Why Solving Easy Problems Isn’t Preparing You for Hard Ones
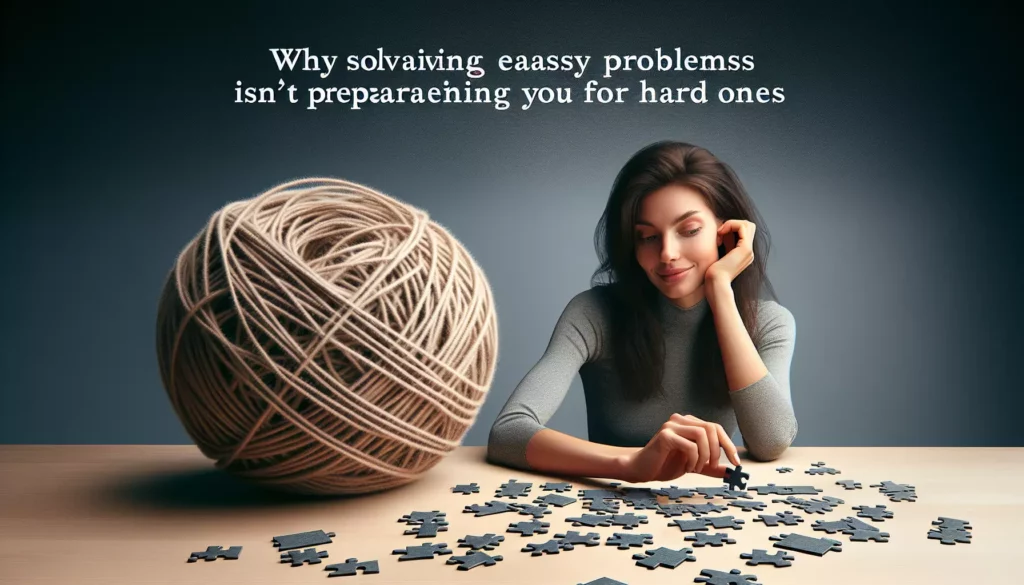
Many aspiring programmers and computer science students fall into a common trap in their learning journey. They spend countless hours solving beginner-level coding problems, gaining confidence with each solution, only to face crushing defeat when confronted with more complex challenges in technical interviews or real-world programming scenarios.
This disconnect between solving easy problems and tackling harder ones isn’t just frustrating—it’s a fundamental issue in how many approach coding education. In this article, we’ll explore why your current approach to problem-solving might be setting you up for failure, and more importantly, how to bridge the gap between simple exercises and complex algorithmic challenges.
The False Confidence of Easy Problems
There’s something deeply satisfying about solving coding problems. You read the requirements, write some code, submit it, and get that green checkmark or “All Tests Passed” notification. Each success delivers a small dopamine hit, reinforcing the behavior and making you feel like you’re making progress.
But here’s the uncomfortable truth: solving a hundred easy problems doesn’t necessarily prepare you for even one moderately difficult one.
Easy problems, by their nature, typically:
- Have straightforward, obvious approaches
- Require minimal algorithmic knowledge
- Can be solved with brute force methods
- Don’t test edge cases extensively
- Can be completed without optimization considerations
Consider this simple problem: “Write a function that returns the sum of two numbers.” The solution is trivial:
function sum(a, b) {
return a + b;
}
Now compare it to this more challenging problem: “Given an array of integers, find the length of the longest subsequence such that elements in the subsequence are consecutive integers, the consecutive numbers can be in any order.”
The gap between these two problems is enormous. The first requires basic syntax knowledge, while the second demands understanding of data structures, algorithmic thinking, and optimization techniques.
The Problem with Pattern Matching
When you solve many easy problems, you begin to develop a mental library of patterns. See a problem about reversing something? Use a stack or work from both ends. Need to count occurrences? Hash map. These patterns become your go-to tools.
This pattern-matching approach works well for easy and even some medium-difficulty problems. However, it falls apart when you encounter truly challenging problems that:
- Combine multiple patterns in non-obvious ways
- Require you to invent a new approach
- Need deep mathematical insights
- Involve complex optimizations
Let’s look at a concrete example. Many beginners learn to solve simple tree traversal problems:
function inorderTraversal(root) {
const result = [];
function traverse(node) {
if (!node) return;
traverse(node.left);
result.push(node.val);
traverse(node.right);
}
traverse(root);
return result;
}
But what happens when they face a problem like “Find the kth smallest element in a BST” or “Serialize and deserialize a binary tree”? The pattern they know helps but isn’t sufficient. They need to think deeper about the properties of trees, consider time and space complexity, and often combine multiple concepts.
The Skill Transfer Problem
Cognitive science research shows that skills don’t always transfer well between contexts, even when they seem related. This phenomenon, known as the “transfer problem,” is particularly relevant in programming education.
Just because you can implement bubble sort doesn’t mean you can develop a complex sorting algorithm for a specific use case. Just because you can write a recursive function to calculate Fibonacci numbers doesn’t mean you can apply recursion to solve a complex graph problem.
The reason? Hard problems require not just knowledge of individual concepts but the ability to:
- Recognize which concepts apply to a new problem
- Combine multiple concepts in creative ways
- Consider tradeoffs between different approaches
- Optimize for constraints that may not be obvious
These meta-skills don’t develop automatically from solving easy problems. They require deliberate practice with increasingly complex challenges.
The Gap Between Academic Knowledge and Application
Many programmers study data structures and algorithms in isolation. They learn how a hash table works, understand the principles of dynamic programming, and can recite the time complexity of various sorting algorithms.
But there’s a vast difference between understanding a concept and applying it to solve a novel problem. This is why many CS graduates struggle in technical interviews despite having good grades.
For example, many students learn the concept of dynamic programming through the classic “Fibonacci sequence” example:
function fibonacci(n) {
const dp = [0, 1];
for (let i = 2; i <= n; i++) {
dp[i] = dp[i-1] + dp[i-2];
}
return dp[n];
}
But when faced with a problem like “Maximum Sum Subarray” or “Longest Common Subsequence,” they struggle to recognize that dynamic programming is applicable, and even if they do, they have difficulty formulating the recurrence relation and implementing the solution.
The Interview Reality
If you’re preparing for technical interviews at top tech companies, the reality is even more stark. Companies like Google, Amazon, Facebook, Apple, and Netflix (often collectively referred to as FAANG) deliberately design their interview questions to test not just your knowledge, but your problem-solving process.
They want to see:
- How you approach unfamiliar problems
- Your ability to think algorithmically
- How you handle constraints and edge cases
- Your communication skills during the problem-solving process
- Your ability to optimize and improve initial solutions
Easy problems don’t exercise these skills effectively. They allow you to jump straight to coding without deeply engaging with the problem-solving process that interviewers want to observe.
Signs You’re Stuck in the Easy Problem Trap
How do you know if you’re falling into this trap? Watch for these warning signs:
- You solve problems quickly or give up quickly – Hard problems require persistence and exploration
- You rarely need to look up algorithmic concepts – Complex problems often require specific techniques
- Your solutions are usually brute force – Optimization is rarely needed for easy problems
- You don’t write test cases before coding – Easy problems don’t have many edge cases to consider
- You feel confident until you try a mock interview – The structured thinking required in interviews is different
If several of these apply to you, it’s time to reconsider your approach to learning and practice.
Bridging the Gap: How to Prepare for Hard Problems
Now that we understand the problem, let’s focus on solutions. How can you effectively prepare for challenging coding problems?
1. Embrace Deliberate Practice
Instead of solving many easy problems, focus on fewer, harder problems with deliberate practice:
- Time yourself: Set a realistic time limit (e.g., 45 minutes for a medium problem)
- Think before coding: Spend at least 10-15 minutes just analyzing the problem
- Write your approach: Document your thought process before writing code
- Consider multiple solutions: Don’t stop at your first working solution
- Review and reflect: After solving (or failing to solve), analyze what you learned
This approach mirrors what happens in actual technical interviews and builds the meta-skills needed for harder problems.
2. Study Problem-Solving Strategies, Not Just Solutions
When you study solutions to problems you couldn’t solve, don’t just memorize the code. Focus on:
- What insight made the solution possible?
- How could you have reached that insight yourself?
- What patterns or principles does this solution illustrate?
- How does this solution connect to other problems you’ve seen?
For example, many hard problems use the “sliding window” technique. Instead of memorizing specific implementations, understand the general pattern:
function slidingWindowExample(arr, k) {
let windowStart = 0;
let result = /* initial value */;
for (let windowEnd = 0; windowEnd < arr.length; windowEnd++) {
// Add element at windowEnd to your calculations
// Check if we need to slide the window
if (windowEnd >= k - 1) {
// Update result based on current window
// Remove element at windowStart from your calculations
windowStart++;
}
}
return result;
}
Understanding this pattern allows you to apply it to many different problems rather than just remembering specific solutions.
3. Build a Systematic Approach to Problem-Solving
Develop a consistent framework for approaching problems:
- Understand the problem completely: Restate it in your own words, ask clarifying questions, identify constraints
- Work through examples: Use simple examples first, then edge cases
- Identify patterns or related problems: Connect to problems you’ve solved before
- Brainstorm approaches: Consider multiple algorithms and data structures
- Analyze complexity: Evaluate time and space requirements
- Implement carefully: Write clean, organized code
- Test thoroughly: Check normal cases, edge cases, and error conditions
- Optimize iteratively: Look for improvements in your solution
This systematic approach forces you to engage deeply with each problem rather than jumping straight to coding.
4. Study Algorithm Design Paradigms
Instead of learning algorithms in isolation, focus on understanding the paradigms that generate them:
- Divide and Conquer: Breaking problems into smaller subproblems (e.g., merge sort, binary search)
- Dynamic Programming: Solving problems by combining solutions to overlapping subproblems
- Greedy Algorithms: Making locally optimal choices at each step
- Backtracking: Building solutions incrementally and abandoning partial solutions that fail
- Graph Algorithms: Techniques for traversing and analyzing graph structures
Understanding these paradigms helps you recognize which approach might work for a new problem, even if you haven’t seen something exactly like it before.
For example, many backtracking problems follow this general structure:
function backtrack(input, currentState, result) {
// Check if we have a complete solution
if (isComplete(currentState)) {
result.push(copyOf(currentState));
return;
}
// Try all possible next steps
for (const choice of getPossibleChoices(input, currentState)) {
// Make the choice
applyChoice(currentState, choice);
// Recurse to next decision level
backtrack(input, currentState, result);
// Undo the choice (backtrack)
undoChoice(currentState, choice);
}
}
Once you understand this pattern, you can apply it to problems like generating permutations, N-Queens, Sudoku solving, and many others.
5. Practice Explaining Your Thought Process
Hard problems require clear thinking, and nothing clarifies thought like having to explain it:
- Practice “thinking aloud” while solving problems
- Find a study partner for mock interviews
- Write detailed explanations of your solutions
- Teach concepts to others (even if just imaginary students)
This practice not only prepares you for the communication aspect of technical interviews but also helps you identify gaps in your understanding.
6. Strategically Increase Difficulty
Rather than jumping directly from easy to hard problems, create a deliberate progression:
- Start with easy problems to learn basic patterns
- Move to medium problems that combine these patterns
- Tackle variations of problems you’ve already solved
- Introduce constraints to familiar problems (e.g., optimize for space or handle larger inputs)
- Finally, approach genuinely hard problems that require novel insights
This gradual progression builds your problem-solving muscles without the discouragement of constantly failing at problems beyond your current abilities.
The Role of Conceptual Understanding
One reason easy problems don’t prepare you for harder ones is that they often don’t require deep conceptual understanding. You can solve many simple problems through trial and error or by following basic patterns without truly understanding the underlying principles.
For example, you might implement a basic binary search:
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
But do you understand why we use left <= right
instead of left < right
? Do you know why Math.floor((left + right) / 2)
can cause integer overflow in some languages and how to prevent it? Can you adapt binary search to find the first occurrence of a value, or to work on rotated sorted arrays?
These deeper questions reveal the difference between knowing how to implement an algorithm and truly understanding it. Hard problems require this deeper understanding.
Building a Knowledge Network
Experts in any field don’t just have isolated pieces of knowledge; they have richly connected knowledge networks that allow them to see relationships between concepts and apply them in novel situations.
To build your own knowledge network:
- Connect new problems to ones you’ve already solved: “This is like problem X, but with constraint Y”
- Categorize problems by underlying principles, not just by data structure
- Create your own problem taxonomy to organize what you learn
- Look for patterns across different problem domains
- Review periodically to strengthen connections between concepts
This networked understanding is what allows experts to quickly recognize the approach needed for new problems, even if they’ve never seen that exact problem before.
The Importance of Struggling
Perhaps counterintuitively, one of the most valuable aspects of hard problems is that they make you struggle. Cognitive science research shows that this “desirable difficulty” leads to better long-term learning.
When you solve an easy problem quickly, you get immediate gratification but little lasting benefit. When you struggle with a hard problem, even if you don’t solve it completely, you:
- Explore multiple approaches and learn why some don’t work
- Form deeper neural connections related to the problem domain
- Develop persistence and resilience
- Learn to manage frustration constructively
- Build the mental stamina needed for extended problem-solving sessions
This doesn’t mean you should constantly bash your head against problems far beyond your current abilities. But it does mean you should seek problems that challenge you enough to require significant mental effort.
Real-World Problem Complexity
While we’ve focused primarily on algorithmic problem-solving for interviews, it’s worth noting that real-world programming problems are often complex in different ways:
- They rarely have a single “correct” solution
- They involve balancing multiple competing constraints
- They require understanding existing codebases and systems
- They need collaboration with others who have different perspectives
- They evolve over time as requirements change
The meta-skills you develop by tackling hard algorithmic problems—systematic thinking, pattern recognition, breaking down complex problems, considering tradeoffs—transfer well to these real-world challenges, even if the specific algorithms don’t.
Tools and Resources for Tackling Harder Problems
As you transition from easy to harder problems, these resources can help:
Interactive Learning Platforms
- AlgoCademy: Offers AI-powered assistance that helps you progress from beginner to advanced problems with guided hints
- LeetCode: Has a large problem database with difficulty ratings and company tags
- HackerRank: Offers structured problem sets organized by domains
- CodeSignal: Provides industry-relevant challenges and assessments
Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Elements of Programming Interviews” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash
- “Algorithm Design Manual” by Steven Skiena
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
Communities
- Reddit communities like r/cscareerquestions and r/leetcode
- Discord servers focused on interview preparation
- Study groups with peers at similar skill levels
Structured Learning Approaches
- The “Blind 75” or “Neetcode 150” problem lists
- Systematic courses on algorithms and data structures
- Mock interview platforms like Pramp or interviewing.io
A Balanced Approach to Problem Difficulty
While this article has emphasized the limitations of focusing solely on easy problems, there is still value in solving problems at various difficulty levels. A balanced approach might include:
- Easy problems (20%): To learn new concepts and build confidence
- Medium problems (60%): To develop pattern recognition and combination skills
- Hard problems (20%): To stretch your abilities and develop novel problem-solving approaches
This distribution ensures you’re constantly challenged without becoming discouraged by constant failure.
Conclusion: Beyond Easy Problems
The path from solving easy coding problems to mastering complex algorithmic challenges isn’t a straight line. It requires not just accumulating knowledge but developing a different way of thinking about problems.
By embracing deliberate practice, studying problem-solving strategies rather than just solutions, building a systematic approach, understanding algorithmic paradigms, and practicing clear communication, you can bridge the gap between easy and hard problems.
Remember that the goal isn’t just to pass interviews or solve coding challenges—it’s to develop the kind of algorithmic thinking and problem-solving abilities that will serve you throughout your programming career.
The next time you feel the temptation to solve another easy problem for that quick dopamine hit, consider pushing yourself toward a more challenging problem instead. Your future self—especially when sitting in that important technical interview—will thank you for it.
Programming is ultimately about solving problems, and the most valuable problems are rarely the easy ones. By developing your ability to tackle difficult challenges systematically, you’ll set yourself apart as a developer and build skills that transcend any specific language, framework, or technology.