Why You Can Explain Recursion But Can’t Identify When to Use It
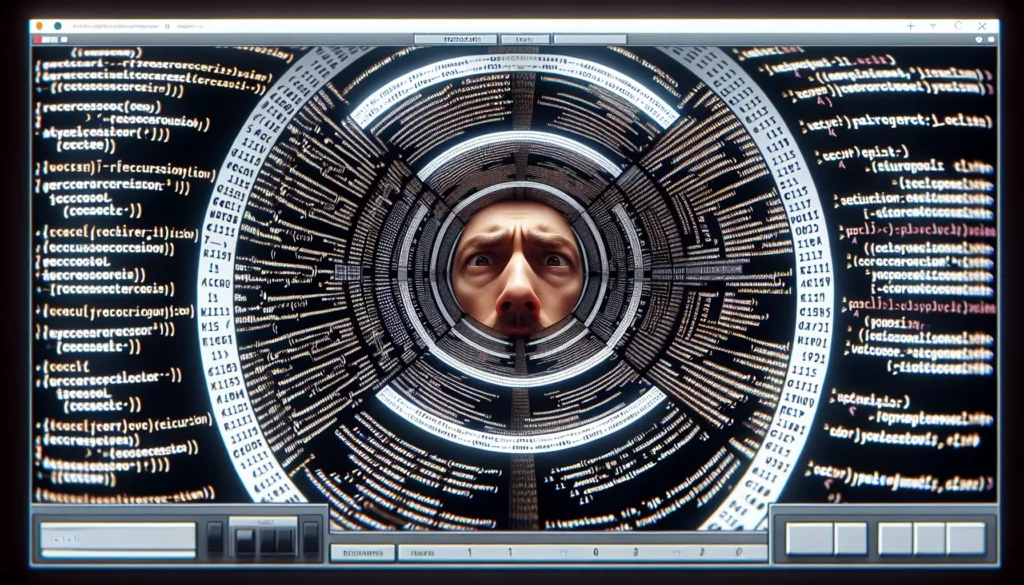
Recursion is one of those programming concepts that many developers can define perfectly, yet struggle to apply effectively in real-world situations. It’s like knowing all the rules of chess but still finding it difficult to develop a winning strategy. This disconnect between understanding recursion conceptually and knowing when to implement it represents a fascinating gap in programming education.
In this article, we’ll explore why many programmers can explain recursion beautifully but freeze when faced with problems where recursion might be the optimal solution. We’ll also provide practical guidelines to help you bridge this gap and develop an intuition for recursive thinking.
The Recursion Paradox
Most programmers can recite the definition of recursion: a function that calls itself to solve smaller instances of the same problem until reaching a base case. Many can even joke about recursion being defined in terms of recursion. Yet when faced with a new problem, the same programmers might resort to iterative solutions even when recursion would be more elegant.
This paradox stems from several factors:
- Recursion requires a different mental model than the imperative thinking most programmers are accustomed to
- There’s a perceived performance penalty associated with recursion
- Identifying recursive substructures in problems isn’t intuitive for many
- The fear of stack overflows and infinite recursion creates hesitation
Let’s break down each of these barriers and see how we can overcome them.
Understanding vs. Application: The Knowledge Gap
When we learn recursion, we typically start with classic examples like factorial calculations or Fibonacci sequences:
// Factorial example
function factorial(n) {
if (n <= 1) return 1;
return n * factorial(n-1);
}
// Fibonacci example
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n-1) + fibonacci(n-2);
}
These examples are excellent for understanding the mechanics of recursion, but they don't necessarily help develop the intuition for identifying when recursion is appropriate in novel situations. It's similar to how knowing the rules of a language doesn't automatically make you fluent in conversation.
The gap exists because:
- Pattern recognition skills develop separately from conceptual understanding - Recognizing that a problem has a recursive structure requires pattern-matching abilities that develop through exposure to many examples.
- Teaching focuses on mechanics over intuition - Most educational resources explain how recursion works, not how to spot opportunities to use it.
- Real-world problems rarely present themselves in textbook format - Unlike academic examples, practical programming challenges don't come with labels indicating the appropriate solution approach.
The Mental Model Mismatch
Most programming beginners learn to think imperatively: do this, then do that, repeat until done. This step-by-step thinking aligns naturally with how we perform tasks in the physical world. Recursion, however, requires a declarative, self-referential mindset that can feel counterintuitive.
Consider how we might explain sorting a deck of cards:
- Iterative explanation: "Compare adjacent cards, swap if needed, and repeat until no more swaps are necessary."
- Recursive explanation: "To sort n cards, sort n-1 cards and then insert the last card in the correct position."
The iterative approach maps more closely to how we'd physically sort cards, while the recursive approach requires a leap of faith that the subproblem (sorting n-1 cards) will somehow be solved.
This mental model mismatch makes it challenging to intuitively reach for recursion when solving new problems, even if you understand the concept perfectly.
Performance Concerns and Optimization Anxiety
Many programmers develop an early aversion to recursion due to warnings about its performance implications:
- Function calls have overhead
- Each recursive call adds a frame to the call stack
- Stack overflows are possible with deep recursion
- Simple recursive implementations of algorithms like Fibonacci have exponential time complexity
These concerns, while valid in certain contexts, create a hesitation that prevents programmers from considering recursive solutions even when appropriate. The reality is more nuanced:
- Many modern languages optimize tail recursion
- The elegance and maintainability of recursive solutions often outweigh minor performance differences
- Techniques like memoization can address performance issues in recursive algorithms
Let's look at a Fibonacci implementation with memoization:
function fibonacciMemoized(n, memo = {}) {
if (n in memo) return memo[n];
if (n <= 1) return n;
memo[n] = fibonacciMemoized(n-1, memo) + fibonacciMemoized(n-2, memo);
return memo[n];
}
This implementation maintains the elegant recursive structure while avoiding the exponential performance pitfall of the naive implementation.
Recognizing Recursive Structures in Problems
Perhaps the most significant challenge is developing the ability to recognize when a problem has a recursive structure. This skill isn't typically taught explicitly but develops through exposure and practice.
Here are some indicators that a problem might be well-suited for recursion:
1. The problem can be broken down into smaller instances of the same problem
This is the most fundamental characteristic of recursively solvable problems. If you can express the solution to a problem in terms of solutions to smaller instances of the same problem, recursion is likely appropriate.
Example: Calculating the sum of an array can be expressed as the first element plus the sum of the rest of the array.
function sum(arr) {
if (arr.length === 0) return 0;
return arr[0] + sum(arr.slice(1));
}
2. The problem involves tree-like or hierarchical structures
Trees are inherently recursive structures, making recursion a natural fit for tree traversal, searching, and manipulation.
Example: Calculating the depth of a binary tree.
function treeDepth(node) {
if (!node) return 0;
return 1 + Math.max(treeDepth(node.left), treeDepth(node.right));
}
3. The problem involves exploring multiple possibilities or paths
Backtracking and search problems often benefit from recursion as they involve exploring different paths and potentially backtracking when a path doesn't lead to a solution.
Example: Finding all possible combinations of elements.
function combinations(elements, k) {
if (k === 0) return [[]];
if (elements.length === 0) return [];
const first = elements[0];
const rest = elements.slice(1);
const combsWithoutFirst = combinations(rest, k);
const combsWithFirst = combinations(rest, k-1)
.map(comb => [first, ...comb]);
return [...combsWithoutFirst, ...combsWithFirst];
}
4. The problem statement includes recursive definitions
Some problems are defined recursively in their statement, making recursion the most natural implementation approach.
Example: The Fibonacci sequence is defined as F(n) = F(n-1) + F(n-2).
Developing Recursive Intuition
Now that we understand the challenges, how can we develop better intuition for when to use recursion? Here are some practical strategies:
1. Practice deliberate decomposition
When approaching a new problem, explicitly ask yourself: "Can I break this down into smaller instances of the same problem?" This simple question can help activate your recursive thinking.
For example, when sorting an array, ask: "If I knew how to sort n-1 elements, could I use that to sort n elements?"
2. Study recursive algorithms in depth
Instead of just memorizing recursive algorithms, deeply understand why recursion is appropriate for them. Some classic examples worth studying include:
- Merge sort and quicksort
- Tree traversal algorithms (preorder, inorder, postorder)
- Graph traversal (depth-first search)
- Dynamic programming problems
For each algorithm, understand:
- What is the base case?
- How is the problem reduced to a smaller instance?
- Why is recursion more elegant than iteration for this problem?
3. Implement recursive and iterative versions of the same algorithm
One of the best exercises is implementing both recursive and iterative solutions to the same problem. This practice helps you see the trade-offs and develops your ability to translate between the two approaches.
For example, let's look at depth-first traversal of a binary tree:
// Recursive DFS
function dfsRecursive(node, values = []) {
if (!node) return values;
values.push(node.value);
dfsRecursive(node.left, values);
dfsRecursive(node.right, values);
return values;
}
// Iterative DFS
function dfsIterative(root) {
if (!root) return [];
const values = [];
const stack = [root];
while (stack.length > 0) {
const node = stack.pop();
values.push(node.value);
if (node.right) stack.push(node.right);
if (node.left) stack.push(node.left);
}
return values;
}
Notice how the iterative version uses an explicit stack to manage what the recursive version handles through the call stack. This pattern appears frequently when converting between recursive and iterative approaches.
4. Start with the base case
When attempting to develop a recursive solution, first identify the simplest version of the problem that can be solved directly. This base case provides the foundation for your recursive solution.
For example, when writing a recursive function to calculate the power of a number:
function power(base, exponent) {
// Base case: any number to the power of 0 is 1
if (exponent === 0) return 1;
// Recursive case
return base * power(base, exponent - 1);
}
The base case (exponent = 0) is simple to solve directly. The recursive case builds on this foundation.
Common Patterns in Recursive Problems
Certain patterns appear frequently in problems well-suited for recursion. Recognizing these patterns can help trigger your recursive thinking.
1. Linear Recursion
In linear recursion, each function call makes at most one recursive call. This is the simplest form of recursion and is often used for processing linear structures like arrays or linked lists.
Example: Calculating the length of a linked list.
function length(list) {
if (list === null) return 0;
return 1 + length(list.next);
}
2. Binary Recursion
Binary recursion involves making two recursive calls in each function invocation. This pattern often appears in divide-and-conquer algorithms and binary tree operations.
Example: Merge sort algorithm.
function mergeSort(arr) {
if (arr.length <= 1) return arr;
const mid = Math.floor(arr.length / 2);
const left = mergeSort(arr.slice(0, mid));
const right = mergeSort(arr.slice(mid));
return merge(left, right);
}
function merge(left, right) {
const result = [];
let leftIndex = 0;
let rightIndex = 0;
while (leftIndex < left.length && rightIndex < right.length) {
if (left[leftIndex] < right[rightIndex]) {
result.push(left[leftIndex]);
leftIndex++;
} else {
result.push(right[rightIndex]);
rightIndex++;
}
}
return result.concat(left.slice(leftIndex)).concat(right.slice(rightIndex));
}
3. Multiple Recursion
Multiple recursion involves making multiple recursive calls, often to explore different possibilities or paths. This pattern is common in combinatorial problems and backtracking algorithms.
Example: Generating all permutations of a string.
function permutations(str) {
if (str.length <= 1) return [str];
const result = [];
for (let i = 0; i < str.length; i++) {
const current = str[i];
const remaining = str.slice(0, i) + str.slice(i + 1);
const subPermutations = permutations(remaining);
for (const perm of subPermutations) {
result.push(current + perm);
}
}
return result;
}
4. Tail Recursion
Tail recursion is a special case where the recursive call is the last operation in the function. Many languages and compilers can optimize tail recursion to avoid stack overflow issues.
Example: Factorial with tail recursion.
function factorialTail(n, accumulator = 1) {
if (n <= 1) return accumulator;
return factorialTail(n - 1, n * accumulator);
}
Notice how the recursive call is the last operation, and no further computation is needed after it returns.
5. Mutual Recursion
Mutual recursion involves two or more functions that call each other. This pattern is useful for problems with alternating states or conditions.
Example: Determining if a number is even or odd.
function isEven(n) {
if (n === 0) return true;
return isOdd(n - 1);
}
function isOdd(n) {
if (n === 0) return false;
return isEven(n - 1);
}
Real-World Applications: When to Actually Use Recursion
Understanding when to use recursion in practical programming scenarios requires balancing several factors:
1. Code Clarity and Maintainability
Recursion often produces more concise, readable code for problems with inherently recursive structures. If a recursive solution makes the code's intent clearer, it's likely a good choice.
For example, traversing a file system hierarchy is naturally expressed recursively:
function listAllFiles(directory) {
let files = [];
const entries = fs.readdirSync(directory);
for (const entry of entries) {
const fullPath = path.join(directory, entry);
const stats = fs.statSync(fullPath);
if (stats.isFile()) {
files.push(fullPath);
} else if (stats.isDirectory()) {
files = files.concat(listAllFiles(fullPath));
}
}
return files;
}
2. Problem Structure
Some problems have an inherently recursive structure where the recursive solution closely matches the problem definition:
- Tree and graph traversal
- Parsing expressions or structures with nested components
- Problems defined by recurrence relations
- Divide-and-conquer algorithms
3. Performance Considerations
While recursion can sometimes introduce overhead, don't automatically dismiss it for performance reasons. Consider:
- Is the recursive solution significantly clearer?
- Is tail recursion optimization possible?
- Can techniques like memoization address performance concerns?
- What is the maximum recursion depth likely to be?
For example, recursive implementations of algorithms like quicksort are often preferred despite the availability of iterative alternatives, due to their clarity and the limited recursion depth for most practical inputs.
4. Language and Environment
Some programming languages and environments are more recursion-friendly than others:
- Functional programming languages like Haskell and Scheme are designed with recursion as a primary control structure
- Languages with tail call optimization (like Scala) make deep recursion safer
- Some environments have restrictive stack size limits that make recursion more risky
Case Study: Converting Between Recursion and Iteration
To develop stronger intuition for recursion, it's valuable to understand how recursive solutions can be converted to iterative ones and vice versa. Let's examine a classic example: depth-first traversal of a binary tree.
The Recursive Approach
function dfsRecursive(node, result = []) {
if (!node) return result;
// Visit the node
result.push(node.value);
// Traverse left subtree
dfsRecursive(node.left, result);
// Traverse right subtree
dfsRecursive(node.right, result);
return result;
}
The Iterative Approach
function dfsIterative(root) {
if (!root) return [];
const result = [];
const stack = [root];
while (stack.length > 0) {
const node = stack.pop();
result.push(node.value);
// Push right child first so left is processed first (LIFO)
if (node.right) stack.push(node.right);
if (node.left) stack.push(node.left);
}
return result;
}
The key insight is that the call stack in the recursive version is explicitly modeled as a stack data structure in the iterative version. This pattern applies to many recursive-to-iterative conversions.
The Conversion Pattern
When converting from recursion to iteration:
- Identify what information is being stored on the call stack
- Create an explicit data structure (usually a stack) to store this information
- Replace recursive calls with operations on this data structure
- Use a loop to process items from the data structure
This pattern can help you understand the relationship between recursive and iterative approaches, strengthening your ability to recognize when recursion is appropriate.
Practical Exercises to Develop Recursive Thinking
To bridge the gap between understanding recursion and applying it effectively, practice is essential. Here are some exercises specifically designed to develop your recursive intuition:
1. Recursive Problem Decomposition
Take a problem and explicitly decompose it into:
- What is the base case?
- How can I express the solution in terms of smaller instances?
Practice this with problems like:
- Reversing a string
- Checking if a string is a palindrome
- Finding the greatest common divisor of two numbers
2. Convert Between Approaches
Take algorithms you know well and implement them both recursively and iteratively:
- Binary search
- Linked list operations (reverse, find, delete)
- Counting elements in nested arrays
3. Solve Classic Recursive Problems
Implement solutions to problems with well-known recursive solutions:
- Tower of Hanoi
- N-Queens problem
- Generating all subsets of a set
- Computing paths in a maze
4. Analyze Real-World Code
Study open-source code that uses recursion effectively:
- Parser implementations
- DOM traversal in web frameworks
- File system operations
Pay attention to how experienced developers decide when to use recursion and how they structure their recursive functions.
Conclusion: Bridging the Understanding-Application Gap
The ability to explain recursion but struggle to apply it represents a common gap in programming knowledge. This gap isn't unique to recursion; it appears in many areas where conceptual understanding doesn't automatically translate to practical application skills.
To bridge this gap:
- Recognize the difference between knowing and doing - Understanding that conceptual knowledge and application skills are distinct capabilities that require different types of practice.
- Develop pattern recognition - Expose yourself to many examples of recursive problems and solutions to train your brain to recognize recursive patterns.
- Practice deliberately - Don't just solve problems; reflect on whether recursion would be appropriate and why.
- Build mental models - Develop visualization techniques for how recursive calls unfold and how the call stack operates.
Remember that the intuition for when to use recursion develops gradually through exposure and practice. Even experienced programmers sometimes miss opportunities for elegant recursive solutions or apply recursion in situations where iteration would be clearer.
By practicing the techniques outlined in this article, you can develop the intuition to not just explain recursion, but to recognize when it's the right tool for the job. This skill will not only make your code more elegant in appropriate situations but will also deepen your overall problem-solving abilities as a programmer.
The next time you face a programming challenge, pause and ask yourself: "Could this problem be naturally decomposed into smaller versions of itself?" That simple question might open the door to a recursive solution you might otherwise have missed.