Why Watching Coding Tutorials Isn’t Improving Your Problem Solving Skills
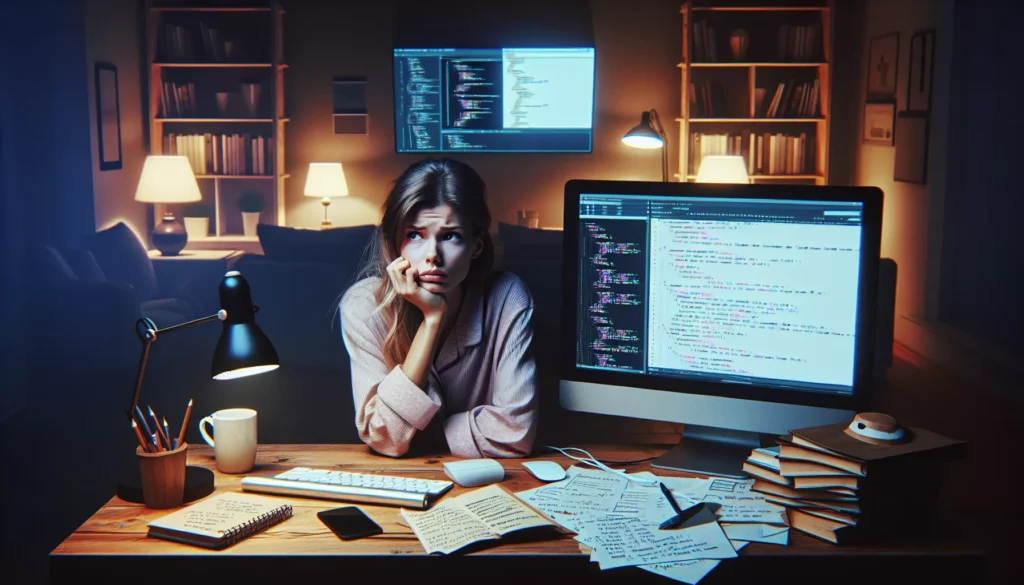
You’ve spent countless hours watching coding tutorials. Your YouTube history is filled with “Learn Python in 10 Hours” and “Become a JavaScript Master” videos. You’ve followed along, typing the same code as the instructor, feeling that rush of accomplishment when your program runs successfully. Yet, when faced with a real coding problem or a technical interview question, you freeze. The solution doesn’t come to you like it seemed to come to the tutorial instructor.
If this sounds familiar, you’re experiencing what many coding learners encounter: the tutorial trap. This phenomenon occurs when learners consume vast amounts of instructional content but struggle to apply that knowledge independently.
In this comprehensive guide, we’ll explore why passive learning through tutorials often fails to develop strong problem solving skills, and more importantly, what you can do about it.
Table of Contents
- The Illusion of Competence: Why Tutorials Feel Effective
- Passive vs. Active Learning in Programming
- The Limitations of Coding Tutorials
- The Cognitive Skills Tutorials Don’t Teach
- How to Learn Programming Effectively
- Practical Exercises to Build Problem Solving Skills
- Balancing Tutorials with Active Problem Solving
- How to Measure Your Progress Beyond Tutorials
- Conclusion: Moving Beyond the Tutorial Trap
The Illusion of Competence: Why Tutorials Feel Effective
When you follow along with a coding tutorial, you experience what psychologists call the “illusion of competence.” This cognitive bias makes you believe you understand a concept better than you actually do.
As you watch an instructor solve a problem step by step, the solution seems obvious. “Of course, that’s how you’d do it!” you think to yourself. The instructor’s explanation makes perfect sense, and when you replicate their code, it works just as expected. This creates a false sense of mastery.
Here’s why this happens:
Recognition vs. Recall
When watching tutorials, you’re engaging in recognition, which is a much simpler cognitive process than recall. Recognition is seeing something and thinking, “Yes, I know that.” Recall, however, is retrieving that information from memory without prompts or hints.
Programming in the real world requires recall. You need to remember syntax, algorithms, patterns, and concepts without someone walking you through them.
Guided Problem Solving
In tutorials, instructors have already:
- Identified the problem
- Broken it down into manageable steps
- Determined the appropriate solution approach
- Anticipated and planned for edge cases
By the time you see the tutorial, all the hard cognitive work has been done for you. You’re essentially tracing their footsteps rather than blazing your own trail.
The Missing Struggle
Real learning happens during moments of productive struggle. When you encounter a problem, try different approaches, hit walls, and eventually break through to a solution, that experience creates strong neural pathways that facilitate future problem solving.
Tutorials eliminate this valuable struggle. Everything works as expected because the instructor has ironed out all the bugs and complications beforehand.
Passive vs. Active Learning in Programming
Understanding the fundamental difference between passive and active learning is crucial for developing programming skills.
Passive Learning: The Tutorial Approach
Passive learning occurs when you consume information without actively engaging with it. In the context of programming, this includes:
- Watching video tutorials without pausing to experiment
- Reading programming books from cover to cover
- Following along with code examples without modifying them
- Listening to programming podcasts without implementing concepts
While passive learning is comfortable and can introduce you to new concepts, it rarely leads to the development of problem solving skills or deep understanding.
Active Learning: The Problem Solver’s Approach
Active learning involves engaging with material in ways that require thinking, creating, and problem solving. For programmers, this includes:
- Writing code from scratch to solve specific problems
- Debugging broken code
- Modifying existing programs to add new features
- Explaining concepts to others (teaching is one of the most effective forms of learning)
- Working on projects without step-by-step guidance
Research consistently shows that active learning leads to better retention, deeper understanding, and stronger problem solving abilities.
The Neuroscience Behind It
From a neuroscience perspective, active learning creates stronger neural connections. When you actively engage with programming concepts by solving problems, your brain forms multiple pathways to access that information.
Additionally, the emotional component of overcoming challenges creates stronger memories. The satisfaction of solving a difficult programming problem triggers dopamine release, which enhances memory formation and creates positive associations with the learning process.
The Limitations of Coding Tutorials
While tutorials have their place in the learning journey, understanding their inherent limitations can help you avoid overreliance on them.
Simplified Problems
Most tutorials use carefully crafted examples that demonstrate concepts cleanly. Real-world programming problems are messier, with:
- Ambiguous requirements
- Multiple valid solution approaches
- Performance and scalability considerations
- Integration with existing systems
- Edge cases that aren’t immediately obvious
This simplification, while helpful for teaching concepts, doesn’t prepare you for the complexity of actual programming tasks.
Linear Learning Path
Tutorials present information in a logical, sequential order. The instructor knows exactly where they’re going and the best path to get there.
Real programming is more exploratory. You often need to research multiple topics simultaneously, backtrack when you hit dead ends, and pivot your approach based on what you discover along the way.
Lack of Debugging Experience
In tutorials, code typically works as expected. When it doesn’t, the instructor quickly identifies and fixes the issue. This deprives you of essential debugging experience, which is where much of a programmer’s time is spent in reality.
Learning to read error messages, use debugging tools, and systematically isolate problems is a crucial skill that tutorials rarely develop adequately.
The “Copy and Paste” Syndrome
Many tutorial followers fall into the habit of copying code without understanding it fully. This creates knowledge gaps that become apparent only when you try to apply concepts in different contexts.
Consider this example: a learner might copy a React component from a tutorial without understanding the component lifecycle or state management principles. When they later need to create a component with different behavior, they struggle because they lack the foundational understanding.
The Cognitive Skills Tutorials Don’t Teach
Problem solving in programming requires specific cognitive skills that are difficult to develop through tutorials alone.
Algorithmic Thinking
Algorithmic thinking involves breaking down problems into logical steps and developing systematic approaches to solve them. This skill requires practice in:
- Identifying patterns in problems
- Creating abstracted, reusable solutions
- Evaluating different algorithms for efficiency
- Understanding time and space complexity
While tutorials might demonstrate algorithms, they rarely force you to develop algorithmic thinking independently.
Pattern Recognition
Experienced programmers recognize common patterns across different problems. This pattern recognition allows them to apply proven solutions to new situations.
For example, recognizing when a problem calls for a specific data structure (like a hash map for quick lookups) or design pattern (like observer pattern for event handling) comes from exposure to many different problems.
Mental Model Building
Effective programmers build mental models of how systems work. These mental models allow them to:
- Predict how code will behave
- Understand interactions between components
- Identify potential failure points
- Reason about code without always executing it
Tutorials often focus on implementation details without helping learners develop these crucial mental models.
Strategic Problem Decomposition
Complex programming problems require strategic decomposition into smaller, manageable subproblems. This skill involves:
- Identifying the core challenges within a larger problem
- Establishing dependencies between subproblems
- Determining appropriate abstraction boundaries
- Creating interfaces between components
In tutorials, this decomposition is already done for you, depriving you of the opportunity to practice this essential skill.
How to Learn Programming Effectively
Now that we understand the limitations of tutorial-based learning, let’s explore more effective approaches to developing programming skills.
The Project-Based Learning Approach
Project-based learning involves building complete applications or systems with minimal guidance. This approach forces you to:
- Define requirements and specifications
- Make architectural and design decisions
- Research and integrate appropriate technologies
- Test and debug your own code
- Refactor and improve your solutions
Start with simple projects and gradually increase complexity. For example, if you’re learning web development, you might progress from a static personal website to a dynamic application with a database backend.
The Deliberate Practice Framework
Deliberate practice, a concept popularized by psychologist Anders Ericsson, involves focused practice on specific aspects of a skill. For programmers, this means:
- Identifying weak areas in your knowledge or abilities
- Creating targeted exercises to address those weaknesses
- Seeking immediate feedback on your performance
- Reflecting on what worked and what didn’t
- Iteratively improving your approach
For example, if you struggle with recursive algorithms, you might focus on solving various recursion problems and analyzing different approaches.
The Feynman Technique
Named after physicist Richard Feynman, this technique involves explaining concepts in simple terms as if teaching someone else. The process includes:
- Choose a concept (e.g., closures in JavaScript)
- Explain it in simple language
- Identify gaps in your explanation
- Review and simplify technical terms
This approach forces you to develop a deeper understanding of programming concepts rather than just recognizing them.
Spaced Repetition and Retrieval Practice
Research in cognitive science shows that spaced repetition (reviewing material at increasing intervals) and retrieval practice (actively recalling information) significantly improve long-term retention.
For programmers, this might involve:
- Revisiting previously solved problems without looking at your solution
- Creating flashcards for programming concepts and syntax
- Implementing the same functionality using different approaches
- Teaching concepts to others after learning them
Practical Exercises to Build Problem Solving Skills
Let’s explore specific exercises that develop the problem solving muscles that tutorials often neglect.
Code Challenges with Constraints
Solving problems with artificial constraints forces creative thinking. Try exercises like:
- Implementing a sorting algorithm without using loops
- Building a web page without JavaScript
- Creating a function using a maximum of 10 lines of code
- Solving a problem using only pure functions (no side effects)
These constraints prevent you from using familiar patterns and push you to explore alternative approaches.
Reverse Engineering Exercises
Take existing code and work backward to understand it. For example:
- Examine an open-source library and document how it works
- Study a complex algorithm implementation and explain each step
- Take a working application and create a detailed architecture diagram
- Predict the output of unfamiliar code before running it
This develops your ability to read and understand code, a critical skill for professional developers.
Debugging Challenges
Deliberately practice debugging by:
- Introducing bugs into working code and then fixing them
- Working with “broken” code examples that you need to repair
- Using debugging tools to trace program execution
- Analyzing and fixing performance issues in inefficient code
Debugging is where many programmers spend significant time, yet it’s rarely covered thoroughly in tutorials.
Implementation from Specification
Instead of following step-by-step tutorials, try implementing features from written specifications:
- Build features based on user stories or requirements documents
- Implement algorithms from academic papers or descriptions
- Create components that match a provided API specification
- Replicate the functionality of existing tools without looking at their code
This simulates real-world development, where you rarely have step-by-step instructions.
Code Review Practice
Reviewing others’ code develops critical analysis skills:
- Contribute to open-source projects by reviewing pull requests
- Exchange code reviews with peers or mentors
- Analyze and suggest improvements for public code examples
- Compare multiple solutions to the same problem
Code review forces you to think critically about code quality, readability, and efficiency.
Balancing Tutorials with Active Problem Solving
Despite their limitations, tutorials do have value in the learning process. The key is finding the right balance and using tutorials effectively.
The Learning Cycle Approach
Consider adopting a cyclical learning approach:
- Concept Introduction: Use tutorials to introduce new concepts and tools
- Guided Practice: Follow along with basic examples
- Independent Application: Apply the concept to a different problem
- Extension and Exploration: Modify and expand on what you’ve learned
- Reflection and Integration: Connect new knowledge with existing skills
This cycle ensures that tutorials serve as a starting point rather than the entire learning experience.
Active Watching Techniques
When you do use tutorials, engage with them actively:
- Predict Next Steps: Pause the tutorial and predict what the instructor will do next
- Code First, Watch Later: Try solving the problem yourself before watching the solution
- Take Notes: Summarize key concepts in your own words
- Ask Questions: Note questions that arise and research them independently
- Experiment: Modify the example code to test your understanding
These techniques transform passive watching into active learning.
The 50/50 Rule
Consider adopting a 50/50 rule for your learning time:
- 50% of your time on instruction (tutorials, books, courses)
- 50% of your time on application (projects, exercises, problems)
As you advance, gradually shift this ratio to favor more application and less instruction.
Tutorial Selection Criteria
Not all tutorials are created equal. Look for tutorials that:
- Explain the “why” behind decisions, not just the “how”
- Include challenges or exercises beyond the basic examples
- Cover common errors and debugging approaches
- Discuss alternative implementations and trade-offs
- Connect concepts to broader programming principles
These higher-quality tutorials provide more transferable knowledge than those focused solely on implementation steps.
How to Measure Your Progress Beyond Tutorials
As you shift from tutorial-based learning to more active problem solving, you need new ways to measure your progress.
Problem Solving Metrics
Track metrics that reflect problem solving ability:
- Time to Solution: How long it takes you to solve new problems
- Solution Quality: Code cleanliness, efficiency, and robustness
- Independence Level: How much external help you needed
- Debugging Efficiency: How quickly you can identify and fix issues
- Knowledge Transfer: Ability to apply concepts across different contexts
These metrics provide more meaningful feedback than simply counting completed tutorials.
Portfolio Development
Build a portfolio of projects that demonstrate your skills:
- Complete projects that solve real problems
- Contribute to open-source initiatives
- Document your problem solving process
- Showcase different programming paradigms and technologies
- Include refactored projects that demonstrate growth over time
A strong portfolio provides concrete evidence of your abilities beyond tutorial completion.
Peer Feedback and Code Reviews
Seek feedback from others to gain outside perspective:
- Join programming communities and share your work
- Participate in pair programming sessions
- Submit your code for formal reviews
- Engage in programming competitions or hackathons
- Contribute to discussions about code quality and best practices
External feedback often reveals blind spots in your knowledge and skills.
Technical Interview Preparation
Use technical interview questions as a benchmark:
- Practice common algorithm and data structure problems
- Explain your solutions verbally (simulating an interview)
- Analyze time and space complexity of your solutions
- Compare your approaches to standard solutions
- Time yourself to measure improvement over time
Interview-style problems test both conceptual understanding and application skills.
Teaching and Explanation
Your ability to teach others reflects your own understanding:
- Explain complex concepts to beginners
- Create tutorials or blog posts on topics you’ve learned
- Answer questions on forums like Stack Overflow
- Mentor less experienced programmers
- Present technical topics at meetups or conferences
Teaching exposes gaps in your knowledge and deepens your understanding of familiar concepts.
Conclusion: Moving Beyond the Tutorial Trap
The journey from tutorial follower to problem solver is neither quick nor easy, but it’s essential for becoming an effective programmer. Here’s a summary of key takeaways:
- Recognize the limitations of tutorial-based learning and the illusion of competence it can create
- Shift from passive consumption to active engagement with programming concepts
- Develop cognitive skills like algorithmic thinking and pattern recognition through deliberate practice
- Balance instruction with application, gradually increasing the proportion of independent problem solving
- Measure progress through meaningful metrics beyond tutorial completion
Remember that struggle is not a sign of failure but an essential part of the learning process. The moments when you’re stuck, confused, and searching for solutions are precisely when your problem solving skills are developing most rapidly.
As you continue your programming journey, use tutorials as a starting point rather than a destination. They can introduce you to new concepts and technologies, but true mastery comes from applying those concepts to solve novel problems independently.
The programming field rewards problem solvers, not tutorial followers. By shifting your learning approach to emphasize active problem solving, you’ll develop the skills that truly matter for success as a programmer.
What will you build today that goes beyond following a tutorial?