Why Understanding Every Line of Code Doesn’t Mean You Can Architect Solutions
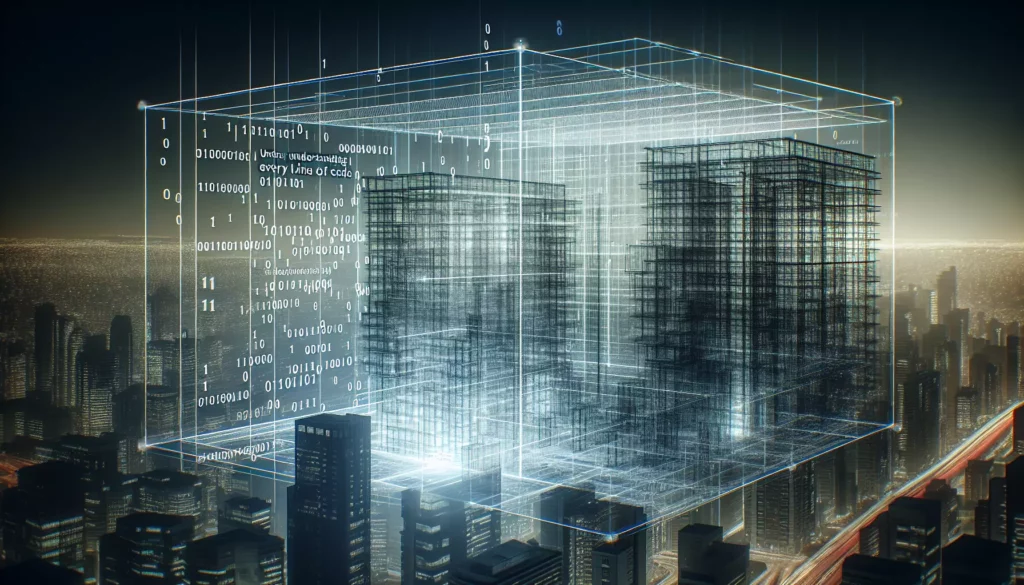
In the world of software development, there’s a common misconception that mastering the syntax of a programming language automatically translates to the ability to design complex systems. Many aspiring developers focus intensely on understanding every line of code they write, believing this granular knowledge is the key to becoming a proficient software architect. However, the reality is far more nuanced.
While code proficiency is undoubtedly important, the ability to architect solutions requires a different set of skills and a broader perspective. This distinction is crucial for anyone looking to advance in their programming career, particularly those aiming for roles at top tech companies.
The Difference Between Coding and Architecting
Let’s start by clarifying the fundamental difference between coding and architecting software solutions:
Coding Skills
- Understanding syntax and language features
- Writing clean, efficient code
- Debugging and troubleshooting issues
- Implementing algorithms correctly
- Working with libraries and frameworks
Architectural Skills
- System design and high-level planning
- Understanding trade-offs between different approaches
- Considering scalability, performance, and maintainability
- Breaking down complex problems into manageable components
- Making technology choices aligned with business goals
The distinction becomes clear when we consider that many excellent coders struggle with system design, while skilled architects may not necessarily write the most elegant code line by line.
The Limitations of Line-by-Line Understanding
Focusing exclusively on understanding every line of code has several limitations when it comes to developing architectural thinking:
Missing the Forest for the Trees
When you’re deeply focused on individual lines of code, it’s easy to lose sight of the broader context. Consider this simple example:
// Function that processes user data
function processUserData(userData) {
const cleanedData = sanitizeInput(userData);
const validatedData = validateUserInfo(cleanedData);
const normalizedData = normalizeUserFields(validatedData);
saveToDatabase(normalizedData);
notifyUser(userData.email);
}
A developer focused only on the implementation details might ensure each function works correctly but miss crucial architectural considerations:
- What happens if the database is temporarily unavailable?
- Should user notification be part of this function or handled separately?
- Is this function handling too many responsibilities?
- How will this approach scale with thousands of concurrent users?
Technology Tunnel Vision
Understanding every line of code in a specific language or framework can lead to “technology tunnel vision,” where developers approach all problems through the lens of their preferred tools. This limits creativity and can result in suboptimal solutions.
For example, a JavaScript expert might default to building everything as a Node.js application, even when another technology might be more appropriate for certain components of the system.
Scalability Blindness
Code that works perfectly for small datasets or low traffic might completely fall apart under real-world conditions. Consider this seemingly innocuous code:
function findRelatedItems(itemId) {
const allItems = database.fetchAllItems();
return allItems.filter(item => item.category === getItemCategory(itemId));
}
This function works fine with a few hundred items but becomes problematic with millions of records. An architect would immediately recognize the potential scalability issue, while someone focused only on the correctness of each line might miss it.
Essential Architectural Thinking Skills
So what skills should developers cultivate to move beyond line-by-line coding to true architectural thinking?
Systems Thinking
Architects must develop the ability to see systems holistically, understanding how components interact and influence each other. This involves:
- Identifying dependencies between components
- Recognizing potential bottlenecks
- Understanding data flow throughout the system
- Anticipating failure points and planning for resilience
For example, when designing a social media platform, an architect considers not just how to store posts and comments, but how the notification system, content delivery network, authentication service, and analytics platform all work together as an integrated whole.
Trade-off Analysis
Perhaps the most critical skill for architects is the ability to analyze trade-offs. Every architectural decision involves compromises between competing factors:
- Performance vs. development speed
- Scalability vs. complexity
- Flexibility vs. maintainability
- Cost vs. reliability
Consider a decision about database selection. A NoSQL solution might offer better scalability for certain workloads, while a relational database provides stronger consistency guarantees. The architect must weigh these factors against the specific needs of the project.
Pattern Recognition
Experienced architects recognize common patterns and know when to apply them. This knowledge comes from exposure to many different systems and problems:
- Design patterns (Factory, Singleton, Observer, etc.)
- Architectural patterns (Microservices, Event-Driven, etc.)
- Integration patterns (API Gateway, Message Queue, etc.)
For instance, when facing a problem involving disparate data sources that need to be combined, an architect might immediately recognize this as a good candidate for an API gateway pattern, rather than having to reinvent a solution from scratch.
Business Domain Understanding
Great architects understand that technology serves business needs, not the other way around. This requires:
- Understanding the problem domain thoroughly
- Aligning technical decisions with business objectives
- Communicating technical concepts to non-technical stakeholders
- Balancing immediate needs with long-term vision
A developer who understands every line of code but fails to grasp how the software delivers business value will struggle to make appropriate architectural decisions.
The Role of Experience in Architecture
There’s a reason why senior architect positions typically require years of experience. Architectural thinking is heavily influenced by exposure to different:
Success and Failure Patterns
Experiencing both successful and failed projects provides invaluable insights. Architects learn what works and what doesn’t through direct experience, often in ways that can’t be taught theoretically.
For example, an architect who has lived through a system failure due to unexpected load will instinctively consider scalability in future designs in ways that someone who has only read about scalability issues might miss.
Evolution of Systems Over Time
Seeing how systems evolve over months and years provides perspective that’s impossible to gain from short-term projects. Architects who have maintained systems long-term understand how today’s decisions impact tomorrow’s flexibility.
A seemingly minor choice about data structure or API design might have minimal impact initially but create significant technical debt as the system grows. This foresight comes primarily from experience.
Cross-Disciplinary Exposure
Great architects often have experience across multiple domains, technologies, and roles. This breadth provides a rich toolkit of approaches and solutions.
A developer who has worked on both front-end and back-end systems, or who has experience with both monoliths and microservices, can draw on these diverse experiences when architecting new solutions.
From Code Understanding to Architectural Thinking
So how can developers bridge the gap between understanding code and developing architectural thinking? Here are practical approaches:
Study System Design
Deliberately study system design principles and practices. Resources like:
- System design interview preparation materials
- Architecture documentation of open-source projects
- Technical blogs from companies explaining their architecture
- Books on software architecture and design patterns
These resources help develop the “architectural vocabulary” needed to think about systems at a higher level.
Analyze Existing Systems
Take time to analyze systems you use or work with:
- Why was this particular architecture chosen?
- What are its strengths and weaknesses?
- How might you design it differently?
- How has it evolved over time and why?
This analysis helps develop critical evaluation skills essential for architectural thinking.
Build Small Systems End-to-End
Rather than focusing solely on individual components, build complete (though small) systems from scratch. This provides experience with all aspects of the development lifecycle and forces consideration of how components interact.
For example, building a simple blog platform requires thinking about user authentication, content storage, rendering, commenting functionality, and how these pieces work together.
Seek Feedback on Design Decisions
Actively seek feedback on your design decisions from more experienced developers or architects. This can be formal (through code reviews or design documents) or informal discussions.
The feedback process helps identify blind spots in your thinking and exposes you to alternative approaches you might not have considered.
Real-World Examples: Code Understanding vs. Architectural Thinking
Let’s examine some concrete examples that illustrate the difference between code-level understanding and architectural thinking:
Example 1: Authentication System
Code-Level Approach:
function authenticateUser(username, password) {
const user = database.findUserByUsername(username);
if (!user) return { success: false, message: "User not found" };
const passwordMatch = comparePassword(password, user.passwordHash);
if (!passwordMatch) return { success: false, message: "Invalid password" };
const token = generateAuthToken(user.id);
return { success: true, token: token };
}
A developer focused on code-level understanding ensures this function correctly authenticates users and generates tokens.
Architectural Thinking:
An architect considers broader questions:
- How will authentication scale across multiple services?
- Should we implement a centralized identity provider?
- What authentication protocols (OAuth, SAML, etc.) should we support?
- How will we handle token revocation and refresh?
- What security considerations exist beyond correct code execution?
- Should authentication be a separate microservice?
Example 2: E-commerce Product Catalog
Code-Level Approach:
function getProductDetails(productId) {
const product = database.getProduct(productId);
const inventory = inventoryService.getStockLevel(productId);
const reviews = reviewService.getProductReviews(productId);
return {
...product,
inStock: inventory > 0,
reviewCount: reviews.length,
averageRating: calculateAverageRating(reviews)
};
}
At the code level, this function correctly retrieves and combines product information.
Architectural Thinking:
An architect considers:
- How to handle high traffic to popular products
- Caching strategies for product information
- Whether to use BFF (Backend for Frontend) patterns for different clients
- How to handle service degradation if the review service is slow or down
- Whether this approach will scale to millions of products
- Data consistency issues between inventory and product information
Example 3: Data Processing Pipeline
Code-Level Approach:
function processDataBatch(dataBatch) {
const filteredData = dataBatch.filter(item => isValidFormat(item));
const transformedData = filteredData.map(item => transformData(item));
const results = transformedData.map(item => analyzeData(item));
database.storeBatchResults(results);
return results;
}
This function correctly processes data through several stages.
Architectural Thinking:
An architect asks:
- Should this be a batch process or a streaming solution?
- How to handle partial failures during processing
- Whether to implement a message queue for resilience
- How to scale processing horizontally for large datasets
- Whether to implement the pipeline as separate microservices
- How to monitor and observe the pipeline in production
The Importance of Both Skills
While this article emphasizes the distinction between code-level understanding and architectural thinking, it’s important to recognize that both skills are valuable and complementary:
Code Understanding Informs Architecture
Deep understanding of code and its execution provides insights that inform architectural decisions. For example, knowing the performance characteristics of different data structures helps when designing systems that must process large volumes of information efficiently.
Architects who maintain coding skills can better evaluate the feasibility of their designs and anticipate implementation challenges.
Architecture Guides Code Implementation
Conversely, architectural thinking provides the framework within which code is written. A well-designed architecture makes implementation more straightforward and helps developers focus on the right problems.
For instance, a clear separation of concerns in the architecture makes it easier to write modular, maintainable code.
The Full-Stack Developer Ideal
The most effective developers cultivate both skills, moving fluidly between detailed implementation and high-level design. This versatility is particularly valuable in smaller teams where individuals may need to wear multiple hats.
However, as systems and organizations grow, some specialization often occurs, with some developers focusing more on architectural concerns while others concentrate on implementation details.
Common Pitfalls in the Journey to Architectural Thinking
As developers work to develop architectural thinking, several common pitfalls can hinder progress:
Over-Engineering
Novice architects often fall into the trap of over-engineering solutions, adding unnecessary complexity in an attempt to demonstrate architectural sophistication. This typically manifests as:
- Introducing design patterns where they’re not needed
- Creating excessive layers of abstraction
- Adopting microservices prematurely
- Implementing distributed systems for problems that could be solved more simply
The antidote is to remember that good architecture serves the needs of the problem at hand, not architectural purity for its own sake.
Analysis Paralysis
The awareness of multiple possible approaches and their trade-offs can sometimes lead to decision paralysis. Developers may spend too much time analyzing options without making progress.
Experienced architects know when to make decisions with incomplete information, recognizing that some aspects of the architecture can evolve as more is learned about the problem domain.
Ignoring Non-Functional Requirements
Developers transitioning to architectural thinking sometimes focus exclusively on functional requirements (what the system should do) while neglecting non-functional requirements (how the system should behave).
Critical non-functional considerations include:
- Performance and scalability
- Security and compliance
- Reliability and fault tolerance
- Maintainability and extensibility
- Operability and observability
Neglecting Operational Concerns
Architecture isn’t just about design; it’s also about how systems operate in production. Neglecting operational concerns leads to systems that work in theory but fail in practice.
Architects must consider:
- Deployment strategies
- Monitoring and alerting
- Backup and disaster recovery
- Scaling procedures
- Maintenance windows and procedures
How Technical Interviews Test Both Skills
Technical interviews, particularly at top tech companies, often assess both code-level understanding and architectural thinking:
Coding Interviews
Traditional coding interviews test your ability to:
- Understand algorithms and data structures
- Implement solutions efficiently
- Debug and optimize your code
- Communicate your thought process
These assessments focus primarily on code-level understanding.
System Design Interviews
System design interviews explicitly test architectural thinking by asking candidates to:
- Design a high-level architecture for a complex system
- Make and justify technology choices
- Explain how the system scales and handles failures
- Discuss trade-offs between different approaches
These interviews reveal a candidate’s ability to think beyond individual lines of code to holistic system design.
Behavioral Questions with Technical Depth
Many interviews include behavioral questions that probe for architectural thinking:
- “Tell me about a time you had to make a significant technical decision.”
- “How did you approach scaling a system you worked on?”
- “Describe a situation where you had to balance competing technical requirements.”
These questions assess how candidates apply architectural thinking in real-world situations.
Developing Architectural Thinking Through Practice
Like any skill, architectural thinking improves with deliberate practice. Here are practical exercises to develop this capability:
Design Reviews
Participate in or initiate design reviews where proposed architectures are critically examined. Ask questions like:
- What are the key components and their responsibilities?
- How will this system scale?
- What happens if component X fails?
- How will this design accommodate future requirements?
The process of questioning and evaluating designs strengthens architectural thinking.
System Design Practice
Regularly practice designing systems on paper or whiteboard, even if you won’t implement them. Choose familiar applications or services and try to design their architecture:
- How would you design Twitter’s backend?
- What architecture would you use for a food delivery app?
- How would you design a distributed file storage system?
Compare your designs with publicly available information about how these systems are actually built.
Post-Mortems and Retrospectives
When systems fail or projects encounter difficulties, participate in post-mortems that analyze what happened. These discussions often reveal architectural insights about resilience, scalability, and maintainability.
Similarly, project retrospectives provide opportunities to reflect on architectural decisions and their consequences.
Open Source Contribution
Contributing to open-source projects exposes you to different architectural approaches and the thinking behind them. Even reading through issues and pull requests can provide insights into how architectural decisions are made and debated.
Conclusion: Balancing Code and Architecture
Understanding every line of code is a valuable skill, but it’s only part of what makes a great software developer. The ability to architect solutions requires a broader perspective, systems thinking, and experience with the full lifecycle of software development.
As you progress in your programming journey, deliberately cultivate both skills:
- Maintain your code-level proficiency through regular practice and learning
- Develop architectural thinking by studying system design, analyzing existing systems, and building end-to-end solutions
- Seek feedback from experienced architects and learn from both successes and failures
- Practice explaining and justifying architectural decisions
Remember that the most effective developers can zoom in to understand detailed implementation and zoom out to see the big picture. This versatility is particularly valuable when preparing for technical interviews at top tech companies, where both skills are often assessed.
By recognizing the distinction between code understanding and architectural thinking, and deliberately developing both capabilities, you’ll be well-positioned to tackle complex software challenges and advance your career in the ever-evolving field of software development.