Why Understanding Design Patterns Isn’t Improving Your System Design
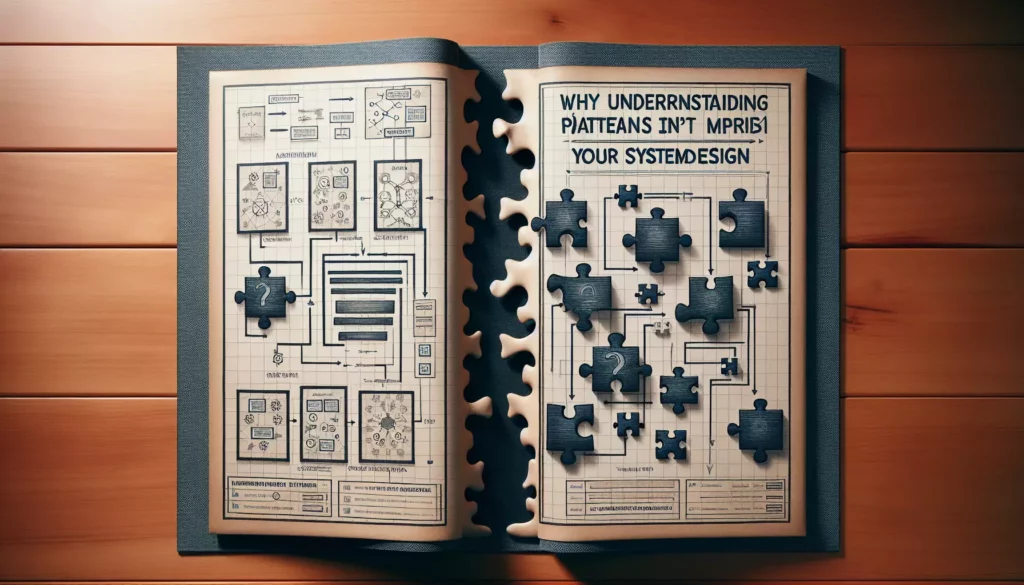
If you’ve been studying software engineering for any amount of time, you’ve likely encountered design patterns. These reusable solutions to common problems in software design have become a cornerstone of programming education. But here’s a reality check: knowing design patterns alone isn’t making you better at system design.
Many developers fall into the trap of collecting design patterns like trading cards, expecting that memorizing the Gang of Four catalog will automatically translate to creating robust, scalable systems. The truth is more nuanced and perhaps a bit uncomfortable.
In this article, we’ll explore why a pattern-first approach often fails to improve your system design skills, and what you should focus on instead to become a more effective software architect.
The False Promise of Pattern Memorization
Design patterns emerged as a way to document recurring solutions that experienced developers found themselves implementing repeatedly. The seminal work “Design Patterns: Elements of Reusable Object-Oriented Software” by Gamma, Helm, Johnson, and Vlissides (the Gang of Four) cataloged 23 patterns that have since become canonical in software engineering education.
However, somewhere along the way, we developed a collective misconception: that memorizing these patterns is the key to mastering system design. This approach is fundamentally flawed for several reasons.
Patterns Are Descriptive, Not Prescriptive
Design patterns were never intended as a cookbook of recipes to follow blindly. They are descriptive observations of solutions that emerged organically from experienced developers solving real problems. When we treat patterns as prescriptive rules, we miss their true value.
Consider the Singleton pattern, perhaps the most recognizable design pattern. It ensures a class has only one instance and provides a global point of access to it:
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Simple enough to memorize, right? But knowing how to implement a Singleton doesn’t tell you when you should use one, or more importantly, when you shouldn’t. In fact, Singletons are often overused, leading to global state, testing difficulties, and tight coupling.
The Context Gap
Design patterns exist within contexts. A Factory Method might be perfect in one situation and overkill in another. Without understanding the underlying problems patterns solve, you can’t effectively decide which pattern (if any) is appropriate.
When interviewing candidates for system design positions, I’ve noticed a recurring issue: developers who recite patterns without connecting them to the problem at hand. They’ll suggest using the Observer pattern because they remember it, not because it addresses the specific challenge we’re discussing.
Why Pattern Knowledge Fails in System Design
System design operates at a higher level of abstraction than individual design patterns. While patterns typically deal with relationships between classes and objects, system design concerns itself with how entire components interact across distributed environments.
Scale Differences
Design patterns typically address code-level concerns within a single application or service. System design, however, deals with:
- Distributed computing across multiple services
- Data storage and retrieval at scale
- Network communication and latency
- Fault tolerance and redundancy
- Horizontal scaling and load balancing
Even if you’ve memorized every pattern in existence, they don’t directly address these system-level concerns. A decorator pattern won’t help you decide between a relational database and a NoSQL solution. A command pattern doesn’t guide you in designing your service discovery mechanism.
The Missing Principles
What’s often missing from pattern-focused learning is an understanding of the underlying principles that guide good design. Patterns are manifestations of principles, not substitutes for them.
For example, the Dependency Inversion Principle states that high-level modules should not depend on low-level modules; both should depend on abstractions. This principle manifests in patterns like Strategy, Factory Method, and Dependency Injection. Understanding the principle helps you recognize when and why these patterns are useful.
Consider this code that violates the principle:
public class ReportGenerator {
private MySQLDatabase database = new MySQLDatabase();
public void generateReport() {
Data data = database.query("SELECT * FROM sales");
// Process data and generate report
}
}
And now with the principle applied:
public class ReportGenerator {
private Database database;
public ReportGenerator(Database database) {
this.database = database;
}
public void generateReport() {
Data data = database.getData();
// Process data and generate report
}
}
public interface Database {
Data getData();
}
public class MySQLDatabase implements Database {
public Data getData() {
return query("SELECT * FROM sales");
}
private Data query(String sql) {
// Execute SQL query
return data;
}
}
The second example follows the Dependency Inversion Principle and uses a form of Dependency Injection. But the value isn’t in naming these patterns; it’s in understanding why this design is more flexible, testable, and maintainable.
What Actually Matters in System Design
If not design patterns, what should you focus on to improve your system design skills? Here are the areas that truly matter:
Understanding Requirements and Constraints
Great system design begins with a deep understanding of what you’re building and why. This includes:
- Functional requirements: What does the system need to do?
- Non-functional requirements: How well does it need to do it? (Performance, reliability, etc.)
- Scale requirements: How many users, transactions, or data points?
- Business constraints: Budget, timeline, existing systems
- Technical constraints: Available technologies, team expertise
No design pattern will help you if you don’t understand what problem you’re solving. In fact, premature application of patterns often leads to overengineered solutions that miss the mark.
Trade-off Analysis
System design is fundamentally about trade-offs. Every decision closes some doors while opening others. Strong system designers can:
- Identify the key trade-offs in a given situation
- Evaluate options against multiple criteria
- Communicate the reasoning behind their choices
- Understand the long-term implications of design decisions
For instance, when choosing between synchronous and asynchronous processing, you’re trading off between consistency and availability. Recognizing this trade-off and its implications is more valuable than knowing how to implement the Command pattern that might facilitate the asynchronous approach.
First Principles Thinking
First principles thinking involves breaking down complex problems into their fundamental truths and then reasoning up from there. Instead of applying patterns by rote, you analyze what’s actually happening:
- What data needs to be stored?
- How does it need to be accessed?
- What operations need to be performed?
- Where are the bottlenecks likely to occur?
This approach leads to solutions tailored to your specific context rather than forcing your problem to fit existing patterns.
System Quality Attributes
Understanding how different architectural choices affect system qualities is crucial:
- Scalability: How the system grows to handle increased load
- Reliability: How the system maintains correctness under stress
- Availability: How the system remains operational
- Performance: How quickly the system responds
- Security: How the system protects data and operations
- Maintainability: How easily the system can be changed
These qualities often conflict with each other, requiring careful balancing based on your specific requirements.
The Right Role for Design Patterns
I’m not suggesting design patterns are worthless. Far from it! They provide valuable shared vocabulary and encapsulate hard-won wisdom. The key is to place them in their proper context within your learning journey.
Patterns as Communication Tools
One of the most valuable aspects of design patterns is the vocabulary they provide. When a developer mentions using the “Observer pattern,” other developers immediately understand the intent without needing a detailed explanation of the implementation.
This communication value extends to documentation and discussions about code. It’s much easier to say “We used a Factory Method here” than to explain the entire rationale from scratch.
Patterns as Implementation Techniques
Once you’ve determined what your system needs to do based on requirements and constraints, patterns can help with the “how” at the implementation level. They provide battle-tested approaches that can save you from common pitfalls.
For example, if you’ve determined that you need a flexible way to create objects without specifying their concrete classes, the Abstract Factory pattern might be appropriate:
public interface DatabaseConnectionFactory {
Connection createConnection();
Statement createStatement();
ResultSet createResultSet();
}
public class MySQLConnectionFactory implements DatabaseConnectionFactory {
public Connection createConnection() {
return new MySQLConnection();
}
public Statement createStatement() {
return new MySQLStatement();
}
public ResultSet createResultSet() {
return new MySQLResultSet();
}
}
public class PostgreSQLConnectionFactory implements DatabaseConnectionFactory {
public Connection createConnection() {
return new PostgreSQLConnection();
}
public Statement createStatement() {
return new PostgreSQLStatement();
}
public ResultSet createResultSet() {
return new PostgreSQLResultSet();
}
}
The pattern itself isn’t the solution; it’s a tool for implementing the solution you’ve already determined you need.
Patterns as Learning Tools
Design patterns can be valuable case studies in good design. By studying why patterns work and the problems they solve, you can internalize design principles that apply more broadly.
For instance, studying the Adapter pattern teaches you about interface segregation and loose coupling. The Composite pattern demonstrates recursive composition and the power of treating individual objects and compositions uniformly.
A Better Approach to Learning System Design
Instead of starting with patterns, here’s a more effective approach to developing your system design skills:
Start with Problems, Not Solutions
Focus on understanding the problems you’re trying to solve first. What are the core challenges? What are the scale requirements? What are the performance constraints?
For example, if you’re designing a social media feed, key challenges might include:
- Fetching relevant content quickly
- Handling spikes in activity
- Ensuring new posts appear promptly
- Managing different content types
- Personalizing feeds for millions of users
Once you understand these challenges, you can evaluate potential solutions against them.
Study Principles, Not Just Patterns
Learn the fundamental principles of good design:
- SOLID principles (Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, Dependency Inversion)
- DRY (Don’t Repeat Yourself)
- Separation of Concerns
- Law of Demeter (Principle of Least Knowledge)
- Composition over Inheritance
These principles transcend specific patterns and apply across different contexts and programming paradigms.
Analyze Real Systems
Study how real-world systems are designed and why they made specific architectural choices:
- Why does Netflix use microservices?
- How does Google achieve such fast search results?
- Why did Twitter move from Ruby on Rails to a more distributed architecture?
- How does Spotify handle music streaming at scale?
Many companies publish engineering blogs and case studies that provide insights into their architecture decisions and the trade-offs they considered.
Practice Incremental Design
System design isn’t a one-time activity where you create the perfect architecture upfront. It’s an iterative process that evolves as you learn more about your problem domain and as requirements change.
Start with a simple design that solves the core problem, then refine it as you encounter specific challenges. This approach allows you to apply patterns where they make sense rather than forcing them into your design prematurely.
Common System Design Pitfalls (Beyond Pattern Misuse)
As you develop your system design skills, be aware of these common pitfalls that transcend specific patterns:
Premature Optimization
One of the most pervasive issues in system design is optimizing too early. This often manifests as:
- Choosing complex distributed architectures before they’re needed
- Implementing caching layers without evidence of performance issues
- Selecting specialized databases when simpler options would suffice
- Over-engineering for scale that may never materialize
Donald Knuth’s famous quote, “Premature optimization is the root of all evil,” applies at the system level as much as it does at the code level. Start with simpler designs and add complexity only when necessary.
Ignoring Operational Concerns
A theoretically elegant design that’s difficult to deploy, monitor, or troubleshoot is not a good design. Consider:
- How will you deploy the system?
- How will you monitor its health?
- How will you detect and diagnose problems?
- How will you roll back changes if needed?
- How will you scale the system as needs grow?
These operational concerns should inform your design from the start, not be treated as afterthoughts.
Reinventing the Wheel
While understanding fundamentals is important, you don’t need to build everything from scratch. Leverage existing tools, frameworks, and services where appropriate:
- Use managed database services instead of setting up your own
- Consider serverless platforms for appropriate workloads
- Utilize existing message queues rather than building your own
- Adopt proven caching solutions instead of custom implementations
The goal is to focus your innovation where it adds the most value to your specific problem, not to recreate infrastructure that others have already perfected.
Case Study: From Patterns to Principles
Let’s examine a concrete example of how focusing on principles rather than patterns leads to better system design.
The Problem: E-commerce Order Processing
You’re designing an order processing system for an e-commerce platform. When a customer places an order:
- Inventory must be checked and reserved
- Payment must be processed
- Order fulfillment must be initiated
- Customer must be notified
- Analytics must be updated
Pattern-First Approach
A developer focused primarily on patterns might approach this by identifying patterns that seem relevant:
- “This involves a sequence of steps, so I’ll use the Chain of Responsibility pattern.”
- “I need to notify multiple systems, so I’ll use the Observer pattern.”
- “I should probably use the Command pattern to encapsulate the order operations.”
- “The Factory Method can create different types of orders.”
While these patterns might be implemented correctly, this approach doesn’t address fundamental system design questions:
- What happens if the payment service is down?
- How do we ensure inventory isn’t double-booked?
- What if order fulfillment takes hours but customers need immediate confirmation?
- How do we handle retry logic for failed operations?
- What consistency guarantees do we need across these different steps?
Principle-First Approach
A principle-focused approach would start with analyzing the fundamental requirements and constraints:
Requirements Analysis:
- Orders must be processed reliably, even if some systems are temporarily unavailable
- Inventory must not be oversold
- Customers need quick confirmation
- Analytics can be eventually consistent
- The system must scale to handle holiday shopping peaks
Architectural Decisions:
- Use event sourcing to maintain a reliable record of all order events
- Implement a SAGA pattern for distributed transactions across services
- Use optimistic concurrency control for inventory updates
- Implement asynchronous processing for non-critical steps
- Design compensating transactions for rollback scenarios
This approach addresses the core system concerns first, then applies appropriate patterns where they serve the overall architecture.
The Implementation
The principle-first approach might result in a system that looks like this:
- Customer places order, generating an OrderCreated event
- Order service stores this event and returns a confirmation to the customer
- Inventory service subscribes to OrderCreated events and attempts to reserve inventory
- If successful, it emits an InventoryReserved event; if not, it emits an InventoryReservationFailed event
- Payment service listens for InventoryReserved events and attempts to process payment
- If successful, it emits a PaymentSucceeded event; if not, a PaymentFailed event
- If PaymentFailed occurs, inventory service listens for this and releases the reservation
- Fulfillment service listens for PaymentSucceeded events and begins fulfillment
- Notification service listens for relevant events and sends appropriate notifications
- Analytics service consumes all events asynchronously for reporting
This design incorporates several patterns (Observer, Command, etc.) but they emerge naturally from the requirements rather than driving the design.
Practical Steps to Improve Your System Design Skills
If you’re looking to enhance your system design abilities beyond pattern memorization, here are practical steps you can take:
1. Build Real Systems
Nothing beats hands-on experience. Build systems that solve real problems, even if they’re small:
- Create a personal project that requires multiple components
- Contribute to open-source projects with non-trivial architectures
- Rebuild simplified versions of existing systems to understand their design choices
As you build, you’ll encounter genuine design challenges that can’t be solved by simply applying a pattern from a book.
2. Analyze Trade-offs Explicitly
For each design decision you make, explicitly consider the trade-offs:
- What am I gaining with this approach?
- What am I giving up?
- Under what circumstances would a different approach be better?
- How does this decision affect other system qualities?
Document these trade-offs, even if it’s just for yourself. The process of articulating them forces clearer thinking.
3. Read Source Code of Well-Designed Systems
Study the source code of well-regarded open-source projects. Look for:
- How they structure their components
- How they manage dependencies
- How they handle error cases
- Where they apply design patterns and why
Projects like Redis, Kubernetes, and the Spring Framework offer masterclasses in system design if you take the time to understand their architectures.
4. Review Post-Mortems and Case Studies
When systems fail, we often learn more than when they succeed. Read post-mortems from companies that have experienced significant outages or performance issues:
- What assumptions proved incorrect?
- What unexpected interactions occurred between components?
- How did they redesign their systems afterward?
These real-world lessons are far more valuable than theoretical pattern knowledge.
5. Practice Explaining Your Designs
Articulating your design decisions to others forces clarity:
- Participate in design reviews at work
- Explain your personal project architecture to fellow developers
- Write blog posts about design challenges you’ve solved
- Draw architecture diagrams and walk others through them
The questions and challenges you receive will highlight gaps in your thinking and help you develop more robust designs.
Conclusion: Beyond Patterns to Principles
Design patterns are a valuable tool in a software architect’s toolkit, but they’re just that: one tool among many. True mastery of system design comes not from memorizing patterns but from understanding fundamental principles, analyzing trade-offs, and developing the judgment to make appropriate decisions in specific contexts.
Instead of asking “Which pattern should I use here?”, ask:
- What problem am I trying to solve?
- What are the key requirements and constraints?
- What principles should guide my solution?
- What trade-offs am I making with this approach?
- How will this design evolve as requirements change?
By shifting your focus from patterns to principles, you’ll develop the critical thinking skills that truly differentiate exceptional system designers from the merely competent. You’ll create architectures that don’t just follow best practices but are thoughtfully tailored to the specific challenges you’re addressing.
Remember: patterns are tools, not solutions. The real art of system design lies in understanding when to apply them, when to adapt them, and when to set them aside in favor of something entirely different. Develop that judgment, and you’ll be well on your way to designing systems that stand the test of time.