Why Skipping Fundamentals Is Hurting Your Programming Progress
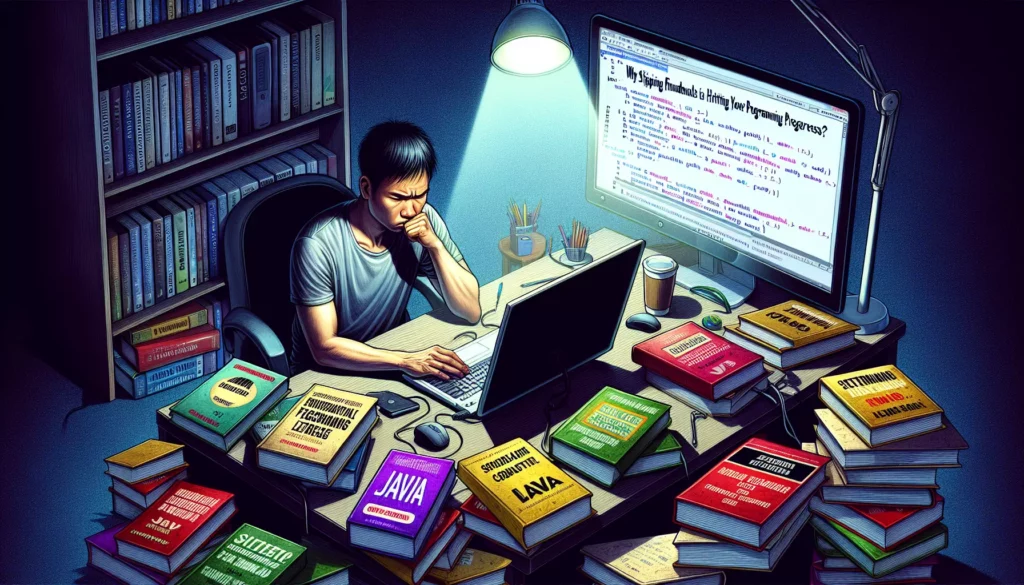
In the fast paced world of technology and programming, there’s an understandable desire to quickly reach advanced topics, build impressive applications, and land that dream job at a top tech company. This rush often leads aspiring developers to skip over the fundamentals, jumping straight into frameworks, libraries, and complex algorithms without building a solid foundation first.
At AlgoCademy, we’ve worked with thousands of learners on their coding journey, and we’ve consistently observed that those who invest time in mastering fundamentals progress faster and more sustainably in the long run. This article explores why skipping the basics is a costly mistake and how a strong foundation can accelerate your path to becoming an exceptional programmer.
Table of Contents
- Understanding the Rush: Why We Skip Fundamentals
- The Consequences of Skipping Fundamentals
- Which Fundamentals Matter Most
- Building Your Foundation: A Structured Approach
- Success Stories: Fundamentals Leading to Breakthroughs
- The Balancing Act: Fundamentals vs. Practical Experience
- How Fundamentals Prepare You for FAANG Interviews
- Conclusion: The Path Forward
Understanding the Rush: Why We Skip Fundamentals
Before we delve into the consequences of skipping fundamentals, it’s important to understand why it happens so frequently:
The Immediacy Bias
We live in an era of immediate gratification. Learning about variables, memory allocation, or time complexity doesn’t provide the same dopamine hit as building a visible project. The allure of creating something tangible often overshadows the importance of understanding what’s happening under the hood.
Career Pressure
With the tech job market becoming increasingly competitive, many feel pressured to quickly develop a portfolio of projects or learn the latest frameworks to stay relevant. This pressure can lead to cutting corners on foundational knowledge.
Tutorial Culture
The proliferation of “learn X in Y minutes” tutorials creates the illusion that programming can be mastered quickly. These resources, while valuable for specific tasks, often gloss over crucial underlying concepts.
Misconception About What Employers Want
There’s a common belief that employers only care about what you can build, not what you understand. While portfolio projects are important, companies (especially top tech firms) value deep understanding over surface level familiarity with tools.
The Consequences of Skipping Fundamentals
Bypassing fundamentals might seem like a shortcut, but it creates significant obstacles to long term growth:
The Fragile Knowledge Problem
Without understanding core principles, your knowledge becomes fragile. You might learn how to use a specific tool or solve a particular problem, but when faced with variations or entirely new challenges, you struggle to adapt.
Consider this scenario: A developer who learned React through tutorials but never fully grasped JavaScript fundamentals encounters a performance issue in their application. They can follow troubleshooting guides, but without understanding closures, the event loop, or memory management, they’re limited to surface level fixes rather than addressing root causes.
The Debugging Nightmare
When things go wrong (and they will), debugging becomes exponentially more difficult without fundamental knowledge. Developers who understand how memory works, how the language processes code, and basic algorithmic principles can trace problems to their source. Those without this foundation often resort to “trial and error” debugging, which is inefficient and frustrating.
The Learning Plateau
Many self taught programmers report hitting a “plateau” where they feel stuck, unable to advance to more complex projects or roles. This plateau often stems from gaps in fundamental knowledge that weren’t apparent when following tutorials but become critical barriers to advancement.
One of our AlgoCademy students described it this way: “I could build basic web apps following tutorials, but whenever I tried to create something from scratch, I’d get stuck on problems that seemed simple but I couldn’t solve. It was like hitting an invisible wall.”
Technical Debt in Learning
Just as skipping good practices in coding creates technical debt in software, skipping fundamentals creates “learning debt” that must eventually be repaid, often with interest. The time you save initially is often lost multiple times over when you have to go back and learn prerequisites you missed.
Which Fundamentals Matter Most
Not all fundamentals are created equal. Here are the core areas that provide the greatest return on investment for your learning time:
Computer Science Basics
- Data Structures: Understanding arrays, linked lists, trees, graphs, stacks, queues, and hash tables provides the vocabulary for solving complex problems.
- Algorithms: Knowing common algorithms and their applications gives you a toolkit for efficient problem solving.
- Time and Space Complexity: The ability to analyze how your code performs as inputs scale is crucial for writing efficient software.
This code example demonstrates the importance of understanding time complexity:
// Inefficient approach: O(n²) time complexity
function findDuplicates(array) {
let duplicates = [];
for (let i = 0; i < array.length; i++) {
for (let j = i + 1; j < array.length; j++) {
if (array[i] === array[j] && !duplicates.includes(array[i])) {
duplicates.push(array[i]);
}
}
}
return duplicates;
}
// Efficient approach: O(n) time complexity
function findDuplicatesEfficient(array) {
let seen = {};
let duplicates = [];
for (let item of array) {
if (seen[item]) {
if (seen[item] === 1) {
duplicates.push(item);
}
seen[item]++;
} else {
seen[item] = 1;
}
}
return duplicates;
}
Without understanding time complexity, you might not recognize why the second solution performs dramatically better with large inputs.
Programming Language Fundamentals
- Memory Management: Understanding how variables are stored and accessed (stack vs. heap, reference vs. value).
- Type Systems: Knowing how types work in your language of choice (static vs. dynamic, strong vs. weak).
- Language Specific Paradigms: Understanding the core paradigms of your language (object oriented programming, functional programming, etc.).
Here’s an example showing why understanding JavaScript’s reference vs. value behavior matters:
// Without understanding references vs. values
function addToCart(cart, item) {
cart.push(item);
return cart;
}
let shoppingCart = ['apple'];
let updatedCart = addToCart(shoppingCart, 'orange');
console.log(shoppingCart); // ['apple', 'orange'] - original cart was modified!
console.log(updatedCart); // ['apple', 'orange']
// With understanding of references
function addToCartImmutable(cart, item) {
return [...cart, item]; // Creates a new array instead of modifying the original
}
let shoppingCart2 = ['apple'];
let updatedCart2 = addToCartImmutable(shoppingCart2, 'orange');
console.log(shoppingCart2); // ['apple'] - original cart preserved
console.log(updatedCart2); // ['apple', 'orange']
System Design Fundamentals
- Client Server Architecture: Understanding how applications communicate over networks.
- Database Fundamentals: Knowing basic database concepts (relational vs. NoSQL, indexing, transactions).
- API Design: Understanding how to create and consume well designed APIs.
Development Process Fundamentals
- Version Control: Mastering Git beyond basic commands.
- Testing: Understanding different testing approaches and when to use them.
- Debugging: Developing systematic debugging skills rather than random tweaking.
Building Your Foundation: A Structured Approach
Now that we understand the importance of fundamentals, let’s explore how to build this foundation effectively:
Start With a Learning Roadmap
Rather than jumping between random tutorials, create a structured learning plan that covers fundamental concepts in a logical progression. At AlgoCademy, we recommend this sequence:
- Programming Basics: Variables, control structures, functions, and basic data types in your chosen language.
- Object Oriented or Functional Programming: Core paradigms that shape how you structure code.
- Data Structures: Starting with arrays and objects/dictionaries, then moving to more complex structures.
- Algorithms: Beginning with search and sort algorithms, then progressing to more specialized algorithms.
- System Interactions: How your code interacts with databases, APIs, and other systems.
Practice Deliberately
Passive learning (watching videos, reading books) should be balanced with active practice. For each concept you learn, implement it from scratch and experiment with variations. This “learning by doing” approach cements understanding in a way that passive consumption cannot.
For example, if you’re learning about linked lists, don’t just read about them. Implement one from scratch:
class Node {
constructor(value) {
this.value = value;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.tail = null;
this.length = 0;
}
append(value) {
const newNode = new Node(value);
if (!this.head) {
this.head = newNode;
this.tail = newNode;
} else {
this.tail.next = newNode;
this.tail = newNode;
}
this.length++;
return this;
}
prepend(value) {
const newNode = new Node(value);
if (!this.head) {
this.head = newNode;
this.tail = newNode;
} else {
newNode.next = this.head;
this.head = newNode;
}
this.length++;
return this;
}
// Add more methods: delete, find, etc.
}
Build Projects That Challenge You
While tutorial projects have their place, real learning happens when you build projects that push beyond your comfort zone. Choose projects that:
- Require you to implement fundamental data structures and algorithms
- Force you to think about performance and optimization
- Involve multiple components interacting (frontend, backend, database)
For example, instead of building yet another todo app, try building a text editor that implements data structures for efficient text manipulation, or a simple database that requires you to think about indexing and query optimization.
Study Source Code of Well Written Projects
One of the best ways to learn fundamentals is to read high quality code written by experienced developers. Find open source projects in your area of interest and study how they’re structured, how they handle edge cases, and how they implement fundamental concepts.
Embrace the “Learn in Public” Approach
Explaining concepts to others is one of the most effective ways to solidify your understanding. Start a blog, create videos, or participate in forums where you explain fundamental concepts. The process of articulating your knowledge exposes gaps in understanding and deepens your mastery.
Success Stories: Fundamentals Leading to Breakthroughs
Let’s look at some real examples from AlgoCademy students who experienced breakthroughs after investing in fundamentals:
Case Study: Sarah’s Journey to Google
Sarah had been coding for two years, primarily building React applications by following tutorials. She could create impressive looking projects but struggled with technical interviews. After failing several interviews at top companies, she decided to take a step back and focus on fundamentals.
She spent three months deeply studying data structures, algorithms, and JavaScript internals. The breakthrough came when she realized that many of the React patterns she had been using without understanding were applications of fundamental computer science concepts like trees and state management.
Her interview approach completely changed. Instead of memorizing solutions to common problems, she could reason through new challenges by applying fundamental principles. Six months after her fundamental review, she passed Google’s interview process.
“Looking back,” Sarah says, “I wish I had spent those first six months of my learning journey on pure fundamentals instead of jumping straight to React. I would have progressed much faster overall.”
Case Study: Miguel’s Performance Optimization
Miguel was a self taught developer who built a successful e commerce site for a client. As the site grew in popularity, it began experiencing performance issues. He spent weeks trying different optimizations suggested in blog posts, but the improvements were minimal.
After joining AlgoCademy, Miguel took a course on algorithmic thinking and database fundamentals. He suddenly realized that his database queries were performing full table scans because he hadn’t properly indexed his database. He also identified a search algorithm in his code that had O(n²) complexity when it could be O(n log n).
By applying these fundamental concepts, he reduced page load times by 80% and database query times by 95%. The client was thrilled, and Miguel credits his newfound focus on fundamentals:
“I used to think performance optimization was about finding magical tricks and hacks. Now I understand it’s about applying fundamental principles of how computers and algorithms work.”
The Balancing Act: Fundamentals vs. Practical Experience
While we’ve emphasized the importance of fundamentals, it’s crucial to find the right balance between theory and practice. Here’s how to strike that balance:
The 70/30 Approach
For beginners, we recommend spending about 70% of your learning time on fundamentals and 30% on practical application through projects. As you advance, this ratio might shift to 50/50 or even 30/70, but never abandon fundamental learning entirely.
Spiral Learning
Rather than trying to master all fundamentals before building anything, adopt a spiral learning approach: learn basic fundamentals, apply them in a project, return to more advanced fundamentals, apply those in a more complex project, and so on.
This approach might look like:
- Learn basic programming syntax and concepts
- Build a simple command line application
- Learn basic data structures (arrays, objects)
- Build a slightly more complex application using these structures
- Learn more advanced data structures (linked lists, trees)
- Incorporate these into your next project
Just In Time Learning
While having a foundation is crucial, you don’t need to learn everything before starting to build. When you encounter a problem in your project that requires a fundamental concept you haven’t learned yet, take that as an opportunity to dive deep into that concept.
This contextual learning often leads to better retention because you immediately see the practical application of the concept.
Recognize When You’re Hitting Fundamental Gaps
Learn to identify when you’re struggling due to missing fundamental knowledge versus when you simply need more practice with a particular technology. Signs you might be missing fundamentals include:
- Repeatedly encountering similar problems across different projects
- Finding yourself copying solutions without understanding why they work
- Struggling to debug issues without step by step guides
- Feeling limited to working with tutorials rather than creating original solutions
How Fundamentals Prepare You for FAANG Interviews
If your goal is to work at a major tech company like Facebook, Apple, Amazon, Netflix, or Google (often collectively referred to as FAANG), fundamentals become even more critical.
Technical Interviews Focus on Fundamentals
Top tech companies design their interview processes to test fundamental computer science knowledge rather than familiarity with specific frameworks or libraries. This is because:
- Fundamental knowledge transfers across projects and technologies
- Strong fundamentals predict a candidate’s ability to learn new technologies quickly
- Understanding core concepts correlates with ability to design efficient, scalable solutions
Common Interview Topics That Require Strong Fundamentals
- Data Structure Selection: Being able to choose the optimal data structure for a given problem.
- Algorithm Design and Analysis: Designing efficient algorithms and analyzing their time/space complexity.
- System Design: Understanding how to design scalable systems using fundamental architectural principles.
- Problem Solving Approach: Demonstrating a methodical approach to breaking down and solving complex problems.
Example: A Typical FAANG Interview Question
Consider this common interview question:
Design a data structure that supports the following operations in O(1) average time complexity: insert, remove, and getRandom (which returns a random element with equal probability).
This problem requires understanding:
- Hash tables (for O(1) insert and remove)
- Arrays (for O(1) random access)
- The tradeoffs between different data structures
- How to combine data structures to achieve desired performance characteristics
A solution might look like:
class RandomizedSet {
constructor() {
this.map = {}; // For O(1) lookup
this.values = []; // For O(1) random access
}
insert(val) {
if (val in this.map) return false;
this.map[val] = this.values.length;
this.values.push(val);
return true;
}
remove(val) {
if (!(val in this.map)) return false;
// Move the last element to the position of the element to delete
const lastElement = this.values[this.values.length - 1];
const indexToRemove = this.map[val];
this.values[indexToRemove] = lastElement;
this.map[lastElement] = indexToRemove;
// Remove the last element and the mapping
this.values.pop();
delete this.map[val];
return true;
}
getRandom() {
const randomIndex = Math.floor(Math.random() * this.values.length);
return this.values[randomIndex];
}
}
Without strong fundamentals in data structures, this kind of solution would be very difficult to derive during an interview.
Preparing for FAANG Interviews
If your goal is to work at a top tech company, here’s how to focus your fundamental learning:
- Master Core Data Structures: Arrays, linked lists, stacks, queues, hash tables, trees, graphs, and heaps. Understand their implementations, operations, and time complexities.
- Learn Key Algorithms: Sorting, searching, traversal, dynamic programming, greedy algorithms, and graph algorithms.
- Practice Problem Solving: Regularly solve algorithmic problems on platforms like LeetCode, HackerRank, or AlgoCademy. Focus on understanding the approach rather than memorizing solutions.
- Study System Design: Understand how to design scalable systems, including concepts like load balancing, caching, database sharding, and microservices.
- Develop a Problem Solving Framework: Create a systematic approach to tackling new problems that includes understanding requirements, considering edge cases, evaluating different approaches, and optimizing solutions.
Conclusion: The Path Forward
The journey to becoming an exceptional programmer isn’t about finding shortcuts; it’s about building a strong foundation that supports continuous growth. Skipping fundamentals might seem efficient in the short term, but it creates invisible barriers to your long term progress.
At AlgoCademy, we’ve seen time and again that students who invest in understanding core principles ultimately progress faster, interview better, and build more impressive projects than those who jump straight to frameworks and libraries.
Key Takeaways
- Rushing past fundamentals creates fragile knowledge that breaks down when faced with new challenges
- Core computer science concepts like data structures, algorithms, and time complexity are essential for solving complex problems efficiently
- Language specific fundamentals help you write better code and debug more effectively
- A structured learning approach that balances theory and practice leads to sustainable growth
- Top tech companies prioritize fundamental knowledge in their interview processes
Next Steps
If you recognize that gaps in your fundamental knowledge might be holding you back, here are some concrete next steps:
- Assess Your Foundation: Identify areas where your fundamental knowledge might be lacking.
- Create a Learning Plan: Develop a structured approach to filling those gaps while continuing to build projects.
- Find Quality Resources: Look for learning materials that explain not just how to do something, but why it works.
- Join a Learning Community: Surround yourself with others who value deep understanding over quick fixes.
- Be Patient: Remember that investing in fundamentals pays dividends over your entire career, even if progress seems slower initially.
Remember, the goal isn’t to spend years on theory before building anything practical. The ideal approach combines fundamental learning with practical application in a reinforcing cycle. Each project you build should incorporate and strengthen your fundamental knowledge, and each fundamental concept you learn should enhance your ability to build better projects.
By embracing this balanced approach, you’ll develop not just the ability to follow tutorials, but the deep understanding that allows you to create original solutions, optimize performance, and ultimately stand out in a competitive field.
Your programming journey is a marathon, not a sprint. By building on solid fundamentals, you’ll not only reach your destination faster in the long run, but you’ll have a much more fulfilling and empowering experience along the way.