Why the Real Problem is Understanding the Problem, Not Coding It
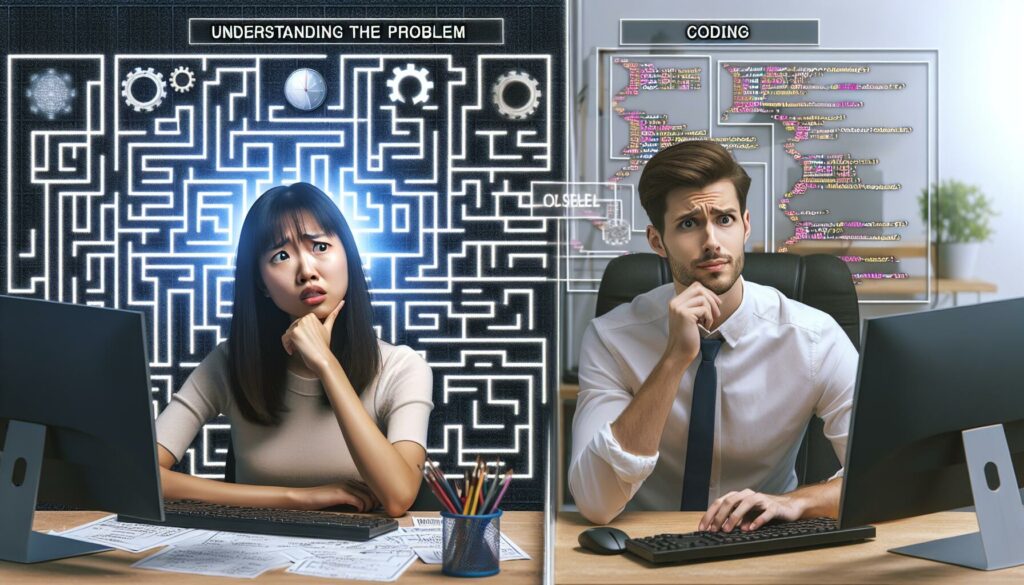
In the world of programming and software development, there’s a common misconception that the most challenging aspect is writing the code itself. However, seasoned developers and industry experts often argue that the real challenge lies in understanding the problem at hand. This article delves into why problem comprehension is the cornerstone of effective coding and how it impacts the entire development process.
The Importance of Problem Understanding in Coding
When aspiring programmers embark on their journey to master coding skills, they often focus heavily on syntax, algorithms, and coding techniques. While these are undoubtedly important, the ability to thoroughly understand and analyze a problem before writing a single line of code is arguably more crucial. Here’s why:
1. Clarity of Purpose
Understanding the problem provides clarity on what needs to be achieved. Without this clarity, developers may end up creating solutions that don’t address the core issues or miss critical requirements. This can lead to wasted time, resources, and potentially unusable code.
2. Efficient Problem-Solving
A clear understanding of the problem allows developers to approach the solution more efficiently. They can break down complex issues into smaller, manageable components and tackle each part systematically. This approach often results in more elegant and optimized solutions.
3. Reduced Debugging Time
When the problem is well-understood from the outset, developers are less likely to make fundamental errors in their code. This can significantly reduce the time spent on debugging and refactoring later in the development process.
4. Better Communication
In team environments, a thorough understanding of the problem facilitates better communication among team members. It ensures that everyone is on the same page regarding the project’s goals and requirements.
The Process of Understanding the Problem
So, how does one go about truly understanding a problem before diving into coding? Here are some key steps:
1. Identify the Core Issue
Start by identifying the central problem that needs to be solved. This involves asking questions like:
- What is the main goal of this project or task?
- What are the pain points that need to be addressed?
- Who are the end-users, and what are their specific needs?
2. Gather and Analyze Requirements
Once the core issue is identified, gather all relevant requirements. This may involve:
- Interviewing stakeholders or clients
- Reviewing existing documentation
- Analyzing user feedback or market research
After gathering this information, analyze it to ensure you have a comprehensive understanding of what needs to be achieved.
3. Break Down the Problem
Complex problems are often easier to tackle when broken down into smaller, more manageable components. This process, known as decomposition, helps in:
- Identifying dependencies between different parts of the problem
- Prioritizing which components to address first
- Creating a roadmap for the development process
4. Research Existing Solutions
Before reinventing the wheel, research existing solutions or approaches to similar problems. This can provide valuable insights and potentially save time in the development process. Consider:
- Open-source libraries or frameworks that address similar issues
- Design patterns that have proven effective in similar scenarios
- Case studies of how other companies or developers have tackled comparable problems
5. Visualize the Solution
Before writing any code, try to visualize the solution. This can involve:
- Creating flowcharts or diagrams
- Sketching user interfaces
- Drafting a high-level architecture of the system
Visualization helps in identifying potential issues early and provides a roadmap for the coding process.
Common Pitfalls in Problem Understanding
Even with the best intentions, developers can fall into several traps when trying to understand a problem. Being aware of these pitfalls can help avoid them:
1. Jumping to Solutions Too Quickly
It’s tempting to start coding as soon as you have a general idea of what needs to be done. However, this can lead to overlooking important details or making incorrect assumptions about the problem.
2. Misinterpreting Requirements
Sometimes, what seems clear at first glance can have hidden complexities or nuances. It’s crucial to verify your understanding of the requirements with stakeholders or team members.
3. Ignoring Edge Cases
When analyzing a problem, it’s easy to focus on the typical use cases and overlook edge cases or exceptional scenarios. These edge cases often reveal important aspects of the problem that need to be addressed.
4. Overcomplicating the Problem
Sometimes, in an attempt to be thorough, developers can overcomplicate the problem, leading to unnecessarily complex solutions. Strive for simplicity while ensuring all requirements are met.
5. Failing to Consider Scalability
While solving the immediate problem is important, failing to consider how the solution will scale in the future can lead to significant issues down the line. Always think about potential growth and changes in requirements.
Tools and Techniques for Better Problem Understanding
To enhance your ability to understand problems effectively, consider utilizing the following tools and techniques:
1. Mind Mapping
Mind mapping is a powerful technique for visually organizing information and exploring relationships between different aspects of a problem. It can help in:
- Identifying connections between various components of the problem
- Brainstorming potential solutions
- Organizing thoughts and ideas in a structured manner
2. User Story Mapping
User story mapping is particularly useful for understanding problems from the user’s perspective. It involves:
- Creating a visual representation of the user’s journey
- Identifying key activities and tasks
- Prioritizing features based on user needs
3. Prototyping
Creating prototypes, even simple ones, can greatly aid in understanding the problem and potential solutions. Prototypes can:
- Help visualize the end product
- Facilitate early feedback from stakeholders
- Reveal unforeseen challenges or requirements
4. The Five Whys Technique
This technique involves asking “why” multiple times to dig deeper into the root cause of a problem. It helps in:
- Uncovering underlying issues that may not be immediately apparent
- Avoiding superficial solutions that don’t address the core problem
- Gaining a more comprehensive understanding of the problem context
5. SWOT Analysis
While typically used in business strategy, a SWOT (Strengths, Weaknesses, Opportunities, Threats) analysis can be adapted for problem understanding in coding. It can help in:
- Identifying potential challenges and opportunities in the problem space
- Evaluating different approaches to solving the problem
- Considering external factors that might impact the solution
The Role of Problem Understanding in Different Programming Paradigms
The importance of understanding the problem extends across various programming paradigms, each with its own nuances:
Object-Oriented Programming (OOP)
In OOP, a thorough understanding of the problem domain is crucial for:
- Identifying appropriate classes and objects
- Defining relationships between objects
- Designing interfaces that accurately represent real-world entities
For example, when designing a library management system, understanding the problem helps in identifying entities like Book, Member, and Loan, and how they interact with each other.
Functional Programming
In functional programming, problem understanding is key to:
- Breaking down the problem into pure functions
- Identifying data transformations
- Ensuring immutability and avoiding side effects
For instance, when implementing a data processing pipeline, a clear understanding of the problem helps in defining the sequence of transformations the data needs to undergo.
Procedural Programming
In procedural programming, problem understanding aids in:
- Defining the sequence of steps needed to solve the problem
- Identifying appropriate data structures
- Organizing code into logical procedures or functions
For example, when writing a program to calculate compound interest, understanding the problem helps in determining the necessary steps and formulas to use.
Case Studies: The Impact of Problem Understanding
Let’s look at a couple of real-world examples that highlight the importance of problem understanding:
Case Study 1: The Mars Climate Orbiter
In 1999, NASA’s Mars Climate Orbiter mission failed due to a simple unit conversion error. The root cause was a misunderstanding between two teams:
- One team used the metric system (newtons) for thrust calculations
- Another team assumed imperial units (pound-force) were being used
This miscommunication led to the spacecraft approaching Mars at the wrong angle, causing it to disintegrate in the Martian atmosphere. This $327 million failure could have been avoided with a better understanding of the problem and clearer communication between teams.
Case Study 2: Therac-25 Radiation Therapy Machine
The Therac-25 was a radiation therapy machine that caused several accidents in the 1980s, resulting in severe injuries and deaths. The root cause was a software error that occurred due to a lack of understanding of the problem’s complexity:
- The software allowed for race conditions in the user interface
- There was inadequate error checking and safety interlocks
- The developers underestimated the importance of thorough testing in a life-critical system
A deeper understanding of the problem, including potential edge cases and the critical nature of the system, could have prevented these tragic incidents.
Developing Problem Understanding Skills
Improving your ability to understand problems is a skill that can be developed over time. Here are some strategies to enhance this crucial ability:
1. Practice Active Listening
When discussing problems with stakeholders or team members:
- Pay close attention to what’s being said
- Ask clarifying questions
- Summarize your understanding to ensure accuracy
2. Engage in Diverse Projects
Exposure to a variety of projects and problem domains can broaden your perspective and improve your problem-solving skills. Consider:
- Contributing to open-source projects
- Taking on side projects in different domains
- Participating in hackathons or coding challenges
3. Study System Design
Understanding how complex systems are designed can provide valuable insights into problem decomposition and analysis. Study:
- Architecture of popular software systems
- Design patterns and their applications
- Case studies of successful (and failed) software projects
4. Develop Critical Thinking Skills
Enhancing your critical thinking abilities can significantly improve your problem understanding. Practice:
- Questioning assumptions
- Analyzing information from multiple perspectives
- Evaluating the credibility of information sources
5. Seek Feedback
Regularly seek feedback on your problem analysis and solutions. This can help identify blind spots and areas for improvement.
The Future of Problem Understanding in Coding
As technology continues to evolve, the importance of problem understanding in coding is likely to increase. Some trends to watch include:
1. Artificial Intelligence and Machine Learning
As AI and ML become more prevalent, understanding complex problem domains will be crucial for developing effective algorithms and models.
2. Ethical Considerations in Technology
With growing concerns about the ethical implications of technology, developers will need to have a deeper understanding of the societal impact of their solutions.
3. Interdisciplinary Problem Solving
As technology intersects with various fields like healthcare, finance, and environmental science, developers will need to understand problems from multiple disciplinary perspectives.
Conclusion
While coding skills are undoubtedly important in software development, the ability to thoroughly understand and analyze problems is the true foundation of effective programming. By focusing on developing strong problem understanding skills, developers can create more robust, efficient, and relevant solutions.
Remember, the next time you’re faced with a coding challenge, resist the urge to dive straight into writing code. Take the time to fully understand the problem, break it down, and consider all aspects before you start implementing a solution. This approach will not only make you a better programmer but will also lead to more successful and impactful software projects.
As you continue your journey in coding and software development, always keep in mind that the real problem is understanding the problem, not coding it. With this perspective, you’ll be well-equipped to tackle even the most complex challenges in the ever-evolving world of technology.