Why You Can Solve Problems on Paper But Not in Code
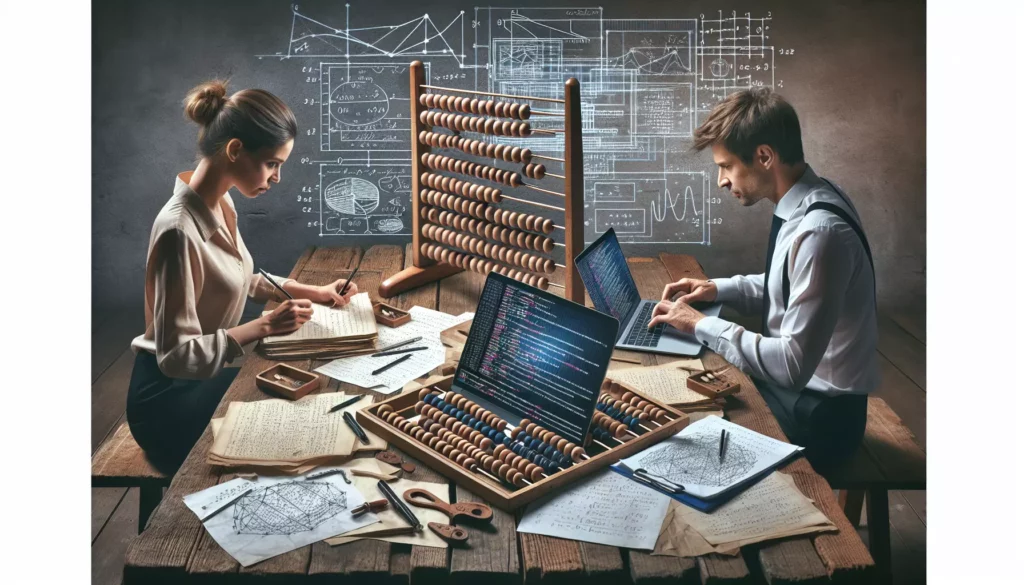
Have you ever experienced the frustration of perfectly understanding a problem, sketching out a solution on paper, only to hit a wall when trying to implement it in code? This disconnect between theoretical understanding and practical implementation is a common hurdle for many programmers, from beginners to experienced developers.
In this comprehensive guide, we’ll explore the gap between conceptual problem-solving and coding implementation, why it exists, and most importantly, how to bridge it. By the end, you’ll have practical strategies to transform your paper solutions into working code more effectively.
The Disconnect Between Theoretical Understanding and Coding Implementation
Let’s start by acknowledging a truth many developers face: understanding a problem and implementing its solution are two distinct skills. This phenomenon has several underlying causes:
Different Cognitive Processes
When solving problems on paper, you engage in high-level abstract thinking. This process is fluid and forgiving. You can make assumptions, use shorthand, and skip implementation details. Your brain fills in the gaps automatically.
In contrast, coding requires precise, detailed instructions that follow strict syntax rules. There’s no room for ambiguity or assumptions. The computer executes exactly what you tell it to, not what you meant to tell it.
The Translation Gap
Converting an abstract solution into concrete code requires translation skills. This translation process introduces complexity that wasn’t present in your conceptual solution. You need to:
- Choose appropriate data structures
- Select efficient algorithms
- Implement correct control flow
- Handle edge cases explicitly
- Follow language-specific syntax and conventions
Each of these steps introduces potential for errors or misalignment with your original vision.
Working Memory Limitations
Our brains have limited working memory capacity. When solving problems on paper, we can offload details to the paper itself, freeing up mental resources for strategic thinking. When coding, we must simultaneously juggle:
- The problem requirements
- Our high-level solution approach
- Programming language syntax
- Variable names and their purposes
- The current state of our implementation
- Potential edge cases
This cognitive load can overwhelm our working memory, leading to mistakes or mental blocks.
Common Scenarios Where Paper Solutions Don’t Translate to Code
Let’s examine some specific situations where this disconnect often manifests:
1. Algorithm Implementation Challenges
You may understand an algorithm conceptually (like binary search or depth-first traversal) but struggle with implementation details like:
- Properly initializing variables
- Setting correct loop conditions
- Managing recursion and base cases
- Handling boundary conditions
For example, a binary search seems straightforward on paper, but in code, off-by-one errors and improper midpoint calculations are common pitfalls.
2. Data Structure Selection and Usage
On paper, you might simplify by saying “use a hash table for O(1) lookups,” but in code you need to:
- Choose the specific implementation (HashMap, Dictionary, object, etc.)
- Initialize it correctly
- Handle collisions or duplicates if necessary
- Understand language-specific behaviors
3. Edge Case Oversight
Your paper solution might address the main problem path but overlook edge cases like:
- Empty inputs
- Single-element inputs
- Maximum or minimum values
- Duplicate values
- Special characters or formatting
These edge cases often become apparent only during implementation or testing.
4. Language-Specific Nuances
Each programming language has unique characteristics that affect implementation:
- Pass-by-value vs. pass-by-reference behavior
- Mutable vs. immutable data types
- Memory management requirements
- Available built-in functions and libraries
Your paper solution likely ignores these details, but your code cannot.
Bridging the Gap: Strategies for Effective Implementation
Now that we understand the challenges, let’s explore practical strategies to overcome them:
1. Develop a Structured Translation Process
Instead of jumping directly from high-level solution to code, create intermediate steps:
Step 1: Create a High-Level Pseudocode Outline
Start with a very high-level pseudocode that captures the core algorithm:
function solve(array):
if array is empty, return appropriate result
initialize necessary variables
perform main algorithm logic
handle edge cases
return result
Step 2: Refine with Implementation Details
Add more specific details while still using pseudocode:
function binarySearch(array, target):
left = 0
right = length of array - 1
while left <= right:
mid = (left + right) / 2
if array[mid] == target:
return mid
else if array[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 // Not found
Step 3: Translate to Actual Code
Now convert to real code with proper syntax:
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (arr[mid] === target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Target not found
}
2. Implement Incrementally with Testing
Instead of writing the entire solution at once, break it down into smaller, testable components:
- Implement the simplest possible version first
- Test thoroughly before adding complexity
- Add one feature or handle one edge case at a time
- Verify each addition works before moving on
This approach reduces cognitive load and makes it easier to identify where issues occur.
3. Visualize Data Transformations
For complex algorithms, track how your data changes at each step:
- Add console logs or use a debugger to visualize state changes
- Create a small example and trace through the execution manually
- Draw out data structures and their transformations
For example, when implementing a merge sort:
function mergeSort(arr) {
console.log("Splitting:", arr);
// Implementation logic
console.log("Merged result:", result);
return result;
}
4. Use Concrete Examples
Work with specific examples throughout your implementation process:
- Choose a simple test case before writing code
- Trace through your algorithm with this example on paper
- Reference this example as you code
- Test your code against this example
- Add more complex examples gradually
This anchors abstract concepts to concrete scenarios, making implementation easier.
Developing Implementation Skills Systematically
Beyond specific strategies for individual problems, you can develop your implementation skills more broadly:
1. Build a Strong Foundation in Programming Fundamentals
Many implementation challenges stem from gaps in fundamental knowledge:
- Master your programming language: Deeply understand syntax, data types, and common patterns in your primary language
- Know your standard library: Familiarize yourself with built-in functions and data structures
- Practice common coding patterns: Implement standard algorithms and data structures from scratch until they become second nature
For example, if you regularly struggle with array manipulations in JavaScript, dedicate time to mastering array methods like map, filter, reduce, and slice.
2. Study Implementation Patterns
Certain patterns appear frequently in coding implementations:
- Two-pointer technique: Used for array problems, string problems, and linked lists
- Sliding window: Efficient for subarray or substring problems
- BFS/DFS traversal: Essential for tree and graph problems
- Dynamic programming templates: Recognition of overlapping subproblems
By studying and practicing these patterns, you develop templates that make implementation more straightforward.
3. Analyze and Learn from Others’ Implementations
When you struggle to implement a solution:
- Look at well-written solutions by others
- Analyze how they structured their approach
- Compare to your paper solution to identify gaps
- Implement their solution from memory to internalize the patterns
This process helps you recognize implementation patterns and techniques you might be missing.
4. Practice Deliberate Implementation
Regular, focused practice is essential:
- Implement the same solution multiple times until it becomes fluent
- Challenge yourself to implement solutions with different approaches
- Set time constraints to simulate interview conditions
- Review and refactor your implementations to identify improvement areas
This deliberate practice builds neural pathways that make implementation more automatic over time.
Common Implementation Pitfalls and How to Avoid Them
Let’s examine specific implementation challenges and their solutions:
1. Off-by-One Errors
These errors occur when you access an array element that’s out of bounds or iterate one too many or too few times.
Prevention strategies:
- Use inclusive/exclusive bounds consistently (e.g., always use < for upper bounds)
- Visualize array indices explicitly (draw them out)
- Test boundary conditions with small examples
- Use descriptive variable names that clarify intent (firstIndex, lastIndex)
// Instead of:
for (let i = 0; i < arr.length; i++) {
// Access arr[i+1] might cause issues
}
// Consider:
for (let i = 0; i < arr.length - 1; i++) {
// Now arr[i+1] is safe
}
2. Initialization Issues
Forgetting to initialize variables or initializing them incorrectly can lead to subtle bugs.
Prevention strategies:
- Initialize variables at declaration time
- Use meaningful default values
- Consider edge cases when choosing initial values
- Comment your initialization choices for complex logic
// Problematic initialization for finding minimum
let min = 0; // What if all values are positive?
// Better initialization
let min = Infinity; // Works for any numeric array
3. Logical Errors in Conditionals
Complex conditional logic often leads to implementation errors.
Prevention strategies:
- Break complex conditions into simpler, named parts
- Use truth tables for complicated logic
- Test each condition independently
- Consider De Morgan’s laws for simplification
// Complex conditional
if (a < b && (c > d || e === f)) {
// Logic
}
// More readable version
const firstCondition = a < b;
const secondCondition = c > d || e === f;
if (firstCondition && secondCondition) {
// Logic
}
4. Recursion Implementation Challenges
Recursive solutions often work well on paper but fail in implementation due to missing base cases or incorrect recursive calls.
Prevention strategies:
- Always start with clearly defined base cases
- Ensure the recursive call moves toward the base case
- Trace a simple example through each recursive call
- Be mindful of stack limitations for deep recursion
function factorial(n) {
// Base case first
if (n === 0 || n === 1) {
return 1;
}
// Recursive case moves toward base case
return n * factorial(n - 1);
}
Language-Specific Implementation Considerations
Different programming languages have unique characteristics that affect implementation:
JavaScript
Common challenges:
- Type coercion leading to unexpected behavior
- Reference vs. value semantics for objects and arrays
- Asynchronous code implementation
- Scope and closure complexities
Implementation tips:
// Use strict equality to avoid type coercion issues
if (value === 5) rather than if (value == 5)
// Clone objects/arrays to avoid unintended mutations
const newArray = [...originalArray];
const newObject = {...originalObject};
// Use descriptive variable names to clarify types
const userIdString = "12345";
const userIdNumber = 12345;
Python
Common challenges:
- Indentation-based syntax errors
- Mutable default arguments
- List comprehension readability
- Generator vs. list behavior differences
Implementation tips:
# Avoid mutable default arguments
def function(data=None):
if data is None:
data = []
# Function logic
# Balance between list comprehension and readability
# Simple comprehension:
squares = [x*x for x in range(10)]
# More complex logic might be better as a loop:
result = []
for x in range(10):
if x % 2 == 0:
result.append(x*x)
else:
result.append(x+1)
Java
Common challenges:
- Verbose syntax for collections and generics
- Primitive vs. wrapper type confusion
- Null pointer exceptions
- Boilerplate code requirements
Implementation tips:
// Use diamond operator for cleaner generics
Map<String, List<Integer>> map = new HashMap<>();
// Check for null to prevent NullPointerException
if (object != null && object.property.equals("value")) {
// Logic
}
// Consider using Optional for nullable returns
public Optional<User> findUser(String id) {
// Implementation
}
Practical Exercises to Improve Implementation Skills
Here are structured exercises to bridge the gap between paper solutions and code:
Exercise 1: Translation Practice
- Find algorithm descriptions in textbooks or online resources
- Write a high-level pseudocode version
- Create a detailed pseudocode implementation
- Translate to actual code
- Test with various inputs
- Compare your implementation with reference solutions
Start with algorithms like binary search, merge sort, or breadth-first search.
Exercise 2: Incremental Implementation
- Choose a medium-complexity problem
- Break it down into 5-7 implementation steps
- Implement one step at a time
- Test each step thoroughly before proceeding
- Refactor for clarity after completing all steps
This teaches you to manage complexity incrementally rather than all at once.
Exercise 3: Implementation Variants
- Implement the same algorithm in multiple ways (iterative vs. recursive, different data structures)
- Implement the same algorithm in different programming languages
- Compare the implementations for readability and efficiency
This develops flexibility in your implementation thinking.
Exercise 4: Debug and Fix
- Find implementations with bugs (from websites like LeetCode discussions)
- Identify and fix the issues
- Document the types of bugs you find most frequently
- Create a personal checklist of common implementation errors to watch for
This builds your debugging skills and error recognition.
Real-world Examples: From Paper to Code
Let’s walk through a complete example of translating a paper solution to code:
Problem: Find the Longest Substring Without Repeating Characters
Paper Solution:
“Use a sliding window approach. Keep track of characters we’ve seen in the current window using a set. Expand the window to the right as long as we don’t see duplicates. When we encounter a duplicate, shrink the window from the left until we remove the duplicate. Track the maximum window size seen.”
Step 1: High-level Pseudocode
function lengthOfLongestSubstring(s):
initialize data structures
use sliding window approach
track maximum length
return result
Step 2: Detailed Pseudocode
function lengthOfLongestSubstring(s):
if s is empty, return 0
create empty set for characters
left = 0, right = 0, maxLength = 0
while right < length of s:
if s[right] is not in set:
add s[right] to set
update maxLength if current window is longer
right++
else:
remove s[left] from set
left++
return maxLength
Step 3: JavaScript Implementation
function lengthOfLongestSubstring(s) {
if (s.length === 0) return 0;
const charSet = new Set();
let left = 0;
let right = 0;
let maxLength = 0;
while (right < s.length) {
if (!charSet.has(s[right])) {
charSet.add(s[right]);
maxLength = Math.max(maxLength, right - left + 1);
right++;
} else {
charSet.delete(s[left]);
left++;
}
}
return maxLength;
}
Step 4: Test with Examples
- Input: "abcabcbb" → Output: 3 ("abc")
- Input: "bbbbb" → Output: 1 ("b")
- Input: "pwwkew" → Output: 3 ("wke")
- Input: "" → Output: 0
Step 5: Identify and Fix Issues
Testing reveals an issue: when we encounter a duplicate, we might be removing characters that aren't the actual duplicate. Let's fix:
function lengthOfLongestSubstring(s) {
if (s.length === 0) return 0;
const charMap = new Map(); // Change to Map to track positions
let left = 0;
let maxLength = 0;
for (let right = 0; right < s.length; right++) {
if (charMap.has(s[right])) {
// Move left pointer to position after the duplicate
left = Math.max(left, charMap.get(s[right]) + 1);
}
charMap.set(s[right], right);
maxLength = Math.max(maxLength, right - left + 1);
}
return maxLength;
}
This example demonstrates the complete translation process from concept to working code, including refinement based on testing.
The Role of Modern Tools in Implementation
Modern development tools can significantly aid the implementation process:
1. Integrated Development Environments (IDEs)
IDEs offer features that reduce implementation friction:
- Syntax highlighting and error detection
- Code completion and suggestions
- Automated refactoring tools
- Integrated debugging capabilities
Leverage these features to focus more on logic and less on syntax details.
2. AI Coding Assistants
Tools like GitHub Copilot, ChatGPT, and other AI assistants can help with implementation:
- Generate boilerplate code
- Suggest implementations based on comments
- Help debug issues
- Explain unfamiliar code or concepts
Use these tools as implementation aids, but ensure you understand the generated code.
3. Visualization Tools
Visualization can bridge the gap between concept and code:
- Algorithm visualizers (like visualgo.net)
- Code execution visualizers (like Python Tutor)
- Debugger visualizations
These tools help you see what's happening in your code, making it easier to align with your mental model.
4. Testing Frameworks
Systematic testing validates your implementation:
- Unit testing frameworks
- Property-based testing tools
- Test-driven development approaches
Writing tests before or alongside implementation helps clarify requirements and verify correctness.
Conclusion: Closing the Gap Between Concept and Code
The ability to translate conceptual solutions into working code is a skill that improves with deliberate practice and the right approach. By understanding why this gap exists and applying structured strategies to bridge it, you can become more effective at implementation.
Remember these key takeaways:
- Translation is a distinct skill that requires practice separate from problem-solving
- Structured implementation processes (high-level pseudocode → detailed pseudocode → code) reduce cognitive load
- Incremental implementation with testing prevents overwhelming complexity
- Visualization and concrete examples help align your mental model with the code
- Language mastery and pattern recognition make implementation more automatic over time
With consistent practice of these techniques, you'll find that the gap between your paper solutions and code implementations gradually narrows. The frustration of "I know how to solve it but can't code it" will become less frequent, replaced by the satisfaction of bringing your solutions to life through code.
The journey from concept to code is a fundamental part of programming mastery. By deliberately developing your implementation skills, you're not just becoming better at coding challenges—you're becoming a more effective problem solver and software developer overall.