Why Top Companies Reject Engineers Who Know the Right Algorithms
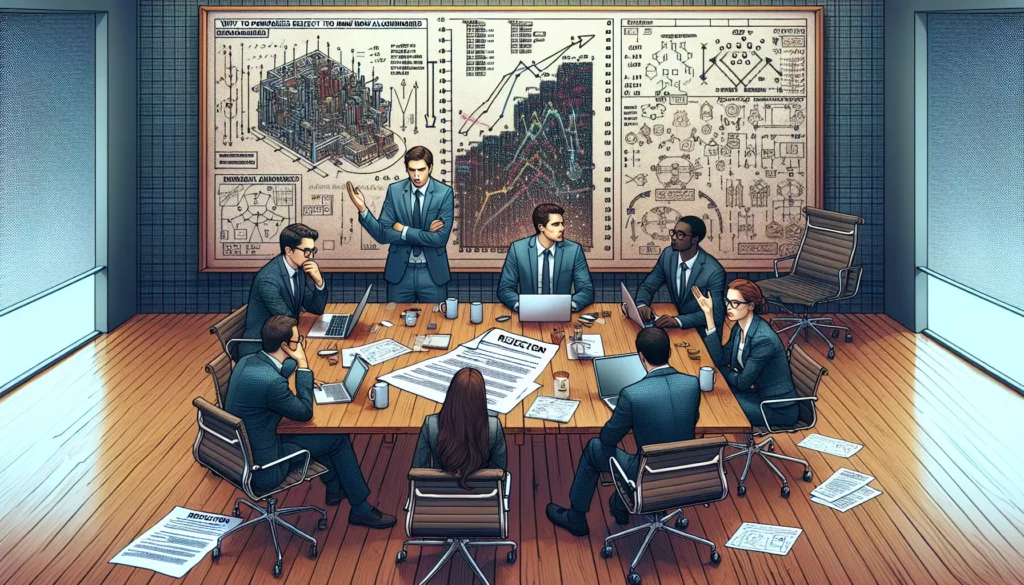
In the competitive landscape of tech recruitment, especially at prestigious companies like Google, Amazon, Meta (formerly Facebook), Apple, and Netflix (often grouped as FAANG), there’s a common misconception that mastering algorithms and data structures is the golden ticket to securing a job offer. However, the reality is more nuanced. Many candidates who excel at algorithmic problem solving still face rejection during the interview process.
This phenomenon can be puzzling: why would companies turn away engineers who demonstrate proficiency in the very skills these organizations publicly emphasize? Let’s explore the multifaceted reasons behind this apparent contradiction and what it means for aspiring software engineers.
The Algorithm Paradox: Necessary but Not Sufficient
Algorithm knowledge represents just one dimension of what makes an effective software engineer. While solving complex algorithmic challenges demonstrates analytical thinking and problem solving capabilities, top companies are looking for a more comprehensive skill set.
Tech giants have evolved their hiring processes to evaluate candidates holistically, recognizing that building successful products requires more than just algorithmic excellence. This shift reflects a deeper understanding of what makes engineers effective in real world environments.
Beyond the Whiteboard: What Companies Actually Need
The disconnect between algorithmic prowess and job offers often stems from a misalignment between what candidates prepare for and what companies truly value in their engineering teams:
- Practical implementation skills: Converting algorithmic solutions into production ready code
- System design capabilities: Understanding how components work together at scale
- Collaboration and communication: Working effectively with diverse teams
- Product thinking: Considering user needs and business requirements
- Adaptability: Learning new technologies and approaches quickly
Algorithmic knowledge serves as a foundation, but without these complementary skills, even the most algorithm savvy engineers may struggle to contribute effectively in complex corporate environments.
The Real Reasons for Rejection
Let’s examine the specific factors that often lead to rejection, even when candidates demonstrate strong algorithmic abilities:
1. Coding Implementation Issues
Many candidates can describe algorithms conceptually but struggle with implementation. Interviewers frequently observe:
- Code that’s difficult to read or maintain
- Poor variable naming and function structure
- Inability to translate pseudocode into working solutions
- Bugs and edge cases missed during implementation
- Inefficient implementations that would cause performance issues
Consider this example: a candidate might correctly identify that a problem requires a breadth first search algorithm, but their implementation might be riddled with bugs, lack proper queue management, or fail to handle edge cases properly.
// Problematic BFS implementation example
function bfs(graph, start) {
let visited = []; // Should be a Set for O(1) lookups
let queue = [start];
while(queue.length > 0) {
let node = queue.shift(); // Inefficient for large queues
visited.push(node); // No check if already visited
// Missing edge case handling
for(let neighbor of graph[node]) {
queue.push(neighbor); // Might add duplicates
}
}
return visited;
}
This code shows algorithmic knowledge but reveals implementation weaknesses that would cause problems in production environments.
2. Communication Breakdowns
Technical interviews evaluate communication as much as coding ability. Common communication issues include:
- Inability to clearly explain thought processes
- Failure to ask clarifying questions before diving into solutions
- Not verbalizing tradeoffs between different approaches
- Resistance to hints or guidance from interviewers
- Difficulty discussing technical concepts in accessible language
Even brilliant solutions lose their impact when candidates can’t effectively communicate their reasoning or collaborate with interviewers during the problem solving process.
3. System Design Deficiencies
As engineers progress in their careers, system design skills become increasingly important. Many algorithmically strong candidates fall short in areas such as:
- Designing scalable architectures
- Understanding distributed systems concepts
- Making appropriate technology choices
- Addressing non functional requirements (reliability, availability, etc.)
- Considering deployment and operational concerns
A candidate might expertly code a sorting algorithm but struggle when asked to design a system that processes millions of user interactions in real time.
4. Lack of Product Sense
Engineers at top companies don’t just implement specifications; they contribute to product decisions. Candidates often fail to demonstrate:
- Understanding of user needs and business contexts
- Ability to prioritize features based on impact
- Awareness of how technical decisions affect user experience
- Consideration of metrics and success criteria
- Balancing technical elegance with practical business requirements
This dimension becomes especially important at companies where engineers are expected to work closely with product managers and designers.
5. Cultural Misalignment
Each organization has its own values and working style. Rejection often occurs when there’s a mismatch between the candidate’s approach and the company culture:
- Collaboration style (individual contributor vs. team player)
- Problem solving approach (quick solutions vs. thoughtful design)
- Learning orientation (fixed mindset vs. growth mindset)
- Work ethic and values alignment
- Communication preferences and interpersonal dynamics
Many companies explicitly evaluate “culture fit” or “values alignment” as part of their interview process, sometimes weighing these factors as heavily as technical skills.
Case Studies: When Algorithm Knowledge Isn’t Enough
Let’s examine some real world scenarios (with details modified for privacy) that illustrate how algorithmic knowledge alone doesn’t guarantee success:
Case 1: The Perfect Algorithm, Imperfect Implementation
An experienced developer interviewing at a major search engine company correctly identified that a particular problem required a trie data structure and a depth first search approach. However, their implementation was problematic:
- The code contained multiple off by one errors
- The candidate didn’t properly handle edge cases
- When bugs were pointed out, the candidate struggled to debug effectively
- The final solution, while algorithmically correct, would have been unmaintainable
Despite knowing the right algorithm, the candidate was rejected because implementation quality matters in production environments.
Case 2: Algorithmic Excellence, Communication Challenges
A PhD in computer science with publications in algorithmic optimization interviewed at a social media company. While solving problems:
- They jumped directly to complex solutions without explaining their reasoning
- When asked to consider alternative approaches, they became defensive
- They used highly academic terminology that team members found difficult to follow
- They didn’t engage collaboratively with interviewers who offered hints
Despite solving all problems correctly, the candidate was rejected because effective teamwork requires clear communication and collaboration.
Case 3: Algorithm Expert, System Design Novice
A candidate who had mastered competitive programming interviewed at a streaming service company:
- They excelled at algorithm questions, providing optimal solutions quickly
- When asked to design a video recommendation system, they focused exclusively on the ranking algorithm
- They overlooked critical aspects like data storage, caching strategies, and service architecture
- They couldn’t articulate how their solution would scale to millions of users
The rejection followed because the role required broader system thinking beyond algorithmic optimization.
What Top Companies Are Really Looking For
To understand why algorithmically strong candidates face rejection, we need to examine what top tech companies truly value in their engineering hires:
1. Technical Breadth and Depth
Companies seek engineers with both specialized knowledge and versatile capabilities:
- Language proficiency: Deep knowledge of programming languages beyond syntax
- Tool mastery: Familiarity with development environments, version control, and deployment tools
- Framework understanding: Experience with relevant frameworks and libraries
- Testing knowledge: Ability to write effective unit and integration tests
- Performance optimization: Skills in profiling and improving application performance
Algorithmic knowledge is just one component of this broader technical toolkit.
2. Problem Solving Process
How candidates approach problems reveals as much as their final solutions:
- Problem clarification: Asking questions to fully understand requirements
- Systematic approach: Breaking complex problems into manageable components
- Incremental development: Building solutions iteratively, starting with simple approaches
- Testing mindset: Proactively identifying edge cases and validating solutions
- Adaptability: Pivoting when initial approaches don’t work
Interviewers often value the journey as much as the destination, especially when it demonstrates thoughtful problem solving.
3. Engineering Maturity
Beyond technical skills, companies evaluate engineering maturity:
- Code quality: Writing clean, maintainable, and well structured code
- Design patterns: Applying appropriate patterns to solve common problems
- Documentation: Creating clear documentation and meaningful comments
- Technical debt awareness: Understanding tradeoffs between quick solutions and long term maintenance
- Debugging skills: Systematically identifying and fixing issues
These qualities reflect an engineer’s ability to contribute to codebases that will be maintained for years.
4. Collaboration and Communication
Software development is inherently collaborative, requiring:
- Clear articulation: Explaining technical concepts at appropriate levels of abstraction
- Active listening: Incorporating feedback and building on others’ ideas
- Documentation: Creating clear documentation and meaningful comments
- Knowledge sharing: Teaching and mentoring teammates
- Constructive feedback: Giving and receiving code reviews effectively
These skills become increasingly important as engineers advance in their careers and take on more leadership responsibilities.
5. Growth Mindset and Learning Ability
Technology evolves rapidly, making adaptability essential:
- Curiosity: Genuine interest in learning new technologies and approaches
- Self improvement: Actively seeking feedback and addressing weaknesses
- Knowledge application: Transferring concepts between different domains
- Learning efficiency: Quickly mastering new languages, frameworks, or tools
- Resilience: Persisting through challenges and setbacks
Companies invest heavily in candidates who demonstrate the ability to grow and adapt as technologies and business needs evolve.
How Interview Processes Evaluate Beyond Algorithms
Understanding how companies structure their interviews reveals the multidimensional evaluation taking place:
The Full Interview Loop
Most top companies use a comprehensive interview process that examines different facets of a candidate’s abilities:
- Coding interviews: Evaluating problem solving and implementation skills
- System design interviews: Assessing architectural thinking and scalability considerations
- Behavioral interviews: Exploring past experiences and working style
- Technical deep dives: Probing specialized knowledge relevant to the role
- Cross functional interviews: Gauging ability to work with product managers, designers, etc.
Candidates might excel in algorithm focused rounds but fall short in these other dimensions.
Hidden Evaluation Criteria
Even within coding interviews, interviewers are often evaluating aspects beyond algorithmic correctness:
- Code organization: Structure, modularity, and readability
- Testing approach: How candidates validate their solutions
- Problem decomposition: Breaking complex problems into manageable parts
- Optimization awareness: Understanding when to optimize and when to prioritize clarity
- Tool proficiency: Comfort with coding environments and debugging tools
These “hidden” criteria often determine success, even when the algorithmic approach is correct.
The Feedback Loop
After interviews, hiring committees typically review feedback across multiple dimensions:
- Technical skills: Algorithm knowledge, coding ability, system design
- Problem solving: Approach, adaptability, and analytical thinking
- Communication: Clarity, listening skills, and collaboration
- Culture alignment: Values fit and working style compatibility
- Leadership potential: Growth trajectory and impact beyond individual contribution
Candidates need to meet thresholds across all these areas, not just excel in one dimension.
How to Succeed Beyond Algorithmic Knowledge
For engineers looking to improve their chances at top companies, here are strategies that address the full spectrum of skills required:
1. Develop Implementation Excellence
Move beyond algorithmic theory to practical implementation:
- Practice writing clean, well structured code for algorithmic solutions
- Focus on edge cases and error handling in your implementations
- Review and refactor your solutions to improve readability and maintainability
- Learn language specific best practices and idioms
- Build complete projects that apply algorithms in real world contexts
Consider this improved implementation of the earlier BFS example:
/**
* Performs breadth first search on a graph starting from a given node
* @param {Object} graph - An adjacency list representation of the graph
* @param {string|number} startNode - The node to start traversal from
* @returns {Array} - The nodes in BFS traversal order
*/
function breadthFirstSearch(graph, startNode) {
// Handle edge cases
if (!graph || !startNode || !(startNode in graph)) {
return [];
}
const visited = new Set([startNode]);
const result = [];
const queue = [startNode];
while (queue.length > 0) {
const currentNode = queue.shift();
result.push(currentNode);
// Process all neighbors
const neighbors = graph[currentNode] || [];
for (const neighbor of neighbors) {
if (!visited.has(neighbor)) {
visited.add(neighbor);
queue.push(neighbor);
}
}
}
return result;
}
This improved version demonstrates not just algorithmic knowledge but implementation quality that would be valued in a professional environment.
2. Master Communication During Problem Solving
Develop the ability to articulate your thinking clearly:
- Practice explaining your approach before writing code
- Verbalize tradeoffs between different solutions
- Ask clarifying questions to ensure you understand requirements
- Use appropriate technical terminology without jargon overload
- Engage collaboratively with interviewers, incorporating their feedback
Consider recording yourself solving problems aloud or practicing with peers to receive feedback on communication clarity.
3. Build System Design Skills
Expand your knowledge beyond algorithms to system architecture:
- Study distributed systems principles and patterns
- Learn about scalability challenges and solutions
- Understand data storage options and their tradeoffs
- Explore caching strategies and their applications
- Practice designing systems that handle realistic constraints and requirements
System design skills become increasingly important as you target senior roles or positions at companies with large scale applications.
4. Develop Product Thinking
Cultivate an understanding of how technical decisions impact users and business outcomes:
- Consider user needs and experiences when designing solutions
- Learn to prioritize features based on business impact
- Understand metrics that matter for different types of products
- Practice making technical tradeoffs with business constraints in mind
- Collaborate with product managers or designers to understand their perspective
Engineers who demonstrate product thinking often stand out in interviews and have greater impact in their roles.
5. Demonstrate Cultural Alignment
Research and align with the values of companies you’re targeting:
- Study the company’s engineering blog and technical talks
- Understand their core values and how they manifest in daily work
- Prepare stories that demonstrate your alignment with these values
- Ask thoughtful questions about team dynamics and work processes
- Show authentic interest in the company’s mission and products
Cultural alignment doesn’t mean conformity; it means showing how your unique perspective and working style can contribute positively to the organization.
Real World Success Stories: Beyond Algorithms
Let’s examine how candidates succeeded by demonstrating the full spectrum of skills valued by top companies:
Case 1: The Thoughtful Implementer
A mid level engineer interviewing at a financial technology company:
- Chose a straightforward algorithmic approach rather than an overly complex one
- Implemented the solution with exceptional clarity and structure
- Added comprehensive error handling and input validation
- Wrote clear comments explaining key decisions
- Proactively discussed testing strategies for their solution
Despite not using the most optimal algorithm in one case, they received an offer because their implementation demonstrated production readiness and engineering maturity.
Case 2: The Collaborative Problem Solver
A senior engineer interviewing at an e commerce platform:
- Started by asking clarifying questions about requirements and constraints
- Discussed multiple approaches before choosing one, explaining tradeoffs
- Incorporated the interviewer’s hints effectively
- Communicated clearly at each step of their solution
- Responded positively to feedback and adjusted their approach
Their strong communication and collaboration skills, combined with solid technical solutions, led to an offer for a senior role.
Case 3: The System Thinker
A backend engineer interviewing at a video streaming service:
- Demonstrated solid algorithm skills in coding interviews
- Excelled in system design by considering scalability, reliability, and performance
- Discussed database choices with nuanced understanding of tradeoffs
- Considered operational aspects like monitoring and deployment
- Connected technical decisions to user experience impacts
Their holistic thinking about systems, beyond just algorithms, secured them a senior position on a critical infrastructure team.
The Balanced Preparation Approach
Based on these insights, here’s a balanced preparation strategy for engineers targeting top companies:
1. Technical Preparation Beyond Algorithms
- Algorithm fundamentals: Master core algorithms and data structures
- Implementation practice: Write clean, well structured code for algorithmic problems
- System design: Study architectural patterns and distributed systems concepts
- Language mastery: Develop deep knowledge of your primary programming language
- Testing strategies: Learn effective testing approaches for different scenarios
Allocate your preparation time across these areas rather than focusing exclusively on algorithmic problems.
2. Soft Skills Development
- Communication practice: Explain technical concepts clearly and concisely
- Collaboration techniques: Learn to incorporate feedback and build on others’ ideas
- Behavioral preparation: Reflect on past experiences and extract meaningful lessons
- Question formulation: Practice asking clarifying questions effectively
- Feedback seeking: Actively request and incorporate feedback from practice sessions
These skills are often best developed through pair programming, mock interviews, and collaborative projects.
3. Company Specific Research
- Values alignment: Understand each company’s core values and principles
- Technical environment: Research their tech stack and engineering challenges
- Interview process: Learn about their specific interview structure and focus areas
- Product understanding: Develop insights about their products and user base
- Team dynamics: Research how teams are structured and work together
Tailoring your preparation to each company demonstrates genuine interest and increases the likelihood of cultural alignment.
Conclusion: The Holistic Engineer
The phenomenon of algorithmically strong candidates facing rejection reveals an important truth about modern software engineering: it demands a diverse set of skills beyond algorithmic problem solving. Top companies aren’t just building clever algorithms; they’re building complex products that serve millions of users in competitive markets.
To succeed in this environment, engineers need to develop a holistic skill set that encompasses technical excellence, effective communication, system thinking, product awareness, and cultural alignment. While algorithm knowledge remains important, it functions as a foundation rather than the complete structure.
For aspiring engineers, this broader perspective offers both a challenge and an opportunity. The path to success at top companies may be more multifaceted than it initially appears, but it also allows individuals with diverse strengths to shine. By developing the full spectrum of skills valued in professional engineering environments, candidates can position themselves not just to pass interviews but to thrive in their careers.
The most successful engineers recognize that algorithms are tools in service of larger goals: building products that solve real problems, collaborating effectively with diverse teams, and creating systems that scale and evolve. By embracing this holistic view, they transform from algorithm experts into the kind of engineers that top companies eagerly welcome to their teams.