Why You Can Read Code But Freeze When Facing a Blank Editor
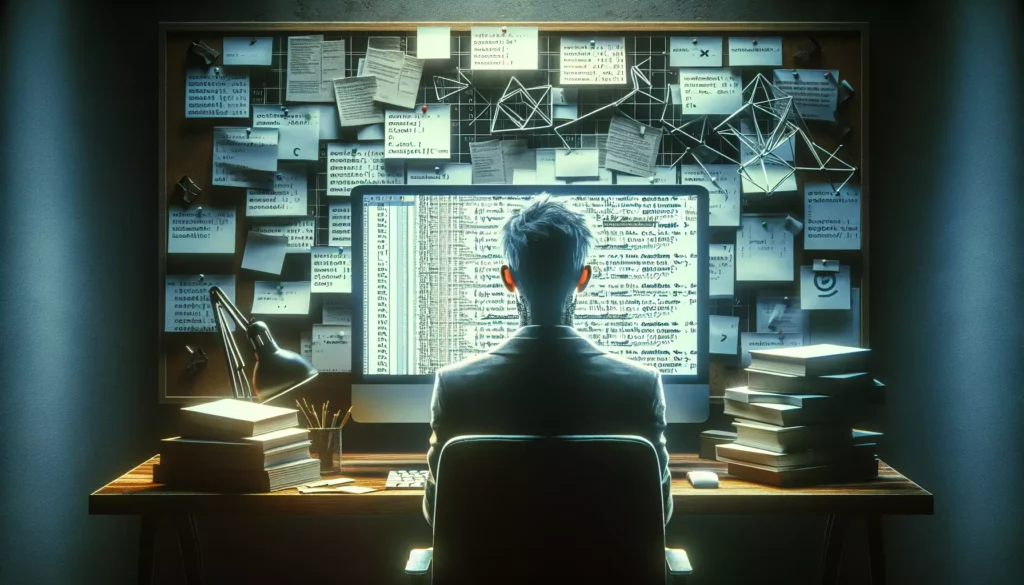
Have you ever found yourself confidently reading through lines of code, understanding the logic, nodding along with the implementation, only to freeze completely when faced with a blank editor? This phenomenon is surprisingly common, even among experienced developers. You’re not alone in this struggle, and there are valid psychological and cognitive reasons behind it.
In this article, we’ll explore why reading code and writing code activate different mental processes, why the blank editor can be so intimidating, and most importantly, how to overcome this hurdle in your programming journey.
The Reading vs. Writing Code Paradox
Reading code and writing code are fundamentally different cognitive processes, much like how reading a book differs from writing one. Let’s break down why these activities engage different parts of your brain.
Why Reading Code Can Feel Easier
When you read code, you’re engaging in a recognition task. Your brain is processing information that’s already structured and organized. Several factors make reading code more approachable:
- Pattern Recognition: As you gain experience, you begin to recognize common coding patterns and structures.
- Context is Provided: The code exists within a framework that guides your understanding.
- Passive Engagement: Reading is relatively passive; you can follow along at your own pace.
- Familiarity Bias: When reading code that others have written, it’s easy to think, “I could have done that” because the solution is already presented to you.
Consider this Python function that calculates the Fibonacci sequence:
def fibonacci(n):
if n <= 0:
return []
elif n == 1:
return [0]
elif n == 2:
return [0, 1]
fib_list = [0, 1]
for i in range(2, n):
fib_list.append(fib_list[i-1] + fib_list[i-2])
return fib_list
When reading this, you can quickly understand the logic: check for edge cases, initialize the sequence, and then build it iteratively. The structure guides your comprehension.
Why Writing Code Can Feel Harder
Writing code, on the other hand, is a production task that requires different mental resources:
- Generation vs. Recognition: You must generate solutions rather than recognize them.
- Decision Fatigue: Every line requires multiple decisions about implementation, naming, structure, and optimization.
- Working Memory Limitations: You need to hold multiple variables, functions, and their relationships in your mind simultaneously.
- Perfectionism Paralysis: The desire to write “perfect” code can prevent you from writing any code at all.
When facing a blank editor and being asked to write a Fibonacci function from scratch, you might find yourself stuck on questions like:
- “Should I use recursion or iteration?”
- “What’s the most efficient approach?”
- “How do I handle edge cases?”
- “What should I name my variables?”
The Psychology Behind the Blank Editor Freeze
That intimidating blank editor represents more than just an empty workspace; it triggers several psychological responses that can impede your ability to start coding.
The Blank Page Syndrome
Writers have struggled with “blank page syndrome” for centuries, and programmers face the same challenge. The empty editor represents infinite possibilities, which paradoxically can be paralyzing rather than liberating. This phenomenon has several psychological components:
- Decision Overload: Too many possible approaches can lead to decision paralysis.
- Fear of Commitment: Once you start typing, you’re committing to a particular approach.
- Perfectionism: The blank editor holds the potential for perfect code, while any actual code you write will inevitably have flaws.
Imposter Syndrome’s Role
Imposter syndrome hits particularly hard when facing a blank editor because it’s the moment when your skills are put to the test. Thoughts like these might emerge:
- “Real programmers wouldn’t struggle with this.”
- “I should be able to solve this immediately.”
- “Everyone else would probably find this easy.”
This internal dialogue creates performance anxiety that further inhibits your ability to think clearly and code effectively.
The Performance Pressure
Whether you’re coding in an interview, a classroom, or even just practicing at home with self-imposed expectations, performance pressure can significantly impact your coding abilities:
- Working Memory Reduction: Anxiety literally reduces your available working memory, making complex problem-solving more difficult.
- Time Pressure: Real or perceived time constraints amplify anxiety.
- Fear of Evaluation: Knowing your code might be judged (by others or yourself) adds another layer of pressure.
The Cognitive Load of Writing Code
From a cognitive science perspective, writing code places substantial demands on your brain’s processing capabilities.
Working Memory Limitations
Humans have limited working memory capacity, typically able to hold only about 4-7 items in mind simultaneously. When coding, you’re juggling multiple elements:
- The problem requirements
- Potential algorithms and data structures
- Syntax rules
- Variable names and their purposes
- The current state of your solution
- Edge cases to handle
This cognitive load can quickly exceed your working memory capacity, especially under pressure.
Context Switching Costs
When writing code, you constantly switch between different levels of abstraction:
- High-level architecture and algorithm design
- Mid-level function organization and data flow
- Low-level syntax and implementation details
Each context switch has a cognitive cost, draining mental resources that could otherwise be used for problem-solving.
Cognitive Bias: The Curse of Knowledge
Experienced programmers often struggle with what’s called “the curse of knowledge” – once you know how to solve a problem, it becomes difficult to remember how challenging it was before you knew the solution. This can lead to:
- Underestimating the difficulty of problems
- Expecting solutions to come quickly
- Being overly critical of your own learning process
The Gap Between Reading and Writing Skills
In language acquisition, there’s a well-documented gap between receptive skills (reading/listening) and productive skills (writing/speaking). The same principle applies to coding.
The Natural Learning Progression
In almost all domains, humans develop receptive skills before productive ones:
- Children understand words before they can speak them
- Language learners can read a foreign language before writing fluently in it
- Musicians can recognize chords before playing them
Programming follows this same pattern. Reading and understanding code naturally comes before fluent code production.
Recognition vs. Recall Memory
From a memory perspective, recognition (identifying something you’ve seen before) is easier than recall (retrieving information from memory without prompts). When reading code, you’re using recognition memory; when writing code, you’re relying on recall memory.
Consider this JavaScript example:
// When reading this code, you can easily recognize what it does
function isPalindrome(str) {
const cleanStr = str.toLowerCase().replace(/[^a-z0-9]/g, '');
return cleanStr === cleanStr.split('').reverse().join('');
}
You might immediately recognize this as a palindrome checker and understand how it works. But when asked to write a palindrome checker from scratch, you need to recall the exact syntax for string manipulation, regular expressions, and the algorithm itself.
Bridging the Gap: Strategies to Overcome Blank Editor Freeze
Now for the good news: there are concrete strategies you can use to overcome blank editor freeze and build your code-writing confidence.
Start with Pseudocode
Instead of diving straight into coding, begin with pseudocode – a plain language description of your approach:
// Problem: Create a function that finds the maximum value in an array
// Pseudocode:
// 1. Initialize a variable to store the maximum value (set to first element)
// 2. Loop through each element in the array
// 3. If current element is greater than current maximum, update maximum
// 4. Return the maximum value after the loop completes
This reduces cognitive load by allowing you to focus on the algorithm before worrying about syntax.
The Skeleton Approach
Start by creating a bare-bones structure of your solution and then fill in the details:
function findMaxValue(arr) {
// Edge case handling
if (!arr || arr.length === 0) {
return null;
}
// Variable initialization
let max = arr[0];
// Main logic
// TODO: Loop through array and find maximum
// Return result
return max;
}
This approach gives you a framework to build upon, making the task less overwhelming.
Incremental Development
Build your solution in small, testable increments:
- Write the function signature and handle edge cases
- Implement the core logic for a simple case
- Test with basic inputs
- Add handling for more complex cases
- Refine and optimize
This approach allows you to see progress and maintain momentum.
Deliberate Practice Techniques
To build your code-writing skills, try these deliberate practice techniques:
1. Code Implementation Exercises
After reading code, challenge yourself to reimplement it without looking at the original. This bridges the gap between reading and writing.
2. Time-Boxed Challenges
Set a timer for 25 minutes and focus solely on making progress on a coding problem. This limits perfectionism and builds the habit of starting.
3. Reverse Engineering
Take working code and break it down, then rebuild it from memory. This helps internalize patterns and approaches.
4. Pair Programming
Coding with a partner can reduce the pressure of the blank editor by making it a collaborative process rather than a solo performance.
Building a Personal Code Library
Develop a repository of code snippets and patterns that you can reference when starting new projects:
- Common algorithms you’ve implemented
- Useful utility functions
- Project starter templates
- Function patterns you use frequently
Having these resources available reduces the need to start completely from scratch.
The Role of Knowledge Gaps
Sometimes, blank editor freeze isn’t just psychological; it can indicate actual knowledge gaps that need addressing.
Identifying Conceptual vs. Implementation Gaps
When you struggle to write code, determine whether you’re facing:
- Conceptual Gaps: Incomplete understanding of the underlying concepts (algorithms, data structures, programming paradigms)
- Implementation Gaps: Difficulty translating concepts into working code (syntax, language features, library usage)
For example, you might understand the concept of recursion but struggle to implement a recursive function correctly.
Strategic Learning to Fill Gaps
Once you’ve identified your gaps, target your learning:
- For Conceptual Gaps: Focus on understanding the principles through explanations, visualizations, and examples.
- For Implementation Gaps: Practice writing code, refer to documentation, and study code examples that demonstrate the correct implementation.
The Importance of Syntax Fluency
One common reason for blank editor freeze is simply not having automated recall of common syntax patterns. Building syntax fluency through repetition can significantly reduce this friction:
- Practice typing common code structures until they become muscle memory
- Create flashcards for syntax patterns you frequently forget
- Do typing exercises with code examples to build speed and accuracy
Practical Exercises to Build Code Writing Confidence
Let’s explore some specific exercises designed to bridge the gap between reading and writing code.
Exercise 1: Code Reading to Writing Transition
- Read a function carefully and make sure you understand how it works
- Close the function or switch to a different window
- Try to rewrite the function from memory
- Compare your version with the original and note differences
Start with simple functions and gradually increase complexity.
Exercise 2: Function Signature First
- When given a problem, immediately write the function signature with parameter names
- Add comments describing what the function should do
- Write pseudocode for the implementation
- Translate pseudocode to actual code one section at a time
For example:
/**
* Merges two sorted arrays into a single sorted array
* @param {number[]} arr1 - First sorted array
* @param {number[]} arr2 - Second sorted array
* @return {number[]} - Merged sorted array
*/
function mergeSortedArrays(arr1, arr2) {
// Initialize result array and pointers
// While both arrays have elements to process
// Handle remaining elements
// Return result
}
Exercise 3: Implement Multiple Approaches
For a single problem, challenge yourself to implement multiple solutions:
- First, implement the simplest solution that works (brute force)
- Then implement a more optimized solution
- Finally, try an alternative approach using different techniques
This builds flexibility in your thinking and reduces attachment to finding the “one right way.”
Exercise 4: Code Expansion
Start with a minimal implementation and gradually expand it:
// Step 1: Basic implementation
function search(arr, target) {
for (let i = 0; i < arr.length; i++) {
if (arr[i] === target) return i;
}
return -1;
}
// Step 2: Add error handling
function search(arr, target) {
if (!Array.isArray(arr)) throw new Error("Input must be an array");
for (let i = 0; i < arr.length; i++) {
if (arr[i] === target) return i;
}
return -1;
}
// Step 3: Add optimization for sorted arrays
function search(arr, target) {
if (!Array.isArray(arr)) throw new Error("Input must be an array");
// Use binary search if array is sorted
if (isSorted(arr)) {
return binarySearch(arr, target);
}
// Fall back to linear search
for (let i = 0; i < arr.length; i++) {
if (arr[i] === target) return i;
}
return -1;
}
The Psychology of Progress
Overcoming blank editor freeze isn't just about technical skills; it's also about managing your psychology and building confidence through incremental progress.
Embracing the Learning Curve
Recognize that the gap between reading and writing code is a normal part of the learning process, not a reflection of your capability as a programmer. Every experienced developer has gone through this stage.
The Power of Small Wins
Research in psychology shows that small wins create momentum and motivation. Rather than focusing on writing perfect code immediately:
- Celebrate getting the first function written, even if it's not optimal
- Acknowledge progress in your problem-solving approach
- Keep a "done list" of coding tasks you've completed
Reframing Mistakes as Learning
When you write code that doesn't work as expected, view it as valuable feedback rather than failure:
- "This error message is giving me information about what to fix next."
- "Each bug I encounter teaches me something about how this language works."
- "Making mistakes now will help me avoid them in more complex projects."
Tools and Resources to Help You Start Coding
Leverage these tools and resources to reduce the friction of starting to code:
Code Editors with Helpful Features
- Snippets and Templates: Most modern IDEs allow you to create and use code snippets that can be quickly inserted.
- IntelliSense and Autocompletion: These features can suggest syntax and methods as you type, reducing recall burden.
- Linters and Formatters: These tools can catch errors and format your code automatically, reducing concerns about syntax.
Interactive Learning Platforms
Platforms like AlgoCademy provide structured environments where you can practice coding with guidance:
- Step-by-step problem breakdowns
- Interactive coding exercises with immediate feedback
- AI-powered assistance when you get stuck
Scaffolding Resources
Use these resources to provide structure when starting new projects:
- Project starter templates
- Boilerplate generators
- Framework CLI tools that generate project structures
From Reading to Writing: A Progressive Path
Let's outline a progressive path to move from being comfortable with reading code to confidently writing it.
Stage 1: Active Reading
Begin by making your code reading more active:
- Predict what each line will do before reading the next one
- Manually trace code execution with different inputs
- Explain the code aloud as if teaching someone else
- Add your own comments explaining how the code works
Stage 2: Modification Exercises
Next, practice modifying existing code:
- Fix bugs in working code
- Add new features to existing functions
- Refactor code to improve readability or performance
- Adapt code to handle additional edge cases
Stage 3: Guided Creation
Progress to creating code with scaffolding:
- Complete partially written functions
- Implement functions based on detailed pseudocode
- Follow step-by-step tutorials, typing the code yourself
- Use test-driven development, where tests guide your implementation
Stage 4: Independent Creation
Finally, move to creating code independently:
- Start with well-defined, small problems
- Use the strategies discussed earlier (pseudocode, skeleton approach, etc.)
- Gradually tackle more complex and open-ended problems
- Practice explaining your code and design decisions to others
Real-world Programming vs. Interview Coding
It's worth noting that the blank editor freeze can be particularly acute in technical interviews, which differ from day-to-day programming in several ways.
Different Contexts, Different Pressures
Real-world Programming | Interview Coding |
---|---|
Access to documentation and resources | Limited or no access to references |
Collaboration with team members | Solo performance under observation |
Extended time for problem-solving | Strict time constraints |
Incremental progress over days/weeks | Complete solution needed in minutes/hours |
Specific Strategies for Interview Settings
If you're preparing for technical interviews, these additional strategies can help:
- Verbalize Your Thinking: Practice explaining your approach before and during coding.
- Time-Boxed Practice: Regularly practice solving problems under timed conditions.
- Mock Interviews: Simulate the interview environment with a friend or using online platforms.
- Problem Pattern Recognition: Study common problem types to recognize patterns quickly.
Conclusion: Embracing the Journey from Reader to Writer
The gap between reading and writing code is a natural part of the programming learning curve. Rather than being discouraged by blank editor freeze, recognize it as a sign that you're pushing yourself to grow as a developer.
Remember these key takeaways:
- Reading and writing code use different cognitive processes; it's normal for writing to be more challenging.
- The blank editor represents infinite possibilities, which can be paralyzing rather than liberating.
- Specific strategies like pseudocode, skeleton approaches, and incremental development can help overcome the initial hurdle.
- Building your skills is a progressive journey that takes time and deliberate practice.
- Every experienced programmer has faced this challenge and overcome it through consistent practice.
By understanding the psychological and cognitive factors at play and applying the strategies outlined in this article, you can build the confidence and skills to transform from someone who can read code to someone who can confidently write it.
The next time you face a blank editor, remember: every line of code ever written started with an empty file and a programmer who took that first step of typing something, anything, to begin the journey.