apply as needed. This might include:
- Multiple programming paradigms (object-oriented, functional, procedural)
- Different architectural patterns (microservices, monoliths, event-driven)
- Various problem-solving strategies (divide and conquer, dynamic programming, greedy algorithms)
- Different languages and their ecosystems
Having this diverse toolkit allows you to choose the most appropriate approach for each problem you encounter.
Conclusion: Embracing the Complexity
The question "Which programming approach is best?" has no simple answer because programming is inherently complex and multifaceted. Different problems, different constraints, and different goals call for different approaches.
Rather than seeking a single "best" approach, focus on understanding the trade-offs involved in different approaches and developing the judgment to choose the right approach for each situation. Embrace the diversity of programming paradigms, languages, and techniques as a source of strength rather than confusion.
Remember that the goal of programming is not to adhere to a particular paradigm or methodology, but to solve problems effectively. The best programmers are those who can adapt their approach to the problem at hand, drawing on a diverse toolkit of techniques and a deep understanding of the trade-offs involved.
So the next time someone asks you which programming approach is best, you can confidently explain why that question doesn't have a simple answer—and why that's actually a good thing for the field of programming.
FAQ: Common Questions About Programming Approaches
Q: Isn't object-oriented programming considered the industry standard?
A: While object-oriented programming has been dominant in many areas of software development for decades, it's not universally superior. Functional programming is gaining popularity for its benefits in handling concurrency and maintaining code quality. Many modern languages and frameworks blend aspects of both paradigms. The industry is increasingly recognizing that different paradigms have different strengths and that a multi-paradigm approach often yields the best results.
Q: Should beginners focus on learning one approach thoroughly before exploring others?
A: There's value in developing depth in one approach to build a solid foundation, but it's also important to be exposed to different ways of thinking about problems. A good strategy for beginners might be to focus primarily on one paradigm while being introduced to concepts from others. This prevents the confusion of trying to learn everything at once while still fostering a flexible mindset from the beginning.
Q: How do I know which approach to use for a specific project?
A: Consider factors like the nature of the problem, the constraints of your environment, the expertise of your team, and the long-term goals of the project. Often, the best approach involves combining elements from different paradigms. Don't be afraid to experiment with different approaches on smaller projects to gain experience that will inform your decisions on larger ones.
Q: Are there situations where one approach is clearly better than others?
A: Yes, there are scenarios where certain approaches have clear advantages. For example, functional programming is particularly well-suited for data processing pipelines, while object-oriented programming can be very effective for modeling complex systems with many interacting entities. However, even in these cases, the "best" approach often involves incorporating elements from multiple paradigms rather than adhering strictly to one.
Q: How do I keep up with evolving best practices in programming?
A: Stay engaged with the programming community through forums, blogs, conferences, and open source projects. Be open to new ideas but critical in evaluating them. Remember that not every new trend represents genuine progress, and sometimes older approaches have stood the test of time for good reason. Focus on understanding the principles behind different approaches rather than just the syntax or tools.