Why Writing Pseudo-Code Can Improve Your Problem-Solving Process
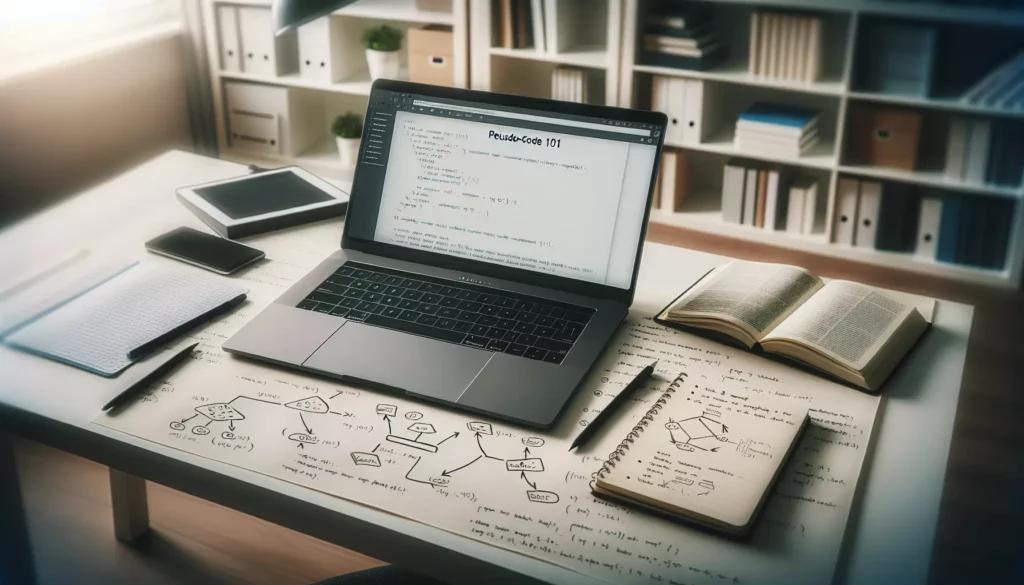
In the world of programming and software development, problem-solving is a crucial skill that separates great developers from the rest. While there are many techniques to approach complex problems, one method stands out for its simplicity and effectiveness: writing pseudo-code. This article will explore why incorporating pseudo-code into your problem-solving process can significantly enhance your coding skills and overall programming efficiency.
What is Pseudo-Code?
Before diving into the benefits of pseudo-code, let’s first define what it is. Pseudo-code is a informal, high-level description of a computer program or algorithm. It uses structural conventions of a normal programming language but is intended for human reading rather than machine reading. Essentially, it’s a way of planning out your code in plain language before diving into the actual implementation.
Here’s a simple example of pseudo-code for a function that finds the maximum number in an array:
function findMaxNumber(array):
set max to first element of array
for each element in array:
if element > max:
set max to element
return max
As you can see, this pseudo-code outlines the logic of the function without getting bogged down in the syntax of a specific programming language.
The Benefits of Writing Pseudo-Code
1. Clarifies Thinking and Problem Understanding
One of the primary advantages of writing pseudo-code is that it forces you to think through the problem thoroughly before you start coding. By breaking down the problem into smaller, manageable steps, you gain a clearer understanding of what needs to be done. This process often reveals aspects of the problem you might have overlooked if you had jumped straight into coding.
2. Language-Independent Planning
Pseudo-code allows you to plan your solution independently of any specific programming language. This is particularly useful when you’re working on complex algorithms or when you’re not sure which language you’ll ultimately use for implementation. It focuses on the logic and structure of the solution rather than the syntax, making it easier to translate into any programming language later.
3. Easier Collaboration and Communication
When working in a team, pseudo-code can serve as an excellent communication tool. It’s often easier for team members to understand and provide feedback on pseudo-code than on actual code, especially if they’re not familiar with the specific programming language being used. This can lead to more productive discussions about algorithms and problem-solving approaches.
4. Reduces Coding Time and Errors
By planning out your solution in pseudo-code first, you can often catch logical errors or inefficiencies before you start actual coding. This can save a significant amount of time in the long run, as it’s generally easier and quicker to modify pseudo-code than to refactor actual code. Additionally, having a clear plan can help reduce the number of bugs in your final code.
5. Improves Documentation
Pseudo-code can serve as a form of documentation for your code. It provides a high-level overview of what your code is doing, which can be invaluable when you need to revisit your code later or when others need to understand your implementation. Some developers even include pseudo-code as comments in their actual code to explain complex algorithms.
How to Write Effective Pseudo-Code
To get the most out of pseudo-code, it’s important to follow some best practices:
1. Keep it Simple and Clear
The main purpose of pseudo-code is to communicate ideas clearly. Use simple, straightforward language and avoid unnecessary complexity. Your pseudo-code should be easily understandable by anyone with basic programming knowledge.
2. Use Consistent Formatting
While there’s no strict syntax for pseudo-code, it’s helpful to use consistent formatting. This might include indentation to show nested structures, capitalization for keywords, and clear separation between different steps or sections of your algorithm.
3. Be Specific, But Not Too Detailed
Your pseudo-code should be specific enough to clearly outline your algorithm, but not so detailed that it becomes as complex as actual code. Find a balance between clarity and brevity.
4. Use Standard Control Structures
Incorporate common programming constructs like loops, conditionals, and functions in your pseudo-code. This makes it easier to translate into actual code later. For example:
WHILE condition
do something
END WHILE
IF condition THEN
do something
ELSE
do something else
END IF
FUNCTION name(parameters)
do something
RETURN value
END FUNCTION
5. Iterate and Refine
Don’t expect to write perfect pseudo-code on the first try. As you think through the problem, you may need to revise and refine your pseudo-code. This iterative process is valuable for improving your solution.
Pseudo-Code in the Context of Technical Interviews
For those preparing for technical interviews, especially with major tech companies often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google), pseudo-code can be an invaluable tool. Here’s why:
1. Demonstrates Thought Process
In technical interviews, the interviewer is often more interested in your problem-solving approach than in the final code itself. Writing pseudo-code allows you to clearly demonstrate your thought process and how you break down complex problems.
2. Facilitates Discussion
Pseudo-code can serve as a basis for discussion with your interviewer. It allows you to quickly sketch out your proposed solution and get feedback before diving into the implementation details.
3. Manages Time Effectively
Technical interviews often have time constraints. By quickly writing out pseudo-code, you can ensure you have a solid plan before you start coding. This can help you manage your time more effectively and avoid getting stuck on implementation details.
4. Handles Complex Problems
For particularly complex problems, it might not be feasible to write out the entire solution in code within the time limit. In such cases, pseudo-code can allow you to outline your entire approach, even if you don’t have time to fully implement it.
Integrating Pseudo-Code into Your Problem-Solving Process
Now that we’ve explored the benefits of pseudo-code, let’s look at how you can integrate it into your problem-solving process:
1. Understand the Problem
Before you start writing any pseudo-code, make sure you fully understand the problem. Ask questions if necessary and clarify any ambiguities.
2. Break Down the Problem
Divide the problem into smaller, manageable sub-problems. This is where you start to think about the steps needed to solve the problem.
3. Write High-Level Pseudo-Code
Start by writing very high-level pseudo-code that outlines the main steps of your solution. For example:
1. Initialize variables
2. Process input data
3. Perform main calculation
4. Handle edge cases
5. Return result
4. Refine and Add Detail
Gradually add more detail to each step, breaking them down further if necessary. This is where you start to think about specific algorithms or data structures you might use.
5. Review and Optimize
Look over your pseudo-code and consider if there are any ways to optimize your approach. This might involve changing the order of operations, using a different data structure, or finding a more efficient algorithm.
6. Translate to Code
Once you’re satisfied with your pseudo-code, you can start translating it into actual code. You’ll find that this process is much smoother when you have a clear plan to follow.
Common Pitfalls to Avoid
While pseudo-code can be a powerful tool, there are some common mistakes to avoid:
1. Making it Too Language-Specific
Remember, the goal of pseudo-code is to be language-independent. Avoid using syntax or features specific to a particular programming language.
2. Over-Complicating
Keep your pseudo-code simple and clear. If it becomes as complex as actual code, you’re defeating the purpose.
3. Neglecting Edge Cases
Don’t forget to consider and include edge cases in your pseudo-code. This can help you catch potential issues early.
4. Not Iterating
Your first attempt at pseudo-code doesn’t have to be perfect. Be prepared to revise and refine as you think through the problem more deeply.
Tools and Resources for Pseudo-Code
While pseudo-code is typically written by hand or in a simple text editor, there are some tools and resources that can help:
1. Flowchart Tools
Tools like Lucidchart or Draw.io can be useful for creating visual representations of your algorithms, which can complement your pseudo-code.
2. IDEs with Pseudo-Code Support
Some Integrated Development Environments (IDEs) have plugins or features that support pseudo-code. For example, the PseudoCode plugin for Visual Studio Code provides syntax highlighting for pseudo-code.
3. Online Resources
Websites like GeeksforGeeks and Codecademy offer tutorials and examples of pseudo-code, which can be helpful for learning and improving your pseudo-code writing skills.
Conclusion
Incorporating pseudo-code into your problem-solving process can significantly enhance your programming skills and efficiency. It provides a structured way to approach complex problems, facilitates clearer thinking, and can improve communication in team settings. For those preparing for technical interviews, particularly with major tech companies, pseudo-code can be an invaluable tool for demonstrating your problem-solving abilities.
Remember, the goal of pseudo-code is not to write perfect code-like instructions, but to create a clear, logical plan for your solution. With practice, you’ll find that writing pseudo-code becomes a natural and invaluable part of your problem-solving toolkit.
As you continue to develop your programming skills, consider making pseudo-code a regular part of your workflow. Whether you’re tackling a complex algorithm, designing a new feature, or preparing for a technical interview, the clarity and structure provided by pseudo-code can help you approach problems with confidence and precision. Happy coding!