Why Thinking Like an Interviewer Can Help You Solve Problems Faster
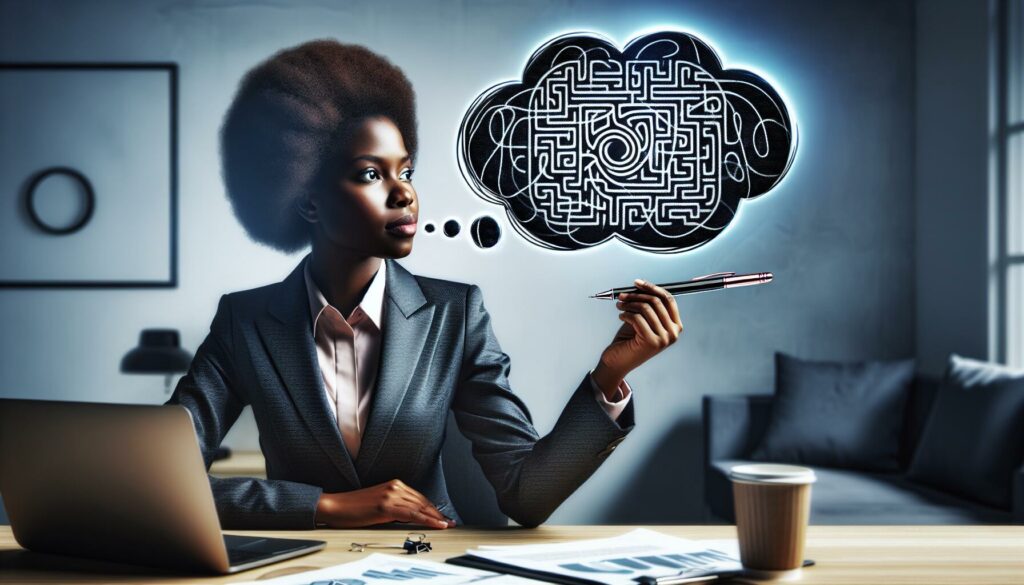
In the competitive world of tech interviews, particularly for positions at prestigious companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), mastering the art of problem-solving is crucial. However, many candidates overlook a powerful strategy that can significantly improve their performance: thinking like an interviewer. This approach not only helps you solve problems faster but also aligns your solutions with what interviewers are truly looking for. In this comprehensive guide, we’ll explore why adopting the interviewer’s perspective is so valuable and how it can transform your problem-solving skills.
Understanding the Interviewer’s Perspective
Before diving into specific strategies, it’s essential to understand what interviewers are evaluating during a technical interview. While the exact criteria may vary depending on the company and position, there are several key aspects that most interviewers focus on:
1. Problem-Solving Approach
Interviewers are keen to observe how you tackle a problem from start to finish. They’re not just interested in whether you can arrive at the correct solution, but how you get there. This includes:
- How you analyze and break down the problem
- Your ability to identify key constraints and edge cases
- The logical steps you take to develop a solution
- Your communication skills as you explain your thought process
2. Optimization Skills
In many cases, finding a working solution is just the beginning. Interviewers want to see if you can optimize your initial approach for better performance. This involves:
- Analyzing time and space complexity
- Identifying bottlenecks in your algorithm
- Proposing and implementing more efficient solutions
- Understanding the trade-offs between different approaches
3. Code Quality and Clarity
Writing clean, readable, and maintainable code is a crucial skill for any software developer. Interviewers assess:
- Your coding style and adherence to best practices
- The clarity and structure of your code
- Your use of appropriate data structures and algorithms
- How well you handle error cases and edge conditions
4. Technical Knowledge
While problem-solving is at the forefront, interviewers also gauge your understanding of fundamental concepts and technologies relevant to the position. This might include:
- Data structures and algorithms
- System design principles
- Language-specific features and best practices
- Relevant frameworks and tools
5. Adaptability and Learning
Interviewers often introduce new constraints or ask follow-up questions to see how well you can adapt your solution. They’re looking for:
- Flexibility in your thinking
- Ability to incorporate feedback
- How you handle challenging or unfamiliar scenarios
Strategies for Aligning Your Thought Process with the Problem’s Intent
Now that we understand what interviewers are looking for, let’s explore strategies to align your thought process with their expectations, which can lead to faster and more effective problem-solving.
1. Start with Clarifying Questions
Before diving into a solution, take a moment to ask clarifying questions. This shows the interviewer that you’re thorough and helps you avoid misunderstandings that could lead you down the wrong path. Some good questions to ask include:
- What are the input constraints?
- Are there any specific performance requirements?
- How should the function/method handle edge cases or invalid inputs?
- Is there any additional context about the problem’s real-world application?
By asking these questions, you’re not only gathering important information but also demonstrating to the interviewer that you approach problems methodically and consider various aspects before coding.
2. Think Aloud and Communicate Your Process
Interviewers want to understand your thought process, so make it a habit to think aloud as you work through the problem. This includes:
- Explaining your initial thoughts and approach
- Discussing potential solutions and their trade-offs
- Walking through your code as you write it
- Explaining your reasoning for specific decisions
This practice not only helps the interviewer follow your logic but also allows them to provide hints or guidance if you’re heading in the wrong direction. Moreover, verbalizing your thoughts can often lead to insights or realizations that help you solve the problem faster.
3. Break Down the Problem
Large, complex problems can be overwhelming. Demonstrate your problem-solving skills by breaking the problem down into smaller, manageable components. This approach has several benefits:
- It makes the problem less daunting and easier to approach
- It allows you to tackle one piece at a time, making progress visible
- It helps identify subproblems that might have known solutions or algorithms
- It makes your thought process clearer to the interviewer
For example, if you’re asked to implement a function to validate a Sudoku board, you might break it down into checking rows, columns, and 3×3 sub-grids separately.
4. Consider Multiple Approaches
Before settling on a solution, briefly consider and discuss multiple approaches. This shows the interviewer that you’re not fixated on a single method and can evaluate different strategies. For each approach, consider:
- Time and space complexity
- Ease of implementation
- Scalability and performance characteristics
- Potential drawbacks or limitations
Even if you end up choosing your initial idea, this exercise demonstrates your ability to think critically about different solutions.
5. Start with a Brute Force Solution
If you’re struggling to find an optimal solution immediately, it’s often beneficial to start with a brute force approach. This strategy has several advantages:
- It ensures you have a working solution, even if it’s not the most efficient
- It helps you understand the problem better, which can lead to optimizations
- It demonstrates to the interviewer that you can at least solve the problem basically
- It provides a baseline for discussing improvements and optimizations
After implementing the brute force solution, you can discuss its limitations and potential optimizations, showing your ability to iterate and improve your code.
6. Focus on Code Quality from the Start
While speed is important, don’t sacrifice code quality for quick implementation. Interviewers are looking for clean, readable, and maintainable code. Some tips to keep in mind:
- Use meaningful variable and function names
- Keep your code modular and well-structured
- Comment on complex logic or non-obvious decisions
- Handle edge cases and potential errors gracefully
Writing quality code from the start not only impresses the interviewer but also makes it easier for you to debug and optimize your solution later.
7. Anticipate and Address Edge Cases
Interviewers often have specific edge cases in mind when they present a problem. By proactively identifying and addressing these cases, you demonstrate thoroughness and attention to detail. Common edge cases to consider include:
- Empty or null inputs
- Extremely large or small inputs
- Boundary conditions (e.g., first or last element of an array)
- Invalid or unexpected inputs
Discussing these cases before the interviewer brings them up shows that you’re thinking comprehensively about the problem.
8. Analyze Time and Space Complexity
After implementing a solution, always analyze its time and space complexity. This demonstrates your understanding of algorithmic efficiency and your ability to evaluate your own code critically. Be prepared to:
- Explain the Big O notation of your solution
- Identify the dominant operations that contribute to the complexity
- Discuss how the complexity changes with different input sizes
- Propose potential optimizations if the current complexity is suboptimal
This analysis often leads to insights about how to improve your solution, which is exactly what interviewers want to see.
9. Use Appropriate Data Structures and Algorithms
Choosing the right data structures and algorithms can significantly impact the efficiency of your solution. Demonstrate your knowledge by:
- Selecting data structures that best fit the problem requirements
- Applying well-known algorithms when appropriate
- Explaining why your chosen approach is suitable for the problem
- Discussing alternatives and their trade-offs
For example, if you’re dealing with a problem that requires frequent lookups, consider using a hash table instead of repeatedly searching through an array.
10. Test Your Code
Before declaring your solution complete, take the time to test it. This shows the interviewer that you value correctness and are proactive about finding and fixing bugs. Consider:
- Writing test cases for normal inputs
- Testing edge cases and boundary conditions
- Walking through your code with a small example
- Checking for off-by-one errors or other common mistakes
If you find issues during testing, calmly explain your process for identifying and fixing the bug. This demonstrates your debugging skills and attention to detail.
Practical Example: Solving a Common Interview Problem
Let’s walk through a practical example of how thinking like an interviewer can help you solve a problem faster and more effectively. We’ll use a classic interview question: “Find the kth largest element in an unsorted array.”
Step 1: Clarify the Problem
Before jumping into coding, ask clarifying questions:
- Is the array guaranteed to have at least k elements?
- How should we handle duplicate elements?
- Are we looking for the kth largest distinct element or just the kth largest including duplicates?
- What should we return if k is out of bounds?
Step 2: Consider Multiple Approaches
Think about different ways to solve the problem:
- Sort the array and return the kth element from the end (simple but not optimal)
- Use a min-heap of size k to keep track of the k largest elements
- Use the QuickSelect algorithm (optimal for average case)
Discuss the time and space complexity of each approach with the interviewer.
Step 3: Implement the Chosen Solution
Let’s implement the QuickSelect algorithm, as it’s often the most efficient solution for this problem. Here’s a Python implementation:
import random
def find_kth_largest(nums, k):
if not nums or k < 1 or k > len(nums):
return None # Handle edge cases
def partition(left, right, pivot_index):
pivot = nums[pivot_index]
nums[pivot_index], nums[right] = nums[right], nums[pivot_index]
store_index = left
for i in range(left, right):
if nums[i] < pivot:
nums[store_index], nums[i] = nums[i], nums[store_index]
store_index += 1
nums[right], nums[store_index] = nums[store_index], nums[right]
return store_index
def select(left, right, k_smallest):
if left == right:
return nums[left]
pivot_index = random.randint(left, right)
pivot_index = partition(left, right, pivot_index)
if k_smallest == pivot_index:
return nums[k_smallest]
elif k_smallest < pivot_index:
return select(left, pivot_index - 1, k_smallest)
else:
return select(pivot_index + 1, right, k_smallest)
return select(0, len(nums) - 1, len(nums) - k)
Step 4: Explain Your Solution
As you implement the solution, explain your thought process:
- The QuickSelect algorithm is based on the partitioning step of QuickSort
- We randomly choose a pivot to avoid worst-case scenarios
- The algorithm recursively partitions the array until it finds the kth largest element
- This approach has an average time complexity of O(n) and worst-case O(n^2)
- The space complexity is O(1) as it’s done in-place
Step 5: Test Your Solution
Demonstrate testing with various inputs:
print(find_kth_largest([3,2,1,5,6,4], 2)) # Expected: 5
print(find_kth_largest([3,2,3,1,2,4,5,5,6], 4)) # Expected: 4
print(find_kth_largest([1], 1)) # Expected: 1
print(find_kth_largest([1,2,3,4,5], 6)) # Expected: None
Step 6: Discuss Optimizations and Trade-offs
After implementing and testing the solution, discuss potential optimizations or alternative approaches:
- The current implementation modifies the original array. We could create a copy if preserving the original is important.
- For very large arrays, we might consider using a min-heap approach to guarantee O(n log k) time complexity.
- If this operation needs to be performed frequently on the same array, we might consider maintaining a sorted data structure.
Conclusion
Thinking like an interviewer is a powerful strategy that can significantly improve your problem-solving speed and effectiveness during technical interviews. By understanding what interviewers are looking for and aligning your approach with their expectations, you can:
- Demonstrate your problem-solving skills more effectively
- Communicate your thought process clearly
- Anticipate and address potential issues before they’re raised
- Show your ability to optimize and improve solutions
- Highlight your technical knowledge and coding best practices
Remember, the goal of a technical interview is not just to solve the problem, but to showcase your overall abilities as a developer. By adopting the interviewer’s perspective, you can ensure that you’re presenting your skills in the best possible light.
As you prepare for interviews, practice these strategies with a variety of coding problems. Use platforms like AlgoCademy to work through interactive coding tutorials and leverage AI-powered assistance to refine your approach. With consistent practice and the right mindset, you’ll be well-equipped to tackle even the most challenging interview questions at top tech companies.
Ultimately, the ability to think like an interviewer is a skill that extends beyond just passing technical interviews. It cultivates a mindset of thorough problem analysis, effective communication, and continuous improvement – qualities that are invaluable throughout your career as a software developer.