Why Understanding Edge Cases is Key to Solving Coding Problems
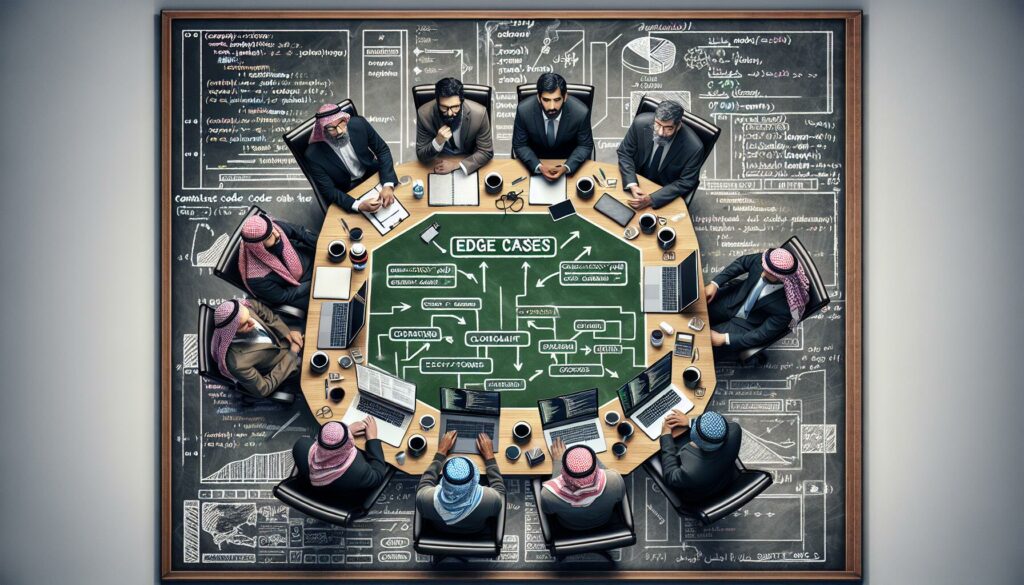
In the world of programming and software development, the ability to solve complex coding problems efficiently is a highly sought-after skill. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, mastering the art of problem-solving is crucial. One of the most important aspects of this skill is understanding and handling edge cases. In this comprehensive guide, we’ll explore why edge cases are so important, how they impact your code, and strategies for identifying and addressing them effectively.
What Are Edge Cases?
Before diving into the importance of edge cases, let’s first define what they are. Edge cases, also known as corner cases, are situations that occur at the extreme ends of the possible range of inputs or conditions for a given problem. These are typically rare or unusual scenarios that may not be immediately obvious when designing a solution but can cause unexpected behavior or errors if not properly handled.
For example, consider a function that calculates the average of a list of numbers. Some edge cases for this problem might include:
- An empty list
- A list with only one element
- A list containing extremely large or small numbers
- A list with non-numeric elements
Each of these scenarios represents an edge case that needs to be considered to ensure the function works correctly in all situations.
Why Are Edge Cases Important?
Understanding and handling edge cases is crucial for several reasons:
1. Ensuring Robustness and Reliability
By addressing edge cases, you make your code more robust and reliable. When your solution can handle extreme or unusual inputs, it becomes more resilient to unexpected situations and less likely to fail in real-world scenarios.
2. Improving Code Quality
Considering edge cases often leads to better overall code quality. It forces you to think critically about your algorithm and implementation, potentially uncovering logical flaws or inefficiencies that might not be apparent when only considering typical inputs.
3. Enhancing User Experience
In user-facing applications, properly handling edge cases can significantly improve the user experience. By anticipating and gracefully handling unusual situations, you can prevent crashes, provide helpful error messages, and ensure a smooth experience for all users.
4. Demonstrating Thoroughness in Technical Interviews
For those preparing for technical interviews, especially at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), demonstrating your ability to identify and handle edge cases is crucial. Interviewers often use edge cases to assess a candidate’s problem-solving skills and attention to detail.
Strategies for Identifying Edge Cases
Now that we understand the importance of edge cases, let’s explore some strategies for identifying them:
1. Analyze Input Boundaries
Consider the extreme ends of your input range. What happens with the smallest or largest possible inputs? What about inputs at the boundaries between different categories or types?
2. Think About Empty or Null Inputs
Always consider what happens when your function or algorithm receives empty collections, null values, or undefined inputs.
3. Consider Type Mismatches
In dynamically typed languages or when working with user input, think about what happens if the input is of an unexpected type.
4. Explore Special Characters and Formatting
For string-based problems, consider inputs with special characters, different encodings, or unusual formatting.
5. Think About Scale
Consider how your solution behaves with extremely large or small inputs. This can help identify potential performance issues or numeric overflow problems.
6. Use the “What If” Technique
Ask yourself “What if…” questions to explore unusual scenarios. For example, “What if all elements are the same?” or “What if the input is already sorted?”
Handling Edge Cases in Your Code
Once you’ve identified potential edge cases, the next step is to handle them effectively in your code. Here are some approaches:
1. Input Validation
Implement thorough input validation at the beginning of your function or algorithm. This allows you to catch and handle invalid inputs early, preventing unexpected behavior later in the code.
Example in Python:
def calculate_average(numbers):
if not numbers:
return None # Handle empty list
if not all(isinstance(num, (int, float)) for num in numbers):
raise ValueError("All elements must be numeric")
return sum(numbers) / len(numbers)
2. Defensive Programming
Use defensive programming techniques to anticipate and handle potential issues. This includes using try-except blocks, checking for null values, and using default values where appropriate.
Example in Java:
public static double calculateAverage(List<Double> numbers) {
if (numbers == null || numbers.isEmpty()) {
throw new IllegalArgumentException("List cannot be null or empty");
}
double sum = 0;
for (Double num : numbers) {
if (num == null) {
throw new IllegalArgumentException("List contains null values");
}
sum += num;
}
return sum / numbers.size();
}
3. Explicit Edge Case Handling
For known edge cases, implement explicit handling logic. This might involve special return values, custom error messages, or alternative processing paths.
Example in JavaScript:
function findMaxElement(arr) {
if (!Array.isArray(arr) || arr.length === 0) {
return null; // Handle empty or non-array input
}
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (typeof arr[i] !== 'number') {
throw new Error('Array contains non-numeric elements');
}
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
4. Comprehensive Testing
Develop a comprehensive test suite that includes edge cases. This helps ensure your solution works correctly for all scenarios and makes it easier to catch regressions when modifying your code.
Example using Python’s unittest framework:
import unittest
def calculate_average(numbers):
if not numbers:
return None
return sum(numbers) / len(numbers)
class TestCalculateAverage(unittest.TestCase):
def test_normal_case(self):
self.assertEqual(calculate_average([1, 2, 3, 4, 5]), 3)
def test_empty_list(self):
self.assertIsNone(calculate_average([]))
def test_single_element(self):
self.assertEqual(calculate_average([42]), 42)
def test_negative_numbers(self):
self.assertEqual(calculate_average([-1, -2, -3]), -2)
def test_large_numbers(self):
self.assertEqual(calculate_average([1e9, 2e9, 3e9]), 2e9)
if __name__ == '__main__':
unittest.main()
Common Edge Cases to Consider
While edge cases can vary depending on the specific problem, here are some common categories to keep in mind:
1. Empty or Null Inputs
Always consider what should happen when your function receives an empty collection (e.g., empty string, empty array) or a null/undefined value.
2. Boundary Values
Test your solution with inputs at the extreme ends of the valid range. For example, if working with integers, consider the minimum and maximum possible values.
3. Single-Element Inputs
For algorithms that work with collections, consider what happens when there’s only one element.
4. Duplicate or Repeated Values
Test scenarios where all elements are the same or where there are many duplicates.
5. Very Large or Very Small Inputs
Consider how your solution behaves with extremely large datasets or very small values that might cause precision issues.
6. Negative Numbers or Zero
For mathematical operations, always consider negative numbers and zero as potential inputs.
7. Invalid Types or Formats
In dynamically typed languages or when working with user input, consider inputs of unexpected types or formats.
8. Special Characters and Unicode
For string manipulation problems, test with special characters, Unicode symbols, and different character encodings.
Real-World Example: Binary Search
Let’s look at a real-world example of how considering edge cases can improve a common algorithm: binary search. Here’s a typical implementation of binary search in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
While this implementation works for many cases, it doesn’t handle several important edge cases:
- Empty array
- Target value smaller than the smallest element or larger than the largest element
- Potential integer overflow when calculating the midpoint
Here’s an improved version that addresses these edge cases:
def binary_search(arr, target):
if not arr:
return -1 # Handle empty array
left, right = 0, len(arr) - 1
# Check if target is out of bounds
if target < arr[left] or target > arr[right]:
return -1
while left <= right:
# Avoid potential integer overflow
mid = left + (right - left) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
This improved version handles the edge cases we identified, making the function more robust and reliable.
The Role of Edge Cases in Technical Interviews
For those preparing for technical interviews, especially at major tech companies, understanding and handling edge cases is crucial. Interviewers often use edge cases to assess a candidate’s problem-solving skills, attention to detail, and ability to write robust code.
Here are some tips for addressing edge cases during technical interviews:
- Clarify the Problem: Always ask clarifying questions about the input range, expected behavior for edge cases, and any assumptions you can make.
- Think Aloud: As you’re solving the problem, verbalize your thought process, including how you’re considering potential edge cases.
- Propose Test Cases: Before diving into coding, propose a set of test cases that include normal scenarios and edge cases. This demonstrates your thorough approach to problem-solving.
- Implement Gracefully: When coding your solution, handle edge cases gracefully. This might involve input validation, error handling, or special return values.
- Test Your Solution: After implementing your solution, walk through it with a few test cases, including edge cases, to demonstrate its correctness.
- Discuss Trade-offs: Be prepared to discuss the trade-offs of your approach, especially in terms of how extensively you handle edge cases versus keeping the code simple and readable.
Conclusion
Understanding and handling edge cases is a critical skill for any programmer, from beginners to experienced developers preparing for technical interviews at top tech companies. By considering edge cases, you create more robust, reliable, and user-friendly code. It demonstrates your attention to detail and your ability to anticipate and handle unexpected situations.
As you continue to develop your coding skills, make it a habit to always consider potential edge cases when designing and implementing your solutions. Practice identifying and handling edge cases in your coding exercises and projects. This will not only improve the quality of your code but also prepare you for the challenges you’ll face in technical interviews and real-world software development.
Remember, the ability to handle edge cases effectively is what often separates good code from great code. By mastering this skill, you’ll be well on your way to becoming a more proficient and sought-after programmer in the competitive world of software development.