Why Speed Isn’t Everything: Focusing on Code Quality Over Quick Solutions in Interviews
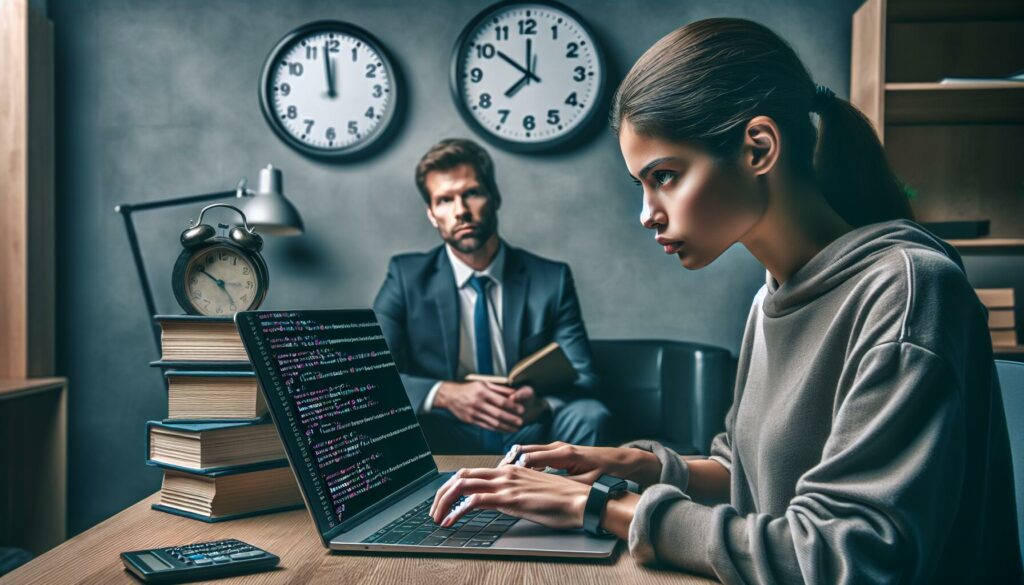
In the high-pressure environment of coding interviews, especially those for prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), there’s often a temptation to prioritize speed over everything else. The ticking clock, the watchful eyes of the interviewer, and the desire to impress can push candidates to rush through problems, aiming for the quickest possible solution. However, this approach can be counterproductive and may not accurately reflect the skills that companies are truly looking for. In this comprehensive guide, we’ll explore why focusing on code quality rather than speed is crucial in coding interviews and how this approach can actually improve your chances of success.
The Myth of Speed in Coding Interviews
There’s a common misconception among coding interview candidates that the faster you solve a problem, the more impressed the interviewer will be. This belief often stems from:
- The time constraints of interview sessions
- The desire to showcase efficiency
- The assumption that quick problem-solving equates to programming prowess
While it’s true that completing problems within the allotted time is important, it’s equally crucial to understand that interviewers are looking for much more than just speed. They want to assess your thought process, problem-solving skills, and ability to write clean, maintainable code.
The Real Goals of Coding Interviews
To understand why code quality trumps speed, let’s first examine what interviewers are actually trying to evaluate:
1. Problem-Solving Ability
Interviewers want to see how you approach and break down complex problems. This includes your ability to:
- Analyze the problem requirements
- Consider edge cases
- Develop a logical solution strategy
2. Code Organization and Structure
The way you structure your code reveals a lot about your programming skills. Interviewers look for:
- Clear and logical code organization
- Appropriate use of functions and modules
- Adherence to coding best practices
3. Attention to Detail
Your code should demonstrate careful consideration of:
- Edge cases and error handling
- Input validation
- Efficient use of data structures and algorithms
4. Communication Skills
How you explain your thought process and discuss your code is crucial. Interviewers assess:
- Your ability to articulate your ideas clearly
- How well you respond to questions and feedback
- Your capacity to collaborate and discuss alternative approaches
5. Code Readability and Maintainability
Writing code that others can easily understand and maintain is a key skill. This includes:
- Clear variable and function naming
- Proper indentation and formatting
- Useful comments where necessary
The Pitfalls of Prioritizing Speed
When candidates focus solely on speed, several problems can arise:
1. Overlooking Important Details
Rushing through a problem often leads to overlooking critical details, such as edge cases or potential errors. This can result in incomplete or incorrect solutions.
2. Sacrificing Code Quality
Quick solutions often come at the cost of code quality. This can manifest as:
- Poorly structured code
- Lack of proper error handling
- Inefficient algorithms or data structures
3. Difficulty in Explaining the Solution
When you rush, you might not fully understand your own solution. This can make it challenging to explain your approach or answer follow-up questions from the interviewer.
4. Increased Stress and Errors
The pressure to solve problems quickly can increase stress levels, leading to more mistakes and a higher likelihood of getting stuck.
5. Missed Opportunities for Optimization
By focusing on speed, you might miss opportunities to optimize your solution or consider more efficient approaches.
Strategies for Focusing on Code Quality in Interviews
Now that we understand the importance of code quality, let’s explore strategies to prioritize it during coding interviews:
1. Start with a Clear Plan
Before diving into coding, take a few minutes to:
- Analyze the problem thoroughly
- Outline your approach
- Consider potential edge cases
This initial investment of time will pay off in the quality of your solution.
2. Communicate Your Thought Process
As you work through the problem:
- Explain your reasoning out loud
- Discuss trade-offs between different approaches
- Ask clarifying questions when needed
This demonstrates your problem-solving skills and allows the interviewer to guide you if necessary.
3. Write Clean, Readable Code
Focus on writing code that is easy to understand:
- Use meaningful variable and function names
- Maintain consistent indentation and formatting
- Break down complex operations into smaller, manageable functions
4. Handle Edge Cases and Errors
Demonstrate your attention to detail by:
- Considering and handling edge cases
- Implementing proper error checking and handling
- Discussing potential limitations of your solution
5. Optimize Gradually
Start with a working solution, then optimize:
- Begin with a straightforward implementation
- Analyze and improve time and space complexity
- Discuss potential optimizations, even if you don’t implement them all
6. Test Your Code
Show your thoroughness by testing your solution:
- Write test cases covering various scenarios
- Walk through your code with sample inputs
- Identify and fix any bugs you find
7. Be Open to Feedback
Demonstrate your ability to collaborate:
- Listen carefully to the interviewer’s suggestions
- Be willing to revise your approach based on feedback
- Show enthusiasm for learning and improving
Example: Quality-Focused Approach vs. Speed-Focused Approach
Let’s consider a common interview problem: implementing a function to reverse a linked list. We’ll compare a speed-focused approach with a quality-focused approach.
Speed-Focused Approach:
def reverse_list(head):
prev = None
current = head
while current:
next = current.next
current.next = prev
prev = current
current = next
return prev
While this solution is correct and concise, it lacks several elements that demonstrate code quality and thorough problem-solving.
Quality-Focused Approach:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_linked_list(head):
"""
Reverses a singly linked list.
Args:
head (ListNode): The head of the linked list to be reversed.
Returns:
ListNode: The new head of the reversed linked list.
Time complexity: O(n), where n is the number of nodes in the list.
Space complexity: O(1), as we only use a constant amount of extra space.
"""
if not head or not head.next:
return head # Handle edge cases: empty list or single node
prev = None
current = head
while current:
# Store the next node
next_node = current.next
# Reverse the link
current.next = prev
# Move pointers one step forward
prev = current
current = next_node
return prev # New head of the reversed list
def print_list(head):
"""Helper function to print the linked list."""
current = head
while current:
print(current.val, end=" -> ")
current = current.next
print("None")
# Test the function
if __name__ == "__main__":
# Create a sample linked list: 1 -> 2 -> 3 -> 4 -> 5
head = ListNode(1)
head.next = ListNode(2)
head.next.next = ListNode(3)
head.next.next.next = ListNode(4)
head.next.next.next.next = ListNode(5)
print("Original list:")
print_list(head)
# Reverse the list
new_head = reverse_linked_list(head)
print("Reversed list:")
print_list(new_head)
This quality-focused approach demonstrates several key aspects of good coding practices:
- Clear Documentation: The function includes a docstring explaining its purpose, parameters, return value, and complexity analysis.
- Edge Case Handling: The code explicitly handles edge cases (empty list and single-node list).
- Readable Variable Names: Variables like
next_node
are more descriptive than simplynext
. - Code Structure: The solution includes a separate
ListNode
class definition, making the code more complete and self-contained. - Testing: A main block is included to demonstrate how the function works with a sample input.
- Helper Function: The
print_list
function aids in visualizing the results, showing attention to detail and user-friendliness.
While this approach may take slightly longer to write in an interview setting, it demonstrates a much higher level of coding proficiency and attention to detail.
The Long-Term Benefits of Prioritizing Code Quality
Focusing on code quality in interviews doesn’t just help you perform better during the assessment; it also prepares you for real-world software development challenges:
1. Improved Problem-Solving Skills
By thoroughly analyzing problems and considering various aspects before coding, you develop stronger problem-solving skills that are valuable in any programming role.
2. Better Collaboration Abilities
Writing clean, well-documented code and being able to explain your thought process clearly are crucial skills for working in a team environment.
3. Reduced Technical Debt
The habits of writing quality code from the start help reduce technical debt in real-world projects, making systems easier to maintain and scale.
4. Enhanced Debugging Skills
Paying attention to detail and considering edge cases during the coding process improves your ability to identify and fix bugs efficiently.
5. Increased Confidence
Knowing that you can produce high-quality code under pressure boosts your confidence as a developer, both in interviews and on the job.
Conclusion
While speed is certainly a factor in coding interviews, it should not come at the expense of code quality. By focusing on writing clean, well-structured, and thoroughly considered code, you demonstrate not just your ability to solve problems, but your capacity to create robust, maintainable solutions. This approach aligns more closely with the skills that top tech companies value in their engineers.
Remember, the goal of a coding interview is not just to solve the problem, but to showcase your overall programming abilities. By prioritizing code quality, you present yourself as a thoughtful, detail-oriented, and skilled developer – qualities that are highly valued in the software engineering field.
As you prepare for coding interviews, practice balancing efficiency with quality. Use platforms like AlgoCademy to hone your skills, focusing not just on getting the right answer, but on developing and articulating high-quality solutions. This approach will serve you well not only in interviews but throughout your career as a software developer.