Why Taking Notes During a Coding Interview Can Boost Your Performance
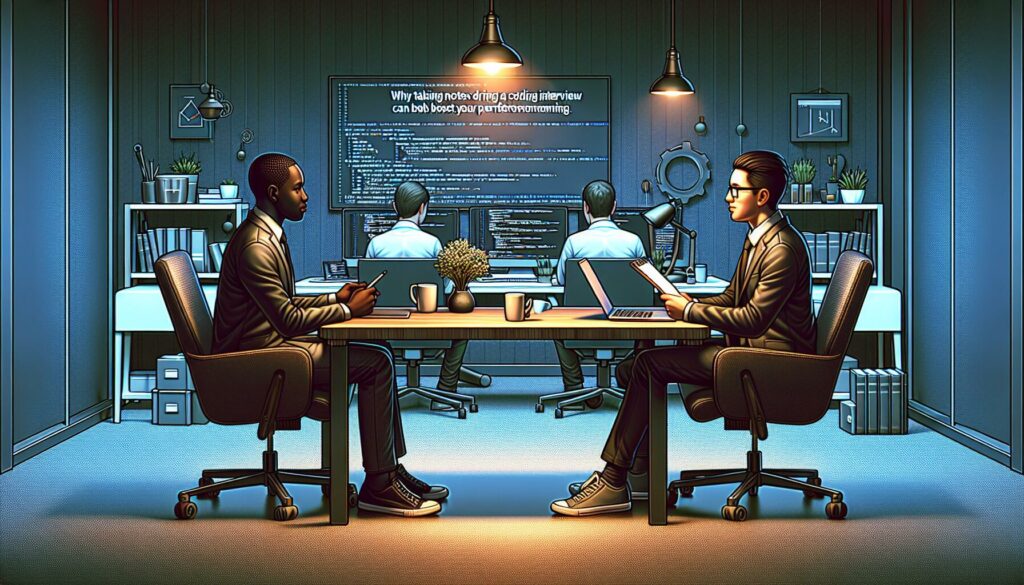
When it comes to coding interviews, particularly those for prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), preparation is key. While most candidates focus on practicing algorithms and data structures, there’s one often overlooked strategy that can significantly improve your performance: taking notes during the interview. In this comprehensive guide, we’ll explore why note-taking is crucial and how it can give you an edge in your next coding interview.
The Importance of Note-Taking in Coding Interviews
Coding interviews can be intense and mentally demanding. You’re expected to solve complex problems under time pressure while explaining your thought process. In such high-stress situations, it’s easy to miss important details or lose track of your ideas. This is where note-taking comes in as a powerful tool to enhance your performance.
Here are some key reasons why taking notes during a coding interview can be beneficial:
- Helps organize your thoughts
- Ensures you don’t miss crucial information
- Aids in problem decomposition
- Serves as a reference throughout the interview
- Demonstrates your attention to detail and professionalism
What to Write Down During a Coding Interview
Now that we understand the importance of note-taking, let’s dive into what specific information you should jot down during your coding interview.
1. Problem Description
The first and most crucial thing to note down is the problem description. As the interviewer explains the problem, write down key points, requirements, and any specific constraints mentioned. This serves multiple purposes:
- Ensures you don’t forget any important details
- Helps you clarify the problem by repeating it back to the interviewer
- Provides a reference point to check your solution against
Example note for a problem description:
Problem: Find the kth largest element in an unsorted array
- Array is unsorted
- K is a positive integer
- Need to return the element, not the index
- Assume 1-based indexing for k
2. Input and Output Examples
Write down any example inputs and outputs provided by the interviewer. If none are given, ask for some and note them down. These examples will help you:
- Understand the problem better
- Test your solution later
- Identify potential edge cases
Example note for input and output:
Input: [3, 2, 1, 5, 6, 4], k = 2
Output: 5
Input: [3, 2, 3, 1, 2, 4, 5, 5, 6], k = 4
Output: 4
3. Edge Cases and Constraints
Identifying and noting down edge cases is crucial for developing a robust solution. Some common edge cases to consider include:
- Empty or null inputs
- Single-element inputs
- Very large inputs
- Inputs with duplicate elements
- Boundary values for variables
Example note for edge cases and constraints:
Edge Cases:
- Empty array
- K > array length
- K = 1 (largest element)
- K = array length (smallest element)
- Array with duplicate elements
Constraints:
- 1 <= k <= array length
- Array elements are integers
- Array length <= 10^5
4. Potential Approaches
As you brainstorm solutions, jot down high-level approaches you’re considering. This helps you:
- Organize your thoughts
- Compare different strategies
- Explain your reasoning to the interviewer
Example note for potential approaches:
Approaches:
1. Sort array and return kth element from end - O(n log n)
2. Use min-heap of size k - O(n log k)
3. QuickSelect algorithm - O(n) average case, O(n^2) worst case
5. Time and Space Complexity
For each approach you consider, note down the time and space complexity. This demonstrates your ability to analyze algorithms and helps you choose the most efficient solution.
Example note for time and space complexity:
Chosen Approach: QuickSelect
Time Complexity:
- Average case: O(n)
- Worst case: O(n^2)
Space Complexity:
- O(1) - in-place partitioning
How to Use Your Notes Effectively
Taking notes is only half the battle. Using them effectively throughout the interview is equally important. Here are some tips on how to leverage your notes:
1. Refer to Your Problem Description
As you develop your solution, frequently check your notes on the problem description. This ensures you’re addressing all requirements and constraints. It also helps you avoid going off-track or solving the wrong problem.
2. Test Your Solution with Examples
Use the input-output examples you’ve noted down to test your solution. Walk through your code with these examples to catch any logical errors or edge cases you might have missed.
3. Address Edge Cases
Before finalizing your solution, review the edge cases you’ve noted. Ensure your code handles all of these scenarios correctly. This demonstrates thoroughness and attention to detail.
4. Explain Your Approach
Use your notes on potential approaches and complexity analysis to explain your reasoning to the interviewer. This shows your ability to consider multiple solutions and make informed decisions.
5. Optimize Your Solution
If time allows, refer to your notes on time and space complexity to identify areas for optimization. This demonstrates your ability to iterate and improve on your initial solution.
Best Practices for Note-Taking in Coding Interviews
To make the most of your note-taking during a coding interview, consider these best practices:
1. Use Clear and Concise Notation
Your notes should be easy to read and understand at a glance. Use bullet points, abbreviations, and simple diagrams where appropriate. Remember, you’ll need to quickly reference these notes while coding.
2. Organize Your Notes
Structure your notes in a logical manner. You might want to divide your page into sections for the problem description, examples, edge cases, and approaches. This makes it easier to find the information you need quickly.
3. Write Legibly
Even if you’re the only one who will read your notes, make sure they’re legible. You don’t want to waste time deciphering your own handwriting during the interview.
4. Don’t Overdo It
While note-taking is valuable, don’t let it distract you from the main task of solving the problem. Find a balance between taking useful notes and actively working on the solution.
5. Practice Note-Taking
Like any skill, effective note-taking during coding interviews improves with practice. Incorporate note-taking into your interview preparation routine to make it feel natural during the actual interview.
How Note-Taking Demonstrates Your Skills
Taking notes during a coding interview doesn’t just help you solve the problem at hand; it also demonstrates several valuable skills to your interviewer:
1. Attention to Detail
By meticulously noting down problem details, constraints, and edge cases, you show that you’re thorough and pay attention to the finer points of a problem.
2. Organization and Methodology
A structured approach to note-taking reflects your ability to organize information and tackle problems methodically – a crucial skill for any software developer.
3. Communication Skills
Using your notes to clarify the problem and explain your approach demonstrates strong communication skills, which are essential in collaborative development environments.
4. Critical Thinking
The act of taking notes, especially when jotting down potential approaches and their trade-offs, showcases your critical thinking and analytical skills.
5. Adaptability
If you need to pivot your approach during the interview, referring to your notes to quickly reorient yourself demonstrates adaptability and resilience under pressure.
Common Pitfalls to Avoid
While note-taking can significantly enhance your coding interview performance, there are some potential pitfalls to be aware of:
1. Over-Reliance on Notes
While notes are helpful, don’t become overly dependent on them. You should still be able to engage in a fluid conversation with the interviewer and code efficiently.
2. Spending Too Much Time Writing
Be mindful of the time you spend taking notes. If you find yourself writing extensively, you might be using it as a procrastination tactic to avoid tackling the problem.
3. Ignoring the Interviewer
Don’t let note-taking prevent you from maintaining good eye contact and engagement with the interviewer. Remember, the interview is also about assessing your interpersonal skills.
4. Neglecting to Use Your Notes
There’s little point in taking notes if you don’t refer back to them. Make sure you actively use your notes throughout the interview process.
5. Unclear or Disorganized Notes
If your notes are messy or poorly organized, they may hinder rather than help your performance. Strive for clarity and structure in your note-taking.
Adapting Note-Taking for Different Interview Formats
Note-taking strategies may need to be adapted based on the interview format. Here’s how you can adjust your approach:
In-Person Interviews
For traditional in-person interviews:
- Bring a notepad and pen
- Position your notes where both you and the interviewer can see them if needed
- Use your notes to guide your verbal explanations
Virtual Interviews
For online or virtual interviews:
- Use a physical notepad off-screen or a digital note-taking tool
- If using a digital tool, make sure it doesn’t interfere with the video call or coding environment
- Be prepared to share your screen, including your notes if requested
Whiteboard Interviews
For whiteboard coding interviews:
- Utilize a section of the whiteboard for your notes
- Organize your whiteboard space effectively, allocating areas for notes, code, and diagrams
- Use your notes as talking points when explaining your approach to the interviewer
Conclusion
Taking notes during a coding interview is a simple yet powerful technique that can significantly boost your performance. It helps you stay organized, ensures you address all aspects of the problem, and demonstrates your professionalism and attention to detail.
By noting down the problem description, examples, edge cases, potential approaches, and complexity analysis, you create a valuable reference that guides you through the problem-solving process. Moreover, the act of taking notes itself can help clarify your thoughts and improve your understanding of the problem.
Remember, the goal of note-taking is to support your problem-solving process, not to replace it. Use your notes as a tool to enhance your performance, but don’t let them distract you from the main task of coding and explaining your solution.
As with any skill, effective note-taking in coding interviews improves with practice. Incorporate this technique into your interview preparation routine, and you’ll likely find it becomes a natural and beneficial part of your problem-solving approach.
By mastering the art of note-taking in coding interviews, you’ll not only improve your chances of success but also demonstrate the kind of organized, methodical, and detail-oriented approach that top tech companies value in their engineers. So grab that pen and paper, and start boosting your coding interview performance today!