Why You Can Solve Puzzles But Struggle With Programming Problems
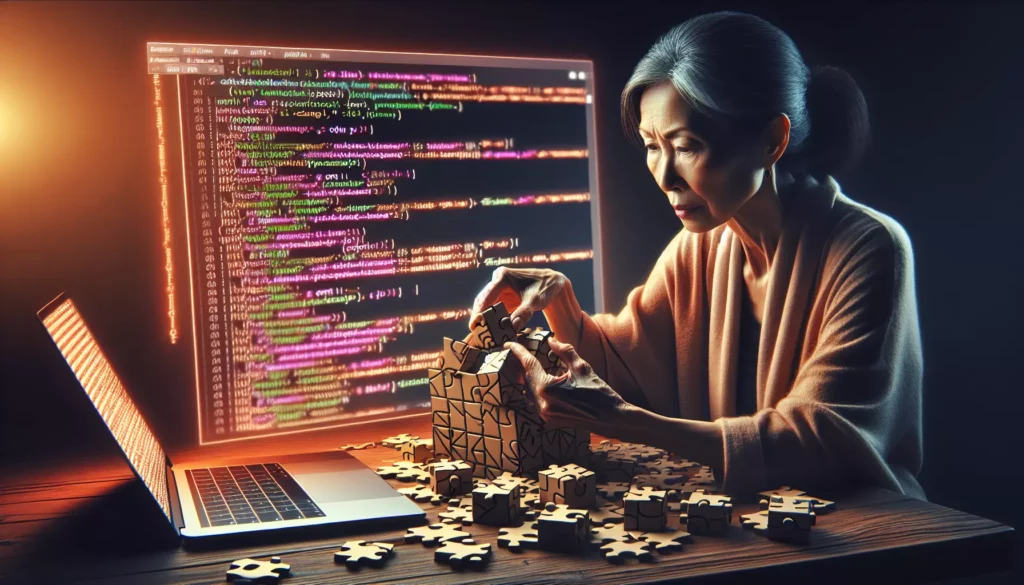
Have you ever noticed that you can solve a Sudoku or complete a jigsaw puzzle with ease, but when faced with a programming challenge, your mind goes blank? This disconnect between puzzle-solving abilities and programming skills is a common experience for many beginners and even intermediate coders. In this article, we’ll explore the fascinating relationship between puzzles and programming, understand why this gap exists, and discover how to bridge it effectively.
The Puzzle Programming Paradox
At first glance, puzzles and programming problems appear remarkably similar. Both require logical thinking, pattern recognition, and methodical approaches to solutions. Yet many people who excel at traditional puzzles find themselves struggling when the puzzle takes the form of a coding challenge.
Consider this scenario: You might spend hours happily solving crosswords or Rubik’s cubes, but when asked to write a simple algorithm to find the largest number in an array, you feel overwhelmed. This phenomenon is what I call the “Puzzle Programming Paradox.”
The Shared Cognitive Skills
Before diving into the differences, let’s acknowledge the significant overlap in cognitive skills used in both activities:
- Pattern Recognition: Both puzzles and programming require identifying patterns and relationships.
- Logical Reasoning: Step-by-step deductive reasoning is essential for both.
- Problem Decomposition: Breaking down complex problems into manageable pieces.
- Spatial Reasoning: Understanding how different components relate to each other.
- Working Memory: Holding multiple pieces of information in mind simultaneously.
With so many shared cognitive requirements, why does the transition from puzzles to programming often feel like stepping into an entirely different intellectual realm?
Key Differences Between Puzzles and Programming
1. The Blank Canvas Effect
When you start a jigsaw puzzle, you have all the pieces in front of you. The challenge is arranging them correctly. In programming, you often start with a blank editor and must create the pieces yourself. This “blank canvas effect” can be paralyzing, especially for beginners.
Traditional puzzles provide all the necessary components and clear boundaries. Programming problems require you to not only solve the problem but also identify and create the building blocks of your solution.
2. Language and Syntax Barriers
Programming requires learning a formal language with strict syntax rules. This adds a layer of complexity that traditional puzzles don’t have. Imagine trying to solve a crossword puzzle where you must also learn a new language simultaneously.
Consider this simple task: “Print all even numbers between 1 and 10.” In English, this is straightforward. In code, you need to understand syntax, loops, conditional statements, and output methods:
for (let i = 1; i <= 10; i++) {
if (i % 2 === 0) {
console.log(i);
}
}
The cognitive load of translating your solution into correct syntax can be overwhelming, even when you conceptually understand the problem.
3. Feedback Loops
Traditional puzzles provide immediate, visual feedback. You know instantly if a puzzle piece fits or if your Sudoku move is valid. Programming feedback is often delayed and sometimes cryptic:
TypeError: Cannot read property 'length' of undefined
This error message might make perfect sense to experienced programmers, but it can be confusing and frustrating for beginners. The disconnect between what you intended and what the computer understood creates a feedback gap that doesn’t exist with traditional puzzles.
4. Abstract vs. Concrete Thinking
Many puzzles operate in concrete, visible spaces. Programming often requires abstract thinking about invisible processes, memory management, and data transformations. This shift from concrete to abstract thinking represents a significant cognitive leap.
For instance, understanding array manipulation requires visualizing data structures that exist only conceptually:
let numbers = [5, 2, 9, 1, 7];
numbers.sort((a, b) => a - b);
Mentally tracing what happens during this sort operation requires a level of abstraction that many traditional puzzles don’t demand.
5. Multiple Solution Paths
Most traditional puzzles have a single correct solution. Programming problems often have multiple valid solutions, each with different trade-offs regarding efficiency, readability, and maintainability. This open-ended nature can be paralyzing for beginners who want to find “the right answer.”
Consider sorting an array. You could use bubble sort, merge sort, quick sort, or many other algorithms. Each has advantages and disadvantages, making the decision process more complex than simply finding the one correct solution.
The Mental Models Gap
Perhaps the most significant difference between puzzles and programming is the requirement for specialized mental models. Programming requires understanding concepts like:
- Variables and memory allocation
- Control flow and execution order
- Data structures and their operations
- Function calls and return values
- Object-oriented concepts
These mental models don’t naturally develop from solving traditional puzzles. They’re unique to computing and require deliberate learning and practice.
When you solve a Sudoku puzzle, you’re working with a mental model you already understand: numbers 1-9 must appear exactly once in each row, column, and box. The rules are clear and intuitive.
Programming requires building new mental models from scratch. Consider recursion, a powerful programming concept where a function calls itself:
function factorial(n) {
if (n <= 1) return 1;
return n * factorial(n - 1);
}
Understanding how this code executes requires a mental model of the call stack and function execution that has no direct parallel in everyday reasoning or traditional puzzles.
Why Traditional Education Often Fails
Many programming courses focus on syntax and specific problems rather than building these crucial mental models. It’s like teaching someone to solve jigsaw puzzles by only showing them completed puzzles without explaining strategies for edge pieces, color matching, or pattern recognition.
Traditional education often follows this pattern:
- Introduce syntax
- Show examples
- Assign problems
This approach works for students who can intuitively develop the necessary mental models, but it leaves many others struggling. The missing step is explicitly teaching the mental models that experienced programmers use to think about code.
Bridging the Gap: From Puzzles to Programming
Now that we understand the differences, how can we leverage puzzle-solving abilities to become better programmers? Here are strategies to bridge this gap:
1. Build Programming Mental Models Explicitly
Rather than focusing solely on syntax, deliberately build mental models for programming concepts:
- Variables: Think of them as labeled boxes holding values.
- Functions: Imagine them as machines that take inputs and produce outputs.
- Arrays: Visualize them as numbered shelves holding items in sequence.
- Objects: Picture them as containers with labeled compartments.
These concrete metaphors provide scaffolding for abstract programming concepts.
2. Use Visualization Tools
Tools like Python Tutor or JavaScript Visualizer allow you to see code execution step-by-step, making abstract processes visible. These tools bridge the gap between concrete puzzle-solving and abstract programming by making the invisible visible.
For example, watching a visualization of the factorial function above helps build an accurate mental model of recursion and the call stack.
3. Practice “Trace Tables”
Trace tables are a technique where you manually track variable values through code execution. This practice builds the skill of mentally simulating code execution:
let x = 5;
let y = 3;
let z = x + y;
x = z * 2;
y = x - z;
Line | x | y | z |
---|---|---|---|
1 | 5 | ? | ? |
2 | 5 | 3 | ? |
3 | 5 | 3 | 8 |
4 | 16 | 3 | 8 |
5 | 16 | 8 | 8 |
This practice helps develop the mental simulation skills that experienced programmers use constantly.
4. Break Down the Syntax Barrier
Reduce cognitive load by separating problem-solving from syntax concerns:
- Solve the problem in plain English first
- Break down your solution into logical steps
- Translate each step into code
This approach allows you to leverage your puzzle-solving skills before tackling the syntax challenge.
5. Use Pseudocode as a Bridge
Pseudocode serves as a middle ground between natural language and programming syntax. It lets you focus on logic without syntax constraints:
// Problem: Find the largest number in an array
// Pseudocode:
Set max to the first element of the array
For each element in the array:
If the element is greater than max:
Set max to the element
Return max
// JavaScript:
function findMax(arr) {
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
This stepped approach bridges natural language problem-solving and formal programming syntax.
6. Leverage Programming Puzzles
Programming puzzles like those found on platforms such as LeetCode, HackerRank, and AlgoCademy provide structured problems that gradually build complexity. These puzzles often come with test cases and expected outputs, creating a more direct feedback loop similar to traditional puzzles.
Start with simple puzzles like:
- Reversing a string
- Finding the sum of array elements
- Checking if a number is prime
These programming puzzles provide the structure of traditional puzzles while building programming-specific skills.
7. Study and Modify Existing Code
Rather than starting with a blank editor, begin by studying and modifying working code. This approach provides scaffolding similar to how puzzle pieces provide structure:
// Original code
function countVowels(str) {
const vowels = ['a', 'e', 'i', 'o', 'u'];
let count = 0;
for (let char of str.toLowerCase()) {
if (vowels.includes(char)) {
count++;
}
}
return count;
}
// Your task: Modify to count consonants instead
This approach reduces the blank canvas effect while still requiring understanding and problem-solving.
The Role of Programming Languages
Some programming languages create a gentler transition from puzzle solving to programming. Python, for example, has syntax that closely resembles pseudocode:
# Finding maximum in Python
def find_max(numbers):
max_num = numbers[0]
for num in numbers:
if num > max_num:
max_num = num
return max_num
Compare this to the equivalent C++ code:
// Finding maximum in C++
int findMax(std::vector<int> numbers) {
int maxNum = numbers[0];
for (int i = 1; i < numbers.size(); i++) {
if (numbers[i] > maxNum) {
maxNum = numbers[i];
}
}
return maxNum;
}
The Python version reduces syntax noise, allowing beginners to focus more on problem-solving logic than language rules. Starting with a more accessible language can help bridge the puzzle-programming gap more effectively.
The Importance of Deliberate Practice
Moving from puzzles to programming requires deliberate practice that specifically targets the gap areas:
1. Syntax Fluency
Practice writing code until the syntax becomes second nature, reducing cognitive load. Create flashcards for common patterns and practice them regularly:
// For loop pattern
for (initialization; condition; update) {
// body
}
// Example
for (let i = 0; i < array.length; i++) {
// do something with array[i]
}
2. Mental Model Building
Deliberately practice visualizing code execution. After writing a function, trace through it manually before running it. This builds the mental simulation skills essential for programming.
3. Pattern Recognition
Study common programming patterns and algorithms. Recognizing these patterns allows you to apply them to new problems, similar to how recognizing edge pieces helps in jigsaw puzzles:
- Two-pointer technique
- Sliding window
- Divide and conquer
- Dynamic programming
Platforms like AlgoCademy categorize problems by these patterns, helping you build a mental library of solutions.
Real World Example: The Fibonacci Sequence
Let’s see how these approaches work with a concrete example: calculating the nth Fibonacci number.
Step 1: Understand the Problem
The Fibonacci sequence is: 0, 1, 1, 2, 3, 5, 8, 13, 21, … where each number is the sum of the two previous numbers.
Step 2: Plain English Solution
“To find the nth Fibonacci number, I need to calculate all Fibonacci numbers up to n. I’ll start with 0 and 1, then repeatedly add the last two numbers to get the next one until I reach the nth number.”
Step 3: Pseudocode
If n is 0, return 0
If n is 1, return 1
Set a to 0
Set b to 1
For i from 2 to n:
Set c to a + b
Set a to b
Set b to c
Return b
Step 4: Code Implementation
function fibonacci(n) {
if (n === 0) return 0;
if (n === 1) return 1;
let a = 0;
let b = 1;
for (let i = 2; i <= n; i++) {
let c = a + b;
a = b;
b = c;
}
return b;
}
Step 5: Trace Table
Let’s trace this for n = 5:
i | a | b | c |
---|---|---|---|
Initial | 0 | 1 | ? |
2 | 1 | 1 | 1 |
3 | 1 | 2 | 2 |
4 | 2 | 3 | 3 |
5 | 3 | 5 | 5 |
The 5th Fibonacci number is 5, which matches our expected output.
Alternative Solution: Recursion
We could also solve this recursively:
function fibonacciRecursive(n) {
if (n === 0) return 0;
if (n === 1) return 1;
return fibonacciRecursive(n - 1) + fibonacciRecursive(n - 2);
}
This solution is elegant but less efficient. Understanding both approaches and their trade-offs is part of developing programming expertise.
From Beginner to Expert: The Learning Curve
The journey from puzzle solver to programmer typically follows these stages:
Stage 1: Syntax Focus
Beginners focus primarily on syntax and rules. Code feels foreign and requires conscious effort to write. Every problem feels new and unique.
Stage 2: Pattern Recognition
With practice, programmers begin recognizing common patterns. Solutions become more intuitive as mental models develop. The “blank canvas effect” becomes less intimidating.
Stage 3: Fluent Problem Solving
Advanced programmers think directly in terms of algorithms and data structures. Syntax becomes automatic, allowing full focus on problem-solving. The puzzle-programming gap closes as programming itself becomes a kind of puzzle-solving.
Stage 4: Creative Solutions
Experts can develop novel solutions by combining patterns in creative ways. Programming becomes less about following rules and more about creative expression within constraints, similar to how master puzzle solvers develop innovative strategies.
The Role of Community and Collaboration
Programming is rarely a solitary activity in professional settings. Learning to read others’ code, explain your solutions, and collaborate on problems accelerates the development of programming mental models.
Strategies for collaborative learning include:
- Code Reviews: Have others review your code and provide feedback.
- Pair Programming: Work with a partner to solve problems together.
- Study Groups: Discuss solutions to common problems and share insights.
- Online Communities: Participate in forums like Stack Overflow or Reddit’s programming communities.
These collaborative approaches provide exposure to different mental models and problem-solving strategies, accelerating your growth as a programmer.
Applying Puzzle-Solving Strategies to Programming
Many strategies used in traditional puzzles can be adapted for programming:
Edge-First Strategy
In jigsaw puzzles, many people start with the edges to create a framework. In programming, this translates to:
- Define input and output formats
- Write test cases before implementation
- Create function signatures before implementation
This approach provides structure before tackling the complex middle parts.
Divide and Conquer
Breaking large puzzles into manageable sections has a direct programming parallel:
- Break the problem into subproblems
- Solve each subproblem independently
- Combine the solutions
This strategy works for both puzzles and programming challenges.
Pattern Recognition
In puzzles like Sudoku, recognizing patterns (like naked pairs or hidden triples) is key. In programming, recognizing algorithmic patterns serves a similar function:
- Identifying when to use binary search
- Recognizing graph traversal problems
- Spotting opportunities for dynamic programming
Building a mental library of these patterns accelerates problem-solving.
Tools and Resources to Bridge the Gap
Several tools and platforms specifically address the puzzle-programming gap:
Visual Programming Environments
- Scratch: Block-based programming that eliminates syntax concerns
- Python Tutor: Visualizes code execution step-by-step
- Algorithm Visualizers: Show how algorithms work visually
Interactive Learning Platforms
- AlgoCademy: Provides structured progression from basics to advanced algorithms
- CodeSignal: Offers programming challenges with increasing difficulty
- Exercism: Provides mentored programming exercises
Books and Courses
- “Think Like a Programmer” by V. Anton Spraul
- “A Mind for Numbers” by Barbara Oakley
- Harvard’s CS50: Builds mental models alongside coding skills
Conclusion: Embracing the Journey
The gap between solving traditional puzzles and programming challenges is real, but it’s not insurmountable. By understanding the specific differences and deliberately building programming mental models, you can leverage your puzzle-solving abilities in the programming realm.
Remember that programming is itself a form of puzzle-solving, just with different rules and tools. The satisfaction of solving a difficult programming problem can be just as rewarding as completing a challenging puzzle.
Key takeaways for bridging the puzzle-programming gap:
- Explicitly build programming mental models
- Use visualization tools to make abstract concepts concrete
- Break down problems before coding
- Practice tracing code execution manually
- Study patterns and algorithms deliberately
- Embrace collaborative learning
- Be patient with the learning process
With deliberate practice and the right approaches, your puzzle-solving abilities can become the foundation for strong programming skills. The journey from puzzles to programming is a fascinating one, full of “aha!” moments and intellectual growth.
Next time you find yourself struggling with a programming problem, remember to apply the same methodical, patient approach that makes you successful with traditional puzzles. The skills are more transferable than they might initially appear, and with practice, the gap between puzzles and programming will gradually disappear.