Why Solving Problems on Paper First Leads to Faster Success in Coding Interviews
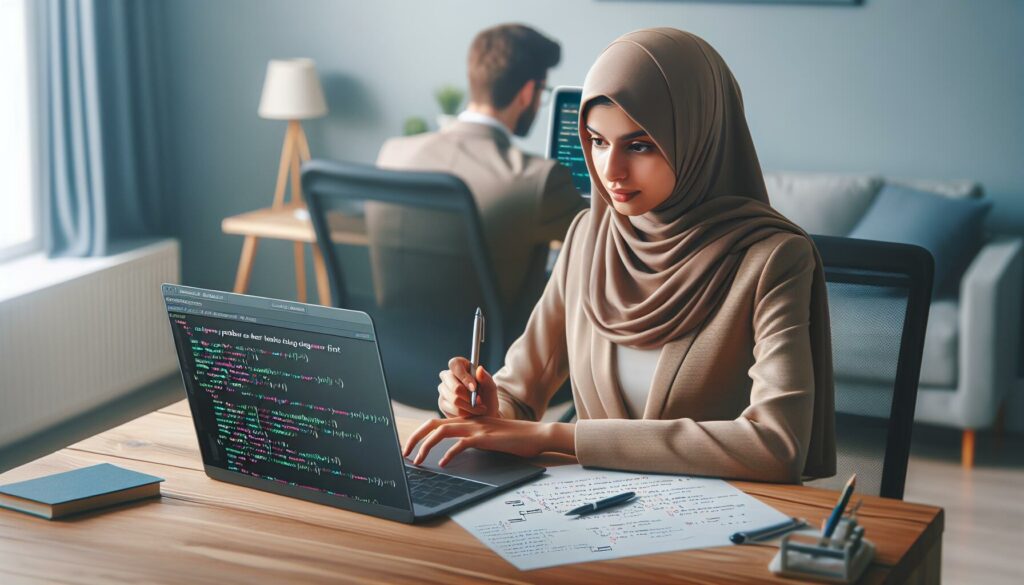
In the fast-paced world of coding interviews, it’s tempting to dive straight into writing code on a computer. However, seasoned programmers and successful interviewees often swear by a seemingly old-fashioned approach: solving problems on paper first. This method, which includes using pen and paper or whiteboarding, can significantly enhance your problem-solving skills and lead to faster success in coding interviews. In this comprehensive guide, we’ll explore why this approach is so effective and how you can leverage it to ace your next technical interview.
The Power of Paper: Clarifying Logic and Preventing Errors
Before we delve into the specific techniques, let’s understand why solving problems on paper is so powerful:
1. Slowing Down to Speed Up
When you start with pen and paper, you’re forced to slow down and think through each step of your solution. This deliberate pace allows you to:
- Fully understand the problem before attempting to solve it
- Consider multiple approaches and choose the most efficient one
- Identify potential edge cases and pitfalls early in the process
By taking the time to think critically at the outset, you’ll often arrive at a better solution faster than if you had jumped straight into coding.
2. Visualizing Complex Problems
Many coding problems, especially those involving data structures like trees, graphs, or complex algorithms, are easier to understand when visualized. Drawing out these structures on paper can help you:
- See patterns and relationships that might not be obvious in code
- Break down complex problems into smaller, manageable parts
- Communicate your thought process clearly to interviewers
3. Reducing Cognitive Load
When you’re coding on a computer, you’re juggling multiple tasks: syntax, logic, variable names, and more. By starting on paper, you can focus solely on the problem-solving aspect, reducing the cognitive load and allowing for clearer thinking.
4. Preventing Syntax Errors
Writing pseudocode or high-level logic on paper eliminates the distraction of syntax errors. This allows you to focus on the core algorithm without getting bogged down by language-specific details.
5. Enhancing Communication
In many interview settings, you’ll be asked to explain your thought process. Having a visual representation of your solution makes it easier to walk the interviewer through your approach, demonstrating your problem-solving skills even before you write a single line of code.
Effective Techniques for Paper-Based Problem Solving
Now that we understand the benefits, let’s explore some techniques to maximize the effectiveness of solving problems on paper:
1. Diagramming
Diagrams are powerful tools for visualizing complex problems and data structures. Here are some common types of diagrams you might use:
- Flowcharts: Ideal for representing the flow of control in an algorithm
- Tree diagrams: Perfect for problems involving hierarchical data structures
- Network diagrams: Useful for graph-based problems or network algorithms
- State diagrams: Helpful for problems involving state machines or complex object lifecycles
When creating diagrams:
- Start with a rough sketch and refine as you understand the problem better
- Use clear labels and annotations to explain each part of the diagram
- Practice creating common data structures (e.g., linked lists, binary trees) so you can quickly draw them during interviews
2. Pseudocode
Pseudocode is a informal, high-level description of an algorithm or program logic. It’s an excellent bridge between your initial thoughts and the final code. When writing pseudocode:
- Use clear, concise statements to describe each step of your algorithm
- Focus on the logic flow rather than exact syntax
- Include comments or explanations for complex parts of your solution
- Use indentation to show nested structures or loops
Here’s an example of pseudocode for a binary search algorithm:
function binarySearch(arr, target):
left = 0
right = length of arr - 1
while left <= right:
mid = (left + right) / 2
if arr[mid] == target:
return mid // Found the target
else if arr[mid] < target:
left = mid + 1 // Target is in the right half
else:
right = mid - 1 // Target is in the left half
return -1 // Target not found
3. Logical Flows
Breaking down your solution into logical steps can help you tackle complex problems. Try these techniques:
- Step-by-step breakdowns: List each major step of your algorithm in order
- Input-process-output (IPO) model: Clearly define what goes in, what transformations occur, and what comes out
- Decision trees: Map out different paths your algorithm might take based on various conditions
For example, a logical flow for a problem to find the longest palindromic substring might look like this:
- Define a function to check if a substring is a palindrome
- Iterate through all possible substrings of the input string
- For each substring:
- Check if it’s a palindrome using the function from step 1
- If it is, compare its length to the current longest palindrome
- Update the longest palindrome if necessary
- Return the longest palindromic substring found
Tips for Effective Paper-First Problem Solving
To make the most of this approach, consider the following tips:
1. Practice Regularly
Like any skill, solving problems on paper improves with practice. Set aside time to work through coding problems using only pen and paper. This will help you become more comfortable with the process and quicker at translating your thoughts to diagrams and pseudocode.
2. Time Yourself
In real interview situations, you’ll be working under time constraints. Practice solving problems on paper within specific time limits to improve your speed and efficiency.
3. Verbalize Your Thought Process
As you work through problems on paper, practice explaining your thought process out loud. This will help you prepare for the communication aspect of coding interviews.
4. Start Simple, Then Refine
Begin with a basic solution, even if it’s not the most efficient. You can then iterate and optimize your approach. This demonstrates your problem-solving process to interviewers.
5. Learn Common Patterns
Familiarize yourself with common algorithm patterns and data structures. Being able to quickly recognize and diagram these patterns will save you time during interviews.
6. Use Templates
Develop templates for common problem-solving approaches, such as two-pointer techniques, sliding windows, or dynamic programming. Having these mental templates will help you approach problems more systematically.
Transitioning from Paper to Code
Once you’ve solved the problem on paper, transitioning to code becomes much easier. Here’s how to make this transition smooth:
1. Review Your Solution
Before you start coding, review your paper solution. Make sure you’ve considered all edge cases and that your logic is sound.
2. Choose the Right Data Structures
Based on your paper solution, decide which data structures will be most efficient for your implementation. Your diagrams and pseudocode should guide this decision.
3. Implement in Chunks
Instead of trying to code the entire solution at once, implement it in chunks based on your logical flow. This makes the coding process more manageable and allows for easier debugging.
4. Comment as You Go
Use your pseudocode as a basis for comments in your actual code. This will make your code more readable and demonstrate your thought process to interviewers.
5. Test Early and Often
As you implement each part of your solution, test it with sample inputs. This incremental testing can help catch errors early and validate your approach.
Common Pitfalls to Avoid
While solving problems on paper is generally beneficial, be aware of these potential pitfalls:
1. Over-Engineering
It’s possible to spend too much time on paper, over-complicating your solution. Remember, the goal is to clarify your thinking, not to create a perfect blueprint.
2. Ignoring Time Complexity
When working on paper, it’s easy to overlook the time complexity of your solution. Always consider the efficiency of your algorithm, even in the planning stage.
3. Neglecting Edge Cases
While paper solutions are great for overall logic, don’t forget to consider and note down potential edge cases that you’ll need to handle in your code.
4. Skipping the Testing Phase
Even on paper, it’s important to “dry run” your solution with sample inputs. This can help you catch logical errors before you start coding.
Real-World Application: A Case Study
Let’s walk through a real-world example of how solving a problem on paper first can lead to a more efficient coding process. We’ll use the classic “Two Sum” problem:
Given an array of integers
nums
and an integertarget
, return indices of the two numbers such that they add up totarget
. You may assume that each input would have exactly one solution, and you may not use the same element twice.
Step 1: Understand the Problem
First, we write down the key points of the problem:
- Input: Array of integers, target sum
- Output: Indices of two numbers that add up to the target
- Constraints: One solution exists, can’t use same element twice
Step 2: Visualize with a Diagram
We can draw a simple array diagram to visualize the problem:
[2, 7, 11, 15] Target: 9 ^ ^ | | These two elements sum to 9
Step 3: Consider Approaches
We brainstorm and jot down possible approaches:
- Brute force: Check all pairs (O(n^2) time)
- Sort and use two pointers (O(n log n) time, but changes array order)
- Use a hash map for complement lookup (O(n) time, O(n) space)
Step 4: Choose and Outline the Best Approach
We decide the hash map approach is best for its O(n) time complexity. We outline the steps:
- Create an empty hash map
- Iterate through the array:
- Calculate the complement (target – current number)
- If complement is in the hash map, return current index and complement’s index
- Otherwise, add current number and its index to the hash map
- If no solution found, return an empty array or throw an exception
Step 5: Write Pseudocode
Based on our outline, we write pseudocode:
function twoSum(nums, target):
create empty hash map
for i from 0 to length of nums - 1:
complement = target - nums[i]
if complement exists in hash map:
return [hash map[complement], i]
add nums[i] and i to hash map
return [] // or throw exception
Step 6: Transition to Code
With our well-thought-out pseudocode, translating to actual code becomes straightforward:
function twoSum(nums: number[], target: number): number[] {
const complementMap = new Map<number, number>();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (complementMap.has(complement)) {
return [complementMap.get(complement)!, i];
}
complementMap.set(nums[i], i);
}
return []; // or throw new Error("No solution found");
}
By solving the problem on paper first, we were able to:
- Clearly understand the problem requirements
- Visualize the input and output
- Consider multiple approaches and choose the most efficient one
- Break down the solution into logical steps
- Write clean, well-structured code based on our pseudocode
This process not only leads to a correct solution but also demonstrates to interviewers your ability to think through problems systematically and communicate your thought process effectively.
Conclusion
Solving problems on paper before coding is a powerful technique that can significantly improve your performance in coding interviews. By clarifying your logic, preventing errors, and allowing for better visualization of complex problems, this approach sets you up for faster and more successful coding implementations.
Remember, the goal of most coding interviews is not just to arrive at a correct solution, but to demonstrate your problem-solving skills and thought process. By starting with pen and paper, you give yourself the best chance to showcase these abilities.
As you prepare for your next coding interview, make paper-first problem solving a key part of your practice routine. With time and consistent effort, you’ll find that this approach not only improves your interview performance but also enhances your overall coding skills and algorithmic thinking.
Happy problem solving, and best of luck in your coding interviews!