Why You Can Code the Solution but Can’t Plan the Approach
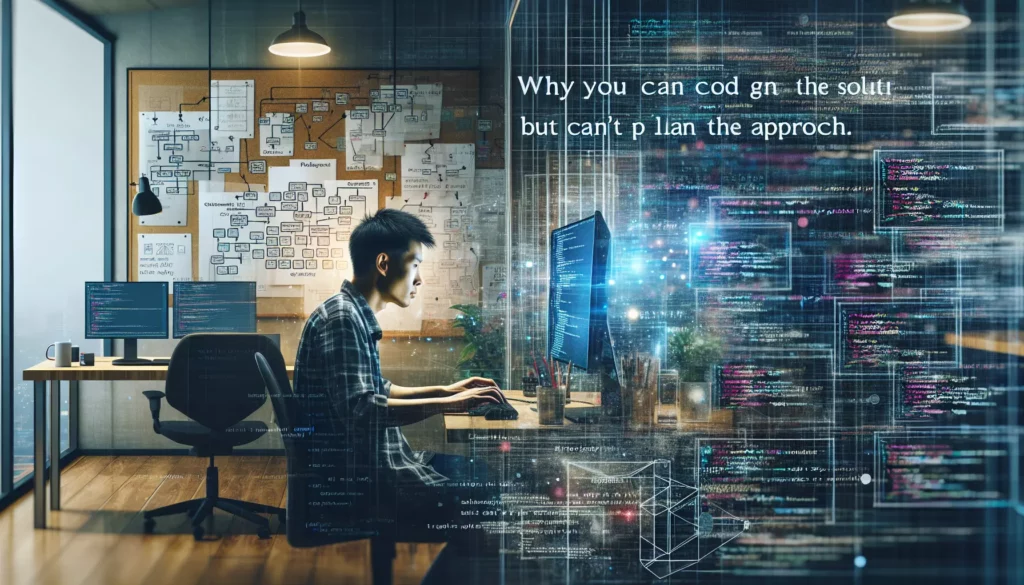
Have you ever found yourself in this frustrating situation: You understand all the programming concepts, you can write clean code in your preferred language, but when faced with a new problem, you freeze? You know you should be able to solve it, but something is missing in your approach.
This phenomenon is incredibly common, even among experienced developers. The ability to implement code doesn’t automatically translate to the ability to plan solutions. Let’s explore why this disconnect exists and how to bridge this critical gap in your programming journey.
The Implementation vs. Planning Gap
Many coding learners experience a peculiar paradox: they can understand and write code when the solution path is clear, but struggle to map out that path themselves. This disconnect between implementation skills and planning abilities manifests in several ways:
- You can follow tutorials perfectly but get stuck on open-ended projects
- You understand solutions when they’re explained but couldn’t have arrived at them yourself
- You know all the syntax and concepts but freeze when given a blank editor and a problem statement
- You feel confident modifying existing code but anxious creating solutions from scratch
If any of these sound familiar, you’re experiencing the implementation vs. planning gap. But why does this gap exist?
Why This Gap Exists
Different Mental Processes
Coding and planning utilize different cognitive functions. Implementation is largely about applying known patterns and syntax—it’s concrete and procedural. Planning, however, requires abstract thinking, pattern recognition, and creative problem-solving. These are fundamentally different mental processes.
When you write code based on a clear plan, you’re engaging in convergent thinking—applying known rules to reach a specific outcome. Planning a solution involves divergent thinking—exploring multiple possibilities before converging on an approach.
Educational Focus on Syntax Over Strategy
Most coding education heavily emphasizes syntax and language features rather than problem-solving strategies. Think about it: how many courses have you taken that spend weeks on language syntax but only briefly touch on how to approach problems?
Many tutorials follow a “monkey see, monkey do” approach where you’re shown a solution and then asked to implement something similar. This builds implementation skills but does little for developing planning abilities.
The Curse of Knowledge
When experts demonstrate solutions, they often skip the messy middle part—the false starts, the dead ends, and the iterative thinking process. They show the polished final product, creating the illusion that they arrived at the solution in one clean, linear thought process.
This “curse of knowledge” makes learners believe that expert programmers simply “see” the solution immediately, rather than working through multiple approaches and refinements.
Lack of Problem Decomposition Skills
Many programmers haven’t developed the crucial skill of breaking down complex problems into smaller, more manageable sub-problems. Without this ability, they approach problems as intimidating monoliths rather than collections of solvable pieces.
Signs You’re Struggling with the Planning Phase
How do you know if planning is your weakness? Watch for these telltale signs:
- You immediately start coding without a clear strategy
- You frequently scrap your code and restart from scratch
- You make significant architectural changes late in development
- You feel overwhelmed by problems that have multiple possible approaches
- You struggle to explain your solution strategy before implementing it
- You find yourself writing code that you later realize is unnecessary
- You have trouble estimating how long a coding task will take
If several of these resonate with you, your planning skills likely need attention.
The Problem-Solving Framework Most Courses Skip
Effective problem-solving in programming follows a framework that many courses neglect to teach explicitly. Here’s what the process actually looks like:
1. Understand the Problem Completely
Before writing a single line of code, ensure you fully understand what you’re trying to solve:
- Restate the problem in your own words
- Identify inputs, outputs, and constraints
- Work through examples manually to understand the expected behavior
- Ask clarifying questions (even if just to yourself)
- Consider edge cases and special conditions
This step is often rushed, leading to solutions that solve the wrong problem or miss critical requirements.
2. Explore Multiple Approaches
Resist the urge to commit to the first solution that comes to mind. Instead:
- Brainstorm at least 2-3 different approaches
- Consider the tradeoffs of each (time complexity, space complexity, readability)
- Think about similar problems you’ve solved before
- Sketch out high-level workflows for each approach
This exploration phase is where most programmers falter, jumping straight from problem understanding to implementation.
3. Break Down the Problem
Once you’ve selected an approach, decompose it into smaller sub-problems:
- Identify the major components or steps
- Determine dependencies between components
- Define interfaces between components
- Consider which parts can be abstracted or reused
This decomposition makes complex problems tractable and helps organize your thoughts.
4. Plan Before Coding
Create a roadmap before writing code:
- Write pseudocode for the overall solution
- Outline function signatures and their purposes
- Decide on data structures and justify your choices
- Plan your testing strategy
This planning stage creates a blueprint that makes the implementation phase much more straightforward.
5. Implement Incrementally
With a solid plan in place, implementation becomes more methodical:
- Build one component at a time
- Test each component as you go
- Create stubs or mock implementations for components you’ll build later
- Refactor as needed before moving to the next component
6. Review and Refine
After implementation, critically evaluate your solution:
- Test with various inputs, including edge cases
- Analyze time and space complexity
- Look for opportunities to simplify or optimize
- Consider alternative implementations
A Case Study: The Two Programmers
Let’s compare two programmers tackling the same problem: implementing a function to find the longest palindromic substring in a given string.
Programmer A: The Implementer
Programmer A immediately starts coding a brute force solution:
function longestPalindrome(s) {
let longest = "";
for (let i = 0; i < s.length; i++) {
for (let j = i; j < s.length; j++) {
let substr = s.substring(i, j + 1);
if (isPalindrome(substr) && substr.length > longest.length) {
longest = substr;
}
}
}
return longest;
}
function isPalindrome(s) {
return s === s.split('').reverse().join('');
}
After testing, Programmer A realizes this solution is too slow for longer strings. They get stuck, try random optimizations, and eventually search for a better solution online.
Programmer B: The Planner
Programmer B takes a different approach:
- Understands the problem: They work through examples manually, noting that palindromes can be odd or even length.
- Explores approaches: They consider brute force but recognize its O(n³) complexity. They think about how palindromes work—they expand outward from a center—and sketch an alternative approach.
- Plans the solution: They decide to implement a function that expands around potential palindrome centers, checking both odd and even length palindromes.
Their solution:
function longestPalindrome(s) {
if (!s || s.length < 1) return "";
let start = 0;
let end = 0;
for (let i = 0; i < s.length; i++) {
// Check for odd-length palindromes with center at i
let len1 = expandAroundCenter(s, i, i);
// Check for even-length palindromes with center between i and i+1
let len2 = expandAroundCenter(s, i, i + 1);
let len = Math.max(len1, len2);
if (len > end - start) {
start = i - Math.floor((len - 1) / 2);
end = i + Math.floor(len / 2);
}
}
return s.substring(start, end + 1);
}
function expandAroundCenter(s, left, right) {
while (left >= 0 && right < s.length && s[left] === s[right]) {
left--;
right++;
}
return right - left - 1; // Length of palindrome
}
The difference? Programmer B spent time understanding the problem structure and planning an efficient approach before writing code. Their solution has O(n²) time complexity versus Programmer A’s O(n³).
Common Planning Pitfalls
Even when attempting to plan, many programmers fall into these traps:
The Premature Optimization Trap
Some programmers get caught overthinking efficiency before they even have a working solution. They try to optimize for every possible scenario, creating overly complex designs that address theoretical edge cases rather than focusing on core functionality first.
The better approach: Get a correct solution first, then optimize if needed. As Donald Knuth famously said, “Premature optimization is the root of all evil.”
The Analysis Paralysis Problem
The opposite problem occurs when programmers get stuck in endless planning, unable to commit to an approach. They keep exploring alternatives without ever moving to implementation.
The better approach: Set a time limit for your planning phase. After exploring a few approaches, commit to one and start implementing. You can always refine as you go.
The Complexity Bias
Many programmers unconsciously favor complex solutions over simple ones, assuming that difficult problems require sophisticated answers. This leads to overengineered solutions when simpler approaches would work better.
The better approach: Always seek the simplest solution that satisfies all requirements. As Einstein supposedly said, “Everything should be made as simple as possible, but no simpler.”
The “I’ll Figure It Out As I Go” Mentality
Some programmers believe they can discover the solution through coding, without any upfront planning. While exploratory coding has its place, it’s inefficient for most non-trivial problems.
The better approach: Do at least minimal planning before coding. Even a rough sketch of your approach will save time compared to purely exploratory coding.
Practical Exercises to Develop Planning Skills
If you recognize your planning skills need work, here are exercises specifically designed to strengthen this muscle:
Exercise 1: Reverse Engineer Solutions
Take existing solutions to problems and work backward:
- Study a solution to a problem you haven’t seen before
- Identify the key insights that make the solution work
- Try to articulate what thought process would lead to discovering these insights
- Create a “planning document” that outlines how you would arrive at this solution step by step
This exercise helps you internalize effective planning patterns by deconstructing successful solutions.
Exercise 2: The Pseudocode-First Challenge
For your next few programming challenges:
- Write complete pseudocode before writing any actual code
- Have someone review your pseudocode if possible
- Only start coding once your pseudocode is complete
- After implementing, compare your code to your pseudocode and note any divergences
This forces you to think through the entire solution before getting lost in implementation details.
Exercise 3: The Multiple Approaches Drill
For each problem you encounter:
- Force yourself to come up with at least three different approaches
- For each approach, list pros and cons
- Estimate the time and space complexity of each
- Only then select the approach to implement
This prevents you from latching onto the first idea that comes to mind and builds the habit of exploring the solution space.
Exercise 4: Explain Before You Code
Before implementing a solution:
- Explain your planned approach to someone else (or even to a rubber duck)
- Articulate why you chose this approach over alternatives
- Walk through how your solution will handle various test cases
- Only start coding after you can clearly explain your strategy
This “explain first” approach forces you to clarify your thinking and often reveals holes in your reasoning before you waste time implementing a flawed solution.
Exercise 5: Decomposition Practice
Take complex problems and practice breaking them down:
- Identify all the sub-problems that need to be solved
- Determine the dependencies between sub-problems
- Create a visual map (like a flowchart) showing how the sub-problems fit together
- Solve each sub-problem independently before integrating them
This builds your ability to tackle complex problems by breaking them into manageable pieces.
Real-world Planning Techniques from Senior Developers
Senior developers have developed various techniques to plan effectively:
The 15-Minute Exploration Rule
Many experienced developers follow a rule: spend at least 15 minutes exploring the problem and potential approaches before writing any code. This small investment in planning prevents hours of wasted implementation time.
Whiteboarding (Even When Alone)
Using a whiteboard (or digital equivalent) to sketch out solutions visually can unlock insights that aren’t apparent when just thinking about the problem. The spatial nature of whiteboarding helps with seeing relationships between components.
The Rubber Duck Method
Explaining your approach to an inanimate object (traditionally a rubber duck) forces you to articulate your thinking clearly. This often reveals flaws in your reasoning or simpler approaches you hadn’t considered.
The Test-First Approach
Writing tests before implementing forces you to think about what your solution should accomplish and how it will behave in various scenarios. This clarifies requirements and provides a roadmap for implementation.
The Spike Solution
When facing uncertainty, experienced developers often create a quick, throwaway “spike” solution to explore the problem space. This code isn’t meant to be kept but serves as a learning tool to inform the actual solution.
How This Affects Technical Interviews
The planning-implementation gap becomes glaringly apparent in technical interviews, where candidates are evaluated not just on their coding ability but on their problem-solving approach.
What Interviewers Actually Look For
Contrary to popular belief, most interviewers aren’t primarily interested in whether you can produce a working solution in the allotted time. They’re evaluating:
- How you approach unfamiliar problems
- Your ability to consider multiple solutions
- How you evaluate tradeoffs between approaches
- Your process for breaking down complex problems
- How you communicate your thinking
- Your ability to plan before diving into code
A candidate who thoughtfully plans their approach before coding, even if they don’t finish implementing, often impresses interviewers more than someone who immediately starts coding without a clear strategy.
The Interview Planning Framework
For technical interviews, follow this framework:
- Clarify the problem (2-3 minutes)
- Restate the problem
- Ask clarifying questions
- Establish constraints and edge cases
- Work through examples (2-3 minutes)
- Use simple examples to understand the problem
- Try edge cases to test your understanding
- Outline approaches (3-5 minutes)
- Discuss 2-3 potential approaches
- Analyze time and space complexity for each
- Select the most appropriate approach
- Plan your solution (3-5 minutes)
- Break down into components
- Sketch the algorithm in pseudocode
- Discuss any helper functions needed
- Implement (remaining time)
- Convert your plan to code
- Test with examples as you go
- Review and optimize (if time permits)
- Walk through your solution with test cases
- Discuss potential optimizations
This structured approach demonstrates your planning abilities and problem-solving process, which are often more valuable to interviewers than raw coding speed.
Building a Planning Habit
Like any skill, planning improves with deliberate practice. Here’s how to make planning a habit:
Start Small
Begin by spending just 5 minutes planning before each coding session. As you see the benefits, gradually increase this time for more complex problems.
Create Planning Templates
Develop personal templates or checklists for the planning phase. These might include:
- Problem understanding checklist
- Approach comparison template
- Pseudocode structure guide
- Testing strategy outline
Having these templates makes planning more concrete and less likely to be skipped.
Track Planning Benefits
Keep a log of problems you solve, noting whether you planned thoroughly or jumped straight to coding. Over time, you’ll likely notice that well-planned solutions require fewer rewrites and have fewer bugs.
Join Planning-Focused Communities
Participate in communities where solution planning is emphasized. Many competitive programming platforms have discussion forums where users share their approaches before implementing.
Pair Program with Planning Focus
When pair programming, explicitly designate time for planning before either person starts coding. Take turns explaining your planned approach to each other.
Conclusion: The Planning Mindset
The gap between being able to code solutions and being able to plan them isn’t a reflection of your programming potential—it’s simply a sign that you need to develop a different set of skills.
Planning is to coding what architecture is to construction. A builder might be excellent at following blueprints and constructing walls, but that doesn’t mean they can design a sound building. Similarly, your implementation skills don’t automatically translate to solution design abilities.
The good news is that planning skills can be systematically developed with practice. By consciously separating the planning and implementation phases of problem-solving, you’ll gradually strengthen your planning muscles.
Remember that even experienced developers sometimes struggle with planning. The difference is that they recognize when they’re stuck in implementation without a clear plan, and they know to step back and invest time in planning before continuing.
As you develop your planning skills, you’ll find that not only do your solutions become more elegant and efficient, but the implementation phase becomes smoother and more enjoyable. You’ll spend less time debugging and rewriting code, and more time confidently building solutions that work correctly the first time.
The next time you find yourself staring at a blank editor, unable to start coding despite knowing all the necessary programming concepts, recognize that you’re not facing a knowledge gap—you’re facing a planning gap. Take a step back, grab a piece of paper, and start planning. Your future self will thank you.