Why Taking Notes During Tutorials Isn’t Helping You Learn To Code
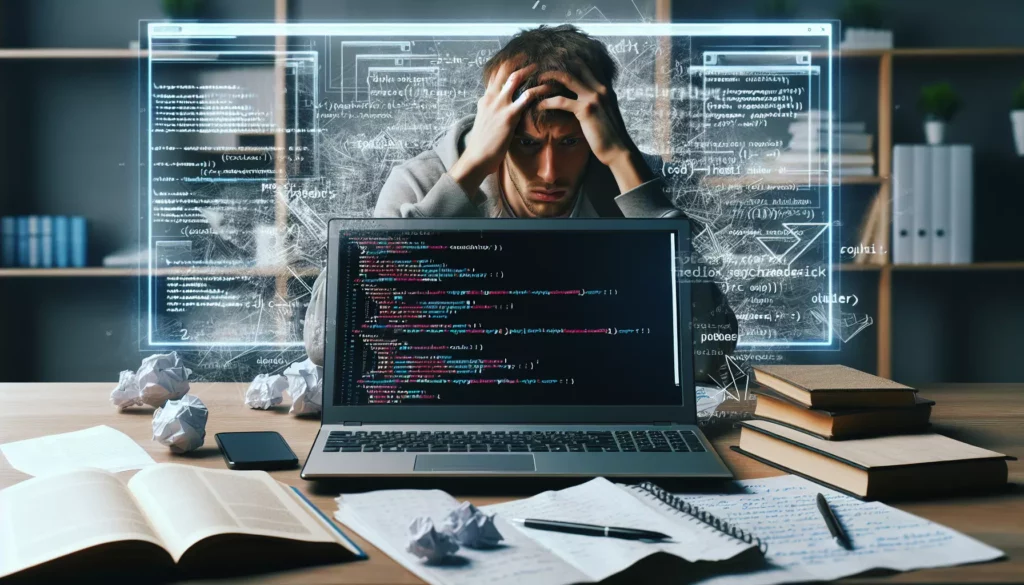
If you’re learning to code, you’ve probably experienced this scenario: you’re watching a tutorial, diligently taking notes, highlighting key concepts, and feeling productive. You finish the tutorial with pages of carefully crafted notes, confident that you’ve absorbed the material.
Then comes the moment of truth. You sit down to code on your own, and suddenly… your mind goes blank. Those concepts that seemed so clear during the tutorial now feel foreign. Your detailed notes don’t seem to help when faced with a blinking cursor and an empty file.
You’re not alone. This disconnect between passive note-taking and active learning is a common challenge for coding students. In this article, we’ll explore why traditional note-taking during programming tutorials often fails to translate into actual coding skills, and what you can do instead to truly master programming concepts.
The False Productivity of Passive Note-Taking
Note-taking gives us a comforting sense of productivity. Writing things down feels like progress, and seeing pages fill up with notes provides tangible evidence that we’re learning. But when it comes to coding, this approach has significant limitations.
The Illusion of Understanding
When we watch someone else code in a tutorial, everything seems logical and straightforward. The instructor navigates problems with ease, making the process look simple. As we take notes on their explanations and solutions, we experience what psychologists call the “illusion of competence” – we mistake recognizing a solution for being able to produce that solution ourselves.
This false sense of understanding is reinforced when we write down concepts in our notes. We think, “I understand this well enough to write it down, so I must know it.” But recognizing information is vastly different from being able to recall and apply it in a new context.
Passive vs. Active Learning
Note-taking during tutorials is predominantly passive learning. You’re absorbing information without actively engaging with it. Programming, however, is fundamentally about doing – it’s a skill that requires active practice and application.
Research consistently shows that passive learning techniques like reading and note-taking are among the least effective methods for retaining information and developing skills. According to a study published in the Proceedings of the National Academy of Sciences, students who actively engage with material learn more than those who passively consume it.
Why Traditional Note-Taking Fails for Programming
Beyond the general limitations of passive learning, there are specific reasons why traditional note-taking is particularly ineffective for learning to code.
Programming Is Procedural Knowledge, Not Declarative Knowledge
Educational psychologists distinguish between two types of knowledge:
- Declarative knowledge: Facts and concepts you can state or declare (e.g., “Variables store data,” “Arrays are indexed from zero in JavaScript”)
- Procedural knowledge: Knowing how to perform actions or skills (e.g., debugging a function, structuring a program)
Traditional note-taking is designed for declarative knowledge – recording facts, definitions, and concepts. But programming is primarily procedural knowledge – it’s about knowing how to perform tasks and solve problems through code.
When you take notes during a coding tutorial, you’re often recording declarative knowledge (definitions, syntax rules) while missing the procedural knowledge (the thought process, problem-solving approaches) that’s crucial for actual coding.
The Context Problem
Programming concepts are highly contextual. A snippet of code that makes perfect sense in one context might be completely inappropriate in another. Traditional notes often fail to capture this context, leaving you with isolated facts or code snippets without the framework for understanding when and how to apply them.
For example, your notes might include:
// Use reduce to sum an array
const sum = arr.reduce((acc, curr) => acc + curr, 0);
But this note doesn’t help you understand when to use reduce versus map or filter, or how to adapt this pattern for more complex operations.
Missing the Mental Models
Effective programming requires developing mental models – internal representations of how programming concepts work and relate to each other. Note-taking often focuses on recording the “what” (syntax, methods, properties) while missing the “why” and “how” that form these crucial mental models.
For instance, understanding how JavaScript’s event loop works is more valuable than memorizing the syntax for setting up an event listener. Yet notes tend to focus on the latter because it’s easier to record.
The Execution Gap
Perhaps the biggest problem with note-taking for programming is what we might call the “execution gap” – the difference between understanding code and being able to write code.
Reading and writing notes about code doesn’t prepare your brain for the actual task of programming, which involves:
- Breaking down problems into smaller steps
- Translating ideas into code syntax
- Identifying and fixing errors
- Testing and refining solutions
These skills can only be developed through active practice, not passive note-taking.
The Cognitive Science Behind Effective Learning
To understand why traditional note-taking falls short for programming education, it helps to look at what cognitive science tells us about effective learning.
The Spacing Effect
Research on the spacing effect shows that learning is more effective when spread out over time rather than crammed into a single session. Traditional note-taking during tutorials often happens in one sitting, without the spaced repetition that helps cement concepts in long-term memory.
The Testing Effect
Studies on the testing effect demonstrate that actively retrieving information from memory (like when solving a coding problem) leads to stronger learning than simply reviewing notes. When you take notes during a tutorial but don’t practice recalling and applying that information, you miss out on this powerful learning mechanism.
Transfer of Learning
Transfer of learning refers to the ability to apply knowledge learned in one context to a new situation. This is particularly important in programming, where you need to adapt concepts to solve novel problems.
Traditional note-taking doesn’t facilitate transfer because it often focuses on specific examples rather than underlying principles. You might diligently note how to sort an array in a particular tutorial example, but struggle to apply that knowledge when faced with a different sorting scenario.
What Experienced Programmers Do Instead
If you observe experienced programmers learning new technologies or concepts, you’ll notice they rarely rely on extensive note-taking. Instead, they use more effective approaches that engage with the material actively.
They Build While They Learn
Experienced programmers don’t just watch tutorials – they code alongside them. They pause videos, try implementing concepts immediately, and experiment with variations. This hands-on approach builds procedural knowledge and reinforces concepts through practice.
They Focus on Understanding, Not Memorizing
Rather than trying to record every detail, experienced learners focus on grasping the underlying principles and mental models. They know that syntax can always be looked up, but the conceptual understanding is what enables problem-solving.
They Embrace Productive Struggle
Skilled learners understand that confusion and mistakes are part of the learning process. Instead of avoiding errors by following tutorials exactly, they intentionally challenge themselves by trying to implement features before they’re explained or by modifying examples to test their understanding.
They Teach and Explain
One of the most effective ways to solidify understanding is to explain concepts to others. Experienced programmers often participate in communities, answer questions, or write blog posts about what they’re learning, forcing them to articulate their understanding clearly.
Better Alternatives to Passive Note-Taking
If traditional note-taking isn’t effective for learning to code, what should you do instead? Here are practical alternatives that align with how our brains actually learn programming skills.
Code Along, Then Code Again
Instead of taking notes while watching tutorials, code alongside them. Then, after completing the tutorial, close it and try to recreate the project or feature from memory. This active recall strengthens your understanding far more than reviewing notes ever could.
For example, if you’re following a tutorial on building a React component:
- First, code along with the tutorial, making sure you understand each step
- Then, close the tutorial and build a similar component from scratch
- Finally, extend the component with additional features not covered in the tutorial
This approach forces you to actively recall and apply what you’ve learned, revealing gaps in your understanding that notes might have hidden.
Practice Deliberate Modification
After completing a tutorial example, deliberately modify it to test your understanding. Change variables, add features, or refactor the code to accomplish the same goal differently.
For instance, if a tutorial shows you how to build a to-do list app using React’s useState hook, try rebuilding it using Redux, or add filtering capabilities, or implement drag-and-drop reordering. These modifications require you to apply your knowledge in new ways, strengthening your understanding.
Create Concept Maps Instead of Linear Notes
If you do want to take notes, consider creating concept maps instead of linear notes. Concept maps visually represent the relationships between different programming concepts, helping you build the mental models necessary for effective problem-solving.
For example, instead of noting down the syntax for different array methods, create a concept map showing:
- Which methods transform arrays vs. which return new values
- Which methods are used for filtering vs. transforming vs. aggregating
- How different methods relate to common programming patterns
This approach focuses on connections and context rather than isolated facts.
Use the Feynman Technique
Named after physicist Richard Feynman, this technique involves explaining concepts in simple language as if teaching someone else. When you learn a new programming concept:
- Write down the concept name
- Explain it in simple terms as if teaching a beginner
- Identify gaps in your explanation where you struggle or resort to jargon
- Review the source material to address those gaps
- Simplify your explanation further
This process forces you to confront what you don’t fully understand, rather than hiding behind copied notes or technical terminology.
Build a Personal Project Portfolio
Instead of accumulating notes, build a portfolio of personal projects that apply what you’re learning. Each project serves as a concrete demonstration of your skills and a reference for future learning.
For example, if you’re learning about APIs and data fetching:
- Complete a tutorial on API integration
- Build a simple project that uses a public API
- Document the challenges you faced and how you overcame them
- Add the project to your portfolio for future reference
This approach creates a practical, usable record of your learning that’s far more valuable than pages of notes.
Use Spaced Repetition Systems
If there are specific facts or syntax rules you need to memorize, use spaced repetition software like Anki rather than static notes. These systems present information for review at optimal intervals based on how well you recall it, making memorization more efficient.
For example, you might create flashcards for:
- Common array methods and their use cases
- SQL query syntax patterns
- Regular expression patterns for common validation tasks
The key difference from notes is that spaced repetition systems actively test your recall, not just your recognition of information.
When Note-Taking Can Still Be Useful
While passive note-taking isn’t effective as a primary learning strategy for coding, there are specific situations where strategic note-taking can complement active learning approaches.
Documentation of Problem-Solving Processes
Taking notes about how you solved a particularly challenging problem can be valuable. Rather than recording the solution itself (which you can find in your code), document your thought process, the approaches you tried, and the insights you gained.
These process notes serve as a record of your problem-solving strategies that you can refer to when facing similar challenges in the future.
Creating Personalized Reference Guides
As you gain experience, you might create concise reference guides for concepts you use regularly but don’t need to memorize in detail. These aren’t learning notes but practical tools for your workflow.
For example, you might maintain a personal reference for:
- Your commonly used Git commands and workflows
- Configuration patterns for tools you use regularly
- Code snippets for tasks you perform frequently
The key is that these references support your coding practice rather than substituting for it.
Capturing Insights and Connections
Sometimes while learning, you’ll have “aha moments” where concepts suddenly connect in a meaningful way. These insights are worth recording, not as a substitute for practice but as markers of your developing understanding.
For instance, you might note down the moment you realized how JavaScript’s event delegation relates to the prototype chain, or how React’s component lifecycle connects to the virtual DOM concept.
Implementing a More Effective Learning Strategy
Now that we understand why traditional note-taking falls short for learning programming, let’s outline a more effective approach you can implement immediately.
Before the Tutorial
Set clear learning objectives before starting any tutorial:
- What specific skills or concepts do you want to gain?
- How will you apply these skills to your own projects?
- What questions do you have that you hope the tutorial will answer?
This preparation helps you engage actively with the material rather than passively consuming it.
During the Tutorial
Instead of taking detailed notes:
- Code alongside the tutorial, typing every line yourself (don’t copy-paste)
- Pause regularly to predict what comes next before the instructor shows it
- When you don’t understand something, experiment by changing the code to see what happens
- Take minimal notes focused on “why” rather than “how” (the code itself documents the “how”)
After the Tutorial
This is where the real learning happens:
- Close the tutorial and rebuild the key components from memory
- Modify the project to add new features or solve related problems
- Teach what you learned to someone else (or pretend to teach if no one is available)
- Create a small project that applies the concepts in a different context
This active application phase is what transforms information into usable skills.
Weekly Review and Integration
Set aside time each week to review and integrate what you’ve learned:
- Look back at projects you’ve built and identify patterns or concepts that appear frequently
- Connect new knowledge with what you already know by building projects that combine multiple concepts
- Identify gaps in your understanding and select resources to address those specific gaps
This regular integration helps build a coherent mental model rather than collecting isolated facts.
Common Objections and Concerns
You might have some reservations about abandoning your note-taking habit. Let’s address some common concerns:
“But I’ll Forget Everything Without Notes!”
It’s true that you’ll forget details, but active practice creates more durable memories than notes. More importantly, programming isn’t about memorizing details – it’s about understanding concepts and knowing how to find information when needed.
Professional developers constantly look up syntax and methods. What makes them effective isn’t perfect recall but strong mental models that help them know what to look for and how to apply it.
“I’m a Visual Learner – I Need to Write Things Down”
Different learning preferences are real, but the fundamental principles of skill acquisition apply to everyone. If you process information better visually, try:
- Creating diagrams of how concepts relate instead of linear notes
- Building visual projects that demonstrate the concepts you’re learning
- Recording screencasts of yourself coding and explaining the process
These approaches honor your visual preference while still engaging actively with the material.
“Taking Notes Helps Me Stay Focused”
If note-taking helps you maintain attention during tutorials, you don’t need to abandon it completely. Instead, shift to more active forms of engagement:
- Take minimal, strategic notes about concepts you find confusing
- Note questions that arise rather than solutions presented
- Use the “pause and predict” technique – pause the tutorial and write down what you think should come next
These approaches keep you engaged while still promoting active learning.
Case Study: Learning React Effectively
Let’s see how these principles might apply to learning a popular technology like React.
The Traditional Approach
With a traditional note-taking approach, you might:
- Watch a React tutorial series, taking detailed notes on components, props, state, and hooks
- Copy down example code and explanations from the instructor
- Complete the tutorial with pages of notes and a project that works but that you don’t fully understand
- Struggle to build your own React project because the concepts don’t transfer
The Active Learning Approach
With an active learning approach, you would instead:
- Watch a section of a React tutorial about a specific concept (e.g., useState hook)
- Code along, building the example component
- Pause the tutorial and modify the component to test your understanding (e.g., add another state variable, change the update logic)
- Try to explain the concept out loud as if teaching someone else
- Build a small, different component that uses the same concept
- Move on to the next concept only when you can comfortably apply the current one
The Results
The active approach might seem slower initially – you cover less tutorial content in the same amount of time. But the learning is much deeper. After completing even part of a tutorial this way, you’ll be able to build your own components and solve real problems, rather than just recognizing concepts when you see them.
Tools and Resources for Active Programming Learning
Several tools and platforms are designed to support active learning approaches for programming:
Interactive Coding Platforms
- CodePen and CodeSandbox: These online environments let you experiment with code immediately without setting up a development environment
- Replit: Offers a full development environment in the browser, making it easy to test ideas quickly
- AlgoCademy: Provides interactive, hands-on learning experiences for algorithms and data structures with immediate feedback
Active Learning Resources
- Exercism.io: Provides coding challenges with mentor feedback, focusing on practice rather than passive consumption
- LeetCode and HackerRank: Offer problem-solving challenges that force you to apply concepts actively
- Project-based courses: Look for tutorials that emphasize building projects rather than just explaining concepts
Spaced Repetition Tools
- Anki: A powerful flashcard system that uses spaced repetition algorithms
- RemNote: Combines note-taking with spaced repetition for more effective review
- Quizlet: Offers various study modes including flashcards and practice tests
Conclusion: Transform Your Learning Approach
The path to programming proficiency isn’t paved with meticulous notes but with consistent, deliberate practice. While traditional note-taking creates an illusion of learning, it’s the active application of concepts that builds real skills.
To truly learn programming:
- Replace passive note-taking with active coding practice
- Focus on understanding concepts rather than memorizing syntax
- Build projects that apply what you’re learning in new contexts
- Embrace the struggle of solving problems independently
- Use strategic documentation to support your practice, not replace it
This approach might feel less comfortable initially. Without the safety net of detailed notes, you’ll make more mistakes and face more confusion. But this productive struggle is precisely what leads to deeper understanding and more transferable skills.
The next time you sit down to watch a coding tutorial, put away your notebook. Instead, open your code editor and prepare to learn by doing. Your future self will thank you when you’re building applications confidently instead of flipping through pages of notes wondering where to start.
Remember: in programming, knowledge isn’t measured by what you can write down, but by what you can build.