Why Understanding Concepts Isn’t the Same as Applying Them in Programming
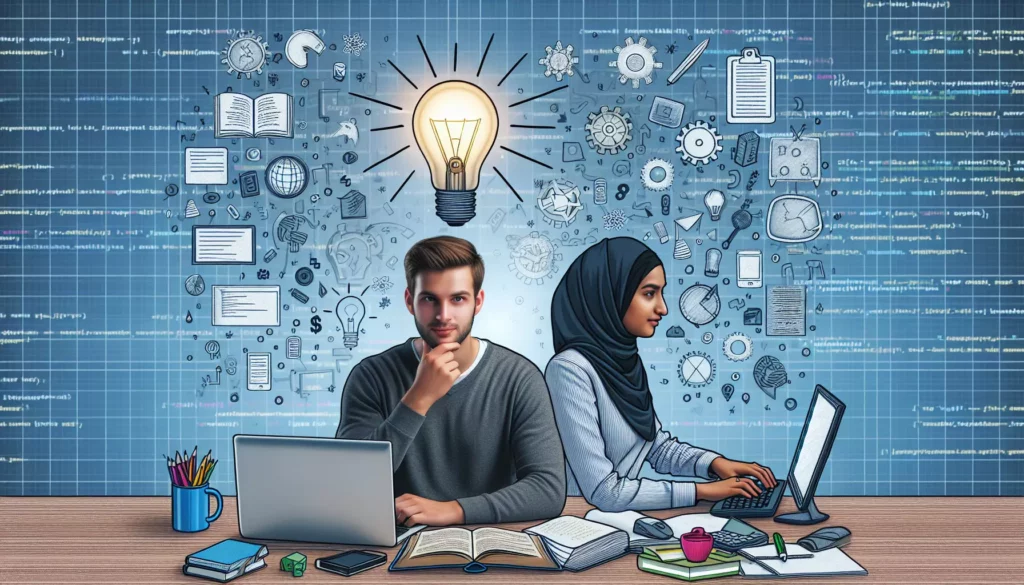
Have you ever watched a YouTube tutorial, understood every concept explained, only to find yourself completely stuck when trying to write the code yourself? Or perhaps you’ve read a programming book cover to cover, nodding along with each explanation, yet when faced with a blank editor, your mind goes equally blank?
If so, you’re experiencing one of the most common challenges in learning to code: the gap between understanding concepts and actually applying them.
This phenomenon isn’t unique to programming, but it’s particularly pronounced in this field. In this article, we’ll explore why understanding programming concepts doesn’t automatically translate to application skills, and more importantly, how to bridge that gap effectively.
The Illusion of Understanding
When we learn programming concepts by reading or watching tutorials, we often experience what psychologists call the “illusion of understanding.” We follow along with the instructor’s explanations and examples, and everything makes perfect sense in the moment.
The problem is that passive learning creates a false sense of mastery. Our brains are processing information, but not in the same way they would if we were actively solving problems. This creates a disconnect that becomes apparent only when we try to apply these concepts independently.
Let’s explore why this happens and what we can do about it.
Understanding vs. Application: What’s the Difference?
Conceptual Knowledge vs. Procedural Knowledge
In cognitive psychology, researchers distinguish between two types of knowledge:
- Conceptual knowledge: Understanding what something is and how it works in theory
- Procedural knowledge: Knowing how to actually do something in practice
When learning to code, conceptual knowledge might involve understanding what a binary search algorithm is and why it’s efficient. Procedural knowledge, on the other hand, involves being able to implement that algorithm correctly in your language of choice.
These two types of knowledge develop differently and require different learning approaches. Reading and watching videos builds conceptual knowledge, but procedural knowledge primarily comes from practice and application.
The Complexity of Programming Application
Applying programming concepts requires more than just recalling information. It involves:
- Problem decomposition: Breaking down complex problems into manageable parts
- Pattern recognition: Identifying which concepts and techniques apply to the current problem
- Syntax recall: Remembering the correct syntax for your programming language
- Error handling: Anticipating and addressing potential issues
- Testing and debugging: Verifying your solution works and fixing it when it doesn’t
Each of these skills requires practice to develop, and they operate simultaneously when you’re applying programming knowledge.
Common Scenarios Where the Gap Becomes Apparent
The Blank Editor Problem
One of the most common manifestations of this gap is what I call the “blank editor problem.” You’ve just learned about a new concept, like recursion or object-oriented programming, and you understand it perfectly. Then you open a code editor to practice and… nothing. Your mind goes blank, and you’re not sure where to start.
This happens because understanding a concept doesn’t automatically give you the ability to generate code from scratch. Generation requires different cognitive processes than comprehension.
The Tutorial Dependency Trap
Another common scenario is becoming dependent on tutorials. You can follow along with a tutorial and write the code without errors, but when asked to make even small modifications to the project, you get stuck.
This happens because following tutorials often involves copying rather than creating, which doesn’t build the same neural pathways needed for independent problem-solving.
The Technical Interview Challenge
Many programmers find that they understand algorithms and data structures conceptually but freeze when asked to implement them during technical interviews. The pressure of the interview amplifies the gap between understanding and application.
This is particularly relevant for those preparing for interviews at major tech companies like Google, Amazon, Apple, or Meta (formerly Facebook), where algorithmic problem-solving is a key assessment area.
The Science Behind the Gap
Cognitive Load Theory
Cognitive load theory helps explain why application is more difficult than understanding. When applying programming concepts, your working memory has to juggle multiple tasks simultaneously:
- Recalling the relevant concepts
- Translating those concepts into code
- Keeping track of variables and program state
- Planning the overall structure of your solution
This creates a much higher cognitive load than simply understanding a concept as it’s explained to you. Your working memory has limited capacity, and when it’s overloaded, performance suffers.
The Role of Mental Models
Effective application requires well-developed mental models of programming concepts. A mental model is an internal representation of how something works in the real world.
When you truly understand a programming concept, you have a robust mental model that allows you to predict how code will behave and reason about it effectively. Building these mental models takes time and experience, not just exposure to information.
The Expertise Reversal Effect
Research in educational psychology has identified something called the “expertise reversal effect.” This phenomenon occurs when teaching methods that work well for beginners become less effective or even counterproductive as learners gain expertise.
For novice programmers, step-by-step tutorials with lots of guidance work well. But as you develop more expertise, you need less guidance and more opportunities for independent problem-solving to continue improving.
Bridging the Gap: Strategies for Turning Understanding into Application
Now that we understand the problem, let’s look at practical strategies to bridge the gap between understanding programming concepts and being able to apply them effectively.
1. Active Learning Over Passive Consumption
The first step is to shift from passive learning (reading, watching) to active learning (doing, creating). This means:
- Code along with tutorials rather than just watching them
- Take notes in your own words to process information more deeply
- Try to predict what will happen next in examples before seeing the answer
- Explain concepts to someone else (or even to yourself) to test your understanding
Active engagement forces your brain to process information more deeply, which strengthens neural connections and improves recall.
2. Deliberate Practice with Incremental Challenges
Deliberate practice is purposeful, systematic practice designed to improve performance. For programming, this means:
- Start with very small, focused exercises that target specific concepts
- Gradually increase complexity as your skills improve
- Seek immediate feedback on your solutions
- Analyze and learn from your mistakes
For example, if you’re learning about arrays, start by creating and manipulating simple arrays before moving on to more complex operations like sorting or filtering.
3. The “Modify, Extend, Create” Progression
When learning a new programming concept, follow this progression:
- Modify existing code that uses the concept (change values, alter behavior slightly)
- Extend existing code by adding new features that use the concept
- Create new code from scratch that applies the concept
This progression gradually reduces scaffolding and increases independence, helping you build application skills step by step.
4. Problem-Based Learning
Problem-based learning involves tackling realistic problems that require applying multiple concepts together. This approach:
- Forces you to think about which concepts apply to the problem
- Builds connections between different areas of knowledge
- Develops your ability to decompose problems
- Creates a more authentic learning experience
Start with well-defined problems that have clear solutions, then gradually move to more open-ended challenges.
5. Spaced Repetition and Retrieval Practice
Research in cognitive science has shown that spaced repetition (reviewing material at increasing intervals) and retrieval practice (actively recalling information from memory) are powerful techniques for building long-term memory.
For programming, this might mean:
- Reviewing concepts you learned days or weeks ago
- Trying to implement solutions without referring to notes or examples
- Using flashcards for key concepts or syntax
- Revisiting old projects and trying to extend them with new features
6. Building Projects That Interest You
Personal projects are one of the most effective ways to bridge the understanding-application gap because they:
- Provide intrinsic motivation that sustains effort through challenges
- Create a meaningful context for applying concepts
- Force you to integrate multiple skills and knowledge areas
- Develop problem-solving abilities in realistic scenarios
Start with small, achievable projects and gradually take on more ambitious ones as your skills grow.
7. Pair Programming and Code Reviews
Learning with others can accelerate your development of application skills:
- Pair programming allows you to observe how others apply concepts and get immediate feedback on your approach
- Code reviews help you identify blind spots in your understanding and learn alternative approaches
- Explaining your code to others forces you to clarify your thinking
Even if you’re learning on your own, joining online communities where you can share code and get feedback provides similar benefits.
Real-World Examples: The Gap in Action
Let’s look at some concrete examples of the gap between understanding and application in programming, and how to address them.
Example 1: Recursion
Recursion is a concept that many programmers understand theoretically but struggle to apply. Here’s a classic example of a recursive function to calculate factorial:
function factorial(n) {
if (n <= 1) {
return 1;
}
return n * factorial(n-1);
}
The concept seems simple: a function that calls itself with a smaller input until it reaches a base case. But when asked to write a recursive solution to a new problem, many programmers get stuck.
Bridging the gap:
- Start by tracing through existing recursive functions step by step, writing down each call and return value
- Modify existing recursive functions to solve slightly different problems
- Practice identifying the base case and recursive case in various problems
- Work through a progression of recursive problems with increasing complexity
Example 2: Object-Oriented Programming
Many learners understand OOP concepts like classes, inheritance, and polymorphism, but struggle to design object-oriented solutions to real problems.
Consider this simple class definition:
class User {
constructor(name, email) {
this.name = name;
this.email = email;
}
sendEmail(message) {
console.log(`Sending email to ${this.email}: ${message}`);
}
}
Understanding this syntax is straightforward, but knowing when to create a class, what properties and methods it should have, and how it should interact with other classes requires deeper application skills.
Bridging the gap:
- Analyze existing object-oriented code to understand design decisions
- Practice identifying objects, attributes, and behaviors in problem descriptions
- Start with UML diagrams before coding to plan your object-oriented design
- Implement the same solution using different OOP patterns to compare approaches
Example 3: Asynchronous Programming
Asynchronous programming concepts like promises, async/await, and callbacks are notoriously difficult to apply correctly, even when the concepts make sense in theory.
Here’s a simple async/await example:
async function fetchUserData() {
try {
const response = await fetch('https://api.example.com/users');
const data = await response.json();
return data;
} catch (error) {
console.error('Error fetching user data:', error);
}
}
The concept seems straightforward, but applying it in complex scenarios with multiple asynchronous operations, error handling, and state management can be challenging.
Bridging the gap:
- Start with simple, controlled examples of asynchronous code
- Practice converting between different async patterns (callbacks to promises to async/await)
- Build small projects that involve multiple async operations
- Use visualization tools to understand the execution flow of async code
Tools and Resources for Bridging the Gap
Fortunately, there are many tools and resources specifically designed to help bridge the gap between understanding and application in programming.
Interactive Coding Platforms
Platforms that combine learning with immediate practice are particularly effective:
- AlgoCademy: Provides interactive coding tutorials with AI-powered assistance that guides you through problem-solving rather than just showing solutions
- LeetCode and HackerRank: Offer structured problem sets with immediate feedback
- CodeWars: Provides progressively challenging “kata” (coding exercises) with exposure to multiple solutions
- Exercism.io: Combines coding exercises with mentor feedback
Project-Based Learning Resources
Resources that focus on building projects help develop application skills:
- The Odin Project: Guides learners through building progressively complex web applications
- App Ideas Collection: A GitHub repository with project ideas at various difficulty levels
- BuildYourOwnX: Tutorials for building your own versions of technologies you use every day
Visualization and Practice Tools
Tools that help you see what’s happening in your code:
- Python Tutor: Visualizes code execution step by step
- Recursion Visualizer: Shows the call stack for recursive functions
- Algorithm Visualizers: Demonstrate how algorithms work with interactive animations
Structured Learning Paths
Resources that provide a structured progression from understanding to application:
- freeCodeCamp: Combines lessons with progressively challenging projects
- CS50: Harvard’s introduction to computer science, focusing on problem-solving
- AlgoCademy’s Technical Interview Preparation: Structured preparation for applying algorithms and data structures in interview settings
The Role of Struggle in Bridging the Gap
It’s important to acknowledge that the struggle to apply concepts is not a sign of failure or lack of aptitude. In fact, this struggle is a necessary part of the learning process.
Productive Struggle vs. Frustration
Educational research distinguishes between “productive struggle” and unproductive frustration:
- Productive struggle: Challenging but achievable tasks that stretch your abilities and lead to growth
- Unproductive frustration: Tasks that are far beyond your current abilities, leading to disengagement
The key is to find the right level of challenge—difficult enough to promote growth, but not so difficult that you give up.
Embracing the Discomfort of Not Knowing
Learning to program effectively requires embracing the discomfort of not immediately knowing how to solve a problem. This discomfort is where real learning happens.
When you encounter a problem you can’t immediately solve:
- Resist the urge to immediately look up the solution
- Break the problem down into smaller parts
- Try different approaches, even if they don’t work
- Reflect on what you’ve learned from failed attempts
This process of struggling with application builds neural pathways that make future application easier.
The Growth Mindset and Learning to Apply
Developing a growth mindset—the belief that abilities can be developed through dedication and hard work—is particularly important for bridging the understanding-application gap.
With a growth mindset, you view challenges in applying concepts as opportunities for growth rather than evidence of limited ability. This perspective helps you persist through the inevitable difficulties of learning to code.
From Learning to Mastery: A Continuous Journey
Bridging the gap between understanding and application isn’t a one-time achievement but a continuous process that evolves as you learn new concepts and technologies.
The Learning Spiral
Rather than seeing learning as a linear progression, think of it as a spiral where you:
- Gain conceptual understanding of a topic
- Practice applying it in simple contexts
- Encounter limitations in your understanding
- Deepen your conceptual knowledge
- Apply the concept in more complex situations
- Repeat the cycle at higher levels of sophistication
This spiral approach acknowledges that understanding and application develop together, each enhancing the other.
From Application to Creation
As you get better at applying programming concepts, you’ll find yourself moving from application to creation—using your skills to build things that didn’t exist before.
This creative aspect of programming is where many find the greatest satisfaction, as you move from solving predefined problems to defining and solving your own problems.
Teaching as the Ultimate Application
Many programmers find that teaching others is the ultimate test of their ability to apply concepts. When you can guide someone else through applying a concept you understand, you’ve truly mastered it.
Consider contributing to open source projects, writing tutorials, or mentoring beginners as a way to solidify your application skills.
Conclusion: Embracing the Challenge
The gap between understanding programming concepts and applying them is real, but it’s also bridgeable with the right approach and mindset.
Remember these key points:
- Understanding without application is incomplete knowledge
- Application skills develop through deliberate practice and productive struggle
- The journey from understanding to application is a continuous spiral, not a linear path
- Tools and resources exist specifically to help bridge this gap
- The struggle to apply concepts is not a sign of failure but a necessary part of learning
By acknowledging the gap between understanding and application and actively working to bridge it, you’ll develop not just theoretical knowledge but practical programming skills that allow you to solve real problems and create meaningful software.
The next time you find yourself understanding a concept perfectly but struggling to apply it, remember: this is not a failure of comprehension but an opportunity for growth. Embrace the challenge, practice deliberately, and watch as your application skills develop alongside your conceptual understanding.
Taking the Next Step
If you’re ready to bridge the gap between understanding and application in your programming journey, consider these next steps:
- Choose one programming concept you understand but struggle to apply
- Find or create three small, focused exercises to practice applying it
- Commit to working through these exercises without looking at solutions first
- Reflect on what was difficult and what strategies helped you succeed
- Apply what you’ve learned to a slightly more complex problem
By systematically working to bridge the understanding-application gap, you’ll develop the practical programming skills that make the difference between knowing about coding and being able to code effectively.
Remember, every expert programmer once struggled with applying the concepts they now use effortlessly. The difference isn’t innate talent but persistent practice in application.