Why You Can Follow Tutorials But Struggle with Real Coding Problems
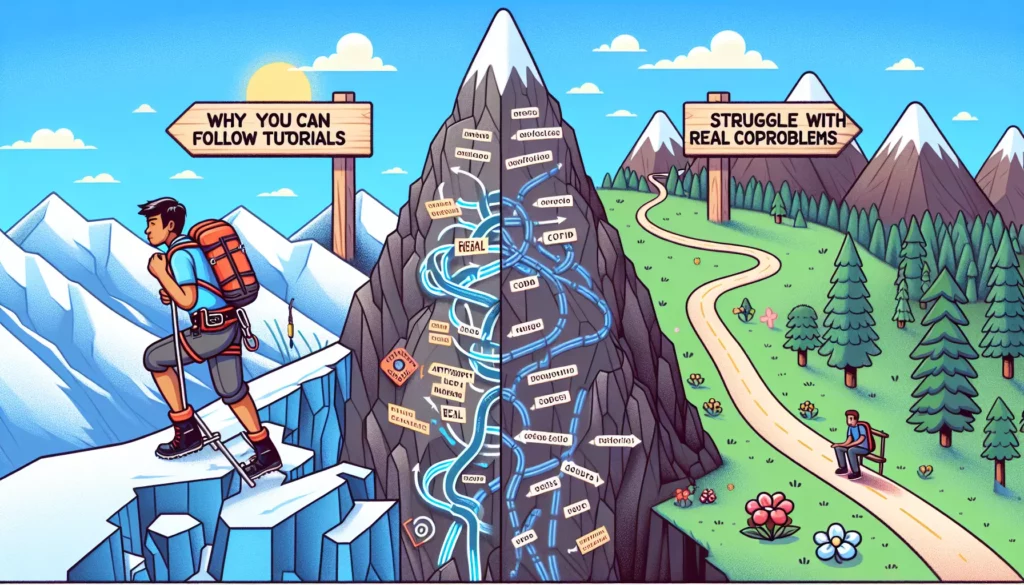
Have you ever felt confident after completing a tutorial, only to freeze when faced with a real coding problem? This common experience frustrates many aspiring programmers. You’re not alone in this struggle, and there are good reasons why tutorials often don’t prepare us for authentic programming challenges.
In this comprehensive guide, we’ll explore the disconnect between tutorial learning and real problem solving, and provide actionable strategies to bridge this gap in your programming journey.
The Tutorial Comfort Zone: Why It Feels So Good
Tutorials provide a structured, guided path through coding concepts. They’re designed to make learning accessible and to build confidence. Here’s why they feel comfortable:
Everything Is Prepared for You
In a well-designed tutorial:
- The problem is clearly defined
- Required knowledge is introduced just before you need it
- Code snippets often build incrementally
- Solutions are immediately available if you get stuck
This scaffolding creates a smooth learning experience. The tutorial author has carefully removed obstacles that might frustrate you, allowing you to focus on the specific concept being taught.
Immediate Feedback Loop
Tutorials typically provide instant validation. You write code, run it, and immediately see if it works as expected. This rapid feedback loop is satisfying and reinforces learning. You know exactly when you’ve succeeded and can move forward with confidence.
Narrow Focus on Specific Concepts
Good tutorials isolate individual concepts, teaching them in a vacuum before combining them with other ideas. This makes learning manageable but doesn’t reflect the complexity of real-world coding where multiple concepts intertwine.
A JavaScript array methods tutorial might show you:
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map(num => num * 2);
console.log(doubled); // [2, 4, 6, 8, 10]
This is clean, focused, and builds confidence. But real-world problems rarely present themselves in such a neatly packaged way.
The Reality Shock: Why Real Problems Feel Different
When faced with a blank editor and a real problem, everything changes. Let’s examine why this transition is so jarring:
Ambiguous Problem Statements
Real problems often come with unclear requirements or multiple interpretations. Unlike tutorials where the goal is explicit, real-world problems might require you to:
- Define what success looks like
- Determine edge cases yourself
- Make assumptions about implementation details
- Decide which constraints matter most
This ambiguity can be paralyzing when you’re used to clear instructions.
The Blank Canvas Problem
Perhaps the most intimidating aspect of real coding is starting from scratch. Without scaffolding code or hints about which approach to take, you face what I call “the blank canvas problem” – the anxiety of deciding where to begin.
In tutorials, you might be told:
// Create a function that takes an array and returns the sum
function sumArray(arr) {
// Your code here
}
But in real scenarios, you must:
- Decide what functions to create
- Determine appropriate data structures
- Design the overall architecture
- Plan how components will interact
Integration Complexity
Real projects require integrating multiple concepts, libraries, and systems. Tutorials typically focus on isolated concepts, whereas actual coding requires:
- Combining different programming patterns
- Managing dependencies between components
- Understanding how your code fits into larger systems
- Dealing with technical constraints not mentioned in tutorials
This integration complexity often catches new programmers off guard.
No Clear Path to the Solution
In tutorials, there’s usually one intended solution the author guides you toward. Real problems can be solved multiple ways, each with different tradeoffs. This freedom is both liberating and overwhelming.
When building a real application feature, you might need to decide between:
- Optimizing for performance vs. readability
- Using a simple approach now vs. a more scalable solution
- Following common patterns vs. creating custom solutions
These decisions require judgment that tutorials rarely help develop.
The Missing Skills: What Tutorials Don’t Teach
Understanding the gap between tutorials and real problem-solving reveals several critical skills that tutorials often fail to develop:
Problem Decomposition
Breaking down complex problems into manageable pieces is perhaps the most fundamental programming skill. Tutorials decompose problems for you, robbing you of the opportunity to practice this essential skill.
Real problem-solving requires:
- Identifying the core components of a problem
- Determining dependencies between components
- Creating a logical sequence for tackling each piece
- Recognizing when a problem contains familiar subproblems
Mental Model Building
Effective programmers build mental models of how systems work. These models allow them to reason about code behavior and predict outcomes. Tutorials often focus on syntax and specific implementations without helping you develop these deeper mental models.
For example, a React tutorial might teach you to write:
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
But it might not help you develop a mental model of how React’s rendering cycle works, how state updates trigger re-renders, or the implications of closure in this context.
Debugging and Troubleshooting
Tutorials rarely go wrong. The code works as expected, and when errors are introduced, they’re typically simple and immediately addressed. Real coding involves:
- Diagnosing unexpected behavior
- Tracing through complex execution paths
- Reading error messages and logs effectively
- Forming and testing hypotheses about what’s wrong
- Using debugging tools beyond simple console.log statements
These troubleshooting skills develop through experience with genuine problems, not through following tutorials.
Research and Resource Navigation
Tutorials provide all necessary information upfront. In contrast, real programming requires knowing:
- How to search for relevant information
- Which documentation sources are reliable
- How to extract what you need from technical documentation
- When to ask for help and how to frame questions effectively
This skill of “learning how to learn” is crucial but seldom taught explicitly in tutorials.
Bridging the Gap: Strategies for Real Problem-Solving
Now that we understand the limitations of tutorial learning, let’s explore practical strategies to develop real problem-solving abilities:
Practice Problem Decomposition
Start by deliberately practicing breaking down problems:
- Write it out: Before coding, write down the steps you’ll need to take in plain language.
- Identify subproblems: Look for familiar patterns within larger problems.
- Create function stubs: Write function signatures and comments before implementation.
For example, if building a todo application, you might decompose it like this:
// 1. Data structure to store todos
// 2. Function to add new todos
function addTodo(text) {
// Implementation will go here
}
// 3. Function to mark todos as complete
function completeTodo(id) {
// Implementation will go here
}
// 4. Function to display todos in the UI
function renderTodos() {
// Implementation will go here
}
// 5. Event handlers for user interactions
Implement Deliberate Practice
Not all practice is equal. Effective practice:
- Pushes your boundaries: Work on problems slightly beyond your current ability.
- Involves reflection: After solving a problem, consider how you could improve your solution.
- Includes variation: Practice similar problems with different constraints or requirements.
Consider using platforms like LeetCode, HackerRank, or Exercism that provide problems with increasing difficulty levels and multiple approaches to solutions.
Build Projects Without Tutorials
The most effective way to bridge the gap is to build projects without following tutorials:
- Start small: Begin with manageable projects that stretch your abilities without overwhelming you.
- Set clear requirements: Define what you want to build before starting.
- Allow yourself to struggle: Resist the urge to immediately search for solutions when stuck.
- Seek feedback: Share your code with others to identify blind spots in your approach.
Some beginner-friendly project ideas include:
- A command-line weather app that fetches data from an API
- A personal portfolio website with responsive design
- A simple calculator with basic operations
- A note-taking application with local storage
Develop Debugging Skills
Strong debugging abilities are crucial for solving real problems:
- Learn to use debugging tools: Familiarize yourself with browser dev tools, IDE debuggers, and logging techniques.
- Practice reading error messages: Don’t just fix errors; understand what they’re telling you.
- Use rubber duck debugging: Explain your code line by line to an imaginary listener (or a rubber duck) to identify logical errors.
Try deliberately introducing bugs into working code and practicing fixing them to build this skill.
Study Others’ Code
Reading high-quality code exposes you to patterns and approaches you might not discover on your own:
- Contribute to open source: Even small contributions can teach you how larger codebases work.
- Read popular libraries: Examine how well-known packages solve common problems.
- Review pull requests: See how experienced developers critique and improve code.
This exposure helps build mental models and expands your problem-solving toolkit.
From Tutorial Follower to Problem Solver: A Progressive Approach
Transitioning from tutorial dependency to independent problem-solving doesn’t happen overnight. Here’s a progressive approach to make this transition smoother:
Stage 1: Guided Projects with Modifications
Begin by following tutorials but deliberately modify the final product:
- Add extra features not covered in the tutorial
- Change the styling or user interface
- Adapt the project to solve a slightly different problem
- Refactor the code to use different approaches
For example, if you complete a tutorial for a weather app that shows the current temperature, extend it to display a 5-day forecast or add geolocation.
Stage 2: Semi-Guided Projects
Next, try projects with partial guidance:
- Follow project outlines without step-by-step instructions
- Use starter templates that provide structure but not implementation
- Work through project-based books that explain concepts but leave implementation details to you
This approach provides enough structure to prevent complete overwhelm while requiring you to solve significant portions independently.
Stage 3: Collaborative Projects
Working with others accelerates growth:
- Join hackathons or coding competitions
- Contribute to open-source projects
- Form or join study groups to build projects together
Collaboration exposes you to different problem-solving approaches and provides built-in feedback on your solutions.
Stage 4: Independent Projects
Finally, take on projects entirely on your own:
- Build solutions to problems you personally experience
- Create portfolio projects that showcase your skills
- Rebuild existing applications to understand how they work
These independent projects develop your ability to define requirements, plan implementation, and overcome obstacles without external guidance.
Common Obstacles and How to Overcome Them
As you transition from tutorials to real problem-solving, you’ll likely encounter several common obstacles:
Analysis Paralysis
The problem: Feeling overwhelmed by too many possible approaches, leading to inaction.
Solution:
- Set a time limit for research and planning
- Commit to implementing the simplest solution first
- Use timeboxing: “I’ll try this approach for 30 minutes, then reassess”
- Remember that any working solution is better than a perfect solution that never gets built
Tutorial Dependency
The problem: The habit of immediately searching for tutorials when faced with new challenges.
Solution:
- Implement a “struggle timer” – try solving problems independently for at least 30 minutes before seeking help
- Use documentation rather than tutorials when possible
- Break the problem into smaller pieces and solve each independently
- Keep a programming journal to track your problem-solving process and build confidence
Imposter Syndrome
The problem: Feeling like you’re not a “real programmer” because you struggle with problems.
Solution:
- Recognize that struggling with problems is part of programming at all levels
- Keep a “win journal” documenting problems you’ve solved
- Join communities where beginners openly discuss challenges
- Remember that even senior developers regularly face problems they don’t immediately know how to solve
Scope Creep
The problem: Taking on projects that are too large or complex, leading to frustration and abandonment.
Solution:
- Define a minimal viable product (MVP) before starting
- Break projects into small, achievable milestones
- Celebrate completing components even if the entire project isn’t finished
- Use the “two pizza rule” – if a project would require more than two pizzas to feed the development team, it’s too big for a learning project
The Role of Algorithmic Thinking in Problem Solving
Developing algorithmic thinking is crucial for bridging the gap between tutorials and real problem-solving. This fundamental skill involves:
Pattern Recognition
Experienced programmers recognize common patterns in problems, allowing them to apply proven solutions. To develop this skill:
- Study classic algorithms and data structures
- Practice categorizing problems by their underlying patterns
- Look for similarities between new problems and ones you’ve solved before
For example, many problems involve searching, sorting, transforming data, or traversing structures. Recognizing these patterns helps identify appropriate approaches.
Logical Reasoning
Breaking down the steps needed to solve a problem requires strong logical reasoning:
- Practice thinking in terms of preconditions and postconditions
- Use flowcharts or pseudocode to map logical flows
- Identify edge cases and boundary conditions
Consider this simple problem: reversing a string. The logical steps might be:
function reverseString(str) {
// 1. Create an empty result string
let reversed = '';
// 2. Iterate through the original string from end to beginning
for (let i = str.length - 1; i >= 0; i--) {
// 3. Add each character to the result
reversed += str[i];
}
// 4. Return the result
return reversed;
}
This logical breakdown makes the solution straightforward, even without a tutorial.
Efficiency Awareness
Understanding algorithmic efficiency helps you evaluate different solutions:
- Learn basic time and space complexity analysis
- Practice identifying bottlenecks in your solutions
- Consider resource constraints relevant to your problem context
For example, the string reversal function above has O(n) time complexity but creates a new string that grows with each iteration. An alternative using array methods might be more efficient:
function reverseString(str) {
return str.split('').reverse().join('');
}
This consideration of efficiency is rarely emphasized in basic tutorials but becomes crucial in real-world problem-solving.
Building a Learning System That Works
To effectively transition from tutorial-dependent learning to independent problem-solving, you need a systematic approach to learning:
The 70-20-10 Learning Framework
Consider allocating your learning time according to this framework:
- 70% Project-Based Learning: Spend most of your time building projects that require applying what you’ve learned.
- 20% Social Learning: Learn from peers, mentors, and communities through code reviews, pair programming, and discussions.
- 10% Structured Learning: Use tutorials, courses, and documentation for acquiring new concepts and filling knowledge gaps.
This balance ensures you develop practical problem-solving skills while continuing to expand your knowledge base.
Spaced Repetition and Interleaving
Effective learning science suggests:
- Spaced Repetition: Revisit concepts at increasing intervals rather than cramming.
- Interleaving: Mix different types of problems rather than focusing on one topic at a time.
For example, instead of spending a week on array methods, then a week on objects, interleave these topics by working on projects that require both, revisiting each concept multiple times in different contexts.
Reflection and Metacognition
Regular reflection accelerates learning:
- Keep a coding journal documenting challenges and solutions
- Review completed projects to identify patterns in your problem-solving approach
- Ask yourself what worked, what didn’t, and why
- Set specific learning goals based on identified weaknesses
This metacognitive practice helps you become more aware of your thinking processes and more intentional about improving them.
Conclusion: Embracing the Struggle
The gap between following tutorials and solving real coding problems is natural and expected. Rather than seeing this gap as a failure, recognize it as an essential part of the learning journey.
Remember these key takeaways:
- Struggle is productive: The mental effort of wrestling with problems builds neural pathways that tutorials alone cannot create.
- Problem-solving is a skill: Like any skill, it improves with deliberate practice and reflection.
- Balance is essential: Combine tutorials, guided projects, and independent problem-solving in a learning system that works for you.
- Community accelerates growth: Connect with other learners to share challenges, solutions, and encouragement.
As you continue your programming journey, embrace the discomfort of real problem-solving. The struggle isn’t an obstacle to becoming a programmer—it’s the path itself.
The most valuable skill you can develop isn’t syntax knowledge or familiarity with specific libraries. It’s the ability to face an unfamiliar problem, break it down methodically, and work through it step by step until you reach a solution. This is the essence of programming, and it’s a skill that grows stronger with every problem you tackle.
So the next time you find yourself staring at a blank editor, feeling lost without the guidance of a tutorial, remember: this is where the real learning happens. Embrace the challenge, trust your problem-solving process, and take that first step toward a solution. Your future self will thank you for it.