Why Self-Taught Programmers Should Focus on Building Projects
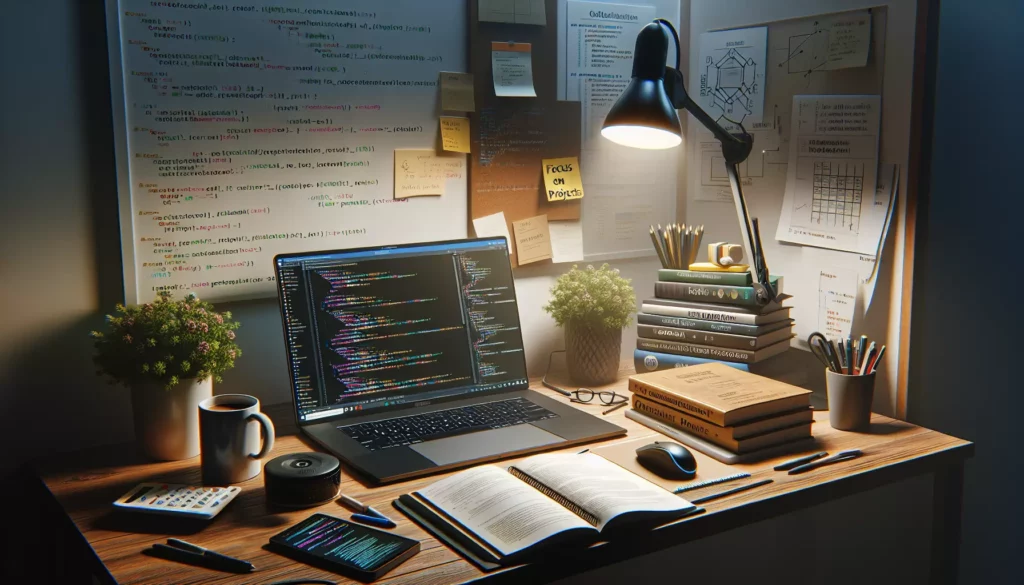
In the rapidly evolving world of technology, self-taught programmers are becoming increasingly common. With a wealth of online resources, tutorials, and coding bootcamps available, many aspiring developers are choosing to forgo traditional computer science degrees in favor of a more hands-on, self-directed approach to learning. While this path can be challenging, it also offers unique opportunities for growth and skill development. One of the most effective strategies for self-taught programmers to enhance their skills and boost their employability is to focus on building projects. In this comprehensive guide, we’ll explore why project-based learning is crucial for self-taught programmers and how it can accelerate their journey towards becoming proficient developers.
The Power of Project-Based Learning
Project-based learning is an educational approach that emphasizes hands-on experience and practical application of knowledge. For self-taught programmers, this method of learning offers several key benefits:
- Real-world application of skills
- Deeper understanding of concepts
- Portfolio development
- Problem-solving practice
- Motivation and engagement
Let’s dive deeper into each of these benefits and explore how they contribute to a self-taught programmer’s growth and success.
1. Real-World Application of Skills
One of the primary advantages of focusing on projects is the opportunity to apply theoretical knowledge in practical scenarios. While reading documentation and following tutorials can provide a foundation, actually building something from scratch allows you to encounter and overcome real-world challenges. This hands-on experience is invaluable for several reasons:
- It helps solidify your understanding of programming concepts
- You learn to integrate different technologies and frameworks
- You gain experience in project planning and management
- You develop troubleshooting and debugging skills
For example, let’s say you’ve been learning about web development and have covered HTML, CSS, and JavaScript. Instead of just completing coding exercises, you decide to build a personal website. This project will require you to:
<!-- Example of HTML structure for a personal website -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My Personal Website</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<header>
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#projects">Projects</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
</header>
<main>
<!-- Add your main content here -->
</main>
<footer>
<p>© 2023 Your Name. All rights reserved.</p>
</footer>
<script src="script.js"></script>
</body>
</html>
By working on this project, you’ll need to structure your HTML properly, style it with CSS, and add interactivity with JavaScript. You might also encounter challenges like making your site responsive or optimizing it for performance, which will push you to learn and apply new skills.
2. Deeper Understanding of Concepts
When you’re building a project, you’re not just following a set of instructions or memorizing syntax. You’re actively engaging with the programming concepts and figuring out how to apply them to solve specific problems. This deeper level of engagement leads to a more thorough understanding of the underlying principles.
For instance, if you’re learning about data structures and algorithms, you might decide to build a simple task management application. This project could involve:
- Implementing a priority queue to manage task importance
- Using sorting algorithms to order tasks by due date
- Applying search algorithms to find specific tasks
Here’s an example of how you might implement a simple priority queue in JavaScript:
class PriorityQueue {
constructor() {
this.items = [];
}
enqueue(element, priority) {
const queueElement = { element, priority };
let added = false;
for (let i = 0; i < this.items.length; i++) {
if (queueElement.priority < this.items[i].priority) {
this.items.splice(i, 0, queueElement);
added = true;
break;
}
}
if (!added) {
this.items.push(queueElement);
}
}
dequeue() {
if (this.isEmpty()) {
return "Queue is empty";
}
return this.items.shift().element;
}
isEmpty() {
return this.items.length === 0;
}
}
// Usage example
const taskQueue = new PriorityQueue();
taskQueue.enqueue("Complete project", 2);
taskQueue.enqueue("Study for exam", 1);
taskQueue.enqueue("Go grocery shopping", 3);
console.log(taskQueue.dequeue()); // Output: "Study for exam"
console.log(taskQueue.dequeue()); // Output: "Complete project"
console.log(taskQueue.dequeue()); // Output: "Go grocery shopping"
By implementing this data structure yourself and using it in a real application, you’ll gain a much deeper understanding of how priority queues work and when they’re useful, compared to simply reading about them or solving isolated coding problems.
3. Portfolio Development
For self-taught programmers, one of the biggest challenges when seeking employment is demonstrating their skills to potential employers. Without a formal degree or extensive work experience, your portfolio of projects becomes your most valuable asset. Each project you complete adds to your portfolio, showcasing your abilities and the range of technologies you’re familiar with.
A strong portfolio can include:
- Personal projects that demonstrate your creativity and problem-solving skills
- Contributions to open-source projects, showing your ability to work with existing codebases and collaborate with others
- Client projects or freelance work, if you have any
- Coding challenges or hackathon projects
When building your portfolio, it’s important to include a variety of projects that demonstrate different skills and technologies. For example:
- A full-stack web application using a popular framework like React or Vue.js for the frontend and Node.js with Express for the backend
- A mobile app developed with React Native or Flutter
- A data analysis project using Python and libraries like Pandas and Matplotlib
- A machine learning project that demonstrates your understanding of AI concepts
Here’s an example of how you might structure the README file for a project in your portfolio:
# Task Manager App
## Description
A full-stack web application for managing tasks and to-do lists. Users can create, update, delete, and prioritize tasks, as well as set due dates and reminders.
## Technologies Used
- Frontend: React.js, Redux for state management
- Backend: Node.js with Express
- Database: MongoDB
- Authentication: JWT (JSON Web Tokens)
## Features
- User registration and authentication
- CRUD operations for tasks
- Task prioritization and categorization
- Due date reminders
- Responsive design for mobile and desktop
## Installation and Setup
1. Clone the repository
2. Install dependencies: `npm install`
3. Set up environment variables (see `.env.example`)
4. Start the server: `npm run server`
5. Start the client: `npm run client`
## Demo
[Link to live demo]
## Screenshots
[Include screenshots of your application here]
## Future Improvements
- Implement task sharing and collaboration features
- Add a calendar view for better task visualization
- Integrate with external calendars (Google Calendar, iCal)
## Contact
[Your Name] - [Your Email]
Project Link: [GitHub repository URL]
By including detailed README files like this for each project in your portfolio, you demonstrate not only your coding skills but also your ability to document your work and communicate effectively about your projects.
4. Problem-Solving Practice
Programming is fundamentally about problem-solving, and building projects provides ample opportunities to hone these critical skills. When you’re working on a project, you’ll inevitably encounter challenges and roadblocks. Overcoming these obstacles helps you develop:
- Analytical thinking skills
- The ability to break down complex problems into smaller, manageable tasks
- Research skills as you search for solutions and best practices
- Debugging techniques to identify and fix issues in your code
For example, let’s say you’re building a weather application that fetches data from an API. You might encounter issues like:
- How to handle API rate limiting
- Dealing with network errors or timeout issues
- Parsing and displaying complex JSON data
- Implementing caching to improve performance
Here’s an example of how you might implement error handling and caching when fetching data from a weather API:
const axios = require('axios');
const NodeCache = require('node-cache');
const cache = new NodeCache({ stdTTL: 600 }); // Cache for 10 minutes
async function getWeatherData(city) {
const cacheKey = `weather_${city}`;
const cachedData = cache.get(cacheKey);
if (cachedData) {
console.log('Returning cached data');
return cachedData;
}
try {
const response = await axios.get(`https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=YOUR_API_KEY`);
const weatherData = response.data;
cache.set(cacheKey, weatherData);
return weatherData;
} catch (error) {
if (error.response) {
// The request was made and the server responded with a status code
// that falls out of the range of 2xx
console.error(`Error ${error.response.status}: ${error.response.data.message}`);
} else if (error.request) {
// The request was made but no response was received
console.error('No response received from the server');
} else {
// Something happened in setting up the request that triggered an Error
console.error('Error setting up the request:', error.message);
}
throw error;
}
}
// Usage
getWeatherData('London')
.then(data => console.log(data))
.catch(error => console.error('Failed to fetch weather data'));
By working through these challenges, you’ll develop problem-solving skills that are applicable across various programming tasks and projects.
5. Motivation and Engagement
Learning to code can be a long and sometimes frustrating journey, especially for self-taught programmers who may not have the structure and support of a formal educational program. Building projects can help maintain motivation and engagement in several ways:
- Sense of accomplishment: Completing a project, even a small one, provides a tangible result of your efforts and can boost your confidence.
- Personal investment: Working on projects that align with your interests or solve problems you care about can make the learning process more enjoyable and meaningful.
- Visible progress: As you build more complex projects over time, you can clearly see your skills improving, which can be very motivating.
- Community engagement: Sharing your projects with others, whether through GitHub, social media, or coding forums, can lead to feedback, collaboration opportunities, and a sense of belonging in the developer community.
To stay motivated, consider setting up a project roadmap that gradually increases in complexity. For example:
- Build a simple static website
- Create a dynamic web application with a backend
- Develop a mobile app version of your web application
- Implement advanced features like real-time updates or machine learning components
- Scale your application to handle high traffic and deploy it to a cloud platform
Strategies for Effective Project-Based Learning
Now that we’ve explored the benefits of focusing on projects, let’s discuss some strategies to make the most of this approach:
1. Start Small and Iterate
Don’t try to build complex applications right away. Start with small, manageable projects and gradually increase the complexity as you gain confidence and skills. This approach allows you to:
- Build a foundation of basic skills
- Experience quick wins, which can boost motivation
- Learn the full development cycle on a smaller scale
For example, if you’re learning web development, your project progression might look like this:
- Build a simple static HTML and CSS website
- Add JavaScript to make the website interactive
- Create a single-page application using a framework like React
- Implement a backend API using Node.js and Express
- Add a database to store and retrieve data
- Implement user authentication and authorization
2. Choose Projects That Align with Your Goals
Select projects that are relevant to the type of programming you want to do professionally. This ensures that the skills you’re developing are directly applicable to your career goals. For instance:
- If you’re interested in frontend development, focus on projects that involve building user interfaces and working with modern JavaScript frameworks.
- For backend development, concentrate on projects that involve server-side programming, databases, and API development.
- If you’re aiming for a full-stack role, try to build projects that encompass both frontend and backend technologies.
3. Embrace Version Control
Learn and use Git for version control from the beginning. This not only helps you manage your project’s codebase but also prepares you for collaborative development in professional settings. Here are some Git commands you should become familiar with:
# Initialize a new Git repository
git init
# Add files to staging area
git add .
# Commit changes
git commit -m "Your commit message"
# Create and switch to a new branch
git checkout -b new-feature
# Merge changes from one branch to another
git merge new-feature
# Push changes to a remote repository
git push origin main
4. Document Your Process
As you work on projects, document your thought process, challenges faced, and solutions implemented. This can be done through:
- Detailed README files for each project
- Inline code comments explaining complex logic
- Blog posts or articles about your project experiences
- Video tutorials or live coding sessions
Documentation not only helps others understand your work but also reinforces your own learning and improves your communication skills.
5. Seek Feedback and Collaborate
Don’t work in isolation. Seek feedback on your projects from more experienced developers or peers. This can be done through:
- Code reviews on GitHub
- Participating in coding forums or communities
- Attending local meetups or hackathons
- Contributing to open-source projects
Collaboration exposes you to different coding styles, best practices, and new technologies, accelerating your learning process.
6. Continuously Learn and Adapt
The tech industry evolves rapidly, and it’s crucial to stay updated with new technologies and best practices. As you work on projects:
- Regularly explore new tools and frameworks
- Refactor old projects with new techniques you’ve learned
- Challenge yourself to use a new technology or approach in each project
- Follow industry blogs, podcasts, and attend webinars to stay informed about trends
Overcoming Common Challenges
While focusing on projects is highly beneficial, self-taught programmers may face some challenges along the way. Here are some common issues and strategies to overcome them:
1. Imposter Syndrome
Many self-taught programmers struggle with feelings of inadequacy or self-doubt, especially when comparing themselves to formally educated peers.
Solution: Remember that even experienced developers face challenges and have to continuously learn. Focus on your progress and the projects you’ve completed rather than comparing yourself to others. Celebrate your achievements, no matter how small they may seem.
2. Project Scope Creep
It’s easy to get carried away and try to add too many features to a project, leading to overwhelm and potentially abandoned projects.
Solution: Practice defining clear project scopes and creating minimum viable products (MVPs). Use project management techniques like user stories and sprints to keep your projects focused and manageable.
3. Lack of Structure
Without the structure of a formal education program, self-taught programmers may struggle to organize their learning and project work effectively.
Solution: Create a learning plan with specific goals and deadlines. Use tools like Trello or Asana to manage your projects and track your progress. Consider following a curriculum from online learning platforms to provide some structure to your studies.
4. Difficulty in Choosing Technologies
With the vast array of programming languages, frameworks, and tools available, it can be challenging to decide what to focus on.
Solution: Research job markets in your area or in the field you’re interested in to identify in-demand technologies. Start with foundational languages and concepts before moving on to specific frameworks. Don’t try to learn everything at once; focus on mastering one stack at a time.
5. Balancing Learning and Building
It can be tempting to either spend too much time learning without applying knowledge or jumping into projects without sufficient foundational knowledge.
Solution: Adopt a balanced approach where you alternate between learning new concepts and applying them in projects. Use the “learn, build, teach” cycle: learn a new concept, build something with it, then try to explain or teach it to someone else to solidify your understanding.
Conclusion
For self-taught programmers, focusing on building projects is an invaluable approach to learning and skill development. It provides real-world experience, deepens understanding of programming concepts, helps build a strong portfolio, enhances problem-solving skills, and maintains motivation throughout the learning journey.
By starting small, choosing relevant projects, embracing best practices like version control and documentation, seeking feedback, and continuously adapting to new technologies, self-taught programmers can effectively bridge the gap between theoretical knowledge and practical skills.
Remember, the path of a self-taught programmer may be challenging, but it’s also incredibly rewarding. Each project you complete is a testament to your growth and dedication. As you continue to build and learn, you’ll not only develop technical skills but also the resilience, creativity, and problem-solving abilities that are hallmarks of successful developers.
So, roll up your sleeves, fire up your code editor, and start building. Your next project could be the key that unlocks new opportunities and takes your programming career to new heights. Happy coding!